Dynamic Webscraping with Selenium#
In the last workshop, we saw how to use BeautifulSoup
to scrape data from a website and read that data into a powerful data structure called a pandas
DataFrame
. In this notebook, we’ll do something very similar again. We’ll be taking a website url, passing it through 3rd party software and extracting useful information that we can use to populate a DataFrame. This time, however, we will be scraping a dynamic website, that is a website whose HTML code is generated by an application.
Our Task in this notebook#
We are going to scrape the headlines from today’s issue of the New York Times. Then, we’ll put this data in a DataFrame
and save it locally as a csv for later use.
A note on copyright: all Tufts logins come with the New York Times, so be sure to log into your Tufts account before you continue. Please find instructions on doing so here.
Goals:#
Understand what a dynamic website is and how it is different from a static website
Install
Selenium
along with it’s associated dependenciesNavigate the content of the site both in
Selenium
andBeautifulSoup
Get more experience with generating DataFrames
What is a dynamic website#
Let’s delve a bit deeper into what a dynamic website is and why we can’t just use BeautifulSoup
to parse it as we can with static websites. While a static webpage would require a manual update before content on the site can change, a dynamic website takes advantage of client and server-side scripting to be more adaptable to a user’s needs.
Client-side scripting: code that is executed by the user’s browser, generally using JavaScript. This scripting renders changes to the site when the user interacts with it. This can be anything from selecting a choice in a drop down menu to full fledged games like Wordle. This type of scripting is also common in many static sites.
Server-side scripting: code that is executed by the server before sending content to the user’s browser. This code can be written in a wide varity of languages like Ruby (
RubyOnRails
), JavaScript (VueJS
,NodeJS
) and Python (Django
,Flask
). This code generally gets inputs from querying a database associated with the site and outputs HTML code from a template. This way, programmers can update elements in their sites without having to rewrite large sections of it. But, it also means that the HTML is not yet generated when we do a get request.
Let’s look at what this means for us in Python code below.
## what we did in part i of the art of webscraping. if this isn't making any sense, check out [LINK TO PART I]
from bs4 import BeautifulSoup
import requests
soup = BeautifulSoup(requests.get('https://www.nytimes.com/').text)
soup
<!DOCTYPE html>
<html class="nytapp-vi-homepage" lang="en" xmlns:og="http://opengraphprotocol.org/schema/">
<head>
<meta charset="utf-8"/>
<title data-rh="true">The New York Times - Breaking News, US News, World News and Videos</title>
<meta content="Live news, investigations, opinion, photos and video by the journalists of The New York Times from more than 150 countries around the world. Subscribe for coverage of U.S. and international news, politics, business, technology, science, health, arts, sports and more." data-rh="true" name="description"/><meta content="https://www.nytimes.com" data-rh="true" property="og:url"/><meta content="website" data-rh="true" property="og:type"/><meta content="The New York Times - Breaking News, US News, World News and Videos" data-rh="true" property="og:title"/><meta content="Live news, investigations, opinion, photos and video by the journalists of The New York Times from more than 150 countries around the world. Subscribe for coverage of U.S. and international news, politics, business, technology, science, health, arts, sports and more." data-rh="true" property="og:description"/><meta content="https://static01.nyt.com/newsgraphics/images/icons/defaultPromoCrop.png" data-rh="true" property="og:image"/> <link data-rh="true" href="https://www.nytimes.com" rel="canonical"/><link data-rh="true" href="https://www.nytimes.com" hreflang="x-default" rel="alternate"/><link data-rh="true" href="https://www.nytimes.com" hreflang="en-US" rel="alternate"/><link data-rh="true" href="https://www.nytimes.com/international/" hreflang="en" rel="alternate"/><link data-rh="true" href="https://www.nytimes.com/international/" hreflang="en-GB" rel="alternate"/><link data-rh="true" href="https://www.nytimes.com/international/" hreflang="en-AU" rel="alternate"/><link data-rh="true" href="https://www.nytimes.com/ca/" hreflang="en-CA" rel="alternate"/><link data-rh="true" href="https://www.nytimes.com/es/" hreflang="es" rel="alternate"/><link data-rh="true" href="https://cn.nytimes.com" hreflang="zh-Hans" rel="alternate"/><link data-rh="true" href="https://cn.nytimes.com/zh-hant/" hreflang="zh-Hant" rel="alternate"/><link data-rh="true" href="https://rss.nytimes.com/services/xml/rss/nyt/HomePage.xml" rel="alternate" title="RSS" type="application/rss+xml"/> <script data-rh="true" type="application/ld+json">{"@context":"https://schema.org","@type":"WebSite","image":[{"@context":"https://schema.org","@type":"ImageObject","url":"https://static01.nyt.com/vi-assets/images/share/1200x675_nameplate.png","height":675,"width":1200,"contentUrl":"https://static01.nyt.com/vi-assets/images/share/1200x675_nameplate.png","creditText":"The New York Times"},{"@context":"https://schema.org","@type":"ImageObject","url":"https://static01.nyt.com/vi-assets/images/share/1200x900_t.png","height":900,"width":1200,"contentUrl":"https://static01.nyt.com/vi-assets/images/share/1200x900_t.png","creditText":"The New York Times"},{"@context":"https://schema.org","@type":"ImageObject","url":"https://static01.nyt.com/vi-assets/images/share/1200x1200_t.png","height":1200,"width":1200,"contentUrl":"https://static01.nyt.com/vi-assets/images/share/1200x1200_t.png","creditText":"The New York Times"}],"url":"https://www.nytimes.com","name":"The New York Times","alternateName":["NYT","new york times","nytimes","ny times"],"potentialAction":{"@type":"SearchAction","target":{"@type":"EntryPoint","urlTemplate":"https://www.nytimes.com/search?query={search_term_string}"},"query-input":"required name=search_term_string"},"mainEntity":{"@context":"https://schema.org","@type":"ItemList","itemListElement":[{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/us/politics/musk-federal-bureaucracy-takeover.html","position":1},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/us/politics/musk-doge-timeline-takeaways.html","position":2},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/podcasts/the-headlines/iowa-trans-rights-zelensky-trump.html","position":3},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/live/2025/02/28/us/trump-news","position":4},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/world/europe/trump-starmer-king-charles.html","position":5},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/us/politics/starmer-ukraine-trump.html","position":6},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/podcasts/the-daily/trump-minerals-ukraine-republican-budget.html","position":7},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/business/economy/trump-tariff-goals-contradictions.html","position":8},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/business/dealbook/inflation-trump-tariffs.html","position":9},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/us/politics/trump-federal-worker-layoffs.html","position":10},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/us/politics/federal-layoffs-trump-opm.html","position":11},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/world/americas/argentina-crypto-scandal-president.html","position":12},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/movies/gene-hackman-death-new-mexico.html","position":13},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/us/gene-hackman-death-updates.html","position":14},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/interactive/2025/02/27/movies/hackman-affidavit.html","position":15},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/movies/gene-hackman-unforgiven-bonnie-clyde.html","position":16},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/world/asia/china-military-drills-pacific.html","position":17},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/world/middleeast/west-bank-jenin-palestinian-authority-israel.html","position":18},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/world/middleeast/syria-ramadan-economy-cash-shortage.html","position":19},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/us/iowa-transgender-civil-rights-bill.html","position":20},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/us/politics/trans-troops-pentagon-figures.html","position":21},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/interactive/2025/02/27/us/politics/medicaid-enrollment.html","position":22},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/movies/best-picture-nominees-oscars-conclave-wicked.html","position":23},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/arts/dance/zoe-saldana-emilia-perez-dance.html","position":24},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/world/americas/emilia-perez-mexico-oscars.html","position":25},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/nyregion/adams-corruption-new-charge.html","position":26},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/nyregion/andrew-cuomo-new-york-mayor.html","position":27},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/business/shopping-addiction-no-buy-2025.html","position":28},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/opinion/donald-trump-birthright-citizenship.html","position":29},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/opinion/andrew-tate-donald-trump.html","position":30},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/opinion/government-great-progressive-abundance.html","position":31},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/opinion/elon-musk-south-africa.html","position":32},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/opinion/texas-measles-vaccine.html","position":33},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/opinion/medicaid-republicans.html","position":34},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/opinion/matter-of-opinion-final-episode.html","position":35},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/live/2025/02/25/opinion/thepoint/barnard-college-sit-in-palestinian-israel-protest","position":36},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/opinion/trump-hegseth-pentagon.html","position":37},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/opinion/trump-republicans-masculinity-gender-traditional.html","position":38},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/opinion/free-speech-trump-maga.html","position":39},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/opinion/horse-racing-government-subsidies.html","position":40},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/arts/television/stephen-colbert-trump-voters-remorse.html","position":41},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/us/politics/mexico-cartel-sheinbaum-trump.html","position":42},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/world/canada/canada-us-trump-tariffs-reactions.html","position":43},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/business/jeffrey-epstein-files-pam-bondi.html","position":44},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/28/nyregion/citigroup-81-trillion-error.html","position":45},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/2025/02/27/style/sharon-van-etten-album.html","position":46},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/games/wordle/index.html","position":47},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/games/connections?GAMES_connectionsRollout_1130=1_ConnectionsV2","position":48},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/games/strands","position":49},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/puzzles/spelling-bee","position":50},{"@context":"https://schema.org","@type":"ListItem","url":"https://www.nytimes.com/crosswords","position":51},{"@context":"https://schema.org","@type":"ListItem","url":"http://www.nytimes.com/crosswords/game/mini","position":52}],"numberOfItems":52},"publisher":{"@id":"https://www.nytimes.com/#publisher","name":"The New York Times"}}</script><script data-rh="true" type="application/ld+json">{"@context":"https://schema.org","@type":"NewsMediaOrganization","name":"The New York Times","logo":{"@context":"https://schema.org","@type":"ImageObject","url":"https://static01.nyt.com/images/icons/t_logo_291_black.png","height":291,"width":291,"contentUrl":"https://static01.nyt.com/images/icons/t_logo_291_black.png","creditText":"The New York Times"},"url":"https://www.nytimes.com/","@id":"https://www.nytimes.com/#publisher","publishingPrinciples":"https://www.nytimes.com/editorial-standards/ethical-journalism.html","diversityPolicy":"https://www.nytco.com/company/diversity-and-inclusion/","ethicsPolicy":"https://www.nytco.com/company/standards-ethics/","masthead":"https://www.nytimes.com/interactive/2023/01/28/admin/the-new-york-times-masthead.html","foundingDate":"1851-09-18","sameAs":["https://www.facebook.com/nytimes/","https://x.com/nytimes","https://www.instagram.com/nytimes/","https://www.youtube.com/user/TheNewYorkTimes","https://www.linkedin.com/company/the-new-york-times","https://www.wikidata.org/wiki/Q9684","https://en.wikipedia.org/wiki/The_New_York_Times"],"alternateName":["NYT","new york times","nytimes","ny times"],"subOrganization":[{"@type":"Organization","name":"NYT Cooking","url":"https://cooking.nytimes.com/","sameAs":["https://www.facebook.com/nytcooking/","https://www.facebook.com/groups/nytcooks/","https://www.instagram.com/nytcooking/","https://www.youtube.com/channel/UC1rIOwTqDuWkFj87HZYRFOg","https://x.com/nytfood"],"logo":[{"url":"https://static01.nyt.com/applications/cooking/37f1abc/assets/SiteLogoRed.svg","height":31,"width":168,"@type":"ImageObject"},{"url":"https://static01.nyt.com/applications/cooking/37f1abc/assets/SiteLogoBlack.svg","height":24,"width":131,"@type":"ImageObject"}]},{"@type":"Organization","name":"Wirecutter","url":"https://www.nytimes.com/wirecutter/","sameAs":["https://www.facebook.com/thewirecutter/","https://www.instagram.com/wirecutter/","https://x.com/wirecutter/","https://www.linkedin.com/company/the-wirecutter/","https://www.youtube.com/wirecutter"],"logo":{"@type":"ImageObject","url":"https://cdn.thewirecutter.com/wp-content/uploads/2020/05/nytimes-wirecutter-logo.png","width":266,"height":34}},{"@type":"Organization","name":"The Athletic","url":"https://www.nytimes.com/athletic/","sameAs":["https://www.facebook.com/TheAthletic","https://instagram.com/TheAthleticHQ","https://x.com/TheAthletic","https://www.linkedin.com/company/the-athletic-media-company/","https://www.youtube.com/TheAthletic"],"logo":{"@type":"ImageObject","url":"https://www.nytimes.com/athletic/app/themes/athletic/assets/img/open-graph-asset.png","width":266,"height":150}}]}</script>
<meta content="The New York Times" data-rh="true" name="application-name"/><meta content="https://www.nytimes.com" data-rh="true" name="msapplication-starturl"/><meta content="name=Search;action-uri=https://www.nytimes.com/search/?src=iepin;icon-uri=https://static01.nyt.com/images/icons/search.ico" data-rh="true" name="msapplication-task"/><meta content="name=Most Popular;action-uri=https://www.nytimes.com/trending/?src=iepin;icon-uri=https://static01.nyt.com/images/icons/mostpopular.ico" data-rh="true" name="msapplication-task"/><meta content="name=Video;action-uri=https://www.nytimes.com/video?src=iepin;icon-uri=https://static01.nyt.com/images/icons/video.ico" data-rh="true" name="msapplication-task"/><meta content="name=Homepage;action-uri=https://www.nytimes.com?src=iepin&adxnnl=1;icon-uri=https://static01.nyt.com/images/icons/homepage.ico" data-rh="true" name="msapplication-task"/><meta content="nyt://programmingnode/1999c500-b740-5ba9-b2c1-57ff6b183315" data-rh="true" name="nyt_uri"/><meta content="Homepage" data-rh="true" name="CG"/><meta content="" data-rh="true" name="SCG"/><meta content="Homepage" data-rh="true" name="PT"/><meta content="" data-rh="true" name="PST"/><meta content="news, live updates, latest news, breaking news, local news, current events, top stories, livestream, live video, world news, us news" data-rh="true" name="keywords"/>
<meta content="width=device-width, initial-scale=1" name="viewport"/>
<meta content="9869919170" property="fb:app_id"/>
<meta content="@nytimes" name="twitter:site"/>
<meta content="A0121HXPPTQ" name="slack-app-id"/>
<script>(function Qn(e){if(new URL(window.location).searchParams.get("sentryOverride")||Math.floor(100*Math.random())<=e){var t=['<script src="https://js.sentry-cdn.com/7bc8bccf5c254286a99b11c68f6bf4ce.min.js" crossorigin="anonymous">',"<","/script>"].join("");document.write(t)}})(1);</script><script>(function Xn(e){var t=e.release,n=e.env,a=e.routeName,i=window.vi&&window.vi.webviewEnvironment||{},r=i.isPreloaded,o=void 0!==r&&r,l="IOS"===i.deviceType;if(window.Sentry){var s=function(){window.Sentry.init({maxBreadcrumbs:c,release:t,environment:n,allowUrls:u,ignoreErrors:d,beforeSend:function(e,t){var n=t.originalException;return n&&n.stack&&n.stack.includes("datadog-rum")?null:e},initialScope:function(e){e.setTags({"nyt.route":a});var t=/nyt-a=(.*?)(;|$)/.exec(document.cookie);null!==t&&e.setUser({id:t[1]});try{if(o){var n,i,r=window.asset||{},s=r.eventId,c=void 0===s?"":s,d=r.uri,u=void 0===d?"":d,f=r.url,p=void 0===f?"":f,m=r.sourceType,g=void 0===m?"":m,h=r.sourceId,v=void 0===h?"":h;c&&e.setTag("eventId",c),u&&e.setTag("uri",u),p&&e.setTag("url",p),g&&e.setTag("sourceType",g),v&&e.setTag("sourceId",v),e.setExtra("visibilityState",document.visibilityState),null!==(n=window)&&void 0!==n&&null!==(i=n.performance)&&void 0!==i&&i.timeOrigin&&e.setExtra("timeOrigin",window.performance.timeOrigin),e.setTag("type","preloaded-webview")}else e.setTag("type","browser");var b=function(){var t=window.config||{},n=t.AppVersion,a=void 0===n?"":n,i=t.ConnectionStatus,r=void 0===i?3:i,o=t.LoggedIn,l=void 0!==o&&o,s=t.Subscriber,c=void 0!==s&&s;null==e||e.setTag("AppVersion",a),null==e||e.setTag("ConnectionStatus",r),null==e||e.setTag("LoggedIn",l),null==e||e.setTag("Subscriber",c)};l?window.addEventListener("initWebview:ios",b):b()}catch(e){console.error(e)}return e},replaysSessionSampleRate:0,replaysOnErrorSampleRate:.01,integrations:[window.Sentry.browserTracingIntegration({enableInp:!0,instrumentNavigation:!1,instrumentPageLoad:!1})]});var e=window.Sentry.getClient(),i={};i[window.Sentry.SEMANTIC_ATTRIBUTE_SENTRY_SOURCE]="url",window.Sentry.startBrowserTracingPageLoadSpan(e,{name:a,attributes:i})},c=70,d=["2mdn","ads-us","amazon-adsystem","amp4ads","ampproject","bk_addPageCtx","boomerang","BOOMR","cb is not a function","chartbeat","google_tag_manager","gsi","gtm","prebid","pubads","scorecardresearch","setConfig is not a function","webkitExitFullScreen","yimg","reading 'campaign'","Object.fromEntries is not a function"];o&&(d=d.concat(["Failed to fetch","Load failed","Something went wrong","Unexpected EOF","The request is not allowed by the user agent","scrollIntoView","No weather-bot homepage data available","UnhandledRejection: Non-Error promise rejection captured with value: false","VHS is currently showing this error message on-screen.","useMessageSelection","messageComponentLibrary"]));var u=[/^https?:\/\/(vi-pr-.*|www|vi|local|alpha|alpha-preview)\.(stg\.|dev\.)?(nytimes\.com|nyt\.net)/];window.Sentry.onLoad(s),window.__isUnitTestEnv&&(window.__testSentryOnload=s)}})({"release":"a33e3b3f2d9932550881a21bdebe3aaec6164b65","env":"prd","routeName":"homepage","allowUrls":[{}]});</script>
<script>
(function qn(){var e,t,n,a,i;e=window,t=document,n="script",a="https://www.datadoghq-browser-agent.com/us1/v5/datadog-rum.js",e=e[i="DD_RUM"]=e[i]||{q:[],onReady:function(t){e.q.push(t)}},(i=t.createElement(n)).async=1,i.src=a,(a=t.getElementsByTagName(n)[0]).parentNode.insertBefore(i,a)})();
(function Yn(e){var t=e.service,n=e.env,a=e.version,i=e.sessionSampleRate,r=e.deploymentId,o=e.routeName,l=e.tenant;if(window.DD_RUM){var s=function(){var e={clientToken:"pube5bf68ea68edb54c35106f34e32ff07c",applicationId:"7d0602a0-8ef8-4d39-985b-c3188887e5b3",site:"datadoghq.com",service:"".concat(t,"-client"),env:n,version:a,sessionSampleRate:parseInt(i,10),sessionReplaySampleRate:v,trackUserInteractions:!0,trackResources:!0,trackLongTasks:!0,trackViewsManually:!0,defaultPrivacyLevel:"mask-user-input",allowedTracingUrls:m,traceSampleRate:20,useCrossSiteSessionCookie:!0,useSecureSessionCookie:!0};u&&(e=Object.assign(e,h)),window.DD_RUM.init(e);var s="web";u&&(s=window.config&&window.config.OS&&"ANDROID"===window.config.OS.toUpperCase()?"android":"ios");var c={billing:{environment:n,deployment:{id:r}},dvsp:{tenant:l},route:{name:o},webview:p,preloaded:u,appType:s};u&&(c=Object.assign(c,g)),window.DD_RUM.setGlobalContextProperty("nyt",c),window.DD_RUM.startView({name:o});var d=/nyt-a=(.*?)(;|$)/.exec(document.cookie);if(null!==d&&window.DD_RUM.setUser({id:d[1]}),u){var f=(window.asset||{}).url,b=void 0===f?"":f;window.DD_RUM.setGlobalContextProperty("url",b);var y=window.getNativeBridgeCookie("nyt-a");y&&window.DD_RUM.setUser({id:y})}},c=window.vi&&window.vi.webviewEnvironment||{},d=c.isPreloaded,u=void 0!==d&&d,f=c.isInWebview,p=void 0!==f&&f,m=[/https:\/\/samizdat-graphql.*\.nytimes\.com/],g={},h={},v=100;u&&(m=[/[https|http|nytresource]:\/\/.*\.nytimes\.com/,/[https|http|nytresource]:\/\/.*\.nyt\.com/],g={team:"web-platforms"},v=0,h={trackFrustrations:!0,allowFallbackToLocalStorage:!0}),window.DD_RUM.onReady(s),window.__isUnitTestEnv&&(window.__testDataDogOnReady=s)}})({"service":"vi","env":"prd","version":"vi-newsreader@v6788-a33e3b3","sessionSampleRate":"1","deploymentId":"aws-491988406224","routeName":"homepage","tenant":"web-platforms"});
</script>
<link data-rh="true" href="/vi-assets/static-assets/favicon-d2483f10ef688e6f89e23806b9700298.ico" rel="shortcut icon"/><link data-rh="true" href="/vi-assets/static-assets/apple-touch-icon-28865b72953380a40aa43318108876cb.png" rel="apple-touch-icon"/><link data-rh="true" href="/vi-assets/static-assets/ios-ipad-144x144-28865b72953380a40aa43318108876cb.png" rel="apple-touch-icon-precomposed" sizes="144×144"/><link data-rh="true" href="/vi-assets/static-assets/ios-iphone-114x144-080e7ec6514fdc62bcbb7966d9b257d2.png" rel="apple-touch-icon-precomposed" sizes="114×114"/><link data-rh="true" href="/vi-assets/static-assets/ios-default-homescreen-57x57-43808a4cd5333b648057a01624d84960.png" rel="apple-touch-icon-precomposed"/>
<link crossorigin="anonymous" href="https://g1.nyt.com" rel="preconnect"/>
<link crossorigin="" href="https://g1.nyt.com/fonts/css/web-fonts.a65411eeb1ab091c1b7eaa3047f86dabe8355f0e.css" rel="stylesheet" type="text/css"/>
<link href="/vi-assets/static-assets/global-75f713f5ef71fb15f77ecbb55c1f03b7.css" rel="stylesheet"/>
<style>[data-timezone] { display: none }</style>
<style data-lights-css="val7tc tvfu69 k008qs 1dv1kvn 8pe5zk 42kif7 1nrdlje 1baq5tz qebcue j184jz vryaoq wsvg60 11kdrny hqhlyo j7qwjs 1nudrh3 1qalmak 1qt2b4r kz1pwz 1n92pmf ed0o7b 1x8fy0n v21ltd 1llhclm uc4bdz 1jmk4jh 1e1s8k7 1qa4qp6 13mf4b4 17ih8de 79elbk ki347z 1baulvz gx0lhm 777zgl rfqw0c 1rr4qq7 stscvm 158f1cv i6bazn 122y91a le4k3i 1ppjtba 1lc519a pnomtl b66c96 r1a9vb 1pqiz9q szc2m3 tm2y8a hdqqnp 5rwu3a 15rwwo 1wd5atx 1jke4yk 12ilnc8 1vzzb6i 19pyzy9 1x6dw1b kvmiee gf25qg 7s6arl 1gq8co7 1bp1dzo 17j7fe1 1qtaxzf u90q6n 1stvlmo kpxlkr 1ho5u4o t8x4fj a7htku 1r6wvpq aljv3 ahe4g0 1hti9pf pqd7q9 1om0j5j xdandi wne2ji 1p5yz2j 1yg7u8y y7g724 hurk9l l08pwh 1a3ibh4 ae0yjg 12tlih8 1ufpbe9 16lxk39 dzl7b5 91bpc3 1tic89u 1r9ysjz rpp6l6 tt22rr dbnm2s h1qsyw sb29ax 1t9uiwm b6n3ve 17jnd0c o0xbcl cw4snp hr63kf uzitgk kuec6r kaomn7 zsumg4 1vmm8al v7w2uh 1cv5kz9 10avpiw 1pwpj83 shshzh 15zbqwq kzazds 1ja5hfe 13ug63a 1rh6kpg 3o0db8 1qpxn73 1d18fn2 1fvhbq8 1u1zmuv 1lel406 wne7fp f6ozty oz0kdv remrbt 4dgob3 1l41i6b 1508xc5 8xdxq2 nhjhh0 1shpxcj 50gbj 15el443 e2a84x bfvq22 9mylee rgq5s4 1szm689 12qvnte 1lvvmm 1l10c03 hep49k f52tr7 1gg6cw2 ofqxyv 1a0ymrn 1e7omub ho9dtj h753dc 1432v6n 1l5zmz6 ih99h 10kglqe 1a5fuvt 160v3a8 tdd4a3 wt2ynm e3mkx1 cn25ca 1as87w5 vrywmf 1njmbkd gd99i5 uf1mhr 1qle2ps u9thtu 1pvdjg1 1w1paqe 18c0apr jsjvin 1p26664 inu6q5 fdpae6 17jkqqy tlxejj 170wooj 125baf0 kuzbnk 6l658f 17sbsu2 11uabb1 50ivt8 2z4gz0 1ichrj1 8ecw4e oi0jtw 77hcv 1qcrlqu 14m0cem ljayxv 13qj3r5 r7t1h4 132p2yg 1didtc4 1xpp3bj b3grxh 167b3qw r6g2pn 1qlpcok dd9fkn 9jpfr0 34zueq 15iksc8 1q7rq5u c38y3b hcfrni hmsvwu m5ahyg 4blv3u 1bjokvb ztw7vs m3y3ka 6gfk0j 1debogi cephuo hf5jg6 fyro28 6w67yl n3ncu4 fi9dry 14ywp0y 1nkoa58 nn5e6h ha69tt name 5j8bii duration delay timing-function">@-webkit-keyframes animation-5j8bii{from{opacity:0;}to{opacity:1;}}@keyframes animation-5j8bii{from{opacity:0;}to{opacity:1;}}.SP_commentsRefactor_1224_1_New .comments-button-container{display:none;}.SP_commentsRefactor_1224_1_New .comments-button-container-v2{display:-webkit-inline-box;display:-webkit-inline-flex;display:-ms-inline-flexbox;display:inline-flex;}.SP_commentsRefactor_1224_1_New .big-bottom-button-v2{display:block;}.css-k008qs{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;}.css-1dv1kvn{border:0;-webkit-clip:rect(0 0 0 0);clip:rect(0 0 0 0);height:1px;margin:-1px;overflow:hidden;padding:0;position:absolute;width:1px;}.css-8pe5zk{display:none;text-align:center;}@media (min-width:1024px){.css-8pe5zk{display:block;}}.css-42kif7{-webkit-letter-spacing:1.4px;-moz-letter-spacing:1.4px;-ms-letter-spacing:1.4px;letter-spacing:1.4px;margin-top:3px;}.css-1nrdlje{display:inline-block;font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.6875rem;text-transform:uppercase;font-weight:500;}.css-1nrdlje:first-child{margin-left:0;}.css-1baq5tz{color:var(--color-content-tertiary,#5A5A5A);display:inline-block;border-radius:3px;padding:7px 10px;}.css-1baq5tz:hover{background-color:#f7f7f7;}@media (min-width:1024px){.css-1baq5tz{padding:7px 8px 6px;-webkit-transition:background 0.6s ease;transition:background 0.6s ease;}}.css-qebcue{display:none;font-size:10px;margin-left:auto;text-transform:uppercase;}.hasLinks .css-qebcue{display:block;min-height:10px;}@media (min-width:740px){return!e.theme.isIntlHomepage.hasLinks .css-qebcue function(e).hasLinks .css-qebcue function(e)!e.theme.homepage.hasLinks .css-qebcue function(e).hasLinks .css-qebcue function(e){margin:"none",position:"absolute",right:"20px";}}@media (min-width:1024px){.hasLinks .css-qebcue{display:none;min-height:0;}}.css-j184jz{display:inline-block;font-size:0.875rem;line-height:1.25rem;-webkit-transition:color 0.6s ease;transition:color 0.6s ease;color:#121212;}.css-j184jz:hover{color:#666666;}.css-vryaoq{display:none;}@media (min-width:1024px){.css-vryaoq{display:unset;height:41px;}}.css-wsvg60{border:0;-webkit-clip:rect(0 0 0 0);clip:rect(0 0 0 0);height:1px;margin:-1px;overflow:hidden;padding:0;position:absolute;width:1px;border-radius:3px;cursor:pointer;font-family:nyt-franklin,helvetica,arial,sans-serif;-webkit-transition:ease 0.6s;transition:ease 0.6s;white-space:nowrap;vertical-align:middle;background-color:transparent;color:#000;font-size:11px;line-height:11px;font-weight:700;-webkit-letter-spacing:0.02em;-moz-letter-spacing:0.02em;-ms-letter-spacing:0.02em;letter-spacing:0.02em;padding:11px 12px 8px;background:#ffffff;display:inline-block;left:44px;text-transform:uppercase;-webkit-transition:none;transition:none;z-index:5;}.css-wsvg60:active,.css-wsvg60:focus{-webkit-clip:auto;clip:auto;overflow:visible;width:auto;height:auto;}.css-wsvg60::-moz-focus-inner{padding:0;border:0;}.css-wsvg60:-moz-focusring{outline:1px dotted;}.css-wsvg60:disabled,.css-wsvg60.disabled{opacity:0.5;cursor:default;}.css-wsvg60:active,.css-wsvg60.active{background-color:#f7f7f7;}@media (min-width:740px){.css-wsvg60:hover{background-color:#f7f7f7;}}.css-wsvg60:focus{margin-top:3px;padding:8px 8px 6px;}@media (max-width:600px){.css-wsvg60:focus{margin-top:12px;margin-left:9px;}}@media (min-width:1024px){.css-wsvg60{left:112px;}}.css-11kdrny{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;margin-bottom:1.25rem;color:var(--color-content-secondary,#363636);cursor:pointer;}.css-11kdrny:hover .e10k0zts0{color:var(--color-content-tertiary,#5A5A5A);}.css-11kdrny img{width:30px;height:30px;margin-right:0.625rem;margin-top:3px;object-fit:content-cover;}.css-hqhlyo{border:1px solid #e2e2e2;}.css-j7qwjs{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;}.css-1nudrh3{color:var(--color-content-tertiary,#5A5A5A);font-family:nyt-franklin,helvetica,arial,sans-serif;font-weight:500;font-size:0.6875rem;line-height:1.25rem;margin-bottom:1rem;-webkit-letter-spacing:0.04em;-moz-letter-spacing:0.04em;-ms-letter-spacing:0.04em;letter-spacing:0.04em;height:27px;cursor:default;text-transform:uppercase;display:block;}.css-1qalmak{display:none;position:absolute;background-color:#ffffff;box-shadow:0 10px 10px rgba(0,0,0,0.1);left:0;top:100%;width:100vw;z-index:1000000002;}.css-1qalmak:first-child{display:block;}@media (max-width:1280px){.css-1qalmak{top:calc(100% - 4px);}}@media (min-width:1278px){.css-1qalmak{left:calc(-1 * (100vw - 1200px) / 2);}}.css-1qt2b4r{max-width:1200px;width:94%;margin:0 auto;padding-bottom:1.5rem;padding-top:1.5rem;text-align:center;color:var(--color-content-secondary,#363636);border-top:1px solid #ebebeb;font-size:0.8125rem;line-height:1.125rem;cursor:default;}.css-1qt2b4r a{font-weight:700;}.css-1qt2b4r a:hover{color:var(--color-content-tertiary,#5A5A5A);}.css-kz1pwz{height:-webkit-fit-content;height:-moz-fit-content;height:fit-content;margin-bottom:0.75rem;display:block;color:var(--color-content-primary,#121212);}.css-kz1pwz:hover{color:var(--color-content-tertiary,#5A5A5A);}.css-1n92pmf{background-size:contain;background-repeat:no-repeat;display:inline-block;min-height:20px;min-width:20px;margin-right:8px;position:relative;top:5px;vertical-align:top;margin-top:-4px;}.css-ed0o7b{height:-webkit-fit-content;height:-moz-fit-content;height:fit-content;margin-bottom:0.5rem;display:block;}.css-ed0o7b p{display:inline-block;margin-top:3px;max-width:calc(100% - 30px);}.css-ed0o7b:hover{color:var(--color-content-tertiary,#5A5A5A);}.css-1x8fy0n{position:fixed;top:-120px;left:0;width:100%;height:120px;z-index:100001;background:#ffffff;}.css-v21ltd{box-shadow:0 0 5px 1px rgba(0,0,0,0.28);background:#ffffff;position:fixed;width:100%;-webkit-transform:translateY(-70px);-ms-transform:translateY(-70px);transform:translateY(-70px);top:0;left:0;z-index:100000;-webkit-transition:0.5s cubic-bezier(0.23,1,0.32,1);transition:0.5s cubic-bezier(0.23,1,0.32,1);}.css-1llhclm{margin:0 auto;padding:0 3%;max-width:1200px;white-space:nowrap;}.css-uc4bdz{min-height:269px;}@media (min-width:1150px){.css-uc4bdz{min-height:431.5px;}}.NYTApp .css-uc4bdz{display:none;}@media (min-width:1150px){.css-1jmk4jh{margin:0 auto;max-width:1200px;padding:0 3% 9px;}}.NYTApp .css-1jmk4jh{display:none;}@media print{.css-1jmk4jh{display:none;}}.css-1e1s8k7{font-size:11px;text-align:center;padding-bottom:25px;}@media (min-width:1024px){.css-1e1s8k7{padding:0 3% 9px;}}.css-1e1s8k7.dockVisible{padding-bottom:45px;}@media (min-width:1024px){.css-1e1s8k7.dockVisible{padding:0 3% 45px;}}@media (min-width:1150px){.css-1e1s8k7{margin:0 auto;max-width:1200px;}}.NYTApp .css-1e1s8k7{display:none;}@media print{.css-1e1s8k7{display:none;}}.css-1qa4qp6{border-top:1px solid #ebebeb;padding-top:9px;margin:0 0 35px;}.css-13mf4b4{color:#666666;font-family:nyt-franklin,helvetica,arial,sans-serif;padding:10px 0;-webkit-text-decoration:none;text-decoration:none;white-space:nowrap;}.css-13mf4b4:hover{-webkit-text-decoration:underline;text-decoration:underline;}.css-17ih8de{box-sizing:border-box;vertical-align:top;}.css-79elbk{position:relative;}.css-ki347z{overflow:hidden;position:relative;display:inline-block;}.css-1baulvz{display:inline-block;}.css-gx0lhm{margin-bottom:0;}.css-777zgl{position:absolute;width:1px;height:1px;margin:-1px;padding:0;border:0;-webkit-clip:rect(0 0 0 0);clip:rect(0 0 0 0);overflow:hidden;text-align:center;}.css-777zgl:focus-visible{background-color:#fff;border-radius:3px;height:auto;width:auto;-webkit-clip:auto;clip:auto;font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.6875rem;font-weight:700;left:50%;padding:8px 8px 6px;-webkit-transform:translateX(-50%);-ms-transform:translateX(-50%);transform:translateX(-50%);top:5px;}.css-rfqw0c{text-align:center;height:100%;display:block;}.css-1rr4qq7{-webkit-flex:1;-ms-flex:1;flex:1;}.css-stscvm{display:none;}@media (min-width:1024px){.css-stscvm{display:block;margin-top:-15px;}}@media (min-width:1024px){.css-158f1cv{text-align:center;}}.css-i6bazn{overflow:hidden;}.css-122y91a{position:absolute;top:0;left:0;width:100%;height:100%;}.css-le4k3i{width:100%;color:var(--color-content-quaternary,#727272);text-transform:uppercase;font-size:10px;font-family:nyt-franklin,helvetica,arial,sans-serif;text-align:center;-webkit-letter-spacing:0.5px;-moz-letter-spacing:0.5px;-ms-letter-spacing:0.5px;letter-spacing:0.5px;padding:11px 0 10px;}.css-1ppjtba{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;margin-right:10px;}.css-1lc519a{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;position:relative;overflow-x:scroll;-webkit-scroll-snap-type:x mandatory;-moz-scroll-snap-type:x mandatory;-ms-scroll-snap-type:x mandatory;scroll-snap-type:x mandatory;-webkit-scrollbar-width:none;-moz-scrollbar-width:none;-ms-scrollbar-width:none;scrollbar-width:none;}.css-1lc519a::-webkit-scrollbar{display:none;}.css-pnomtl{height:20px;width:100%;display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:row;-ms-flex-direction:row;flex-direction:row;-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;pointer-events:none;z-index:100;margin-top:10px;}.css-b66c96{width:8px;height:8px;background-color:var(--color-stroke-tertiary,#C7C7C7);border-radius:4px;-webkit-transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;margin:3px;}.css-r1a9vb{margin:0.75rem 0 1.25rem;text-align:center;position:relative;}.css-1pqiz9q{display:none;position:absolute;right:0;top:50%;-webkit-transform:translateY(-50%);-ms-transform:translateY(-50%);transform:translateY(-50%);}@media (min-width:740px){.css-1pqiz9q{display:block;}}.css-szc2m3::after{content:'';display:block;clear:both;}.css-tm2y8a{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:end;-webkit-justify-content:flex-end;-ms-flex-pack:end;justify-content:flex-end;text-align:right;color:var(--color-content-quaternary,#727272);margin-top:4px;font-size:0.5625rem;font-family:'nyt-franklin',helvetica,arial,sans-serif;font-style:normal;font-weight:500;line-height:0.625rem;-webkit-letter-spacing:0.012em;-moz-letter-spacing:0.012em;-ms-letter-spacing:0.012em;-webkit-letter-spacing:0.012em;-moz-letter-spacing:0.012em;-ms-letter-spacing:0.012em;letter-spacing:0.012em;}@media (max-width:740px){.css-tm2y8a{padding:0 20px;font-size:0.625rem;}}.css-hdqqnp{width:100%;vertical-align:bottom;}@media (min-width:740px){.css-5rwu3a:hover svg circle{fill:var(--color-stroke-quaternary,#DFDFDF);}}.css-15rwwo{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;width:100%;-webkit-box-pack:start;-webkit-justify-content:flex-start;-ms-flex-pack:start;justify-content:flex-start;}.css-1wd5atx{display:-webkit-inline-box;display:-webkit-inline-flex;display:-ms-inline-flexbox;display:inline-flex;vertical-align:top;}.css-1jke4yk{position:relative;width:100%;}.css-12ilnc8{overflow:hidden;position:relative;width:100%;}.css-1vzzb6i{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-wrap:wrap;-ms-flex-wrap:wrap;flex-wrap:wrap;margin-bottom:20px;}.css-19pyzy9{-webkit-order:90;-ms-flex-order:90;order:90;}.css-1x6dw1b{background-color:var(--color-background-tertiary,#EBEBEB);min-height:22px;}.css-kvmiee{background-color:var(--color-background-tertiary,#EBEBEB);min-height:144px;margin-bottom:11px;}.css-gf25qg{background-color:var(--color-background-tertiary,#EBEBEB);min-height:51px;margin-bottom:10px;}.css-7s6arl h3{color:var(--color-content-primary,#121212);font-family:nyt-karnak,georgia,'times new roman',times,serif;font-weight:400;font-size:1.25rem;line-height:1.5rem;margin:-0.3125rem 0 0.4375rem 0;}.css-7s6arl p{color:var(--color-content-secondary,#363636);max-width:11rem;}.css-7s6arl a:hover h3,.css-7s6arl a:hover p{color:var(--color-content-tertiary,#5A5A5A);}.css-1gq8co7{width:8px;}.css-1bp1dzo{background:#ffffff;display:inline-block;text-transform:uppercase;-webkit-transition:none;transition:none;z-index:1000000140;margin-top:-5px;cursor:default;}.css-17j7fe1{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;padding:0;}@media (min-width:1277px){.css-17j7fe1{position:relative;}}.css-1qtaxzf{font-family:nyt-franklin,helvetica,arial,sans-serif;font-weight:500;font-size:0.875rem;line-height:0.875rem;padding:1rem 12px;}.css-1qtaxzf:hover > a{-webkit-text-decoration:underline;text-decoration:underline;-webkit-text-decoration-thickness:2px;text-decoration-thickness:2px;text-underline-offset:4px;}.css-1qtaxzf:nth-child(7){padding-left:12px;}.css-1qtaxzf:nth-child(7)::before{content:'';height:20px;margin-right:20px;margin-left:-4px;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-u90q6n{padding:0 6px 0 0;display:inline-block;color:var(--color-content-primary,#121212);-webkit-letter-spacing:0.1px;-moz-letter-spacing:0.1px;-ms-letter-spacing:0.1px;letter-spacing:0.1px;}.css-1stvlmo{-webkit-transform:translateY(-110%);-ms-transform:translateY(-110%);transform:translateY(-110%);-webkit-transition:-webkit-transform 0.3s ease-in-out;-webkit-transition:transform 0.3s ease-in-out;transition:transform 0.3s ease-in-out;display:inline-block;}.css-kpxlkr{-webkit-transform:translateY(110%);-ms-transform:translateY(110%);transform:translateY(110%);left:0;position:absolute;-webkit-transition:-webkit-transform 0.3s ease-in-out;-webkit-transition:transform 0.3s ease-in-out;transition:transform 0.3s ease-in-out;display:inline-block;-webkit-transform:translateY(0);-ms-transform:translateY(0);transform:translateY(0);display:inline-block;}@media (max-width:600px){.css-kpxlkr{display:block;left:unset;top:0;}}.css-1ho5u4o{list-style:none;margin:0 0 15px;padding:0;}@media (min-width:600px){.css-1ho5u4o{display:inline-block;}}.css-t8x4fj{list-style:none;line-height:8px;padding:0;}.css-t8x4fj:last-child > li{margin:16px 0 0 0;}@media (min-width:600px){.css-t8x4fj{display:inline-block;}}.css-a7htku{display:inline-block;line-height:20px;padding:0 10px;}.css-a7htku:first-child{border-left:none;}.css-a7htku.desktop{display:none;}@media (min-width:740px){.css-a7htku.smartphone{display:none;}.css-a7htku.desktop{display:inline-block;}.css-a7htku.mobileOnly{display:none;}}.css-1r6wvpq{opacity:1;visibility:visible;-webkit-animation-name:animation-5j8bii;animation-name:animation-5j8bii;-webkit-animation-duration:300ms;animation-duration:300ms;-webkit-animation-delay:0ms;animation-delay:0ms;-webkit-animation-timing-function:ease-out;animation-timing-function:ease-out;}@media print{.css-1r6wvpq{display:none;}}.css-aljv3{position:relative;position:relative;min-height:90px;width:100%;overflow:hidden;}@media (min-width:1024px){.css-ahe4g0{position:relative;border-bottom:none;}}@media (min-width:1024px){.css-ahe4g0{margin:0 auto;padding:0 3%;}}@media (min-width:1150px){.css-ahe4g0{margin:auto;padding:0 3%;max-width:1200px;}}.css-1hti9pf{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:space-around;-webkit-justify-content:space-around;-ms-flex-pack:space-around;justify-content:space-around;left:10px;position:absolute;}@media (min-width:1024px){.css-1hti9pf{left:0;}}@media print{.css-1hti9pf{display:none;}}.css-pqd7q9{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;margin:0 auto;}@media screen and (-ms-high-contrast:active),(-ms-high-contrast:none){.css-pqd7q9 > *{min-height:1px;}}@media (min-width:740px){.css-pqd7q9{padding:0 3%;}}@media (min-width:1070px){.css-pqd7q9{max-width:1200px;}}.css-1om0j5j:before{background:var(--color-background-tertiary,#EBEBEB);-webkit-mask-image:url('data:image/svg+xml, %3Csvg%20xmlns%3D%22http%3A%2F%2Fwww.w3.org%2F2000%2Fsvg%22%3E%3Crect%20height%3D%2216%22%20width%3D%22100%25%22%3E%3C%2Frect%3E%3Crect%20height%3D%2216%22%20width%3D%22100%25%22%20y%3D%2224%22%3E%3C%2Frect%3E%3C%2Fsvg%3E');mask-image:url('data:image/svg+xml, %3Csvg%20xmlns%3D%22http%3A%2F%2Fwww.w3.org%2F2000%2Fsvg%22%3E%3Crect%20height%3D%2216%22%20width%3D%22100%25%22%3E%3C%2Frect%3E%3Crect%20height%3D%2216%22%20width%3D%22100%25%22%20y%3D%2224%22%3E%3C%2Frect%3E%3C%2Fsvg%3E');content:'';display:block;height:40px;}.css-wne2ji{background-color:var(--color-background-tertiary,#EBEBEB);display:block;width:100%;height:0;position:relative;overflow:hidden;padding:0 0 100% 0;}.css-wne2ji > *{position:absolute;top:0;left:0;width:100%;height:100%;}.css-1p5yz2j{font-family:nyt-imperial,georgia,'times new roman',times,serif;font-size:0.6875rem;line-height:1.4em;margin:0;color:var(--color-content-quaternary,#727272);text-align:right;margin-top:0.25rem;}.css-1yg7u8y{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.3em;margin:0;color:var(--color-content-quaternary,#727272);display:inline-block;vertical-align:text-top;line-height:1.2em;}@media (min-width:481px){.css-1yg7u8y{font-size:0.56rem;}}.css-y7g724{-webkit-flex:1;-ms-flex:1;flex:1;vertical-align:top;margin-bottom:12px;margin-top:-4px;}@media (min-width:740px){.css-y7g724{margin-top:-11px;}}.css-hurk9l{margin:0;margin-bottom:0;}.css-l08pwh{width:100%;margin:0 auto;height:auto;}.css-1a3ibh4{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-align-items:baseline;-webkit-box-align:baseline;-ms-flex-align:baseline;align-items:baseline;display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;margin-bottom:0.25rem;}.css-ae0yjg{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.6875rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-primary,#121212);margin-right:0;}.css-12tlih8{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.6875rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-primary,#121212);font-weight:800;margin-right:0;color:var(--color-signal-breaking,#D0021B);white-space:normal;}.css-1ufpbe9{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.6875rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-primary,#121212);font-weight:800;color:var(--color-signal-breaking,#D0021B);white-space:normal;}.css-16lxk39{text-transform:none;font-weight:initial;-webkit-letter-spacing:0;-moz-letter-spacing:0;-ms-letter-spacing:0;letter-spacing:0;margin-left:13px;height:12px;display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;white-space:nowrap;}.css-dzl7b5{opacity:0;-webkit-transition:opacity 300ms ease-in-out;transition:opacity 300ms ease-in-out;width:100%;vertical-align:bottom;}.css-91bpc3{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.25rem;line-height:1.15em;-webkit-letter-spacing:0.01em;-moz-letter-spacing:0.01em;-ms-letter-spacing:0.01em;letter-spacing:0.01em;margin:0;color:var(--color-content-primary,#121212);font-weight:700;-webkit-font-smoothing:antialiased;}@media (min-width:481px){.css-91bpc3{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.125rem;line-height:1.2em;-webkit-letter-spacing:0.01em;-moz-letter-spacing:0.01em;-ms-letter-spacing:0.01em;letter-spacing:0.01em;}}.css-1tic89u{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:justify;-webkit-justify-content:space-between;-ms-flex-pack:justify;justify-content:space-between;-webkit-align-items:start;-webkit-box-align:start;-ms-flex-align:start;align-items:start;-webkit-align-content:stretch;-ms-flex-line-pack:stretch;align-content:stretch;margin-top:0.5rem;margin-bottom:0;}@media (min-width:740px){.css-1tic89u{margin-top:0.25rem;}}.css-1r9ysjz:not(:empty){padding-bottom:2.5rem;padding-bottom:2.5rem;}@media (min-width:740px){.css-1r9ysjz:not(:empty){padding-bottom:1.5rem;}}@media (min-width:740px){.css-rpp6l6{position:relative;width:100vw;left:50%;right:50%;margin-left:-50vw;margin-right:-50vw;}}.css-tt22rr{background-color:var(--color-background-secondary,#F8F8F8);border-top:1px solid var(--color-stroke-quaternary,#DFDFDF);border-bottom:1px solid var(--color-stroke-quaternary,#DFDFDF);padding-bottom:16px;min-height:250px;}@media (min-width:740px){.css-tt22rr{padding-bottom:32px;margin-bottom:0;}}.css-dbnm2s{width:8px;height:8px;background-color:var(--color-stroke-tertiary,#C7C7C7);border-radius:4px;-webkit-transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;margin:3px;background-color:var(--color-stroke-primary,#121212);}.css-h1qsyw{width:8px;height:8px;background-color:var(--color-stroke-tertiary,#C7C7C7);border-radius:4px;-webkit-transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;margin:3px;width:6px;height:6px;}.css-sb29ax{width:8px;height:8px;background-color:var(--color-stroke-tertiary,#C7C7C7);border-radius:4px;-webkit-transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;margin:3px;width:6px;height:6px;width:3px;height:3px;}.css-1t9uiwm{width:8px;height:8px;background-color:var(--color-stroke-tertiary,#C7C7C7);border-radius:4px;-webkit-transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;transition:width 0.5s,height 0.5s,background-color 0.01s ease-out;margin:3px;width:6px;height:6px;width:3px;height:3px;width:0;height:0;margin:0;}.css-b6n3ve{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(6,1fr);}.css-17jnd0c{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(6,1fr);grid-column-gap:calc(1rem - 1px);}.css-o0xbcl{min-width:0;position:relative;grid-column:span 2;}.css-o0xbcl:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-cw4snp{min-width:0;position:relative;grid-column:span 4;}.css-hr63kf{font-family:nyt-cheltenham-small,nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:0.75rem;line-height:1.25em;-webkit-letter-spacing:0.01em;-moz-letter-spacing:0.01em;-ms-letter-spacing:0.01em;letter-spacing:0.01em;margin:0;color:var(--color-content-quaternary,#727272);font-weight:700;margin-bottom:0.25rem;text-transform:uppercase;line-height:1.35em !important;-webkit-letter-spacing:1px !important;-moz-letter-spacing:1px !important;-ms-letter-spacing:1px !important;letter-spacing:1px !important;}@media (min-width:481px){.css-hr63kf{font-family:nyt-cheltenham-small,nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:0.6875rem;line-height:1.25em;-webkit-letter-spacing:0.01em;-moz-letter-spacing:0.01em;-ms-letter-spacing:0.01em;letter-spacing:0.01em;}}.css-uzitgk{font-family:nyt-cheltenham-text-cond,nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.5rem;line-height:1.15em;-webkit-letter-spacing:0.0025em;-moz-letter-spacing:0.0025em;-ms-letter-spacing:0.0025em;letter-spacing:0.0025em;margin:0;color:var(--color-content-primary,#121212);font-weight:700;-webkit-font-smoothing:antialiased;}@media (min-width:481px){.css-uzitgk{font-family:nyt-cheltenham-text-cond,nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.25rem;line-height:1.15em;-webkit-letter-spacing:0.0025em;-moz-letter-spacing:0.0025em;-ms-letter-spacing:0.0025em;letter-spacing:0.0025em;}}.css-kuec6r{min-width:0;position:relative;grid-column:span 4;margin-right:-1rem;}.css-kuec6r:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-kaomn7{font-family:nyt-cheltenham-text-cond,nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.375rem;line-height:1.15em;-webkit-letter-spacing:0.0025em;-moz-letter-spacing:0.0025em;-ms-letter-spacing:0.0025em;letter-spacing:0.0025em;margin:0;color:var(--color-content-primary,#121212);font-weight:700;-webkit-font-smoothing:antialiased;}@media (min-width:481px){.css-kaomn7{font-family:nyt-cheltenham-text-cond,nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.125rem;line-height:1.15em;-webkit-letter-spacing:0.0025em;-moz-letter-spacing:0.0025em;-ms-letter-spacing:0.0025em;letter-spacing:0.0025em;}}.css-zsumg4{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:left;-webkit-justify-content:left;-ms-flex-pack:left;justify-content:left;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;}.css-1vmm8al{display:inline-block;margin-top:8px;margin-right:8px;}.css-v7w2uh{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:1rem;line-height:1.3em;font-weight:700;color:var(--color-content-primary,#121212);-webkit-letter-spacing:0.5px;-moz-letter-spacing:0.5px;-ms-letter-spacing:0.5px;letter-spacing:0.5px;margin-bottom:12px;margin-top:-4px;}@media (min-width:740px){.css-v7w2uh{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.875rem;line-height:1.3em;margin-top:-11px;}}.css-1cv5kz9{margin:0 auto;max-width:945px;}.css-10avpiw{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-scroll-snap-align:start;-moz-scroll-snap-align:start;-ms-scroll-snap-align:start;scroll-snap-align:start;min-width:100%;margin-right:40px;box-sizing:border-box;padding:0;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;padding-left:20px;padding-right:20px;margin:0;}.css-10avpiw:last-child{margin-right:0;}@media (min-width:600px){.css-10avpiw{padding-left:0;padding-right:0;margin-left:40px;}}.css-1pwpj83{color:inherit;-webkit-text-decoration:underline;text-decoration:underline;-webkit-text-decoration:none;text-decoration:none;}.css-1pwpj83:hover{-webkit-text-decoration:none;text-decoration:none;}.css-1pwpj83[target="_blank"]::after{-webkit-clip:rect(0 0 0 0);clip:rect(0 0 0 0);-webkit-clip-path:inset(50%);clip-path:inset(50%);height:1px;overflow:hidden;position:absolute;white-space:nowrap;width:1px;content:'(opens in new tab)';}@supports (content:"primary" / "alt"){.css-1pwpj83[target="_blank"]::after{content:'\FEFF' / '(opens in new tab)';display:contents;}}.css-shshzh{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;margin:20px 0;margin:0 auto 10px;width:100%;}.css-15zbqwq{margin-right:10px;display:inline-block;width:40px;height:40px;border-radius:50%;display:block;margin-right:10px;-webkit-flex-shrink:0;-ms-flex-negative:0;flex-shrink:0;width:44px;height:44px;}.css-15zbqwq img{margin-right:0;}.css-15zbqwq img{border-radius:50%;}@media (min-width:1024px){.css-15zbqwq{width:40px;height:40px;}}.css-kzazds{display:inline-block;margin:0;font-size:0.875rem;line-height:1.125rem;font-family:nyt-franklin,helvetica,arial,sans-serif;font-weight:700;color:var(--color-content-secondary,#363636);font-weight:500;margin-top:2px;color:var(--color-content-secondary,#363636);padding-right:0.25rem;font-family:nyt-franklin,helvetica,arial,sans-serif;font-weight:700;margin-top:2px;color:var(--color-content-secondary,#363636);padding-right:0.25rem;}@media (min-width:740px){.css-kzazds{font-size:0.9375rem;line-height:1.25rem;}}.css-kzazds a{color:var(--color-content-secondary,#363636);}.css-kzazds,.css-kzazds span{font-size:0.8125rem;line-height:1rem;display:inline;}.css-kzazds .last-byline,.css-kzazds span .last-byline{margin-right:0.25rem;}.css-kzazds a{color:var(--color-content-secondary,#363636);}.css-kzazds,.css-kzazds span{font-size:0.875rem;font-weight:700;line-height:1.125rem;display:block;}.css-kzazds .last-byline,.css-kzazds span .last-byline{margin-right:0.25rem;}.css-1ja5hfe{margin:0;margin-bottom:1rem;color:var(--color-content-tertiary,#5A5A5A);display:inline;margin-bottom:0;font-weight:500;font:500 1rem/1.3 nyt-franklin,helvetica,arial,sans-serif;}@media (min-width:1024px){.css-1ja5hfe{margin-bottom:0.75rem;}}.desktop .css-1ja5hfe{font:500 0.875rem/1.3 nyt-franklin,helvetica,arial,sans-serif;}.css-13ug63a{background-color:transparent;display:inline-block;width:2.75em;height:2.75em;-webkit-align-self:center;-ms-flex-item-align:center;align-self:center;border:0;right:0;border-radius:50%;padding:0;fill:var(--color-background-primary,#FFFFFF);stroke:var(--color-stroke-primary,#121212);-webkit-transform:rotate(180deg);-ms-transform:rotate(180deg);transform:rotate(180deg);left:0;width:1.5rem;height:1.5rem;}.css-13ug63a:disabled svg{stroke:var(--color-stroke-quaternary,#DFDFDF);}.css-13ug63a svg{width:100%;height:100%;cursor:pointer;-webkit-transition:fill 0.2s,stroke 0.2s;transition:fill 0.2s,stroke 0.2s;}.css-13ug63a:hover:enabled svg{fill:var(--color-stroke-quaternary,#DFDFDF);}.css-13ug63a:first-child{margin-right:0.25em;}.css-1rh6kpg{background-color:transparent;display:inline-block;width:2.75em;height:2.75em;-webkit-align-self:center;-ms-flex-item-align:center;align-self:center;border:0;right:0;border-radius:50%;padding:0;fill:var(--color-background-primary,#FFFFFF);stroke:var(--color-stroke-primary,#121212);width:1.5rem;height:1.5rem;}.css-1rh6kpg:disabled svg{stroke:var(--color-stroke-quaternary,#DFDFDF);}.css-1rh6kpg svg{width:100%;height:100%;cursor:pointer;-webkit-transition:fill 0.2s,stroke 0.2s;transition:fill 0.2s,stroke 0.2s;}.css-1rh6kpg:hover:enabled svg{fill:var(--color-stroke-quaternary,#DFDFDF);}.css-1rh6kpg:first-child{margin-right:0.25em;}.css-3o0db8:after{background:var(--color-background-tertiary,#EBEBEB);-webkit-mask-image:url('data:image/svg+xml, %3Csvg%20xmlns%3D%22http%3A%2F%2Fwww.w3.org%2F2000%2Fsvg%22%3E%3Crect%20height%3D%2216%22%20width%3D%22100%25%22%3E%3C%2Frect%3E%3Crect%20height%3D%2216%22%20width%3D%22100%25%22%20y%3D%2224%22%3E%3C%2Frect%3E%3C%2Fsvg%3E');mask-image:url('data:image/svg+xml, %3Csvg%20xmlns%3D%22http%3A%2F%2Fwww.w3.org%2F2000%2Fsvg%22%3E%3Crect%20height%3D%2216%22%20width%3D%22100%25%22%3E%3C%2Frect%3E%3Crect%20height%3D%2216%22%20width%3D%22100%25%22%20y%3D%2224%22%3E%3C%2Frect%3E%3C%2Fsvg%3E');content:'';display:block;height:40px;}.css-1qpxn73{min-width:0;position:relative;grid-column:span 6;}.css-1d18fn2{min-width:0;position:relative;grid-column:span 6;}.css-1d18fn2:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-1fvhbq8{min-width:0;position:relative;grid-column:span 2;}.css-1u1zmuv:not(:empty){padding-bottom:2.5rem;}@media (min-width:740px){.css-1u1zmuv:not(:empty){padding-bottom:1.5rem;}}.css-1u1zmuv:not(:empty):not(:first-child)::before{border-bottom:2px solid var(--color-stroke-primary,#121212);content:'';display:block;margin-bottom:12px;margin-left:20px;margin-right:20px;}@media (min-width:740px){.css-1u1zmuv:not(:empty):not(:first-child)::before{border-bottom:1px solid var(--color-stroke-primary,#121212);margin-bottom:1.25rem;margin-left:0;margin-right:0;}}.css-1lel406{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:1rem;line-height:1.3em;font-weight:700;color:var(--color-content-primary,#121212);-webkit-letter-spacing:0.5px;-moz-letter-spacing:0.5px;-ms-letter-spacing:0.5px;letter-spacing:0.5px;margin-bottom:12px;margin-top:-4px;margin-bottom:16px;}@media (min-width:740px){.css-1lel406{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.875rem;line-height:1.3em;margin-top:-11px;}}.css-wne7fp{display:inline;font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1rem;line-height:1.2em;-webkit-letter-spacing:0.01em;-moz-letter-spacing:0.01em;-ms-letter-spacing:0.01em;letter-spacing:0.01em;font-weight:normal;font-style:italic;-webkit-letter-spacing:0.1px;-moz-letter-spacing:0.1px;-ms-letter-spacing:0.1px;letter-spacing:0.1px;padding-left:8px;}@media (min-width:740px){.css-wne7fp{font-family:nyt-cheltenham-small,nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:0.875rem;line-height:1.25em;-webkit-letter-spacing:0.01em;-moz-letter-spacing:0.01em;-ms-letter-spacing:0.01em;letter-spacing:0.01em;padding-left:8px;}}.css-f6ozty{height:-webkit-max-content;height:-moz-max-content;height:max-content;}.css-f6ozty > *::after{content:'';display:block;margin-top:0;margin-bottom:0;}.css-f6ozty > *:last-child::after{display:none;}.css-oz0kdv{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(3,1fr);}.css-remrbt{min-width:0;position:relative;grid-column:span 3;}.css-remrbt:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-4dgob3{min-width:0;position:relative;grid-column:span 3;}:root{--color-background-elevated:hsla(0,0%,100%,1);--color-background-primary:hsla(0,0%,100%,1);--color-background-quaternary:hsla(0,0%,78.04%,1);--color-background-secondary:hsla(0,0%,97.26%,1);--color-background-tertiary:hsla(0,0%,92.16%,1);--color-background-inversePrimary:hsla(0,0%,7.06%,1);--color-background-inverseSecondary:hsla(0,0%,21.18%,1);--color-background-overlay:hsla(0,0%,7.06%,0.58);--color-content-primary:hsla(0,0%,7.06%,1);--color-content-quaternary:hsla(0,0%,44.71%,1);--color-content-quintary:hsla(0,0%,54.51%,1);--color-content-secondary:hsla(0,0%,21.18%,1);--color-content-tertiary:hsla(0,0%,35.3%,1);--color-content-inversePrimary:hsla(0,0%,100%,1);--color-overlay-black:hsla(0,0%,0%,1);--color-signal-accent:hsla(215.28,72.23%,50.59%,1);--color-signal-breaking:hsla(352.72,98.1%,41.18%,1);--color-signal-developing:hsla(20.19,91.67%,47.06%,1);--color-signal-editorial:hsla(205.9,48.72%,38.24%,1);--color-signal-highlight:hsla(54.67,95.75%,90.79%,1);--color-signal-negative:hsla(354.29,98.83%,33.34%,1);--color-signal-positive:hsla(126.98,53.09%,31.77%,1);--color-stroke-primary:hsla(0,0%,7.06%,1);--color-stroke-quaternary:hsla(0,0%,87.46%,1);--color-stroke-secondary:hsla(0,0%,54.51%,1);--color-stroke-tertiary:hsla(0,0%,78.04%,1);--color-stroke-inversePrimary:hsla(0,0%,100%,1);--color-static-white:hsla(0,0%,100%,1);--color-static-gray100:hsla(0,0%,0%,1);}body{color:var(--color-content-primary,#121212);background:var(--color-background-primary,#FFFFFF);}.css-1508xc5{background:#ffffff;border-bottom:1px solid #e2e2e2;height:36px;padding:8px 15px 3px;position:relative;}@media (min-width:740px){.css-1508xc5{background:#ffffff;padding:10px 15px 6px;}}@media (min-width:1024px){.css-1508xc5{background:transparent;border-bottom:0;padding:4px 15px 8px;}}@media (min-width:1024px){.css-1508xc5{margin:0 auto;max-width:1605px;}}.css-8xdxq2{text-align:center;}@media (min-width:740px){.css-8xdxq2{padding-top:0;}}@media (min-width:1024px){.css-8xdxq2{display:none;}}@media print{.css-8xdxq2 a[href]::after{content:'';}.css-8xdxq2 svg{fill:black;}}.css-nhjhh0{display:block;width:189px;height:26px;margin:5px auto 0;}@media (min-width:740px){.css-nhjhh0{width:225px;height:31px;margin:4px auto 0;}}@media (min-width:1024px){.css-nhjhh0{width:195px;height:26px;margin:6px auto 0;}}.css-1shpxcj{display:inline-block;font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.6875rem;text-transform:uppercase;font-weight:500;display:inline-block;font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.6875rem;text-transform:uppercase;font-weight:600;}.css-1shpxcj:first-child{margin-left:0;}.css-1shpxcj a{color:var(--color-content-primary,#121212);}.css-50gbj{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:space-around;-webkit-justify-content:space-around;-ms-flex-pack:space-around;justify-content:space-around;position:absolute;right:10px;top:8px;}@media (min-width:740px){.css-50gbj{top:10px;}}@media (min-width:1024px){.css-50gbj{top:4px;right:0;}}@media print{.css-50gbj{display:none;}}.css-15el443{-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:11px;-webkit-box-pack:space-around;-webkit-justify-content:space-around;-ms-flex-pack:space-around;justify-content:space-around;padding:13px 20px 12px;border-bottom:1px solid #e2e2e2;background-color:#f7f7f7;-webkit-letter-spacing:0.1px;-moz-letter-spacing:0.1px;-ms-letter-spacing:0.1px;letter-spacing:0.1px;}@media (min-width:740px){.css-15el443{background-color:#ffffff;}}@media (min-width:740px){.css-15el443{position:relative;}}@media (min-width:1024px){.css-15el443{-webkit-box-pack:justify;-webkit-justify-content:space-between;-ms-flex-pack:justify;justify-content:space-between;border:none;padding:0;height:0;-webkit-transform:translateY(48px);-ms-transform:translateY(48px);transform:translateY(48px);-webkit-align-items:flex-end;-webkit-box-align:flex-end;-ms-flex-align:flex-end;align-items:flex-end;}}@media print{.css-15el443{display:none;}}.css-e2a84x{color:#121212;font-size:0.6875rem;font-family:nyt-franklin,helvetica,arial,sans-serif;display:none;width:auto;font-weight:700;display:block;}@media (min-width:740px){.css-e2a84x{text-align:center;width:100%;font-weight:700;}}@media (min-width:1024px){.css-e2a84x{font-size:0.875rem;line-height:1.25rem;width:auto;margin-bottom:4px;font-weight:400;}}.css-bfvq22{display:none;}@media (min-width:1024px){.css-bfvq22{display:block;}}.css-9mylee{-webkit-text-decoration:none;text-decoration:none;}.package-title-wrapper:hover .css-9mylee > *{color:var(--color-content-quaternary,#727272);}@media (hover:hover){.css-9mylee:hover .indicate-hover{color:var(--color-content-tertiary,#5A5A5A);}}.css-rgq5s4{-webkit-text-decoration:none;text-decoration:none;}.package-title-wrapper:hover .css-rgq5s4 *{color:var(--color-content-quaternary,#727272);}@media (hover:hover){.css-rgq5s4:hover .indicate-hover{color:var(--color-content-tertiary,#5A5A5A);}}.css-1szm689{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:justify;-webkit-justify-content:space-between;-ms-flex-pack:justify;justify-content:space-between;}.css-12qvnte:not(:empty):not(:first-child)::before{border-bottom:2px solid var(--color-stroke-primary,#121212);content:'';display:block;margin-bottom:12px;margin-left:20px;margin-right:20px;}@media (min-width:740px){.css-12qvnte:not(:empty):not(:first-child)::before{border-bottom:1px solid var(--color-stroke-primary,#121212);margin-bottom:1.25rem;margin-left:0;margin-right:0;}}.css-1lvvmm{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(14,1fr);}.css-1l10c03{min-width:0;position:relative;grid-column:span 14;}.css-1l10c03:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-hep49k{height:-webkit-max-content;height:-moz-max-content;height:max-content;}.css-hep49k > *::after{content:'';display:block;border-bottom:1px solid var(--color-stroke-quaternary,#DFDFDF);margin-top:1rem;margin-bottom:1rem;clear:both;grid-column:1/-1;}.css-hep49k > *:last-child::after{display:none;}.css-f52tr7{min-width:0;position:relative;grid-column:span 5;}.css-f52tr7:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-1gg6cw2{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.375rem;line-height:1.15em;margin:0;color:var(--color-content-primary,#121212);font-weight:700;-webkit-font-smoothing:antialiased;}.css-ofqxyv{color:var(--color-content-tertiary,#5A5A5A);font-family:nyt-imperial,georgia,'times new roman',times,Songti SC,simsun,serif;font-size:0.875rem;-webkit-letter-spacing:0.1px;-moz-letter-spacing:0.1px;-ms-letter-spacing:0.1px;letter-spacing:0.1px;line-height:1.1875rem;margin:0;padding:0;position:relative;margin-top:0.5rem;-webkit-font-smoothing:auto;}.css-1a0ymrn{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-quaternary,#727272);margin-top:0px;margin-bottom:0;}.css-1e7omub{min-width:0;position:relative;grid-column:span 9;}.css-ho9dtj{background-color:var(--color-background-tertiary,#EBEBEB);display:block;width:100%;height:0;position:relative;overflow:hidden;padding:0 0 66.66666666666666% 0;}.css-ho9dtj > *{position:absolute;top:0;left:0;width:100%;height:100%;}.css-h753dc:not(:empty){padding-bottom:2.5rem;}.css-h753dc:not(:empty):not(:first-child)::before{border-bottom:2px solid var(--color-stroke-primary,#121212);content:'';display:block;margin-bottom:12px;margin-left:20px;margin-right:20px;}@media (min-width:740px){.css-h753dc:not(:empty):not(:first-child)::before{border-bottom:1px solid var(--color-stroke-primary,#121212);margin-bottom:1.25rem;margin-left:0;margin-right:0;}}.css-1432v6n{min-width:0;position:relative;grid-column:span 5;margin-right:-1rem;}.css-1432v6n:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-1l5zmz6{color:var(--color-content-tertiary,#5A5A5A);font-family:nyt-imperial,georgia,'times new roman',times,Songti SC,simsun,serif;font-size:0.875rem;-webkit-letter-spacing:0.1px;-moz-letter-spacing:0.1px;-ms-letter-spacing:0.1px;letter-spacing:0.1px;line-height:1.1875rem;margin:0;padding:0;position:relative;margin-top:0.25rem;-webkit-font-smoothing:auto;}.css-ih99h{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:1rem;line-height:1.3em;margin:0;color:var(--color-content-secondary,#363636);margin-top:0.5rem;margin-bottom:0;color:var(--color-content-primary,#121212);font-size:0.9375rem;font-family:nyt-franklin,helvetica,arial,sans-serif;line-height:0.9375rem;}@media (min-width:740px){.css-ih99h{font-size:0.8125rem;margin-top:0.375rem;}}.css-10kglqe{min-width:0;position:relative;grid-column:span 7;}.css-10kglqe:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-1a5fuvt{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.125rem;line-height:1.2em;-webkit-letter-spacing:0.01em;-moz-letter-spacing:0.01em;-ms-letter-spacing:0.01em;letter-spacing:0.01em;margin:0;color:var(--color-content-primary,#121212);font-weight:700;-webkit-font-smoothing:antialiased;}@media (min-width:481px){.css-1a5fuvt{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1rem;line-height:1.2em;-webkit-letter-spacing:0.01em;-moz-letter-spacing:0.01em;-ms-letter-spacing:0.01em;letter-spacing:0.01em;}}.css-160v3a8{margin:0;margin-top:0;margin-bottom:0;}.css-tdd4a3{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-primary,#121212);margin-right:0;}.css-wt2ynm{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-primary,#121212);font-weight:600;margin-right:0;color:var(--color-content-primary,#121212);white-space:normal;}.css-e3mkx1{margin:0;border:0;height:0;border-top:1px solid var(--color-stroke-quaternary,#DFDFDF);margin-top:1rem;margin-bottom:1rem;}.css-cn25ca{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-auto-columns:1fr;grid-auto-flow:column;grid-template-columns:repeat(4,calc(50% - 17px));-webkit-transform:translateX(0%) translateX(0px);-ms-transform:translateX(0%) translateX(0px);transform:translateX(0%) translateX(0px);-webkit-transition:0.3s cubic-bezier(0.49,0.49,0.55,0.95);transition:0.3s cubic-bezier(0.49,0.49,0.55,0.95);}.css-cn25ca .carousel-story-1 > div:last-child{padding-right:29px;}.css-1as87w5{min-width:0;position:relative;grid-column:span 1;}.css-1as87w5:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-vrywmf{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(7,1fr);}.css-1njmbkd{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(7,1fr);grid-column-gap:calc(1rem - 1px);}.css-gd99i5{min-width:0;position:relative;grid-column:span 5;}.css-uf1mhr{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:justify;-webkit-justify-content:space-between;-ms-flex-pack:justify;justify-content:space-between;pointer-events:none;position:absolute;top:50%;-webkit-transform:translateY(-50%);-ms-transform:translateY(-50%);transform:translateY(-50%);width:100%;z-index:2;width:calc(100% + 26px + 2px);left:-16px;}.css-uf1mhr button{pointer-events:all;}.css-1qle2ps{background-color:transparent;display:inline-block;width:26px;height:26px;-webkit-align-self:center;-ms-flex-item-align:center;align-self:center;border:0;right:0;border-radius:50%;padding:0;fill:var(--color-background-primary,#FFFFFF);stroke:var(--color-stroke-primary,#121212);}.css-1qle2ps:disabled svg{stroke:var(--color-stroke-quaternary,#DFDFDF);}.css-1qle2ps svg{width:100%;height:100%;cursor:pointer;-webkit-transition:fill 0.2s,stroke 0.2s;transition:fill 0.2s,stroke 0.2s;}.css-1qle2ps:hover:enabled svg{fill:var(--color-stroke-quaternary,#DFDFDF);}.css-u9thtu{background-color:transparent;display:inline-block;width:26px;height:26px;-webkit-align-self:center;-ms-flex-item-align:center;align-self:center;border:0;right:0;border-radius:50%;padding:0;fill:var(--color-background-primary,#FFFFFF);stroke:var(--color-stroke-primary,#121212);-webkit-transform:rotate(180deg);-ms-transform:rotate(180deg);transform:rotate(180deg);left:0;-webkit-transition:visibility 0.2s,opacity 0.2s;transition:visibility 0.2s,opacity 0.2s;}.css-u9thtu:disabled svg{stroke:var(--color-stroke-quaternary,#DFDFDF);}.css-u9thtu svg{width:100%;height:100%;cursor:pointer;-webkit-transition:fill 0.2s,stroke 0.2s;transition:fill 0.2s,stroke 0.2s;}.css-u9thtu:hover:enabled svg{fill:var(--color-stroke-quaternary,#DFDFDF);}.css-u9thtu:disabled{visibility:hidden;opacity:0;}.css-1pvdjg1{background-color:transparent;display:inline-block;width:26px;height:26px;-webkit-align-self:center;-ms-flex-item-align:center;align-self:center;border:0;right:0;border-radius:50%;padding:0;fill:var(--color-background-primary,#FFFFFF);stroke:var(--color-stroke-primary,#121212);-webkit-transition:visibility 0.2s,opacity 0.2s;transition:visibility 0.2s,opacity 0.2s;}.css-1pvdjg1:disabled svg{stroke:var(--color-stroke-quaternary,#DFDFDF);}.css-1pvdjg1 svg{width:100%;height:100%;cursor:pointer;-webkit-transition:fill 0.2s,stroke 0.2s;transition:fill 0.2s,stroke 0.2s;}.css-1pvdjg1:hover:enabled svg{fill:var(--color-stroke-quaternary,#DFDFDF);}.css-1pvdjg1:disabled{visibility:hidden;opacity:0;}.css-1w1paqe{height:-webkit-max-content;height:-moz-max-content;height:max-content;}.css-1w1paqe:not(:empty){padding-bottom:2.5rem;}@media (min-width:740px){.css-1w1paqe:not(:empty){padding-bottom:1.5rem;}}.css-1w1paqe:not(:empty):not(:first-child)::before{border-bottom:2px solid var(--color-stroke-primary,#121212);content:'';display:block;margin-bottom:12px;margin-left:20px;margin-right:20px;}@media (min-width:740px){.css-1w1paqe:not(:empty):not(:first-child)::before{border-bottom:1px solid var(--color-stroke-primary,#121212);margin-bottom:1.25rem;margin-left:0;margin-right:0;}}.css-18c0apr{min-width:0;position:relative;grid-column:span 9;}.css-18c0apr:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-jsjvin{-webkit-flex:1;-ms-flex:1;flex:1;display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:justify;-webkit-justify-content:space-between;-ms-flex-pack:justify;justify-content:space-between;}.css-jsjvin:focus{box-sizing:border-box;border:1px solid transparent;}.css-jsjvin > *:not(:first-child){margin-left:16px;padding-left:16px;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}[aria-roledescription='carousel'] .css-jsjvin > *{margin-left:16px;padding-left:16px;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-1p26664{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-primary,#121212);font-weight:600;margin-right:0.5rem;color:var(--color-content-primary,#121212);white-space:normal;}.css-inu6q5{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-primary,#121212);font-weight:600;color:var(--color-content-quaternary,#727272);display:inline-block;}.css-fdpae6{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-quaternary,#727272);margin-top:0.25rem;display:inline;}.css-17jkqqy{height:-webkit-max-content;height:-moz-max-content;height:max-content;}.css-17jkqqy:not(:empty):not(:first-child)::before{border-bottom:2px solid var(--color-stroke-primary,#121212);content:'';display:block;margin-bottom:12px;margin-left:20px;margin-right:20px;}@media (min-width:740px){.css-17jkqqy:not(:empty):not(:first-child)::before{border-bottom:1px solid var(--color-stroke-primary,#121212);margin-bottom:1.25rem;margin-left:0;margin-right:0;}}.css-tlxejj{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(5,1fr);}.css-170wooj{margin:0;margin-bottom:0.25rem;}.css-125baf0{margin:0;margin-bottom:0.5rem;}.css-kuzbnk{min-width:0;position:relative;grid-column:span 5;margin-right:-1rem;-webkit-align-self:center;-ms-flex-item-align:center;align-self:center;}.css-kuzbnk:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-6l658f{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:2rem;line-height:1.15em;margin:0;color:var(--color-content-primary,#121212);font-weight:300;-webkit-font-smoothing:antialiased;margin-bottom:0.5rem;font-weight:300;}@media (min-width:481px){.css-6l658f{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.75rem;line-height:1.15em;}}.css-17sbsu2{color:var(--color-content-tertiary,#5A5A5A);font-family:nyt-imperial,georgia,'times new roman',times,Songti SC,simsun,serif;font-size:0.875rem;-webkit-letter-spacing:0.1px;-moz-letter-spacing:0.1px;-ms-letter-spacing:0.1px;letter-spacing:0.1px;line-height:1.1875rem;margin:0;padding:0;position:relative;-webkit-font-smoothing:auto;}.css-11uabb1{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-quaternary,#727272);margin-top:0.25rem;margin-bottom:0;}.css-50ivt8{min-width:0;position:relative;grid-column:span 3;margin-right:-1rem;}.css-50ivt8:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-2z4gz0{margin:0;font:700 1rem/1.15 nyt-karnak,georgia,'times new roman',times,serif;display:inline;-webkit-letter-spacing:0.25px;-moz-letter-spacing:0.25px;-ms-letter-spacing:0.25px;letter-spacing:0.25px;}@media (min-width:740px){.css-1ichrj1{position:relative;width:100vw;left:50%;right:50%;margin-left:-50vw;margin-right:-50vw;}}.css-1ichrj1::after{content:'';display:block;margin:20px 25px 0;}@media (min-width:740px){.css-1ichrj1::after{margin:0;}}.css-8ecw4e{background-color:var(--color-background-secondary,#F8F8F8);border-top:1px solid var(--color-stroke-quaternary,#DFDFDF);border-bottom:1px solid var(--color-stroke-quaternary,#DFDFDF);padding-bottom:16px;min-height:250px;}@media (min-width:740px){.css-8ecw4e{padding-bottom:32px;padding-top:15px;padding-bottom:15px;border-top:0;}}.css-oi0jtw{display:none;border-bottom:1px solid #000000;padding-top:2px;padding-bottom:2px;}@media (min-width:1024px){.css-oi0jtw{display:block;}}.css-oi0jtw::after{content:'';border-bottom:1px double #000000;display:block;}.css-77hcv{display:none;}.css-1qcrlqu{display:block;width:189px;height:26px;margin:4px auto 0;}@media (min-width:740px){.css-1qcrlqu{width:225px;height:31px;margin:3px auto 0;}}@media (min-width:1024px){.css-1qcrlqu{width:450px;height:61px;margin:10px auto 6px;}}@media (min-width:1070px){.css-1qcrlqu{width:485px;height:64px;}}.css-14m0cem{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;padding:0;}@media (min-width:1277px){.css-14m0cem{position:relative;}}@media (min-width:1277px){.css-14m0cem{position:static;}}.css-ljayxv{display:none;position:absolute;background-color:#ffffff;box-shadow:0 10px 10px rgba(0,0,0,0.1);left:0;top:100%;width:100vw;z-index:1000000002;}.css-ljayxv:first-child{display:block;}@media (max-width:1280px){.css-ljayxv{top:calc(100% - 4px);}}@media (min-width:1278px){.css-ljayxv{left:calc(-1 * (100vw - 1200px) / 2);}}@media (min-width:1278px){.css-ljayxv{left:0;}}.css-13qj3r5{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(20,1fr);margin:0 auto;max-width:1200px;width:94%;padding:1.5rem 0 2.5rem;color:var(--color-content-primary,#121212);font-family:nyt-franklin,helvetica,arial,sans-serif;font-weight:500;font-size:0.8125rem;line-height:1.125rem;white-space:normal;border-top:1px solid #ebebeb;}.css-r7t1h4{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;grid-column:span 2;}.css-132p2yg{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;grid-column:span 4;}.css-1didtc4{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;display:none;}.css-1xpp3bj{font-weight:700;color:var(--color-content-primary,#121212);}.css-1xpp3bj:hover{color:var(--color-content-tertiary,#5A5A5A);}.css-b3grxh{font-size:0.6875rem;line-height:1.125rem;color:var(--color-content-tertiary,#5A5A5A);margin-left:40px;}.css-b3grxh:hover{-webkit-text-decoration:underline;text-decoration:underline;color:var(--color-content-quaternary,#727272);}.css-167b3qw{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;grid-column:span 1;}.css-r6g2pn{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;grid-column:span 5;}.css-1qlpcok{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;display:inline-block;-webkit-columns:2;columns:2;margin-right:0;}.css-1qlpcok a{display:inline-block;}.css-dd9fkn{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;grid-column:span 3;}.css-9jpfr0{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;grid-column:span 9;}.css-34zueq{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;display:inline-block;-webkit-columns:3;columns:3;margin-right:50px;}.css-34zueq a{display:inline-block;}.css-15iksc8{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-flex-direction:column;-ms-flex-direction:column;flex-direction:column;grid-column:span 6;}.css-1q7rq5u{font-size:0.6875rem;line-height:1.125rem;color:var(--color-content-tertiary,#5A5A5A);margin-left:0;margin-top:1rem;}.css-1q7rq5u:hover{-webkit-text-decoration:underline;text-decoration:underline;color:var(--color-content-quaternary,#727272);}.css-c38y3b{font-size:0.6875rem;line-height:1.125rem;color:var(--color-content-tertiary,#5A5A5A);margin-top:1rem;width:-webkit-fit-content;width:-moz-fit-content;width:fit-content;}.css-c38y3b:hover{-webkit-text-decoration:underline;text-decoration:underline;color:var(--color-content-quaternary,#727272);}.css-hcfrni{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;margin-bottom:1.25rem;color:var(--color-content-secondary,#363636);cursor:pointer;}.css-hcfrni:hover .e10k0zts0{color:var(--color-content-tertiary,#5A5A5A);}.css-hcfrni img{width:30px;height:30px;margin-right:0.625rem;margin-top:3px;object-fit:content-cover;}.css-hcfrni img{height:56px;}@media (min-width:1024px){.css-hcfrni img{height:37px;}}.css-hmsvwu:not(:empty){padding-top:1.25rem;padding-bottom:2.5rem;}@media (min-width:740px){.css-hmsvwu:not(:empty){padding-top:1.25rem;}}.css-hmsvwu:not(:empty) .e1ppw5w20:last-child{padding-bottom:0;}.css-m5ahyg{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(20,1fr);}.css-4blv3u{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:2rem;line-height:1.15em;margin:0;color:var(--color-content-primary,#121212);font-weight:200;-webkit-font-smoothing:antialiased;}@media (min-width:481px){.css-4blv3u{font-family:nyt-cheltenham,cheltenham-fallback-georgia,cheltenham-fallback-noto,georgia,'times new roman',times,serif;font-size:1.5rem;line-height:1.15em;font-weight:300;}}.css-1bjokvb{min-width:0;position:relative;grid-column:span 6;}.css-1bjokvb:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-tertiary,#C7C7C7);}.css-ztw7vs{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-auto-columns:1fr;grid-auto-flow:column;grid-template-columns:repeat(4,calc(50% - 17px));-webkit-transform:translateX(0%) translateX(0px);-ms-transform:translateX(0%) translateX(0px);transform:translateX(0%) translateX(0px);-webkit-transition:0.3s cubic-bezier(0.49,0.49,0.55,0.95);transition:0.3s cubic-bezier(0.49,0.49,0.55,0.95);}.css-m3y3ka{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:end;-webkit-justify-content:flex-end;-ms-flex-pack:end;justify-content:flex-end;padding:1px;margin-top:0.75rem;}.css-6gfk0j{background-color:transparent;display:inline-block;width:26px;height:26px;-webkit-align-self:center;-ms-flex-item-align:center;align-self:center;border:0;right:0;border-radius:50%;padding:0;fill:var(--color-background-primary,#FFFFFF);stroke:var(--color-stroke-primary,#121212);-webkit-transform:rotate(180deg);-ms-transform:rotate(180deg);transform:rotate(180deg);left:0;margin-right:10px;}.css-6gfk0j:disabled svg{stroke:var(--color-stroke-quaternary,#DFDFDF);}.css-6gfk0j svg{width:100%;height:100%;cursor:pointer;-webkit-transition:fill 0.2s,stroke 0.2s;transition:fill 0.2s,stroke 0.2s;}.css-6gfk0j:hover:enabled svg{fill:var(--color-stroke-quaternary,#DFDFDF);}.css-1debogi{margin:0;margin-top:0.5rem;margin-bottom:0;}.css-cephuo{width:calc(50% - 16px);float:left;margin-left:16px;float:right;margin-left:16px;}.css-hf5jg6{font-family:nyt-franklin,helvetica,arial,sans-serif;font-size:0.625rem;line-height:1.25em;-webkit-letter-spacing:0.1em;-moz-letter-spacing:0.1em;-ms-letter-spacing:0.1em;letter-spacing:0.1em;text-transform:uppercase;margin:0;color:var(--color-content-quaternary,#727272);margin-top:0.25rem;margin-bottom:0;width:calc(50%);}.css-fyro28{overflow:hidden;margin-left:-33px;}.css-6w67yl{-webkit-transition:0.3s cubic-bezier(.49,.49,.55,.95);transition:0.3s cubic-bezier(.49,.49,.55,.95);display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;overflow:hidden;width:400%;}.css-n3ncu4{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-auto-columns:1fr;grid-auto-flow:column;grid-template-columns:repeat(5,calc(20% - 26.6px));-webkit-transform:translateX(0%) translateX(0px);-ms-transform:translateX(0%) translateX(0px);transform:translateX(0%) translateX(0px);-webkit-transition:0.3s cubic-bezier(0.49,0.49,0.55,0.95);transition:0.3s cubic-bezier(0.49,0.49,0.55,0.95);}.css-fi9dry{display:grid;grid-column-gap:calc(1rem * 2 + 1px);grid-template-columns:repeat(4,1fr);grid-column-gap:calc(1rem - 1px);}.css-14ywp0y{min-width:0;position:relative;grid-column:span 20;}.css-14ywp0y:not(:first-child)::before{content:'';height:100%;position:absolute;left:calc(-1rem - 1px);bottom:0;border-left:1px solid var(--color-stroke-quaternary,#DFDFDF);}.css-1nkoa58{-webkit-flex-basis:calc(33% - 19px);-ms-flex-preferred-size:calc(33% - 19px);flex-basis:calc(33% - 19px);margin-left:31px;margin-bottom:30px;min-height:0;min-width:0;}.css-1nkoa58:nth-child(3n + 1){margin-left:0;}@media (min-width:1069.99999px){.css-1nkoa58{-webkit-flex-basis:calc(25% - 24px);-ms-flex-preferred-size:calc(25% - 24px);flex-basis:calc(25% - 24px);}.css-1nkoa58:nth-child(3n + 1){margin-left:31px;}.css-1nkoa58:nth-child(4n + 1){margin-left:0;}}@media (min-width:1070px){.css-1nkoa58{-webkit-flex-basis:calc(20% - 25px);-ms-flex-preferred-size:calc(20% - 25px);flex-basis:calc(20% - 25px);}.css-1nkoa58:nth-child(4n + 1){margin-left:31px;}.css-1nkoa58:nth-child(5n + 1){margin-left:0;}}@media (min-width:740px){.css-1nkoa58:nth-child(4),.css-1nkoa58:nth-child(5){display:none;}}@media (min-width:1069.99999px){.css-1nkoa58:nth-child(4){display:block;}}@media (min-width:1070px){.css-1nkoa58:nth-child(5){display:block;}}.css-nn5e6h{-webkit-flex:0 0 91px;-ms-flex:0 0 91px;flex:0 0 91px;width:91px;margin-right:15px;}.css-ha69tt{display:grid;gap:4px;margin:0;grid-template-columns:repeat(2,minmax(0,1fr));}@media (min-width:600px){.css-ha69tt{height:auto;margin:0 auto;gap:4px;}}</style>
<script type="text/javascript">
(function(e,t){function n(){if(!o()&&r()){var n=document.createElement("script");n.id="nyt-fides",n.src=e,t.document.head.appendChild(n)}}var a=new URLSearchParams(t.location.search),i=function(e){return(t.document.cookie.split(";").find((function(t){return t.includes(e)}))||"").replace(e,"").trim().charAt(16)},r=function(){var e,n,r,o=a.get("fides-override"),l=i("nyt-purr="),s=i("override-purr=");return 0<(null===(e=t.config)||void 0===e||null===(n=e.tc_info)||void 0===n||null===(r=n.fides_string)||void 0===r?void 0:r.length)||"s"===s||"s"===l||"true"===o},o=function(){return null!==t.document.getElementById("nyt-fides")};n(),t.addEventListener("initWebview:ios",n),t.fidesUtils={isFidesEligible:r}})('https://static01.nyt.com/vi-assets/static-assets/fides-8ff3b866ef707d7b05879c1b02bc31aa.js', window);
(function(e){function t(e){var t=e.layerType;return{event:"impression",priority:!0,module:{name:t,label:t,region:"bottom"}}}function n(t){(e.dataLayer||(e.dataLayer=[])).push(t)}function a(t){var n=e.document.getElementById("fides-banner"),a=e.document.getElementById("fides-modal"),i=e.document.getElementById("fides-embed-container"),r="true"===(null==a?void 0:a.getAttribute("aria-hidden"));if(i)return"cmp_layer_2";if(null!==t){if(!n||!a)return"";if(n.contains(t))return"cmp_layer_1";if(a.contains(t))return"cmp_layer_2"}return r?"cmp_layer_1":"cmp_layer_2"}function i(t){var n=new CustomEvent("NYTFidesUpdated",{detail:t});e.dispatchEvent(n)}var r;!0===(null===(r=e.fidesUtils)||void 0===r?void 0:r.isFidesEligible())&&(e.addEventListener("FidesUIShown",(function(e){var a,i=(null==e||null===(a=e.detail)||void 0===a?void 0:a.extraDetails).servingComponent;"tcf_banner"===(void 0===i?"":i)&&n(t({layerType:"cmp_layer_1"}))})),e.addEventListener("click",(function(e){var t=e.target,r="button"===t.type,o="a"===t.nodeName.toLowerCase();if(r||o){var l=function(e){return{"accept all":"accept_all","reject all":"reject_all","manage preferences":"manage_prefs","cookie policy":"cookie_policy","privacy policy":"privacy_policy",purposes:"purposes",features:"features",vendors:"vendors",save:"save"}[e.textContent.toLowerCase()]||""}(t),s=a(t);if(l)n(function(e){var t=e.actionType,n=void 0===t?"":t,a=e.layerType,i=void 0===a?"":a;return{event:"moduleInteraction",eventData:{trigger:"module",type:"click"},module:{name:i,label:i,element:{name:n,label:n}}}}({actionType:l,layerType:s})),i(function(e){var t=e.actionType,n=void 0===t?"":t,a=e.layerType,i=void 0===a?"":a;return{subject:"interaction",data:{name:i,label:i,element:{name:n,label:n}}}}({actionType:l,layerType:s})),e.stopPropagation()}})),e.addEventListener("beforeunload",(function(){var e=a(null);n(t({layerType:"".concat(e,"_exit")}))})))})(window);
</script>
<script type="text/javascript">
// 36.535000000000004kB
window.viHeadScriptSize = 36.535000000000004;
window.NYTD = {};
window.vi = window.vi || {};
window.vi.pageType = { type: 'Homepage', edge: 'vi-homepage'};
(function () { var userAgent=window.navigator.userAgent||window.navigator.vendor||window.opera||"",inNewsreaderApp=userAgent.includes("nytios")||userAgent.includes("nyt_android"),inXWordsApp=userAgent.includes("nyt_xwords_ios")||userAgent.includes("Crosswords"),inAndroid=userAgent.includes("nyt_android")||userAgent.includes("Crosswords"),iniOS=userAgent.includes("nytios")||userAgent.includes("nyt_xwords_ios"),isInWebviewByUserAgent=(inAndroid||iniOS)&&(inNewsreaderApp||inXWordsApp);function appType(){return inNewsreaderApp?"newsreader":inXWordsApp?"crosswords":""}function deviceType(){return inAndroid?"ANDROID":iniOS?"IOS":""}var _f=function(e){window.vi.webviewEnvironment={appType:appType(),deviceType:deviceType(),isInWebview:e.webviewEnvironment.isInWebview||isInWebviewByUserAgent,isPreloaded:e.webviewEnvironment.isPreloaded}};;_f.apply(null, [{"gqlUrlClient":"https://samizdat-graphql.nytimes.com/graphql/v2","gqlRequestHeaders":{"nyt-app-type":"project-vi","nyt-app-version":"0.0.5","nyt-token":"MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAs+/oUCTBmD/cLdmcecrnBMHiU/pxQCn2DDyaPKUOXxi4p0uUSZQzsuq1pJ1m5z1i0YGPd1U1OeGHAChWtqoxC7bFMCXcwnE1oyui9G1uobgpm1GdhtwkR7ta7akVTcsF8zxiXx7DNXIPd2nIJFH83rmkZueKrC4JVaNzjvD+Z03piLn5bHWU6+w+rA+kyJtGgZNTXKyPh6EC6o5N+rknNMG5+CdTq35p8f99WjFawSvYgP9V64kgckbTbtdJ6YhVP58TnuYgr12urtwnIqWP9KSJ1e5vmgf3tunMqWNm6+AnsqNj8mCLdCuc5cEB74CwUeQcP2HQQmbCddBy2y0mEwIDAQAB","x-nyt-programming-abtest":".ver=23710.000","x-nyt-targeting-dimensions-map":"newsTenure=anon_user,agent_id=uUPwe3rT6adYXJo-C9KZFi,regi_id"},"gqlFetchTimeout":1500,"disablePersistedQueries":false,"initialDeviceType":"desktop","fastlyAbraConfig":{".ver":"23577.000","AMS_FrictionCircumventionDesktop_cwv":"2_low-mid-truncation","AMS_FrictionCircumventionMobile_cwv":"2_low-mid-truncation","DFP_MediaNet_0123":"","HOME_cwv_chartbeat":"0_Control",".programming_ver":"23710.000"},"fastlyEntitlements":[],"internalPreviewConfig":{},"webviewEnvironment":{"isInWebview":false,"isPreloaded":false},"isOptimisticallyTruncated":false,"optimisticTruncationDropzone":6,"requestPath":"/","isProvisionallyLoggedIn":false,"routeName":"homepage","serviceWorkerFile":"service-worker-test-1740698352070.js","preloadedServiceWorkerFile":"/vi-assets/static-assets/preloaded-service-worker-1740698539526.js"}]); })();;(function () { var _f=function(i,w){window.vi=window.vi||{},window.vi.env=Object.freeze(i),window.hybrid=w};;_f.apply(null, [{"JKIDD_PATH":"https://a.nytimes.com/svc/nyt/data-layer","ET2_URL":"https://a.et.nytimes.com","ALS_URL":"https://als-svc.nytimes.com","WEDDINGS_PATH":"https://content.api.nytimes.com","GDPR_PATH":"https://us-central1-nyt-dsra-prd.cloudfunctions.net/datagov-dsr-formhandler","RECAPTCHA_SITEKEY":"6LevSGcUAAAAAF-7fVZF05VTRiXvBDAY4vBSPaTF","ABRA_ET_URL":"//et.nytimes.com","NODE_ENV":"production","EXPERIMENTAL_ROUTE_PREFIX":"","ENVIRONMENT":"prd","RELEASE":"a33e3b3f2d9932550881a21bdebe3aaec6164b65","RELEASE_TAG":"","AUTH_HOST":"https://myaccount.nytimes.com","METER_HOST":"https://meter-svc.nytimes.com","MESSAGING_LANDING_PAGE_HOST":"https://www.nytimes.com","CAPI_HOST":"https://mwcm.nytimes.com","SWG_PUBLICATION_ID":"nytimes.com","GQL_FETCH_TIMEOUT":"1500","GOOGLE_CLIENT_ID":"1005640118348-amh5tgkq641oru4fbhr3psm3gt2tcc94.apps.googleusercontent.com","STORY_SURROGATE_CONTROL":"max-age=300, stale-if-error=259200, stale-while-revalidate=259200","ONBOARDING_API_KEY":"","PURR_ENV":"prd"},false]); })();;(function () { var _f=function(){var e=window;e.initWebview=function(e){var t=document.documentElement;if(e.OS){var i=e.OS.toUpperCase();t.classList.add(i)}if(e.BaseFontSize&&(t.dataset.baseFontSize=e.BaseFontSize),e.trackingSensitive&&(t.dataset.trackingSensitive=!0),e.hasOptedOutOfTracking&&(t.dataset.hasOptedOutOfTracking=!0),e.PurrDirectives){var a=e.PurrDirectives.PURR_AdConfiguration_v3||e.PurrDirectives.PURR_AdConfiguration_v2;a&&(t.dataset.optedOutOfAds=["adluce","adluce-socrates"].includes(a))}e.OS&&"IOS"===e.OS.toUpperCase()&&window.dispatchEvent(new CustomEvent("initWebview:ios",{detail:e}))};var t=e.navigator.userAgent.toLowerCase();/iphone|ipod|ipad/.test(t)||void 0===e.config||e.initWebview(e.config)};;_f.apply(null, []); })();;
(()=>{var Q=1e3,b="pageViewID",Y="getPageViewID",h="contextID",Z="getContextID",ee="NativeBridge unavailable.";async function X(e){if(!T())return Promise.reject("not in webview");try{await U();let t={};return await Promise.all([L(b,Y,e),L(h,Z,e)]).then(o=>{o.forEach(a=>{a.name==b?t[b]=a.id:t[h]=a.id})}),Promise.resolve(t)}catch(t){return Promise.reject(t)}}function T(){if(window?.vi?.webviewEnvironment!==void 0)return window.vi.webviewEnvironment.isInWebview&&window.vi.webviewEnvironment.deviceType=="IOS";if(window?.navigator?.userAgent!==void 0){for(var e=["nytios"],t=0;t<e.length;t++)if(window.navigator.userAgent.includes(e[t]))return!0}return!1}function J(){var e={subject:"page_update",assetUrl:(document.querySelector("link[rel=canonical]")||{}).href,assetId:(document.querySelector("meta[name=articleid]")||{}).content,nyt_uri:(document.querySelector("meta[name=nyt_uri]")||{}).content,url:location.href,client_tz_offset:new Date().getTimezoneOffset(),sourceApp:(document.querySelector("meta[name=sourceApp]")||{}).content||"nytcooking"};window.nyt_et("send",e)}function te(e,t){window.NativeBridge.addEventListener(e+"DidChange",function(o){t(e,o)})}async function L(e,t,o){return window.NativeBridge.callNativeBridgeCommand(t).then(a=>{let _=a.values[e];return te(e,o),Promise.resolve({name:e,id:_})})}async function U(e=0){return window.NativeBridge?Promise.resolve():e>=Q?Promise.reject(ee):new Promise((t,o)=>{setTimeout(function(){return U(e+1).then(t).catch(o)},10)})}function z(e){return!!(Object.keys(e).length==2&&e.hasOwnProperty("gtm")&&e.hasOwnProperty("activeTime")||e.hasOwnProperty("eventData")&&Object.keys(e.eventData).length==2&&e.eventData.hasOwnProperty("gtm")&&e.eventData.hasOwnProperty("activeTime"))}function D(){var e,t,o=window.crypto||window.msCrypto;if(o)t=o.getRandomValues(new Uint8Array(18));else for(t=[];t.length<18;)t.push(Math.random()*256^(e=e||+new Date)&255),e=Math.floor(e/256);return btoa(String.fromCharCode.apply(String,t)).replace(/\+/g,"-").replace(/\//g,"_")}function F(e,t){var o="",a="",_=!1,c=!1,l=!1,g=!1;if(typeof e=="string"&&e=="init"&&typeof t=="object"&&(_=!!t.intranet,c=!!t.force_xhr,l=!!t.store_last_response,g=!!t.use_webview_dedup_logic,typeof t.pv_id_override=="string"&&typeof t.ctx_id_override=="string"))if(t.pv_id_override.length>=24&&t.ctx_id_override.length>=24)o=t.pv_id_override,a=t.ctx_id_override;else try{console.warn("override id(s) must be >= 24 chars long")}catch{}return{pv_id:o,ctx_id:a,intra:_,store_last_response:l,force_xhr:c,use_webview_dedup_logic:g}}function $(e,t,o,a,_,c,l,g){if(!a&&(l==="beacon"||g&&o)){var f=window.navigator.sendBeacon(e,t);return _&&(last_send_response=f),f}else{var s=typeof XMLHttpRequest<"u"?new XMLHttpRequest:new ActiveXObject("Microsoft.XMLHTTP");return s.open("POST",e),s.withCredentials=!0,s.setRequestHeader("Accept","*/*"),typeof t=="string"?s.setRequestHeader("Content-Type","text/plain;charset=UTF-8"):{}.toString.call(t)==="[object Blob]"&&t.type&&s.setRequestHeader("Content-Type",t.type),c&&s.setRequestHeader("ETJS-Ignore-Session-Info","true"),_&&!s.onload&&(s.onload=function(){last_send_response=s.response},s.onerror=function(P){last_send_response=!1}),s.send(t),!0}}(function(e,t){if(e.nyt_et){console.warn("et2 snippet should only load once per page");return}var o,a,_,c,l=0,g,f=[],s,P,p={pv_id:"",ctx_id:"",intra:!1,force_xhr:!1,store_last_response:!1,use_webview_dedup_logic:!1},B=typeof e.navigator=="object"&&typeof e.navigator.userAgent=="string"&&/iP(ad|hone|od)/.test(e.navigator.userAgent),O=typeof e.navigator=="object"&&e.navigator.sendBeacon,C=O?B?"xhr_ios":"beacon":"xhr",u=T(),d="",m="",S=0;function K(n,i){if(n!==b&&n!==h){console.error("syncID got unknown id="+n);return}if(!(!i||!i.detail)){var r=i.detail[n]||i.detail.newID;r&&(n===b?d=r:m=r,J())}}function A(n){if(f.length!==0){if(u&&(d===void 0||d==="")){console.log("ERROR: in webview attempting to transmit() but native_pvid not set. Doing nothing...");return}$(a,JSON.stringify(f),n,p.force_xhr,p.store_last_response,u,C,O),f.length=0,clearTimeout(s),s=null}}function x(n){var i,r,v,w,R,H,y=n[0];if(typeof y=="string"&&/init/.test(y)&&(""+JSON.stringify(n),H=!0,p=F(y,n[3]),c=p.pv_id||D(),l+=1,y=="init"&&!_)){if(_=p.ctx_id||D(),typeof n[1]=="string"&&/^http/.test(n[1]))o=String(n[1]).replace(/([^\/])$/,"$1/"),a=o+"track";else throw new Error("init must include an et host url");if(typeof n[2]=="string")g=n[2];else throw new Error("init must include a source app name")}var N=n.length-1;if(n[N]&&typeof n[N]=="object"&&(i=n[N]),!i&&!/init/.test(y)?console.warn("when invoked without 'init' or 'pageinit', nyt_et() must include a event data"):i&&!i.subject&&console.warn("event data {} must include a subject"),!o||!(i&&i.subject)){console.log("ERROR: data must contain subject and et_base_url must be set");return}let j=l==1;var V=!1,I=p.use_webview_dedup_logic;if(u&&p.use_webview_dedup_logic&&H&&j&&i.subject=="page"&&(i.subject="page_update",V=!0),r=i.subject,delete i.subject,w=r=="page_exit"||(i.eventData||{}).type=="ob_click",R=r=="page_update",I&&u&&w&&(r="page_update",w=!1,R=!0),I&&u&&R&&z(i)){S+=1;return}r=="page"||r=="page_soft"?v=c:v=D();let W=c,G=_;I&&u&&j&&(W=d,G=m),f.push({context_id:G,pageview_id:W,event_id:v,client_lib:t,sourceApp:g,intranet:p.intra?1:void 0,subject:r,how:w&&B&&O?"beacon_ios":C,client_ts:+new Date,data:JSON.parse(JSON.stringify(i))}),y=="send"||v==c||w||V?A(w):(y=="soon"&&(clearTimeout(s),s=setTimeout(A,200)),s||(s=setTimeout(A,5500)))}e.nyt_et=function(){var n=arguments;if(u&&d===""){e.nyt_et_buffer=e.nyt_et_buffer||[],e.nyt_et_buffer.push(n);return}x(n)};var k=!1;function M(){if(u&&d===""){return}if(k){q(e.nyt_et_buffer);return}let n=e.nyt_et_buffer;e.nyt_et_buffer=e.nyt_et_buffer||[],q(n),e.nyt_et_buffer.push=new Proxy(e.nyt_et_buffer.push,{apply:function(i,r,v){return console.table("calling window.nyt_et",r,v),x(v),i.apply(r,v)}}),k=!0}M();var E=0;function q(n=[]){if(!g){let i=n?.findIndex(r=>typeof r[0]=="string"&&r[0]==="init");""+i.toString(),i>-1&&x(n[i])}""+E;for(let i=E;i<n.length;i++){let r=n[i];""+E+i,typeof r[0]=="string"&&r[0]!=="init"&&(console.log("non-init event at pos="+i.toString()),x(r)),E++}}e.nyt_et.get_pageview_id=function(){return p.use_webview_dedup_logic&&u&&l>=1?d:c},e.nyt_et.get_context_id=function(){return p.use_webview_dedup_logic&&u&&l>=1?m:_},e.nyt_et.get_host=function(){return o},e.nyt_et.is_running_in_webview=function(){return u},e.nyt_et.get_native_pageview_id=function(){return d},e.nyt_et.get_native_context_id=function(){return m},e.nyt_et.get_buffer_len=function(){f.length},e.nyt_et.get_buffer=function(){return f},e.nyt_et.call_is_running_in_webview=function(){return T()},e.nyt_et.get_last_send_response=function(){var n=P;return n&&(P=null),n},e.nyt_et.get_num_blocked_heartbeats=function(){return S},e.nyt_et.reset_blocked_heartbeat_counts=function(){S=0},u&&X(K).then(n=>{m=n[h],d=n[b],M()}).catch(console.error).finally(console.log)})(window,"v1.4.0");})();
;(function c(e){var t,n=(null===(t=e=e||("undefined"!=typeof window?window:void 0))||void 0===t?void 0:t.UnifiedTracking)||{};return n.context="web",e?(e.UnifiedTracking=n,n.sendAnalytic=function(t,n){return e.dataLayer=e.dataLayer||[],Array.isArray(e.dataLayer)&&(n.event=n.event||t,e.dataLayer.push(n)),Promise.resolve({success:!0})},n):n})(window);!function(r){var n,t;r=r||self,n=r.Abra,(t=r.Abra=function(){"use strict";var r=Array.isArray,n=function(r,n,t){var e=r(t,n),u=e[0],o=e[1];if(null==u||""===u)return n;for(var i=String(u).split("."),a=0;a<i.length&&(n=n[i[a]]);a++);return null==n&&(n=o),null!=n?n:null},t=function(r,n,t){return r(t,n).reduce((function(r,n){return parseFloat(r)+parseFloat(n)}),0)},e=function(r,n,t){var e=r(t,n);return e[0]/e[1]},u=function(r,n,t){var e=r(t,n);return e[0]%e[1]},o=function(r,n,t){return r(t,n).reduce((function(r,n){return parseFloat(r)*parseFloat(n)}),1)},i=function(r,n,t){var e=r(t,n),u=e[0],o=e[1];return void 0===o?-u:u-o};function a(n){return!(r(n)&&0===n.length||!n)}var f=function(r,n,t){for(var e,u=0;u<t.length;u++)if(!a(e=r(t[u],n)))return e;return e},c=function(r,n,t){var e;for(e=0;e<t.length-1;e+=2)if(a(r(t[e],n)))return r(t[e+1],n);return t.length===e+1?r(t[e],n):null},l=function(r,n,t){return!a(r(t,n)[0])},v=function(r,n,t){for(var e,u=0;u<t.length;u++)if(a(e=r(t[u],n)))return e;return e},d=function(r,n,t){var e=r(t,n);return e[0]===e[1]},s=function(r,n,t){var e=r(t,n);return e[0]!==e[1]},h=function(r,n,t){var e=r(t,n),u=e[0],o=e[1];return!(!o||void 0===o.indexOf)&&-1!==o.indexOf(u)},g=function(r,n,t){var e=r(t,n);return e[0]>e[1]},p=function(r,n,t){var e=r(t,n);return e[0]>=e[1]},b=function(r,n,t){var e=r(t,n),u=e[0],o=e[1],i=e[2];return void 0===i?u<o:u<o&&o<i},w=function(r,n,t){var e=r(t,n),u=e[0],o=e[1],i=e[2];return void 0===i?u<=o:u<=o&&o<=i},y=function(r,n,t){var e=t[0],u=t[1],o=t.slice(2),i=r(e,n);if(!i)return null;if(0===o.length)return null;if(1===o.length)return r(o[0],n);if(4294967295===o[0])return r(o[1],n);for(var a=function(r){var n,t,e,u,o,i=[],a=[t=1732584193,e=4023233417,~t,~e,3285377520],f=[],c=unescape(encodeURI(r))+"\x80",l=c.length;for(f[r=--l/4+2|15]=8*l;~l;)f[l>>2]|=c.charCodeAt(l)<<8*~l--;for(n=l=0;n<r;n+=16){for(t=a;l<80;t=[t[4]+(i[l]=l<16?~~f[n+l]:2*c|c<0)+1518500249+[e&u|~e&o,c=341275144+(e^u^o),882459459+(e&u|e&o|u&o),c+1535694389][l++/5>>2]+((c=t[0])<<5|c>>>27),c,e<<30|e>>>2,u,o])c=i[l-3]^i[l-8]^i[l-14]^i[l-16],e=t[1],u=t[2],o=t[3];for(l=5;l;)a[--l]+=t[l]}return a[0]>>>0}(i+" "+r(u,n));o.length>1;){var f=o.splice(0,2),c=f[0],l=f[1];if(a<=r(c,n))return r(l,n)}return 0===o.length?null:r(o[0],n)},k=function(r,n,t){var e=t[0],u=t[1],o=r(e,n);return null==o?null:new RegExp(u).test(o)};return function(a,m,O,A){void 0===a&&(a={}),void 0===m&&(m={}),void 0===O&&(O={}),void 0===A&&(A=!1);var j=function(){var r={},n=function(n){if(n)for(var t,e=decodeURIComponent(n[1]),u=/(?:^|,)([^,=]+)=([^,]*)/g;t=u.exec(e);){var o=t,i=o[1],a=o[2];r[i]=a||null}};n(document.cookie.match(/(?:^|;) *abra-overrides=([^;]+)/)),n(window.location.search.match(/(?:\?|&)abra-overrides=([^&]+)/));var t=/(?:^|;) *abra-nuke=true(?:;|$)/.test(document.cookie)||/(?:\?|&)abra-nuke=true(?:&|$)/.test(window.location.search);return[r,t]}(),x=j[0],E=j[1];Object.keys(O).forEach((function(r){x[r]=O[r]}));var F,C=A||E,R=(F={var:n,if:c,"===":d,"!==":s,and:f,or:v,"!":l,">":g,">=":p,"<":b,"<=":w,"+":t,"-":i,"*":o,"/":e,"%":u,in:h,abtest_partition:y,regex_match:k,ref:function(r,n,t){var e=r(t,n)[0];return U(e)}},function n(t,e){if(e||(e={}),r(t))return t.map((function(r){return n(r,e)}));if(!function(n){return"object"==typeof n&&null!==n&&!r(n)&&1===Object.keys(n).length}(t))return t;var u=function(r){return Object.keys(r)[0]}(t),o=t[u];r(o)||(o=[o]);var i=F[u];if(!i)throw new Error("Unrecognized operation "+u);return i(n,e,o)}),U=function(r){if(!r)return null;var n=x[r];if(void 0===n){if(!C){if(Object.prototype.hasOwnProperty.call(x,r))throw new Error("circular logic");x[r]=void 0,n=R(a[r],m)}void 0===n&&(n=null),x[r]=n}return n};return U}}()).noConflict=function(){return r.Abra=n,t}}(this);
;(function () { var NYTD="undefined"!=typeof window&&window.NYTD?window.NYTD:{};function setupTimeZone(){var e='[data-timezone][data-timezone~="'+(new Date).getHours()+'"] { display: block }',i=document.createElement("style");i.innerHTML=e,document.head.appendChild(i)}function addNYTAppClass(){var e=window.vi&&window.vi.webviewEnvironment||{};e&&e.isInWebview&&document.documentElement.classList.add("NYTApp"),e&&e.isPreloaded&&document.documentElement.classList.add("NYTAppPreloaded"),e&&e.deviceType&&document.documentElement.classList.add(window.vi.webviewEnvironment.deviceType)}function addNYTPageTypeClass(){var e=window.vi.pageType.edge;e&&document.documentElement.classList.add("nytapp-"+e)}function isIOSNewsreaderWebview(){return void 0!==window.vi&&void 0!==window.vi.webviewEnvironment&&"IOS"===window.vi.webviewEnvironment.deviceType&&"newsreader"===window.vi.webviewEnvironment.appType}function shouldUseNativePvid(){return void 0!==window.vi&&void 0!==window.vi.webviewEnvironment&&window.vi.webviewEnvironment.isPreloaded||isIOSNewsreaderWebview()}function setNativePageViewId(e){return e?NYTD.PageViewId.current=e:isIOSNewsreaderWebview()?window.NativeBridge.getPageViewID().then(function(e){window.NYTD=window.NYTD||{},window.NYTD.PageViewId=window.NYTD.PageViewId||{},e&&e.values&&e.values.pageViewID?NYTD.PageViewId.current=e.values.pageViewID:NYTD.PageViewId.current="native-bridge-pageview_id-undefined"}).catch(function(){NYTD.PageViewId.current="native-bridge-getpageviewid-catch"}):window.config&&window.config.AdRequirements&&window.config.AdRequirements.page_view_id?NYTD.PageViewId.current=window.config.AdRequirements.page_view_id:NYTD.PageViewId.current="native-pageview_id-unavailable",NYTD.PageViewId.current}function forceNativePageViewIdUpdate(e){setNativePageViewId(e),window.AdSlot4&&window.AdSlot4.updateAdReq&&window.AdSlot4.updateAdReq({page_view_id:e})}function handlePvidDidChange(e){forceNativePageViewIdUpdate(e&&e.detail&&(e.detail.pageViewID||e.detail.newID))}function setupPageViewId(){NYTD.PageViewId={},NYTD.PageViewId.update=function(e){return e?NYTD.PageViewId.current=e:shouldUseNativePvid()?setNativePageViewId():"undefined"!=typeof nyt_et&&"function"==typeof window.nyt_et.get_pageview_id?(window.nyt_et("pageinit"),NYTD.PageViewId.current=window.nyt_et.get_pageview_id()):NYTD.PageViewId.current="no-native-bridge-nor-nyt_et",NYTD.PageViewId.current},shouldUseNativePvid()&&(isIOSNewsreaderWebview()?window.NativeBridge.addEventListener("pageViewIDDidChange",handlePvidDidChange):window.updatePageViewID=forceNativePageViewIdUpdate)}function reRenderEvent(){window.dispatchEvent(new CustomEvent("rerender-programming",{detail:{viewport:window.innerWidth}}))}function setupResizeListeners(){var e=window.vi&&window.vi.webviewEnvironment||{},i=window.vi&&window.vi.pageType&&window.vi.pageType.edge;e&&e.isPreloaded&&"vi-homepage"===i&&(window.addEventListener("resize",function(){reRenderEvent("window.onresize callback")}),window.addEventListener("rerender-ready",function(){reRenderEvent("rerender-ready Custom Event callback")}))}var _f=function(){try{document.domain="nytimes.com"}catch(e){}window.swgUserInfoXhrObject=new XMLHttpRequest,setupPageViewId(),setupTimeZone(),addNYTAppClass(),addNYTPageTypeClass(),setupResizeListeners(),window.nyt_et&&"function"==typeof window.nyt_et&&window.nyt_et("init",vi.env.ET2_URL,"nyt-vi",{use_webview_dedup_logic:!0},{subject:"page",canonicalUrl:(document.querySelector("link[rel=canonical]")||{}).href,articleId:(document.querySelector("meta[name=articleid]")||{}).content,nyt_uri:(document.querySelector("meta[name=nyt_uri]")||{}).content,pubpEventId:(document.querySelector("meta[name=pubp_event_id]")||{}).content,url:location.href,referrer:document.referrer||void 0,client_tz_offset:(new Date).getTimezoneOffset()}),shouldUseNativePvid()?setNativePageViewId():"undefined"!=typeof nyt_et&&"function"==typeof window.nyt_et.get_pageview_id?NYTD.PageViewId.current=window.nyt_et.get_pageview_id()||"nyt_et-pageview_id-undefined":NYTD.PageViewId.update();var e=window.reportError;window.reportError=function(){var i=Array.prototype.slice.call(arguments),n=i[0];(n.message.includes("root will switch to client rendering.")||n.message.includes("Minified React error #423"))&&window.dispatchEvent(new CustomEvent("react-hydration-error")),e.apply(null,i)},window.NYTD=NYTD||{}};;_f.apply(null, []); })();;(function () { var NYTD="undefined"!=typeof window&&window.NYTD?window.NYTD:{};var _f=function(n){var e=window.vi&&window.vi.webviewEnvironment&&window.vi.webviewEnvironment.isPreloaded;function t(){if(window.Abra&&"function"==typeof window.Abra){NYTD.Abra=function(){var t=e?window.getNativeBridgeCookie("nyt-a"):(window.document.cookie.match(/(?:^|;) *nyt-a=([^;]*)/)||[])[1],o=[];window.dataLayer=window.dataLayer||[],c.config=n.abraConfig||{},c.reportedAllocations={},c.reportedExposures={};var a=(n.abraURL||"").match(/current[/]([a-zA-Z-]+).json/i);c.integration=a&&a.length>1?a[1]:"";try{c.version=window.Abra(c.config)(".ver")}catch(n){c.version=0}var i=c.config,r={agent_id:t},d=window.Abra(i,r);function c(n){return c.getAbraSync(n).variant}return c.getAbraSync=function(n){var e=c.reportedAllocations[n];if(void 0!==e)return{variant:e,allocated:!0};var t=null,o=!1;try{t=d(n),o=!0}catch(n){}return{variant:t,allocated:o}},c.reportExposure=function(n){var e=c.getAbraSync(n).variant;if(null!=e&&(void 0===c.reportedExposures[n]||e!==c.reportedExposures[n])){c.reportedExposures[n]=e;var t={event:"ab-expose",abtest:{test:n,variant:e,config_ver:c.version,integration:c.integration}};window.UnifiedTracking.sendAnalytic(t.event,t)}},c.alloc=function(){Object.keys(c.config).filter(function(n){return!n.includes(".")}).forEach(function(n){var e=c.getAbraSync(n);e.allocated&&(c.reportedAllocations[n]=e.variant,o.push({test:n,variant:e.variant}))});var n={event:"ab-alloc",abtest:{batch:o}};window.UnifiedTracking.sendAnalytic(n.event,n)},c.alloc(),c}();var t=window.NYTD.Abra.getAbraSync("SP_commentsRefactor_1224").variant;t&&document.documentElement.classList.add("SP_commentsRefactor_1224_"+t);var o=window.NYTD.Abra.getAbraSync("STCO_bookModule_0325").variant;o&&document.documentElement.classList.add("STCO_bookModule_0325_"+o),window.NYTD=NYTD||{}}}e&&!window.nativeBridgeCookies?window.initNativeBridgeCookies(t):t()};;_f.apply(null, [{"abraConfig":{".ver":23730,"UPSHOT_wordleStateV2_0306":{"abtest_partition":[{"var":"agent_id"},"UPSHOT_wordleStateV2_0306",4294967295,"0_Control"]},"UJ_ResurfaceRegiOnboarding_0924":{"if":[{"and":[{"!":{"in":[{"ref":"SUBX_regi_alloc_holdout_2024H1"},["0_holdout"]]}}]},{"abtest_partition":[{"var":"regi_id"},"UJ_ResurfaceRegiOnboarding_0924",107374181,"1_ResurfaceRegiOnboarding",214748364,"2_ResurfaceRegiOnboarding",2254857829,"1_ResurfaceRegiOnboarding",4294967295,"2_ResurfaceRegiOnboarding"]}]},"UJ_ResurfaceAAOnboarding_0924":{"if":[{"and":[{"!":{"in":[{"ref":"SUBX_regi_alloc_holdout_2024H1"},["0_holdout"]]}}]},{"abtest_partition":[{"var":"regi_id"},"49c3c84d-7935-4652-80a7-50bfc2aaee45",2147483647,"1_ResurfaceAAOnboarding",4294967295,"2_ResurfaceAAOnboarding"]}]},"SUBX_regi_alloc_holdout_2024H1":{"abtest_partition":[{"var":"regi_id"},"SUBX_regi_alloc_holdout_2024H1",214748364,"1_best_experience",429496729,"1_best_experience",4294967295,"1_best_experience"]},"SUBX_agent_alloc_holdout_2024H1":{"abtest_partition":[{"var":"agent_id"},"SUBX_agent_alloc_holdout_2024H1",214748364,"1_best_experience",429496729,"1_best_experience",4294967295,"1_best_experience"]},"SUBCON_RES_AIG_SURVEY_228":{"abtest_partition":[{"var":"agent_id"},"SUBCON_RES_AIG_SURVEY_228",4080218930,"0_Control",4294967295,"1_Variant"]},"STYLN_LIVE_USER_STATE_API_SWITCH":{"abtest_partition":[{"var":"agent_id"},"STYLN_LIVE_USER_STATE_API_SWITCH",4294967295,null]},"STCO_bookModule_0325":{"abtest_partition":[{"var":"regi_id"},"STCO_bookModule_0325",2147483647,"0_Control",4294967295,"1_TestVariant"]},"SP_ReqToComRollout_Web":{"abtest_partition":[{"var":"agent_id"},"SP_ReqToComRollout_Web",4080218930,"0_Off",4294967295,"1_On"]},"SP_commentsRefactor_1224":{"abtest_partition":[{"var":"agent_id"},"SP_commentsRefactor_1224",4294967295,"0_Control"]},"SJ_disrupter_V2_increased_cadence":{"if":[{"and":[{"!":{"in":[{"ref":"SUBX_regi_alloc_holdout_2024H1"},["0_holdout"]]}}]},{"abtest_partition":[{"var":"regi_id"},"SJ_disrupter_V2_increased_cadence",4294967295,"2_high_intensity"]}]},"SHA_LiveNativeShare_1024":{"abtest_partition":[{"var":"agent_id"},"SHA_LiveNativeShare_1024",4294967295,"1_Native"]},"SHA_cardShare_0424":{"abtest_partition":[{"var":"agent_id"},"SHA_cardShare_0424",4294967295,"1_Share"]},"SEO_spanishSearchTranslation_0824":{"abtest_partition":[{"var":"agent_id"},"SEO_spanishSearchTranslation_0824",4294967295,null]},"SEO_langInputSearchBar_0824":{"abtest_partition":[{"var":"agent_id"},"SEO_langInputSearchBar_0824",4294967295,"1_Rollout"]},"PERSX_electionNLSignup_0926":{"abtest_partition":[{"var":"agent_id"},"PERSX_electionNLSignup_0926",4294967295,"1_Variant"]},"ON_news_upsell_sale":{"abtest_partition":[{"var":"agent_id"},"ON_news_upsell_sale",3865470565,"1_news_upsell",4294967295,"1_news_upsell"]},"OMA_WEB_LIBRARY_OPS_HARDCODED_FALLBACK":{"abtest_partition":[{"var":"agent_id"},"OMA_WEB_LIBRARY_OPS_HARDCODED_FALLBACK",1073741823,null]},"OMA_TIMEOUTROLLOUT_062024":{"abtest_partition":[{"var":"agent_id"},"OMA_TIMEOUTROLLOUT_062024",4294967295,"on"]},"OMA_TermsOfService_0624":{"abtest_partition":[{"var":"agent_id"},"OMA_TermsOfService_0624",214748364,"1_Variant",429496729,"1_Variant",4294967295,"1_Variant"]},"OMA_SAMIZDAT_SELECTION_KILL_SWITCH":{"abtest_partition":[{"var":"agent_id"},"OMA_SAMIZDAT_SELECTION_KILL_SWITCH"]},"OMA_SAMIZDAT_OPS_KILL_SWITCH":{"abtest_partition":[{"var":"agent_id"},"OMA_SAMIZDAT_OPS_KILL_SWITCH"]},"OMA_SAMIZDAT_KILL_SWITCH":{"abtest_partition":[{"var":"agent_id"},"OMA_SAMIZDAT_KILL_SWITCH"]},"OMA_FEDERATED_QUERY":{"abtest_partition":[{"var":"agent_id"},"OMA_FEDERATED_QUERY",64424508,"on",85899345,"on",107374181,"on",128849018,"on",150323854,"on",171798691,"on",193273527,"on",214748364,"on",236223200,"on",257698037,"on",279172873,"on",300647710,"on",322122546,"on",343597383,"on",365072219,"on",386547056,"on",408021892,"on",429496729,"on",450971565,"on",472446402,"on",493921238,"on",515396075,"on",536870911,"on",558345747,"on",579820584,"on",601295420,"on",773094112,"on",1116691496,"on",1503238553,"on",1717986917,"on",1889785609,"on",2126008811,"on",4294967295,"on"]},"OMA_FASTLY_ROLLOUT_09_24":{"abtest_partition":[{"var":"agent_id"},"OMA_FASTLY_ROLLOUT_09_24",214748364,"1_fastly",429496729,"1_fastly",644245093,"1_fastly",858993458,"1_fastly",1288490188,"1_fastly",1717986917,"1_fastly",2576980377,"1_fastly",3435973836,"1_fastly",3865470565,"1_fastly",4294967295,"1_fastly"]},"OMA_DVSP_ROLLOUT":{"abtest_partition":[{"var":"agent_id"},"OMA_DVSP_ROLLOUT",4294967295,"on"]},"OMA_DISABLE_USER_STATE_HYBRID":{"abtest_partition":[{"var":"agent_id"},"OMA_DISABLE_USER_STATE_HYBRID",1073741823,"enabled",4294967295,"enabled"]},"MX_Turn_Off_CAPI_0324":{"abtest_partition":[{"var":"agent_id"},"MX_Turn_Off_CAPI_0324",4294967295,"0_no_capi"]},"MX_NewArchitecture_gateway":{"if":[{"and":[{"===":[{"ref":"MX_NewArchitecture_MeterReal"},"1_variant"]}]},{"abtest_partition":[{"var":"agent_id"},"MX_NewArchitecture_gateway",21474835,"1_variant",107374181,"1_variant",1073741823,"1_variant",1116691496,"1_variant",1159641169,"1_variant",1267015351,"1_variant",1503238553,"1_variant",1717986917,"1_variant",1932735282,"1_variant",2126008811,"1_variant",4294967295,"1_variant"]}]},"MX_FF_WELCOME_AD":{"abtest_partition":[{"var":"agent_id"},"MX_FF_WELCOME_AD",4294967295,"1_Variant"]},"MKT_first_party_data_survey_subs_dock":{"abtest_partition":[{"var":"regi_id"},"MKT_first_party_data_survey_subs_dock",2748779068,"0_control",2963527433,"1_survey",4294967295,"0_control"]},"MKT_first_party_data_survey_regi_dock":{"abtest_partition":[{"var":"regi_id"},"MKT_first_party_data_survey_regi_dock",3822520892,"0_control",3908420238,"1_survey",4294967295,"0_control"]},"MKT_first_party_data_survey":{"abtest_partition":[{"var":"agent_id"},"MKT_first_party_data_survey",2061584301,"0_control",2276332666,"1_survey",3650722201,"0_control",4294967295,"1_survey"]},"GUAC_GROWTH_EXISTING_SUBS_BAR1_SPLIT":{"abtest_partition":[{"var":"agent_id"},"84bd05a9-a853-4748-90ac-cefc321921e3",858993458,"v7",1288490188,"v7",4294967295,"v7"]},"DFP_Prebid_0624":{"abtest_partition":[{"var":"agent_id"},"DFP_Prebid_0624",4294967295,"1_Criteo"]},"CONV_TA_PLOPRO":{"abtest_partition":[{"var":"agent_id"},"7291d95b-8aed-444f-b38e-ff2c59dc2f00",1434519076,"0_control",2864743185,"2_value_cards",4294967295,"2_value_cards"]},"CONV_GUAC_PLOPRO_SLAYER_0224":{"abtest_partition":[{"var":"agent_id"},"CONV_GUAC_PLOPRO_SLAYER_0224",4294967295,"1_slayer"]},"CONV_GUAC_NewsPaywall_SLAYER_0324":{"abtest_partition":[{"var":"agent_id"},"CONV_GUAC_NewsPaywall_SLAYER_0324",4294967295,"1_Slayer"]},"CONV_GUAC_CKLP_ExpressCheckOut_RollOut_0124":{"abtest_partition":[{"var":"agent_id"},"CONV_GUAC_CKLP_ExpressCheckOut_RollOut_0124",4294967295,"1_expresscheckout"]},"CONV_GUAC_CK_AA_Intl_AnnualOffer_0524":{"abtest_partition":[{"var":"agent_id"},"CONV_GUAC_CK_AA_Intl_AnnualOffer_0524",4294967295,null]},"CONV_GUAC_AALP_SLAYER_0224":{"abtest_partition":[{"var":"agent_id"},"CONV_GUAC_AALP_SLAYER_0224",4294967295,"1_slayer"]},"CONV_GUAC_AALP_HDAnchor_Test_0424":{"abtest_partition":[{"var":"agent_id"},"CONV_GUAC_AALP_HDAnchor_Test_0424",1417339207,"1_anchor",4294967295,"1_anchor"]},"CONV_GUAC_AADock_SLAYER_0324":{"abtest_partition":[{"var":"regi_id"},"CONV_GUAC_AADock_SLAYER_0324",4294967295,"1_slayer"]},"CONV_GAMES_GUAC_PRO_REMOVED_TEST":{"abtest_partition":[{"var":"agent_id"},"70610249-c5a4-4001-9df1-a622b81db68a",4294967295,"1_pro_removed"]},"AMS_FrictionCircumventionMobile_cwv":{"abtest_partition":[{"var":"agent_id"},"AMS_FrictionCircumventionMobile_cwv",14315125,"2_low-mid-truncation",214726889,"2_low-mid-truncation",243357141,"2_low-mid-truncation",257672267,"2_low-mid-truncation",271987393,"2_low-mid-truncation",286302519,"2_low-mid-truncation",300617645,"2_low-mid-truncation",314932771,"2_low-mid-truncation",329247897,"2_low-mid-truncation",343563023,"2_low-mid-truncation",357878149,"2_low-mid-truncation",372193275,"2_low-mid-truncation",386508401,"2_low-mid-truncation",400823527,"2_low-mid-truncation",415138653,"2_low-mid-truncation",429453779,"2_low-mid-truncation",443768905,"2_low-mid-truncation",558289913,"2_low-mid-truncation",830277307,"2_low-mid-truncation",1259731087,"2_low-mid-truncation",4294967295,"2_low-mid-truncation"]},"AMS_FrictionCircumventionDesktop_cwv":{"abtest_partition":[{"var":"agent_id"},"AMS_FrictionCircumventionDesktop_cwv",14315125,"2_low-mid-truncation",214726889,"2_low-mid-truncation",243357141,"2_low-mid-truncation",257672267,"2_low-mid-truncation",271987393,"2_low-mid-truncation",286302519,"2_low-mid-truncation",300617645,"2_low-mid-truncation",314932771,"2_low-mid-truncation",329247897,"2_low-mid-truncation",343563023,"2_low-mid-truncation",357878149,"2_low-mid-truncation",372193275,"2_low-mid-truncation",386508401,"2_low-mid-truncation",400823527,"2_low-mid-truncation",415138653,"2_low-mid-truncation",429453779,"2_low-mid-truncation",443768905,"2_low-mid-truncation",458084031,"2_low-mid-truncation",644180669,"2_low-mid-truncation",844592433,"2_low-mid-truncation",1245415961,"2_low-mid-truncation",4294967295,"2_low-mid-truncation"]},"ACCT_PRODUCT_SWITCH_REDESIGN_DIRECT_TO_REVIEW_TEST":{"abtest_partition":[{"var":"regi_id"},"ACCT_PRODUCT_SWITCH_REDESIGN_DIRECT_TO_REVIEW_TEST",1431942095,"0_Control",2863454695,"1_No_AA_Merchandising_Card",4294967295,"2_AA_Merchandising_Card"]},"ACAC_SupergraphRollout":{"abtest_partition":[{"var":"agent_id"},"ACAC_SupergraphRollout",4294967295,"supergraph"]},"AA_OnboardingFlow_MVPFlowAppSequence_WebandApp_V1":{"if":[{"and":[{"regex_match":[{"var":"user_entitlements"},"(^|\\W)MM($|\\W)"]},{"regex_match":[{"var":"user_entitlements"},"(^|\\W)CKG($|\\W)"]},{"regex_match":[{"var":"user_entitlements"},"(^|\\W)ATH($|\\W)"]},{"regex_match":[{"var":"user_entitlements"},"(^|\\W)WC($|\\W)"]},{"regex_match":[{"var":"user_entitlements"},"(^|\\W)XWD($|\\W)"]},{"!":{"in":[{"ref":"SUBX_regi_alloc_holdout_2024H1"},["0_holdout"]]}},{"!==":[{"var":"app_version"},"10.44.0"]}]},{"abtest_partition":[{"var":"regi_id"},"AA_OnboardingFlow_MVPFlowAppSequence_WebandApp_V1",773094112,"2_appLater",1275605286,"2_appLater",1346472246,"2_appLater",1360645638,"2_appLater",1374819030,"2_appLater",1388992423,"2_appLater",1403165815,"2_appLater",4294967295,"2_appLater"]}]}},"abraURL":"https://a1.nyt.com/abra-config/current/vi-prd.json"}]); })();;(function () { var _f=function(e,r){var t=window.vi&&window.vi.webviewEnvironment&&window.vi.webviewEnvironment.isPreloaded;function n(){var n,s,o=e.url;try{n=JSON.parse(e.body)}catch(e){return void console.error("Error parsing body:",e)}try{var i=window.location.search.slice(1).split("&").reduce(function(e,r){return"ip-override"===r.split("=")[0]?"?"+r:e},"");o+=i}catch(e){console.warn(e)}function a(r,n,s,o){var i=new XMLHttpRequest;for(var a in i.withCredentials=!0,i.open(r,n,!0),i.setRequestHeader("Content-Type","application/json"),i.setRequestHeader("Accept","application/json"),t&&(i.setRequestHeader("NYT-User-Token",window.getNativeBridgeCookie("NYT-S")),i.setRequestHeader("NYT-Agent-ID",window.getNativeBridgeCookie("nyt-a"))),e.headers)i.setRequestHeader(a,e.headers[a]);i.onreadystatechange=function(){i.readyState===XMLHttpRequest.DONE&&o(i)},i.send(s?JSON.stringify(s):null),window.userXhrObject=i}function d(e){var t;if(200===e.status)try{var s=JSON.parse(e.responseText);!s.data&&s.errors&&s.errors.length>0&&"PersistedQueryNotFound"===s.errors[0].message&&(console.warn("Persisted query not found for GET request."),t={operationName:n.operationName,query:n.query,extensions:{persistedQuery:{version:1,sha256Hash:r}},variables:n.variables},a("POST",o,t,function(e){200!==e.status&&console.warn("POST request failed:",e.status,e.statusText)}))}catch(e){console.warn("Error processing response:",e)}else console.warn("Request failed:",e.status,e.statusText)}s="extensions="+encodeURIComponent(JSON.stringify({persistedQuery:{version:1,sha256Hash:r}}))+"&operationName="+n.operationName+"&variables="+encodeURIComponent(JSON.stringify(n.variables)),a("GET",o+"?"+s,null,d)}t&&!window.nativeBridgeCookies?window.initNativeBridgeCookies(function(){n()}):n(),window.userXhrRefresh=function(){return n(),window.userXhrObject}};;_f.apply(null, [{"url":"https://samizdat-graphql.nytimes.com/graphql/v2","body":"{\"operationName\":\"UserQuery\",\"variables\":{},\"query\":\" query UserQuery { user { __typename profile { displayName email givenName } newsletterSubscriptions { newsletters { productCode isFreeTrial freeTrialSignupTime } } userInfo { regiId entitlements demographics { emailSubscriptions wat } } subscriptionDetails { bundleType cancellationDate graceStartDate graceEndDate isFreeTrial hasQueuedSub startDate endDate status hasActiveEntitlements entitlements billingSource promotionTierType subscriptionName subscriptionProducts subscriptionLabels } } } \"}","headers":{"nyt-app-type":"project-vi","nyt-app-version":"0.0.5","nyt-token":"MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAs+/oUCTBmD/cLdmcecrnBMHiU/pxQCn2DDyaPKUOXxi4p0uUSZQzsuq1pJ1m5z1i0YGPd1U1OeGHAChWtqoxC7bFMCXcwnE1oyui9G1uobgpm1GdhtwkR7ta7akVTcsF8zxiXx7DNXIPd2nIJFH83rmkZueKrC4JVaNzjvD+Z03piLn5bHWU6+w+rA+kyJtGgZNTXKyPh6EC6o5N+rknNMG5+CdTq35p8f99WjFawSvYgP9V64kgckbTbtdJ6YhVP58TnuYgr12urtwnIqWP9KSJ1e5vmgf3tunMqWNm6+AnsqNj8mCLdCuc5cEB74CwUeQcP2HQQmbCddBy2y0mEwIDAQAB","x-nyt-programming-abtest":".ver=23710.000","x-nyt-targeting-dimensions-map":"newsTenure=anon_user,agent_id=uUPwe3rT6adYXJo-C9KZFi,regi_id"}},"18b49c689a1a1dda8e2237a503fe06644b44b0f5ca7b1e821a310d9ea9b64bd9"]); })();;(function () { var registry=window._interactiveRegistry={};function getId(e){for(;(e=e.parentElement)&&!e.matches("[data-sourceid].interactive-body"););return e?e.getAttribute("data-sourceid"):null}window.registerInteractive=function(e){var t={_subs:{cleanup:[],remount:[]},id:e,on:function(e,r){return this._subs[e].push(r),t},errorFound:!1},r=document.getElementById("embed-id-"+e),n=new MutationObserver(function(){(r.textContent.includes("500 Internal Error")||r.textContent.includes("200 undefined"))&&(t.errorFound||(window.dispatchEvent(new CustomEvent("trigger-embed-hydration-"+e)),window.Sentry&&window.Sentry.captureException&&window.Sentry.captureException(new Error("Embed load error, triggered manual rehydration: "+e)),t.errorFound=!0))});r&&n.observe(r,{characterData:!0,attributes:!1,childList:!0,subtree:!0}),registry[e]=t},window.getInteractiveBridge=function(e){var t="string"==typeof e?e:getId(e);return registry[t]}; })();;(function () { var _f=function(){"function"!=typeof window.onInitNativeAds&&(window.onInitNativeAds=function(){})};;_f.apply(null, []); })();
</script>
<script>!function(){try{var e="undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:{},n=(new Error).stack;n&&(e._sentryDebugIds=e._sentryDebugIds||{},e._sentryDebugIds[n]="b94694c8-7092-471b-b60e-41fd729f1762",e._sentryDebugIdIdentifier="sentry-dbid-b94694c8-7092-471b-b60e-41fd729f1762")}catch(e){}}();var _global="undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:{};_global.SENTRY_RELEASE={id:"a33e3b3f2d9932550881a21bdebe3aaec6164b65"},function(e){function n(n){for(var r,u,l=n[0],i=n[1],a=n[2],s=0,b=[];s<l.length;s++)u=l[s],Object.prototype.hasOwnProperty.call(o,u)&&o[u]&&b.push(o[u][0]),o[u]=0;for(r in i)Object.prototype.hasOwnProperty.call(i,r)&&(e[r]=i[r]);for(d&&d(n);b.length;)b.shift()();return f.push.apply(f,a||[]),t()}function t(){for(var e,n=0;n<f.length;n++){for(var t=f[n],r=!0,l=1;l<t.length;l++){var i=t[l];0!==o[i]&&(r=!1)}r&&(f.splice(n--,1),e=u(u.s=t[0]))}return e}var r={},o={152:0},f=[];function u(n){if(r[n])return r[n].exports;var t=r[n]={i:n,l:!1,exports:{}};return e[n].call(t.exports,t,t.exports,u),t.l=!0,t.exports}u.m=e,u.c=r,u.d=function(e,n,t){u.o(e,n)||Object.defineProperty(e,n,{enumerable:!0,get:t})},u.r=function(e){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(e,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(e,"__esModule",{value:!0})},u.t=function(e,n){if(1&n&&(e=u(e)),8&n)return e;if(4&n&&"object"==typeof e&&e&&e.__esModule)return e;var t=Object.create(null);if(u.r(t),Object.defineProperty(t,"default",{enumerable:!0,value:e}),2&n&&"string"!=typeof e)for(var r in e)u.d(t,r,function(n){return e[n]}.bind(null,r));return t},u.n=function(e){var n=e&&e.__esModule?function(){return e.default}:function(){return e};return u.d(n,"a",n),n},u.o=function(e,n){return Object.prototype.hasOwnProperty.call(e,n)},u.p="/vi-assets/static-assets/";var l=window.webpackJsonp=window.webpackJsonp||[],i=l.push.bind(l);l.push=n,l=l.slice();for(var a=0;a<l.length;a++)n(l[a]);var d=i;t()}([]);
//# sourceMappingURL=runtime~adslot-3055f3d2d5dcb595fc6f.js.map</script>
<script async="" src="/vi-assets/static-assets/adslot-7fdd87617fc98f98a245.js"></script>
<script data-rh="true">
(function () { var _f=function(){const n="1_block";function o(n){return window&&window.NYTD&&window.NYTD.Abra?window.NYTD.Abra(n):""}window.adClientUtils={hasActiveToggle:function(r){return o(r)!==n},getAbraVar:o,reportExposure:function(n){window&&window.NYTD&&window.NYTD.Abra&&window.NYTD.Abra.reportExposure&&window.NYTD.Abra.reportExposure(n)},getAdsPurrDirective:function(){let n;if(window&&window.config&&window.config.PurrDirectives)n=window.config.PurrDirectives.PURR_AdConfiguration_v3;else{const o={f:"full",r:"rdp",n:"npa",l:"ltd",s:"adluce-socrates",a:"no-ads"},r=function(n){if("string"!=typeof n)return"";const o=document.cookie.match(new RegExp(n.concat("=([^;]+)")));return o?o[1]:""}("nyt-purr");n=o[r&&r.substring(14,15)]}return n}}};;_f.apply(null, []); })();
(function () { var _f=function(t){try{if(!(e=t,!!(window&&window.adClientUtils&&window.adClientUtils.hasActiveToggle)&&window.adClientUtils.hasActiveToggle(e)))return;!function(){const t=window&&window.adClientUtils&&window.adClientUtils.getAdsPurrDirective?window.adClientUtils.getAdsPurrDirective():"";if("adluce-socrates"!==t&&"no-ads"!==t){var e=document.createElement("script");e.async="async",e.src="ltd"===t?"//pagead2.googlesyndication.com/tag/js/gpt.js":"//securepubads.g.doubleclick.net/tag/js/gpt.js",document.head.appendChild(e)}}()}catch(t){console.log(t)}var e};;_f.apply(null, ["dfp_home_toggle"]); })();
(function () { var _f=function(){var t,e,o=50,n=50;function i(t){if(!document.getElementById("3pCheckIframeId")){if(t||(t=1),!document.body){if(t>o)return;return t+=1,setTimeout(i.bind(null,t),n)}var e,a,r;e="https://static01.nyt.com/ads/tpc-check.html",a=document.body,(r=document.createElement("iframe")).src=e,r.id="3pCheckIframeId",r.style="display:none;",r.height=0,r.width=0,a.insertBefore(r,a.firstChild)}}function a(t){if("https://static01.nyt.com"===t.origin)try{"3PCookieSupported"===t.data&&googletag.cmd.push(function(){googletag.pubads().setTargeting("cookie","true")}),"3PCookieNotSupported"===t.data&&googletag.cmd.push(function(){googletag.pubads().setTargeting("cookie","false")})}catch(t){}}function r(){if(function(){if(Object.prototype.toString.call(window.HTMLElement).indexOf("Constructor")>0)return!0;if("[object SafariRemoteNotification]"===(!window.safari||safari.pushNotification).toString())return!0;try{return window.localStorage&&/Safari/.test(window.navigator.userAgent)}catch(t){return!1}}()){try{window.openDatabase(null,null,null,null)}catch(e){return t(),!0}try{localStorage.length?e():(localStorage.x=1,localStorage.removeItem("x"),e())}catch(o){navigator.cookieEnabled?t():e()}return!0}}!function(){try{googletag.cmd.push(function(){googletag.pubads().setTargeting("cookie","unknown")})}catch(t){}}(),t=function(){try{googletag.cmd.push(function(){googletag.pubads().setTargeting("cookie","private")})}catch(t){}}||function(){},e=function(){window.addEventListener("message",a,!1),i(0)}||function(){},function(){if(window.webkitRequestFileSystem)return window.webkitRequestFileSystem(window.TEMPORARY,1,e,t),!0}()||r()||function(){if(!window.indexedDB&&(window.PointerEvent||window.MSPointerEvent))return t(),!0}()||e()};;_f.apply(null, ["dfp_home_toggle"]); })();
</script><script data-rh="true" id="als-svc">(function () { var _f=function(e,t,n,i){function l(e,t="impression"){window.UnifiedTracking&&window.UnifiedTracking.sendAnalytic&&window.UnifiedTracking.sendAnalytic(t,e)}var o;if(!(o=n,!!(window&&window.adClientUtils&&window.adClientUtils.hasActiveToggle)&&window.adClientUtils.hasActiveToggle(o)))return;!function(e){window&&window.NYTD&&window.NYTD.Abra&&window.NYTD.Abra.reportExposure(e)}(n);let a=()=>{var e=new Date,t=e.getFullYear();return e.getMonth()<9&&(t+="0"),t+=e.getMonth()+1,e.getDate()<10&&(t+="0"),t+=e.getDate(),e.getHours()<10&&(t+="0"),t+=e.getHours(),e.getMinutes()<10&&(t+="0"),t+=e.getMinutes(),e.getSeconds()<10&&(t+="0"),t+e.getSeconds()};window.googletag=window.googletag||{},googletag.cmd=googletag.cmd||[];let s=new URLSearchParams(location.search).get("alice_rules_test");var r,d=new XMLHttpRequest,g=window.vi.env.ALS_URL,c=document.querySelector('[name="nyt_uri"]');if(t)r="uri="+(u=t);else if("/"===location.pathname){var u=encodeURIComponent("https://www.nytimes.com/pages/index.html");r="uri="+u}else if(void 0===c||""===c||null===c){var m=e||location.protocol+"//"+location.hostname+location.pathname;r="url="+encodeURIComponent(m)}else{u=encodeURIComponent(c.content);r="uri="+u}var w=i;if(!w){var _=document.querySelector('[name="template"]');w=null==_||null==_.content?"":_.content}var p=document.querySelector('[name="prop"]'),b=document.querySelector('[name="plat"]'),f=null==p||null==p.content?"nyt":p.content,v=null==b||null==b.content?"web":b.content;window.innerWidth<740&&(f="mnyt",v="mweb");var U=window.localStorage.getItem("als_test_clientside"),h=null;window.googletag.cmd.push(function(){var e=U&&0!==U.length?U:"empty_empty_empty_"+a(),t=h||e;googletag.pubads().setTargeting("als_test_clientside",t)});var T=window.localStorage.getItem("mktg"),S=null;window.googletag.cmd.push(function(){var e=S||T;e&&googletag.pubads().setTargeting("mktg",e)});var C,y=window.localStorage.getItem("bt");window.googletag.cmd.push(function(){var e=null!=C?C:y;null!=e&&googletag.pubads().setTargeting("bt",e)});var x=window.localStorage.getItem("sub"),k=null;window.googletag.cmd.push(function(){var e=k||x;e&&googletag.pubads().setTargeting("sub",e)}),r=null==s?r:r+"&alice_rules_test="+s,d.open("GET",g+"/als?"+r+"&typ="+w+"&prop="+f+"&plat="+v,!0),d.withCredentials=!0,d.send(),d.onerror=function(){var e="reqfailed_reqfailed_reqfailed_"+a();h=e,window.googletag.cmd.push(function(){googletag.pubads().setTargeting("als_test_clientside",e)}),l({module:{name:"alice-timing",context:"script-load",label:"alice-error-reqfail-"+r}})},d.onreadystatechange=function(){if(4===d.readyState)if(200===d.status){var e=JSON.parse(d.responseText);h=e.als_test_clientside&&0!==e.als_test_clientside.length?e.als_test_clientside:"bou_bou_bou_"+a(),void 0!==e.User&&(void 0!==e.User.mktg&&(S=e.User.mktg,window.localStorage.setItem("mktg",e.User.mktg)),void 0!==e.User.bt&&(C=e.User.bt,window.localStorage.setItem("bt",e.User.bt)),void 0!==e.User.sub&&(k=e.User.sub,window.localStorage.setItem("sub",e.User.sub)),void 0!==e.User.aid&&(server_aid=e.User.aid,window.localStorage.setItem("aid",e.User.aid))),window.googletag.cmd.push(function(){if(e.als_test_clientside&&0!==e.als_test_clientside.length)googletag.pubads().setTargeting("als_test_clientside",e.als_test_clientside),window.localStorage.setItem("als_test_clientside","ls-"+e.als_test_clientside);else{var t=void 0===e.als_test_clientside?"undefined_undefined_undefined_"+a():"blank_blank_blank_"+a();googletag.pubads().setTargeting("als_test_clientside",t),l({module:{name:"alice-timing",context:"script-load",label:"alice-error-test-client-undefined"}})}if(void 0!==e.User){if(S)googletag.pubads().setTargeting("mktg",S);else l({module:{name:"alice-timing",context:"script-load",label:"alice-error-mktg-undefined"}});if(void 0!==C)googletag.pubads().setTargeting("bt",C);else l({module:{name:"alice-timing",context:"script-load",label:"alice-error-bt-undefined"}});if(k)googletag.pubads().setTargeting("sub",k);else l({module:{name:"alice-timing",context:"script-load",label:"alice-error-sub-undefined"}});(e.User.lucidC1||e.User.lucidC2||e.User.lucidC3||e.User.lucidC4||e.User.lucidC5)&&l({c1_val:e.User.lucidC1,c2_val:e.User.lucidC2,c3_val:e.User.lucidC3,c4_val:e.User.lucidC4,c5_val:e.User.lucidC5},"lucidtest")}else{l({module:{name:"alice-timing",context:"script-load",label:"alice-error-user-undefined"}})}if(void 0!==e.Asset)for(var n in e.Asset){var i=e.Asset[n];if(i)googletag.pubads().setTargeting(n,i);else l({module:{name:"alice-timing",context:"script-load",label:"alice-error-"+n+"-undefined"}})}else l({module:{name:"alice-timing",context:"script-load",label:"alice-error-asset-undefined"}})})}else{d.responseText.substring(0,40);var t="error_"+d.status+"_error_"+a();window.googletag.cmd.push(function(){googletag.pubads().setTargeting("als_test_clientside",t)}),l({module:{name:"alice-timing",context:"script-load",label:"alice-error-ajaxreq-"+d.status+"-"+r}})}}};;_f.apply(null, [null,null,"als_toggle","hp"]); })();</script><script data-rh="true" id="adslot-config">(function() {
var AdSlot4=function(){"use strict";var e=function(){return window.AdSlot4=window.AdSlot4||{},window.AdSlot4.cmd=window.AdSlot4.cmd||[],window.AdSlot4};var t=function(){return t=Object.assign||function(e){for(var t,n=1,i=arguments.length;n<i;n++)for(var o in t=arguments[n])Object.prototype.hasOwnProperty.call(t,o)&&(e[o]=t[o]);return e},t.apply(this,arguments)};"function"==typeof SuppressedError&&SuppressedError;var n=[{name:"PURR_AcceptableTrackers",valueMapping:{controllers:"c",processors:"p"}},{name:"PURR_AdConfiguration",valueMapping:{full:"f",npa:"n","adluce-socrates":"s"}},{name:"PURR_DataSaleOptOutUI",valueMapping:{hide:"h",show:"s"}},{name:"PURR_DataProcessingConsentUI",valueMapping:{hide:"h",show:"s"}},{name:"PURR_AcceptableTrackers_v2",valueMapping:{controllers:"c",processors:"p",essentials:"e"}},{name:"PURR_AdConfiguration_v2",valueMapping:{full:"f",rdp:"r",npa:"n",adluce:"a","adluce-socrates":"s"}},{name:"PURR_DataProcessingPreferenceUI",valueMapping:{hide:"h","allow-opt-out":"o","allow-opt-in":"i","allow-opt-in-or-out":"a"}},{name:"PURR_DataSaleOptOutUI_v2",valueMapping:{hide:"h",show:"s","show-opted-out":"o"}},{name:"PURR_CaliforniaNoticesUI",valueMapping:{hide:"h",show:"s"}},{name:"PURR_EmailMarketingOptInUI",valueMapping:{checked:"c",unchecked:"u"}},{name:"PURR_DeleteIPAddress",valueMapping:{delete:"d",keep:"k"}},{name:"PURR_AdConfiguration_v3",valueMapping:{full:"f",rdp:"r",npa:"n",ltd:"l","adluce-socrates":"s"}},{name:"PURR_LimitSensitivePI",valueMapping:{hide:"h",show:"s"}},{name:"PURR_EmailMarketingOptInUI_v2",valueMapping:{checked:"c",unchecked:"u","do-not-display":"d"}},{name:"PURR_AdConfiguration_v4",valueMapping:{full:"f",rdp:"r",npa:"n",ltd:"l","adluce-socrates":"s","no-ads":"a"}}],i=function(e){return-1!==document.cookie.indexOf(e)},o=function(e){var t,i,o={PURR_AcceptableTrackers:0,PURR_AdConfiguration:5,PURR_DataSaleOptOutUI:2,PURR_DataProcessingConsentUI:3,PURR_AcceptableTrackers_v2:4,PURR_AdConfiguration_v2:5,PURR_DataProcessingPreferenceUI:6,PURR_DataSaleOptOutUI_v2:7,PURR_CaliforniaNoticesUI:8,PURR_EmailMarketingOptInUI:9,PURR_DeleteIPAddress:10,PURR_AdConfiguration_v3:11,PURR_LimitSensitivePI:12,PURR_EmailMarketingOptInUI_v2:13,PURR_AdConfiguration_v4:14},r=(t=e,2===(i="; ".concat(document.cookie).split("; ".concat(t,"="))).length?i.pop().split(";").shift():null),a={};return Object.keys(o).forEach((function(e){var t,n,i;a[e]=(t=r,n=new RegExp("^.{".concat(o[e],"}(.)")),(null==(i=t.match(n))?void 0:i[1])||"")})),n.forEach((function(e){Object.keys(e.valueMapping).forEach((function(t){e.valueMapping[t]===a[e.name]&&(a[e.name]=t)}))})),a},r={PURR_DataSaleOptOutUI:"hide",PURR_DataSaleOptOutUI_v2:"hide",PURR_CaliforniaNoticesUI:"hide",PURR_DataProcessingConsentUI:"hide",PURR_DataProcessingPreferenceUI:"hide",PURR_AcceptableTrackers_v2:"controllers",PURR_AcceptableTrackers:"controllers",PURR_AdConfiguration_v2:"full",PURR_AdConfiguration:"full",PURR_EmailMarketingOptInUI:"unchecked",PURR_DeleteIPAddress:"delete",PURR_AdConfiguration_v3:"full",PURR_LimitSensitivePI:"hide",PURR_EmailMarketingOptInUI_v2:"unchecked",PURR_AdConfiguration_v4:"full"},a=function(){var e;try{return function(){if("undefined"!=typeof window){var e=window.navigator.userAgent||window.navigator.vendor,t=-1!==e.indexOf("nyt_android"),n=-1!==e.indexOf("nytios"),i=-1!==e.indexOf("nyt_xwords_ios"),o=-1!==e.indexOf("Crosswords");return t||n||i||o}return!1}()?(null===(e=null===window||void 0===window?void 0:window.config)||void 0===e?void 0:e.PurrDirectives)?window.config.PurrDirectives:i("override-purr")?o("override-purr"):t({},r):i("nyt-purr")?o("nyt-purr"):t({},r)}catch(e){return console.warn("can’t get directives from cookie or config",e),t({},r)}},d=function(){var e={};return"undefined"!=typeof window&&window.document&&window.document.createElement&&(e=a().PURR_AdConfiguration_v3||a().PURR_AdConfiguration_v2),e};var c=function(){return"full"===d()};function s(e,t,n){var i=document.getElementsByTagName("head")[0],o=document.createElement("script");t&&(o.onload=t),n&&(o.onerror=n),o.src=e,o.async=!0,i.appendChild(o)}var u={32074718:!0,4792640386:!0,21966278:!0,4558311760:!0,4552626466:!0,4400775978:!0,39318518:!0,4874174581:!0,33597638:!0,38636678:!0,38637278:!0,33597998:!0,33613118:!0,30252878:!0,33597758:!0,5154427359:!0},l="script_loader_error",p=function(){e().cmd.push((function(){var t="".concat("GeoEdge"," failed to load");e().events.publish({name:l,value:{message:t}})}))},m=function(){return!window.grumi&&(s("//rumcdn.geoedge.be/b3960cc6-bfd2-4adc-910c-6e917e8a6a0e/grumi-ip.js",null,p),window.grumi={key:"b3960cc6-bfd2-4adc-910c-6e917e8a6a0e",cfg:{advs:u}},!0)},f="A9",v=[[300,50],[320,50],[300,250],[728,90],[970,90],[970,250]],g=function(){if(!window.apstag){var e="".concat(f," not loading properly");console.warn(e)}},w="BidderError",b=function(){e().cmd.push((function(){var t="".concat(f," failed to load");e().events.publish({name:w,value:{type:f,message:t}})}))},_=function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:window;return e.googletag=e.googletag||{},e.googletag.cmd=e.googletag.cmd||[],e.googletag},h=function(e){return!(!window.apstag||!window.apstag.fetchBids)&&(window.apstag.fetchBids({slots:e},void(window.apstag&&window.apstag.setDisplayBids&&_().cmd.push(window.apstag.setDisplayBids()))),!0)},R="AdEmpty",P="AdBlockOn",y="AdDefined",U="AdRefreshed";var I=function(e,t){var n=[].concat(function(e,t){return[].concat(e).slice().sort((function(e,t){return t[0]-e[0]})).find((function(e){return!Number.isNaN(e[0])&&e[0]<t}))}(e,t)).pop();return n&&n.length?n:null},A=function(e,t,n){return function(){var i=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},o=i.sizes,r=void 0===o?[]:o,a=i.truePosition,d=i.id;if(t&&n.fastFetchSlots.map((function(e){return e.id})).includes(d))return!1;var c,s=I(r,window.innerWidth),u=(c=s,Array.isArray(c)?v.filter((function(e){return c.some((function(t){return t[0]===e[0]&&t[1]===e[1]}))})):(console.warn("filterSizes() did not receive an array"),[]));if(u.length>0){var l=(null==n?void 0:n.pageName)||e,p=[{slotID:a||d,slotName:"".concat(a||d,"_").concat(l,"_web"),sizes:u}];return h(p),!0}return!1}},O=function(t,n,i){e().cmd.push((function(){t&&h(function(e,t,n){var i=(null==n?void 0:n.pageName)||t;return n.fastFetchSlots.map((function(e){var t=e.id,n=e.sizes;return{slotID:t,slotName:"".concat(t,"_").concat(i,"_web"),sizes:n}}))}(window.innerWidth,n,i)),e().events.subscribe({name:y,scope:"all",callback:A(n,t,i)})}))},k=function(e,t,n,i){var o=function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:"apstag",t=arguments.length>1&&void 0!==arguments[1]?arguments[1]:window;if(!t[e]){var n=function(n,i){return t[e]._Q.push([n,i])};t[e]={_Q:[],init:function(){n("i",arguments)},fetchBids:function(){n("f",arguments)},setDisplayBids:function(){},targetingKeys:function(){return[]}}}return t[e]}("apstag",window);o.init({pubID:"3030",deals:!0,adServer:"googletag",params:{si_section:t}}),O(e,n,i)};function x(e,t,n){return(t=function(e){var t=function(e,t){if("object"!=typeof e||!e)return e;var n=e[Symbol.toPrimitive];if(void 0!==n){var i=n.call(e,t||"default");if("object"!=typeof i)return i;throw new TypeError("@@toPrimitive must return a primitive value.")}return("string"===t?String:Number)(e)}(e,"string");return"symbol"==typeof t?t:t+""}(t))in e?Object.defineProperty(e,t,{value:n,enumerable:!0,configurable:!0,writable:!0}):e[t]=n,e}function D(e,t){var n=Object.keys(e);if(Object.getOwnPropertySymbols){var i=Object.getOwnPropertySymbols(e);t&&(i=i.filter((function(t){return Object.getOwnPropertyDescriptor(e,t).enumerable}))),n.push.apply(n,i)}return n}function S(e){for(var t=1;t<arguments.length;t++){var n=null!=arguments[t]?arguments[t]:{};t%2?D(Object(n),!0).forEach((function(t){x(e,t,n[t])})):Object.getOwnPropertyDescriptors?Object.defineProperties(e,Object.getOwnPropertyDescriptors(n)):D(Object(n)).forEach((function(t){Object.defineProperty(e,t,Object.getOwnPropertyDescriptor(n,t))}))}return e}var j={art:{id:["mobile_top","top","story-ad-1","story-ad-2","story-ad-3","story-ad-4","story-ad-5","story-ad-6","bottom"],pos:["mobile_top","top","mid1","mid2","mid3","mid4","mid5","mid6","bottom"]},int:{id:["top","mid1","mid2","bottom"],pos:["top","mid1","mid2","bottom"]},hp:{id:["dfp-ad-top","dfp-ad-mid1","dfp-ad-mid2","dfp-ad-mid3","dfp-ad-bottom"],pos:["top","mid1","mid2","mid3","bottom"]},ss:{id:["right-0","right-1","right-2","right-3"],pos:["mid1","mid1","mid1","mid1"],size:{small:[[300,250]],medium:[[300,250]],large:[[300,250]]}},sf:{id:["top","mid1","mid2"],pos:["top","mid1","mid2"],size:{small:[[300,250]],medium:[[300,250]],large:[[300,250]]}},games:{id:["ad-top","ad-mid1","ad-bottom","intsl"],pos:["top","mid1","bottom","intsl"]},default:{id:["top","mid1","mid2"],pos:["top","mid1","mid2"],size:{small:[[300,250]],medium:[[728,90]],large:[[728,90],[970,90],[970,250]]}}},C={top:2088370,mid:2088372,bottom:2088374,intsl:3614988,default:2088376},E={top:544112060,mid:544112063,bottom:544112062,intsl:561454180,default:544112065},M={top:684296214,mid:190706820,bottom:932254072,intsl:868466422,default:153468583},T={top:"NYTimes_728x90_970_top_PB",mid:"NYTimes_728x90_970_mid_PB",bottom:"NYTimes_728x90_970_bot_PB",intsl:"NYT_mobile_Interstitial_Prebid",default:"NYTimes_728x90_970_mid_PB"},z={top:"nytimes_top",mid:"nytimes_mid",bottom:"nytimes_bottom",intsl:"6401830",default:"nytimes_catchall"},N={top:"995821",mid:"995822",bottom:"995823",intsl:"11499610",default:"995824"},B={TOP:"top",MID:"mid",BOTTOM:"bottom",INTSL:"intsl"},Y={buckets:[{max:3,increment:.05},{max:10,increment:.01},{max:20,increment:.1},{max:50,increment:.5},{max:101,increment:1}]},F=function(e,t){return e[Object.values(B).reduce((function(e,n){return t.includes(n)?n:e}),t)]||e.default},Q=function(e,t){var n=arguments.length>2&&void 0!==arguments[2]?arguments[2]:[],i=[{bidder:"appnexus",params:{member:3661,invCode:"nyt_".concat(e,"_").concat(t)}},{bidder:"criteo",params:{pubid:"B7HPLK",networkId:10470}},{bidder:"medianet",params:{cid:"8CU4WQK98",crid:F(M,t)}},{bidder:"rubicon",params:{accountId:12330,siteId:"crosswords"==e?571306:378266,inventory:{invCode:["nyt_".concat(e,"_").concat(t)]},zoneId:F(C,t),position:"top"===t?"atf":"btf"}},{bidder:"openx",params:{unit:F(E,t),delDomain:"nytimes-d.openx.net",customParams:{invCode:"nyt_".concat(e,"_").concat(t)}}},{bidder:"triplelift",params:{inventoryCode:F(T,t)}},{bidder:"pubmatic",params:{publisherId:"163427",adSlot:F(z,t)}},{bidder:"ix",params:{siteId:F(N,t)}}],o=i.filter((function(e){return!["pubmatic","ix"].includes(e.bidder)}));return n.length?i.filter((function(e){return n.includes(e.bidder)})):o},L=function(e){var t;switch(e){case"livebl":t="hp";break;case"coll":t="sf";break;default:t=e}return t in j||(t="default"),t};function V(e,t,n){var i=arguments.length>3&&void 0!==arguments[3]?arguments[3]:{},o=i.sizeConfig,r=i.activeBidders,a=i.positionsToFilter,d=void 0===a?[]:a,c=L(t),s=j[c].size?c:"default";return j[c].pos.reduce((function(t,i,a){var u;if(d.includes(i))return t;var l=j[c].id[a],p=S(S({},j[s].size),null==o||null===(u=o[l])||void 0===u?void 0:u.size),m=function(e,t){if(!t||""===t)return{};var n="".concat(t,"/").concat(e);return{ortb2Imp:S(S({},"intsl"===e&&{instl:1}),{},{ext:{gpid:n,data:{pbadslot:n}}})}}(l,n),f=S(S({code:l},m),{},{mediaTypes:{banner:{sizeConfig:[{minViewPort:[970,0],sizes:p.large},{minViewPort:[728,0],sizes:p.medium},{minViewPort:[0,0],sizes:p.small}]}},bids:Q(e,i,r)});return t.push(f),t}),[])}var H="PreBid",K={userSync:{userIds:[{name:"identityLink",params:{pid:"14158",notUse3P:!0},storage:{type:"html5",name:"idl_env",expires:15,refreshInSeconds:1800}},{name:"pairId",params:{liveramp:{storageKey:"_lr_pairId"}}},{name:"uid2",params:{serverPublicKey:"UID2-X-P-MFkwEwYHKoZIzj0CAQYIKoZIzj0DAQcDQgAEb2nFEsnJ0npRQbsCE7O7IINIMdgu4J29kiA1Hm7GYqfTQAsmqgAQD1YfVkuBR319JpxJYrJzO6Vp73++LTlIwA==",subscriptionId:"jCQUsadB4p"}}],syncDelay:3e3}};var q=function(t){window.pbjs=window.pbjs||{},window.pbjs.initAdserverSet||(window.pbjs.initAdserverSet=!0,e().cmd.push((function(){e().events.subscribe({name:y,scope:"all",callback:function(e){_().cmd.push((function(){var n=function(e){var t=L(e);return j[t].id}(t);n.includes(e.id)&&window.pbjs.setTargetingForGPTAsync([e.id])}))}})})))},G=function(t,n,i){var o=arguments.length>3&&void 0!==arguments[3]?arguments[3]:{},r=function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:window;return e.pbjs=e.pbjs||{},e.pbjs.que=e.pbjs.que||[],e.pbjs}(),a=o.priceGranularity;r.setConfig(S({priceGranularity:a||Y},K)),function(t,n,i,o,r){var a=function(e,n){window.pbjs.initAdserverSet=!1,t.requestBids({bidsBackHandler:function(){q(e)},timeout:n})};e().cmd.push((function(){t.que.push((function(){t.addAdUnits(V(n,i,o,r)),a(i,1e4),e().events.subscribe({name:U,scope:"all",callback:function(){a(i,800)}})}))}))}(r,t,n,i,o)},J=function(){e().cmd.push((function(){var t="".concat(H," failed to load");e().events.publish({name:w,value:{type:H,message:t}})}))},Z=function(e,t,n,i){if(!window.pbjs){return s("https://www.nytimes.com/ads/prebid9.26.0.js",function(e,t,n,i){return function(){window.pbjs||console.log("prebid did not load"),G(e,t,n,i)}}(e,t,n,i),J),!0}return!1},W=function(){try{var e=((i=document.createElement("div")).innerHTML=" ",i.className="ad adsbox pub_300x250 pub_300x250m pub_728x90 text-ad textAd text_ad ad-server",i.style="width: 1px !important; height: 1px !important; position: absolute !important; left: -10000px !important; top: -1000px !important;",document.body.prepend(i),document.getElementsByClassName("ad adsbox")[0]),t=!(!(n=e)||0!==n.offsetHeight&&0!==n.clientHeight)||function(e){if(void 0!==window.getComputedStyle){var t=window.getComputedStyle(e,null);if(t&&("none"===t.getPropertyValue("display")||"hidden"===t.getPropertyValue("visibility")))return!0}return!1}(e);return function(e){document.body.removeChild(e)}(e),t}catch(e){console.error("ad class check failed",e)}var n,i;return!1},X=function(){return!(window&&window.AdSlot&&window.AdSlot.AdSlotReady)||(!(window&&window.googletag&&window.googletag.apiReady)||W())},$=function(){var e=window&&window.nyt_et&&window.nyt_et.get_host&&window.nyt_et.get_host();return e?fetch("".concat(e,"/.status"),{credentials:"include",headers:{accept:"*/*","content-type":"text/plain;charset=UTF-8"},mode:"no-cors"}).then((function(){return{success:!0}})).catch((function(e){return console.error("et track blocked",e),{success:!1}})):Promise.resolve({success:!1})};var ee,te,ne=function(e,t,n){var i=function(e){if(!document||!document.cookie||!document.cookie.match)return"";var t=document.cookie.match(new RegExp("".concat(e,"=([^;]+)")));return t?t[1]:""}("nyt-a")||null,o=!!(window&&window.matchMedia&&window.matchMedia("(max-width: 739px)").matches);return"".concat("https://a-reporting.nytimes.com/report.jpg","?mobile=").concat(o,"&block=").concat(n,"&aid=").concat(i,"&pvid=").concat(e,"&et=").concat(t)},ie=function(e,t,n){return!!(window&&window.NYTD&&window.NYTD.Abra&&"1_network_detection"===window.NYTD.Abra("DFP_blockDetect_0221"))&&((new Image).src=ne(e,t,n),!0)},oe=function(t,n){n&&e().cmd.push((function(){var t=e();t.events&&t.events.publish({name:R,value:{type:P}})}));var i=!1;return $().then((function(){i=(arguments.length>0&&void 0!==arguments[0]?arguments[0]:{success:!1}).success})).catch((function(){})).finally((function(){ie(t,i,n)}))},re=function(e){window.addEventListener("load",function(e){return function(){oe(e,X())}}(e))},ae=function(){var e=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{};if(ee)return!1;var t,n,i=e.loadAmazon,o=void 0===i||i,r=e.loadPrebid,a=void 0===r||r,d=e.setFastFetch,u=void 0!==d&&d,l=e.loadGeoEdge,p=void 0===l||l,f=e.section,v=void 0===f?"none":f,w=e.adUnitPath,_=void 0===w?"":w,h=e.pageViewId,R=void 0===h?"":h,P=e.pageType,y=void 0===P?"":P,U=e.prebidOverrides,I=void 0===U?{}:U,A=e.amazonOverrides,O=void 0===A?{}:A;if(t=document.referrer||"",(n=/([a-zA-Z0-9_\-.]+)(@|%40)([a-zA-Z0-9_\-.]+).([a-zA-Z]{2,5})/).test(t)||n.test(window.location.href))return!1;if(c()){var x=new RegExp(/art/).test(y)?"art":y;p&&m(),o&&function(e,t,n,i){!window.apstag&&(s("//c.amazon-adsystem.com/aax2/apstag.js",g,b),k(e,t,n,i))}(u,v,x,O),a&&Z(v,x,_,I)}return re(R),ee=!0,!0};return(te=e()).loadScripts=te.loadScripts||ae,window.AdSlot4=te,te}();
(function () { var _f=function(e={}){const i=window&&window.AdSlot4,t=!!window.adClientUtils.getAbraVar("DFP_Prebid_Web_Toggle_0924"),n=!!window.adClientUtils.getAbraVar("DFP_Amzn_Web_Toggle_0924"),o=!!window.adClientUtils.getAbraVar("DFP_MediaNet_Web_Toggle_0125"),a=window.adClientUtils.getAbraVar("DFP_Prebid_0624"),d=window.vi&&window.vi.webviewEnvironment||{},r=d&&"IOS"===d.deviceType&&d.isInWebview;function w(){const e=window.matchMedia("(max-width: 739px)");return e&&e.matches}try{const{adToggleMap:d,pageType:s,prebidOverrides:c,adSizesMapping:l,section:p,adUnitPath:g,isSectionThirdPartyEligible:u,setFastFetch:b,headerBidding:m={}}=e,h=function(e,i){const t=window.matchMedia("(min-width: 741px)").matches?"large":window.matchMedia("(min-width: 601px)").matches?"medium":"small";return{pageName:e,fastFetchSlots:function(e,i){return"art"!==i&&"hp"!==i?["top"]:"art"===i?"small"===e?["mobile_top"]:["top"]:"small"===e?[]:["dfp-ad-top"]}(t,e).map(e=>({id:e,sizes:i[e][t]}))}}(s,l),{useAmazon:_,usePrebid:A,useMediaNet:P}=m,y=Object.keys(d).reduce((e,i)=>{const t=d[i]||"";return e[i]=function(e){return!!(window&&window.adClientUtils&&window.adClientUtils.hasActiveToggle)&&window.adClientUtils.hasActiveToggle(e)}(t),e},{}),{amazon:S,geoedge:T}=y,v=c&&c.positionsToFilter||[],x=w()?v.concat(["top"]):v,f=["appnexus","medianet","rubicon","openx","triplelift","ix","pubmatic"];if(a&&window.adClientUtils.reportExposure("DFP_Prebid_0624"),"1_Criteo"===a&&f.push("criteo"),"function"==typeof i.loadScripts&&i.loadScripts({loadAmazon:_&&S&&u&&!n,loadPrebid:A&&u&&!t&&!r,loadMediaNet:P&&u&&!o,setFastFetch:"art"===s?b:!w()&&b,section:p,adUnitPath:g,prebidOverrides:{...c,positionsToFilter:x,activeBidders:f},amazonOverrides:h,pageType:s,pageViewId:window&&window.NYTD&&window.NYTD.PageViewId&&window.NYTD.PageViewId.current?window.NYTD.PageViewId.current:"",loadGeoEdge:T}),t&&window.Sentry&&window.Sentry.captureException){const e=new Error("Ads are disabled via Abra toggle - Prebid");window.Sentry.captureException(e)}if(n&&window.Sentry&&window.Sentry.captureException){const e=new Error("Ads are disabled via Abra toggle - Amazon");window.Sentry.captureException(e)}if(o&&window.Sentry&&window.Sentry.captureException){const e=new Error("Ads are disabled via Abra toggle - MediaNet");window.Sentry.captureException(e)}}catch(e){console.error(e)}};;_f.apply(null, [{"adToggleMap":{"amazon":"amazon_home_toggle","medianet":"medianet_home_toggle","dfp":"dfp_home_toggle","geoedge":"geoedge_toggle"},"pageType":"hp","section":"home","adUnitPath":"/29390238/nyt/homepage","isSectionThirdPartyEligible":true,"setFastFetch":true,"prebidOverrides":{},"adSizesMapping":{"mobile_top":{"medium":[[300,50],[320,50]],"small":[[300,50],[320,50]]},"top":{"large":[[728,90],[970,90],[970,250]],"medium":[[728,90],[300,250]],"small":[[300,250]]},"dfp-ad-top":{"large":[[728,90],[970,90],[970,250]],"medium":[[728,90],[300,250]],"small":[[300,250]]}},"headerBidding":{"useAmazon":true,"usePrebid":true,"useMediaNet":false}}]); })();
(function () { var _f=function(t={},e={},n=""){window.AdSlot4=window.AdSlot4||{},window.AdSlot4.cmd=window.AdSlot4.cmd||[],window.AdSlot4.clientRequirements={mergeObjects:function(t,...e){return e.reduce(function(t,e){return Object.entries(e).reduce(function(t,[e,n]){return t[e]&&null==n?t:Object.assign({},t,{[e]:n})},t)},t)},isFunction:function(t){return"[object Function]"===Object.prototype.toString.call(t)},getWebviewEnv:function(){return window.vi&&window.vi.webviewEnvironment||{}},getAbraVariant:function(t){if(!(window.NYTD&&window.NYTD.Abra&&window.NYTD.Abra.getAbraSync&&this.isFunction(window.NYTD.Abra.getAbraSync)))return void console.warn("Abra does not exist or is not a function");const e=window.NYTD.Abra.getAbraSync(t);return e&&e.variant},shouldHaltDFP:function(t){return"1_block"===this.getAbraVariant(t)},isAdsDisabled:function(t={}){const{adTargeting:{section:e}={},adsDisabled:n,adUnitPath:i=""}=t,o=i&&i.toLowerCase&&i.toLowerCase().includes("tragedy"),r=!!this.getAbraVariant("DFP_GPT_Web_Toggle_0924");if(r&&window.Sentry&&window.Sentry.captureException){const t=new Error("Ads are disabled via Abra toggle");window.Sentry.captureException(t)}return n||"learning"===e||o||r},getSov:function(t={}){return t.sov=t.sov||(Math.floor(4*Math.random())+1).toString(),{sov:t.sov}},getPageViewId:function(t){return{page_view_id:t&&t.current}},getUserData:function(t="{}"){try{const e=JSON.parse(t).data;return e&&e.user}catch(t){console.warn("userinfo data unavailable")}},getEm:function(t){return t&&t.length?{em:t.toString().toLowerCase()}:{}},getWat:function(t){return t?{wat:t.toLowerCase()}:{}},getDemographics:function(t){return this.mergeObjects(this.getEm(t&&t.emailSubscriptions),this.getWat(t&&t.wat))},isValidDfpTest:function(t){return t.toLowerCase().indexOf("dfp")>-1},joinArgumentsForVariant:function(){if(arguments.length)return[].slice.call(arguments).join("_").toLowerCase()},reduceAbraConfigKeysToDfpVariants:function(t=[],e=""){const n=this.getAbraVariant(e),i=this.joinArgumentsForVariant(e,n);return n&&i?t.concat(i):t},reduceFastlyAbraToDfpVariants:function(t=[],[e,n]=[]){const i=this.isValidDfpTest(e)&&n&&this.joinArgumentsForVariant(e,n);return i?t.concat(i):t},getDFPTestNames:function(t={}){if(!t)return[];const e=this.isValidDfpTest;return Object.keys(t).filter(function(t){return e(t)})},getAbraDfpVariants:function(t={},e={}){let n=[],i=[];if(Object.keys(e).length&&(i=Object.entries(e).reduce(this.reduceFastlyAbraToDfpVariants,[])),t.config&&t.getAbraSync){n=this.getDFPTestNames(t.config).reduce(this.reduceAbraConfigKeysToDfpVariants,[])}return{abra_dfp:[...n,...i]}},isMobile:function(t){const e=t.matchMedia("(max-width: 739px)");return e&&e.matches},isManualRefresh:function(t={}){return!(!t.navigation||1!==t.navigation.type)},getAltLangFromPathname:function(t=""){return 0===t.indexOf("/es/")?"es":""},getAdTargetingProperty:function(t=!1,e=""){let n=t?"m":"";return{prop:(n+=e)+"nyt"}},getAdTargetingPlatform:function(t=!1){return{plat:(t?"m":"")+"web"}},getAdTargetingEdition:function(t=""){return t.length?{edn:t}:{}},getAdTargetingVersion:function(t=!1){return{ver:(t?"m":"")+"vi"}},getNYTA:function(){var t=window.document.cookie.split(";").find(t=>t.includes("nyt-a"))||null;return t&&t.split("=").pop()||null},getHash53:function(t,e){let n=3735928559^e,i=1103547991^e;for(let e,o=0;o<t.length;o++)e=t.charCodeAt(o),n=Math.imul(n^e,2654435761),i=Math.imul(i^e,1597334677);return n=Math.imul(n^n>>>16,2246822507),n^=Math.imul(i^i>>>13,3266489909),i=Math.imul(i^i>>>16,2246822507),4294967296*(2097151&(i^=Math.imul(n^n>>>13,3266489909)))+(n>>>0)},getHashNYTA:function(){var t=this.getNYTA();if(!t)return;let e=this.getHash53(t,64).toString();return e.length<22&&(e=parseInt(e).toString(36)+this.getHash53(t,32).toString(36)+this.getHash53(t,16).toString(36)),e},getPublisherProvidedID:function(){return window.config&&window.config.userInfo&&window.config.userInfo.publisherProvidedID?window.config.userInfo.publisherProvidedID:this.getHashNYTA()},getLazyLoadOffset:function(t){let e=400;if(this.getWebviewEnv().isPreloaded){const n=window.screen&&window.screen.height,i=window.innerHeight,o="hp"===t?8:2;(n||i)&&(e=(n||i)*o)}return e},getAdTargetingHome:function(t,e,n){let i={},o={};return"hp"===t&&(i=e?{topRef:e}:{},o=n?{refresh:"manual"}:{}),this.mergeObjects(i,o)},getHybridConfigAdTargeting:function(t){let e={};return t&&t.config&&t.config.AdRequirements&&this.getWebviewEnv().isPreloaded&&(e=t.config.AdRequirements,"hp"===t.config.AdRequirements.typ&&(delete e.artlen,delete e.ledemedsz)),e},updateAdTargeting:function(t){if(!t)return;let e=t;if("string"==typeof e)try{e=JSON.parse(e)}catch(t){return void console.error("[updateAdTargeting - error parsing ad targeting]",t)}window.AdSlot4&&window.AdSlot4.updateAdReq&&window.AdSlot4.updateAdReq(e)},getAdTargeting:function(t={},n={}){const i=window&&window.NYTD&&window.NYTD.Abra?window.NYTD.Abra:{},o=this.isMobile(window),r=this.getAltLangFromPathname(window.location.pathname),a=this.isManualRefresh(performance);return this.mergeObjects(t,this.getDemographics(n),this.getAdTargetingProperty(o,r),this.getAdTargetingPlatform(o),this.getAdTargetingEdition(r),this.getAdTargetingVersion(o),this.getAdTargetingHome(t.typ,document.referrer,a),this.getAbraDfpVariants(i,e),this.getSov(window),this.getHybridConfigAdTargeting(window),this.getPageViewId(window.NYTD.PageViewId))},testReadyForAds:function(){const t="complete"===document.readyState,e=window.NYTD&&window.NYTD.PageViewId&&window.NYTD.PageViewId.current;return!(!t||!e)},whenReadyForAds:function(t){this.isFunction(t)?this.testReadyForAds()?t():window.addEventListener("readyForAds",t):console.warn("whenReadyForAds expects function")},signalIfReadyForAds:function(){this.testReadyForAds()&&window.dispatchEvent(new CustomEvent("readyForAds",{}))},init:function(t){window.AdSlot4.init&&this.isFunction(window.AdSlot4.init)?window.AdSlot4.init&&window.AdSlot4.init(t):console.warn("AdSlot4.init does not exist or is not a function")},generateConfig:function(t={},e={},n={}){const i=n&&n.userInfo&&n.userInfo.demographics;return this.mergeObjects(t,e,{offset:this.getLazyLoadOffset(e.adTargeting&&e.adTargeting.typ),adTargeting:this.getAdTargeting(e.adTargeting,i),haltDFP:this.shouldHaltDFP(e.dfpToggleName||t.dfpToggleName),adsDisabled:this.isAdsDisabled(e),enablePPID:AdSlot4.clientRequirements.getPublisherProvidedID()})}};for(let t in window.AdSlot4.clientRequirements)window.AdSlot4.clientRequirements[t]=window.AdSlot4.clientRequirements[t].bind(window.AdSlot4.clientRequirements);const i={adUnitPath:"/29390238/nyt/thisIsNotAPath",hideTopAd:AdSlot4.clientRequirements.isMobile(window),lockdownAds:!1,sizeMapping:{top:[[970,["fluid",[728,90],[970,90],[970,250],[1605,300]]],[728,["fluid",[728,90],[1605,300]]],[0,[]]],fp1:[[0,[[195,250],[215,270]]]],fp2:[[0,[[195,250],[215,270]]]],fp3:[[0,[[195,250],[215,270]]]],feat1:[[0,["fluid"]]],feat2:[[0,["fluid"]]],feat3:[[0,["fluid"]]],feat4:[[0,["fluid"]]],mktg:[[1020,[300,250]],[0,[]]],pencil:[[728,[[336,46]],[0,[]]]],pp_edpick:[[0,["fluid"]]],pp_morein:[[0,["fluid"],[210,218]]],ribbon:[[0,["fluid"]]],sponsor:[[765,[150,50]],[0,[320,25]]],supplemental:[[1020,[[300,250],[300,600]]],[0,[]]],chat:[[0,[[300,250],[300,420]]]],column:[[0,[[300,250],[300,420]]]],ressint:[[600,["fluid"]],[0,[[300,250]]]],mobile_top:[[0,[[300,50],[320,50]]]],default:[[970,["fluid",[728,90],[970,90],[970,250],[1605,300]]],[728,["fluid",[728,90],[300,250],[1605,300]]],[0,["fluid",[300,250],[300,420]]]]},adTargeting:{},haltDFP:!1,dfpToggleName:t.dfpToggleName,lazyApi:t.lazyApi||{},adsDisabled:!1};window.AdSlot4.cmd.push(function(){const e=window.AdSlot4.clientRequirements,o=e.getUserData(window&&window.userXhrObject&&window.userXhrObject.responseText);if(e.getWebviewEnv().isPreloaded){if(window.updateAdTargeting=e.updateAdTargeting,window.addEventListener("load",e.signalIfReadyForAds),n){window.googletag=window.googletag||{},window.googletag.cmd=window.googletag.cmd||[];const t="https://www.nytimes.com"+n;window.googletag.cmd.push(function(){window.googletag.pubads().set("page_url",t)})}e.whenReadyForAds(function(){const n=e.generateConfig(i,t,o);e.init(n)})}else{const n=e.generateConfig(i,t,o);e.init(n)}})};;_f.apply(null, [{"adTargeting":{"edn":"us","test":"projectvi","ver":"vi","typ":"hp"},"adUnitPath":"/29390238/nyt/homepage","dfpToggleName":"dfp_home_toggle","adsDisabled":false},{".ver":"23577.000","AMS_FrictionCircumventionDesktop_cwv":"2_low-mid-truncation","AMS_FrictionCircumventionMobile_cwv":"2_low-mid-truncation","DFP_MediaNet_0123":"","HOME_cwv_chartbeat":"0_Control",".programming_ver":"23710.000"},"/"]); })();
})();
</script><script data-rh="true" id="live-ramp">(function () { var _f=function(){var e=function(e){var t=document.cookie.match(new RegExp(e+"=([^;]+)"));if(t)return t[1]}("nyt-purr"),t=e&&e.substring(14,15)||"",a=window.navigator.userAgent.match(/(nyt)[_wd-]*(ios)/i),n="atsd",o="_lr_atsDirect";if(!(e&&"r"===t||e&&"l"===t||a)){!function(){if(document||document.head){var e=document.createElement("link");e.href="https://launchpad.privacymanager.io/latest/launchpad.bundle.js",e.as="script",document.head.appendChild(e);var t=document.createElement("script");t.async=!1,t.defer=!0,t.src="https://launchpad-wrapper.privacymanager.io/9fab0bf6-df63-42ca-acc5-caf4de668f40/launchpad-liveramp.js",document.head.appendChild(t)}}();var c=function(){var e,t=[];if(-1!==document.cookie.indexOf(o))try{e=(e=(e=document.cookie.split(";")).filter(e=>e.includes(o)))[0].split("=")[1],e=atob(decodeURIComponent(e)),t=JSON.parse(e).envelope}catch(e){console.warn("Error parsing targeting from cookie.",e)}return t}();c.length>0&&function(e){window.googletag=window.googletag||{cmd:[]},googletag.cmd.push(()=>{googletag.pubads().setTargeting(n,e)})}(c)}};;_f.apply(null, []); })();</script>
<!-- hoisted interactive css-->
<style>.css-14sajpe{color:var(--color-content-primary,#121212) !important;font-weight:700;-webkit-letter-spacing:0.5px;-moz-letter-spacing:0.5px;-ms-letter-spacing:0.5px;letter-spacing:0.5px;font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-14sajpe{font-size:0.875rem;line-height:1.0625rem;}}.css-wc84xp{font-weight:500;color:var(--color-content-primary,#121212);font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-wc84xp{font-size:0.8125rem;line-height:1rem;}}.css-wc84xp:last-child{margin-right:20px;}@media (min-width:740px){.css-wc84xp:last-child{margin-right:unset;}}.css-wc84xp strong{font-weight:700;}.css-wc84xp a{-webkit-text-decoration:none;text-decoration:none;-webkit-transition:color 0.6s ease;transition:color 0.6s ease;color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:visited{color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:hover,.css-wc84xp a:active{color:var(--color-content-quaternary,#727272) !important;}.css-qvlk0g{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:start;-webkit-justify-content:flex-start;-ms-flex-pack:start;justify-content:flex-start;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;gap:16px;background-color:var(--color-background-primary,#FFFFFF);font-family:nyt-franklin,helvetica,arial,sans-serif;padding:2px 0 10px;overflow-x:auto;white-space:nowrap;-webkit-scrollbar-width:none;-moz-scrollbar-width:none;-ms-scrollbar-width:none;scrollbar-width:none;width:calc(100vw - 20px);margin-left:20px;}@media (min-width:740px){.css-qvlk0g{width:auto;margin-left:unset;}}.has-banner-override .css-qvlk0g{-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;margin-left:0;width:100%;}.lede-package-title-override .css-qvlk0g{display:none;}.css-qvlk0g::-webkit-scrollbar{display:none;}@media (pointer:coarse){.css-qvlk0g{overflow-x:scroll;-webkit-overflow-scrolling:touch;}}@media (max-width:599px){.css-qvlk0g::after{content:'';background:linear-gradient(90deg,rgba(255,255,255,0),var(--color-background-primary,#FFFFFF));height:40px;position:absolute;right:0;width:24px;z-index:2;}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g::after{background:linear-gradient(90deg,rgba(42,42,42,0),var(--color-background-primary,#FFFFFF));}}}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g svg#olympics2024brand path.paris{fill:#ffffff;}}.css-11xr2bo{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;gap:16px;}</style><style>.css-14sajpe{color:var(--color-content-primary,#121212) !important;font-weight:700;-webkit-letter-spacing:0.5px;-moz-letter-spacing:0.5px;-ms-letter-spacing:0.5px;letter-spacing:0.5px;font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-14sajpe{font-size:0.875rem;line-height:1.0625rem;}}.css-wc84xp{font-weight:500;color:var(--color-content-primary,#121212);font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-wc84xp{font-size:0.8125rem;line-height:1rem;}}.css-wc84xp:last-child{margin-right:20px;}@media (min-width:740px){.css-wc84xp:last-child{margin-right:unset;}}.css-wc84xp strong{font-weight:700;}.css-wc84xp a{-webkit-text-decoration:none;text-decoration:none;-webkit-transition:color 0.6s ease;transition:color 0.6s ease;color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:visited{color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:hover,.css-wc84xp a:active{color:var(--color-content-quaternary,#727272) !important;}.css-qvlk0g{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:start;-webkit-justify-content:flex-start;-ms-flex-pack:start;justify-content:flex-start;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;gap:16px;background-color:var(--color-background-primary,#FFFFFF);font-family:nyt-franklin,helvetica,arial,sans-serif;padding:2px 0 10px;overflow-x:auto;white-space:nowrap;-webkit-scrollbar-width:none;-moz-scrollbar-width:none;-ms-scrollbar-width:none;scrollbar-width:none;width:calc(100vw - 20px);margin-left:20px;}@media (min-width:740px){.css-qvlk0g{width:auto;margin-left:unset;}}.has-banner-override .css-qvlk0g{-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;margin-left:0;width:100%;}.lede-package-title-override .css-qvlk0g{display:none;}.css-qvlk0g::-webkit-scrollbar{display:none;}@media (pointer:coarse){.css-qvlk0g{overflow-x:scroll;-webkit-overflow-scrolling:touch;}}@media (max-width:599px){.css-qvlk0g::after{content:'';background:linear-gradient(90deg,rgba(255,255,255,0),var(--color-background-primary,#FFFFFF));height:40px;position:absolute;right:0;width:24px;z-index:2;}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g::after{background:linear-gradient(90deg,rgba(42,42,42,0),var(--color-background-primary,#FFFFFF));}}}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g svg#olympics2024brand path.paris{fill:#ffffff;}}.css-11xr2bo{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;gap:16px;}</style><style>
.g-demo-sentence {
text-align: center;
font-family: nyt-cheltenham;
font-size: 16px;
line-height: 20px;
color: var(--color-content-tertiary, #5A5A5A);
margin-top: 23px
}
.g-demo-sentence a {
color: var(--color-content-primary, black);
}
.g-demo-sentence b {
font-weight: bold;
}
@media(max-width: 768px) {
.g-demo-sentence {
text-align: left;
margin-left: 20px;
margin-right: 20px;
margin-top: 15px;
margin-bottom: -8px;
font-size: 20px !important;
line-height: 24px !important;
}
}
</style><style>.css-14sajpe{color:var(--color-content-primary,#121212) !important;font-weight:700;-webkit-letter-spacing:0.5px;-moz-letter-spacing:0.5px;-ms-letter-spacing:0.5px;letter-spacing:0.5px;font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-14sajpe{font-size:0.875rem;line-height:1.0625rem;}}.css-wc84xp{font-weight:500;color:var(--color-content-primary,#121212);font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-wc84xp{font-size:0.8125rem;line-height:1rem;}}.css-wc84xp:last-child{margin-right:20px;}@media (min-width:740px){.css-wc84xp:last-child{margin-right:unset;}}.css-wc84xp strong{font-weight:700;}.css-wc84xp a{-webkit-text-decoration:none;text-decoration:none;-webkit-transition:color 0.6s ease;transition:color 0.6s ease;color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:visited{color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:hover,.css-wc84xp a:active{color:var(--color-content-quaternary,#727272) !important;}.css-qvlk0g{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:start;-webkit-justify-content:flex-start;-ms-flex-pack:start;justify-content:flex-start;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;gap:16px;background-color:var(--color-background-primary,#FFFFFF);font-family:nyt-franklin,helvetica,arial,sans-serif;padding:2px 0 10px;overflow-x:auto;white-space:nowrap;-webkit-scrollbar-width:none;-moz-scrollbar-width:none;-ms-scrollbar-width:none;scrollbar-width:none;width:calc(100vw - 20px);margin-left:20px;}@media (min-width:740px){.css-qvlk0g{width:auto;margin-left:unset;}}.has-banner-override .css-qvlk0g{-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;margin-left:0;width:100%;}.lede-package-title-override .css-qvlk0g{display:none;}.css-qvlk0g::-webkit-scrollbar{display:none;}@media (pointer:coarse){.css-qvlk0g{overflow-x:scroll;-webkit-overflow-scrolling:touch;}}@media (max-width:599px){.css-qvlk0g::after{content:'';background:linear-gradient(90deg,rgba(255,255,255,0),var(--color-background-primary,#FFFFFF));height:40px;position:absolute;right:0;width:24px;z-index:2;}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g::after{background:linear-gradient(90deg,rgba(42,42,42,0),var(--color-background-primary,#FFFFFF));}}}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g svg#olympics2024brand path.paris{fill:#ffffff;}}.css-11xr2bo{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;gap:16px;}</style><style>.css-14sajpe{color:var(--color-content-primary,#121212) !important;font-weight:700;-webkit-letter-spacing:0.5px;-moz-letter-spacing:0.5px;-ms-letter-spacing:0.5px;letter-spacing:0.5px;font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-14sajpe{font-size:0.875rem;line-height:1.0625rem;}}.css-wc84xp{font-weight:500;color:var(--color-content-primary,#121212);font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-wc84xp{font-size:0.8125rem;line-height:1rem;}}.css-wc84xp:last-child{margin-right:20px;}@media (min-width:740px){.css-wc84xp:last-child{margin-right:unset;}}.css-wc84xp strong{font-weight:700;}.css-wc84xp a{-webkit-text-decoration:none;text-decoration:none;-webkit-transition:color 0.6s ease;transition:color 0.6s ease;color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:visited{color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:hover,.css-wc84xp a:active{color:var(--color-content-quaternary,#727272) !important;}.css-qvlk0g{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:start;-webkit-justify-content:flex-start;-ms-flex-pack:start;justify-content:flex-start;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;gap:16px;background-color:var(--color-background-primary,#FFFFFF);font-family:nyt-franklin,helvetica,arial,sans-serif;padding:2px 0 10px;overflow-x:auto;white-space:nowrap;-webkit-scrollbar-width:none;-moz-scrollbar-width:none;-ms-scrollbar-width:none;scrollbar-width:none;width:calc(100vw - 20px);margin-left:20px;}@media (min-width:740px){.css-qvlk0g{width:auto;margin-left:unset;}}.has-banner-override .css-qvlk0g{-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;margin-left:0;width:100%;}.lede-package-title-override .css-qvlk0g{display:none;}.css-qvlk0g::-webkit-scrollbar{display:none;}@media (pointer:coarse){.css-qvlk0g{overflow-x:scroll;-webkit-overflow-scrolling:touch;}}@media (max-width:599px){.css-qvlk0g::after{content:'';background:linear-gradient(90deg,rgba(255,255,255,0),var(--color-background-primary,#FFFFFF));height:40px;position:absolute;right:0;width:24px;z-index:2;}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g::after{background:linear-gradient(90deg,rgba(42,42,42,0),var(--color-background-primary,#FFFFFF));}}}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g svg#olympics2024brand path.paris{fill:#ffffff;}}.css-11xr2bo{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;gap:16px;}</style><style>.css-14sajpe{color:var(--color-content-primary,#121212) !important;font-weight:700;-webkit-letter-spacing:0.5px;-moz-letter-spacing:0.5px;-ms-letter-spacing:0.5px;letter-spacing:0.5px;font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-14sajpe{font-size:0.875rem;line-height:1.0625rem;}}.css-wc84xp{font-weight:500;color:var(--color-content-primary,#121212);font-size:1rem;line-height:1.1875rem;}@media (min-width:740px){.css-wc84xp{font-size:0.8125rem;line-height:1rem;}}.css-wc84xp:last-child{margin-right:20px;}@media (min-width:740px){.css-wc84xp:last-child{margin-right:unset;}}.css-wc84xp strong{font-weight:700;}.css-wc84xp a{-webkit-text-decoration:none;text-decoration:none;-webkit-transition:color 0.6s ease;transition:color 0.6s ease;color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:visited{color:var(--color-content-primary,#121212) !important;}.css-wc84xp a:hover,.css-wc84xp a:active{color:var(--color-content-quaternary,#727272) !important;}.css-qvlk0g{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;-webkit-box-pack:start;-webkit-justify-content:flex-start;-ms-flex-pack:start;justify-content:flex-start;-webkit-align-items:center;-webkit-box-align:center;-ms-flex-align:center;align-items:center;gap:16px;background-color:var(--color-background-primary,#FFFFFF);font-family:nyt-franklin,helvetica,arial,sans-serif;padding:2px 0 10px;overflow-x:auto;white-space:nowrap;-webkit-scrollbar-width:none;-moz-scrollbar-width:none;-ms-scrollbar-width:none;scrollbar-width:none;width:calc(100vw - 20px);margin-left:20px;}@media (min-width:740px){.css-qvlk0g{width:auto;margin-left:unset;}}.has-banner-override .css-qvlk0g{-webkit-box-pack:center;-webkit-justify-content:center;-ms-flex-pack:center;justify-content:center;margin-left:0;width:100%;}.lede-package-title-override .css-qvlk0g{display:none;}.css-qvlk0g::-webkit-scrollbar{display:none;}@media (pointer:coarse){.css-qvlk0g{overflow-x:scroll;-webkit-overflow-scrolling:touch;}}@media (max-width:599px){.css-qvlk0g::after{content:'';background:linear-gradient(90deg,rgba(255,255,255,0),var(--color-background-primary,#FFFFFF));height:40px;position:absolute;right:0;width:24px;z-index:2;}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g::after{background:linear-gradient(90deg,rgba(42,42,42,0),var(--color-background-primary,#FFFFFF));}}}@media (prefers-color-scheme:dark){.NYTApp .css-qvlk0g svg#olympics2024brand path.paris{fill:#ffffff;}}.css-11xr2bo{display:-webkit-box;display:-webkit-flex;display:-ms-flexbox;display:flex;gap:16px;}</style><link href="https://static01.nytimes.com/newsgraphics/weather-hp-strip/cb02c00a-e5f5-41fb-b9ab-85ea8382b647/_assets/_app/immutable/assets/weather-hp-modules.cbd92ddb.css" rel="stylesheet"/><style>
.g-large-opinion-label {
font-family: nyt-franklin;
font-size: 14px;
letter-spacing: 0.5px;
font-weight: 700;
line-height: 1;
color: var(--color-content-primary, #121212);
}
.g-large-opinion-label a {
color: var(--color-content-primary, #121212);
text-decoration: none;
}
.g-large-opinion-label a:hover {
color: var(--color-content-quaternary, #727272);
}
@media (max-width: 739px) {
.g-large-opinion-label {
margin-left: 20px;
font-family: nyt-cheltenham;
letter-spacing: unset;
font-size: 30px;
font-weight: 400;
margin-bottom: 8px;
}
}
</style>
<!-- end hoisted interactive css-->
</head>
<body>
<div id="app"><a class="css-wsvg60" href="#site-content">Skip to content</a><a class="css-wsvg60" href="#site-index">Skip to site index</a><div class="css-1ichrj1 e1xxpj0j0"><div class="css-8ecw4e e1xxpj0j1"><div class="css-aljv3" data-testid="StandardAd"><a class="css-777zgl" href="#after-dfp-ad-top">SKIP ADVERTISEMENT</a><div class="ad dfp-ad-top-wrapper css-rfqw0c"><div class="place-ad" data-lazy-load="true" data-position="top" data-size-key="top" id="dfp-ad-top"></div></div><div id="after-dfp-ad-top"></div></div></div></div><div><div><div class="NYTAppHideMasthead css-1r6wvpq e1m0pzr40" data-agent-experiment="loading" data-dynamic-config="loading" data-feature-gate="loading" data-layer="loading" data-regi-experiment="loading" data-testid="masthead-container"><header class="css-ahe4g0 e1m0pzr41"><section class="css-1508xc5 e1m0pzr42"><div class="css-1hti9pf ea180rp0"></div><div class="css-8xdxq2 ell52qj0"><a aria-label="New York Times homepage" class="css-nhjhh0 ell52qj1" data-testid="masthead-mobile-logo" href="/"><svg aria-hidden="true" fill="#000000" viewbox="0 0 184 25"><path d="M14.57,2.57C14.57,.55,12.65-.06,11.04,.01V.19c.96,.07,1.7,.46,1.7,1.11,0,.45-.32,1.01-1.28,1.01-.76,0-2.02-.45-3.2-.84-1.3-.45-2.54-.87-3.57-.87-2.02,0-3.55,1.5-3.55,3.36,0,1.5,1.16,2.02,1.63,2.21l.03-.07c-.3-.2-.49-.42-.49-1.06,0-.54,.39-1.26,1.43-1.26,.94,0,2.17,.42,3.8,.88,1.4,.39,2.91,.76,3.75,.87v3.28l-1.58,1.3,1.58,1.36v4.49c-.81,.46-1.75,.61-2.56,.61-1.5,0-2.88-.42-4.02-1.68l4.26-2.07V5.73l-5.2,2.32c.54-1.38,1.55-2.41,2.66-3.08l-.03-.08C3.31,5.73,.5,8.56,.5,12.06c0,4.19,3.35,7.3,7.22,7.3,4.19,0,6.65-3.28,6.61-6.75h-.08c-.61,1.33-1.63,2.59-2.78,3.25v-4.38l1.65-1.33-1.65-1.33v-3.28c1.53,0,3.11-1.01,3.11-2.96M5.8,14.13l-1.21,.61c-.74-.96-1.23-2.32-1.23-4.07,0-.72,.08-1.7,.32-2.39l2.14-.96-.03,6.8h0Zm19.47-5.76l-.81,.64-2.47-2.2-2.86,2.21V.48l-3.89,2.69c.45,.15,.99,.39,.99,1.43v11.81l-1.33,1.01,.12,.12,.67-.46,2.32,2.12,3.11-2.07-.1-.15-.79,.52-1.08-1.08v-7.12l.74-.54,1.7,1.48v6.19c0,3.92-.87,4.73-2.63,5.37v.1c2.93,.12,5.57-.87,5.57-5.89v-6.75l.88-.72-.12-.15h0Zm5.22,10.8l4.51-3.62-.12-.17-2.36,1.87-2.71-2.14v-1.33l4.68-3.3-2.36-3.67-5.2,2.86v6.8l-1.01,.79,.12,.15,.96-.76,3.5,2.54h-.01Zm-.69-5.67v-5.15l2.27,3.55-2.27,1.6ZM53.65,1.61c0-.32-.08-.59-.2-.96h-.07c-.32,.87-.67,1.33-1.68,1.33-.88,0-1.58-.54-1.95-.94,0,.03-2.96,3.42-2.96,3.42l.15,.12,.84-.96c.64,.49,1.21,1.06,2.63,1.08V13.34l-6.06-10.5c-.47-.79-1.28-1.97-2.66-1.97-1.63,0-2.86,1.4-2.66,3.77h.1c.12-.59,.47-1.33,1.18-1.33,.57,0,1.03,.54,1.3,1.03v3.38c-1.87,0-2.93,.87-2.93,2.34,0,.61,.45,1.94,1.72,2.17v-.07c-.17-.17-.34-.32-.34-.67,0-.57,.42-.88,1.18-.88,.12,0,.3,.03,.37,.05v4.38c-2.2,.03-3.89,1.23-3.89,3.31s1.7,2.88,3.47,2.78v-.07c-1.11-.12-1.68-.69-1.68-1.5,0-.88,.64-1.36,1.45-1.36s1.43,.52,1.95,1.11l2.96-3.33-.12-.12-.76,.87c-1.14-1.01-1.87-1.48-3.18-1.68V4.67l8.36,14.57h.45V4.72c1.6-.1,3.03-1.3,3.03-3.11m2.81,17.54l4.51-3.62-.12-.17-2.36,1.87-2.71-2.14v-1.33l4.68-3.3-2.36-3.67-5.2,2.86v6.8l-1.01,.79,.12,.15,.96-.76,3.5,2.54h0Zm-.69-5.67v-5.15l2.27,3.55-2.27,1.6Zm21.22-5.52l-.69,.52-1.97-1.68-2.29,2.07,.94,.88v7.72l-2.34-1.6v-6.26l.81-.57-2.41-2.24-2.24,2.07,.94,.88v7.46l-.15,.1-2.2-1.6v-6.13c0-1.43-.72-1.85-1.63-2.41-.76-.47-1.16-.91-1.16-1.63,0-.79,.69-1.11,.91-1.23-.79-.03-2.98,.76-3.03,2.76-.03,1.03,.47,1.48,.99,1.97,.52,.49,1.01,.96,1.01,1.83v6.01l-1.06,.84,.12,.12,1.01-.79,2.63,2.14,2.51-1.75,2.76,1.75,5.42-3.2v-6.95l1.21-.94-.1-.15h0Zm18.15-5.84l-1.03,.94-2.32-2.02-3.13,2.51V1.19h-.19V18.12c-.34-.05-1.06-.25-1.85-.37V3.58c0-1.03-.74-2.47-2.59-2.47s-3.01,1.56-3.01,2.91h.08c.1-.61,.52-1.16,1.13-1.16s1.18,.39,1.18,1.78v4.04c-1.75,.07-2.81,1.16-2.81,2.34,0,.67,.42,1.92,1.75,1.97v-.1c-.45-.19-.54-.42-.54-.67,0-.59,.57-.79,1.36-.79h.19v6.51c-1.5,.52-2.2,1.53-2.2,2.78,0,1.72,1.38,3.05,3.4,3.05,1.43,0,2.44-.25,3.75-.54,1.06-.22,2.21-.47,2.83-.47,.79,0,1.14,.35,1.14,.91,0,.72-.27,1.08-.69,1.21v.1c1.7-.32,2.69-1.3,2.69-2.83s-1.5-2.54-3.18-2.54c-.87,0-2.44,.27-3.72,.57-1.43,.32-2.66,.47-3.11,.47-.72,0-1.6-.32-1.6-1.28,0-.87,.72-1.56,2.49-1.56,.96,0,1.9,.15,3.08,.42,1.26,.27,2.12,.64,3.2,.64,1.5,0,2.71-.54,2.71-2.74V3.29l1.11-1.01-.12-.15h0Zm-4.24,6.78c-.27,.3-.59,.54-1.11,.54-.57,0-.87-.3-1.14-.54V3.81l.74-.59,1.5,1.28v4.41h0Zm0,2.41c-.25-.25-.57-.47-1.11-.47s-.91,.27-1.14,.47v-2.17c.22,.19,.59,.49,1.14,.49s.87-.25,1.11-.49v2.17Zm0,5.1c0,.84-.42,1.78-1.5,1.78-.17,0-.57-.03-.74-.05v-6.58c.25-.22,.57-.52,1.14-.52,.52,0,.81,.25,1.11,.52v4.86h0Zm8.78,2.74l5.03-3.13v-6.85l-3.25-2.39-5.03,2.88v6.78l-.99,.79,.1,.15,.81-.67,3.33,2.44h0Zm-.37-3.55v-7.3l2.51,1.87v7.3l-2.51-1.87Zm15.01-8.65c-.39,.27-.74,.42-1.11,.42-.39,0-.88-.25-1.14-.57,0,.03-1.87,2.02-1.87,2.02l-1.87-2.02-3.05,2.12,.1,.17,.81-.54,1.11,1.21v6.63l-1.33,1.01,.12,.12,.67-.46,2.49,2.12,3.15-2.09-.1-.15-.81,.49-1.28-1.16v-7.28c.52,.57,1.11,1.06,1.82,1.06,1.28,0,2.14-1.53,2.29-3.11m11.88,9.81l-.94,.59-5.2-7.76,.27-.37c.57,.34,1.08,.81,2.17,.81s2.47-1.14,2.59-3.23c-.27,.37-.81,.81-1.7,.81-.64,0-1.28-.42-1.67-.81l-3.55,5.22,4.71,7.17,3.42-2.27-.1-.17h0Zm-6.31,.19l-.79,.52-1.08-1.08V.48l-3.89,2.69c.45,.15,.99,.39,.99,1.43v11.81l-1.33,1.01,.12,.12,.67-.46,2.32,2.12,3.11-2.07-.1-.15h0Zm22.89-14.39c0-2.02-1.92-2.63-3.53-2.56V.19c.96,.07,1.7,.46,1.7,1.11,0,.45-.32,1.01-1.28,1.01-.76,0-2.02-.45-3.2-.84-1.3-.45-2.54-.87-3.57-.87-2.02,0-3.55,1.5-3.55,3.35,0,1.5,1.16,2.02,1.63,2.21l.03-.07c-.3-.2-.49-.42-.49-1.06,0-.54,.39-1.26,1.43-1.26,.94,0,2.17,.42,3.8,.88,1.4,.39,2.91,.76,3.75,.87v3.28l-1.58,1.3,1.58,1.36v4.49c-.81,.46-1.75,.61-2.56,.61-1.5,0-2.89-.42-4.02-1.68l4.26-2.07V5.73l-5.2,2.32c.54-1.38,1.55-2.41,2.66-3.08l-.03-.08c-3.08,.84-5.89,3.67-5.89,7.17,0,4.19,3.35,7.3,7.22,7.3,4.19,0,6.65-3.28,6.61-6.75h-.07c-.61,1.33-1.63,2.59-2.78,3.25v-4.38l1.65-1.33-1.65-1.33v-3.28c1.53,0,3.11-1.01,3.11-2.96m-8.78,11.56l-1.21,.61c-.74-.96-1.23-2.32-1.23-4.07,0-.72,.07-1.7,.32-2.39l2.14-.96-.03,6.8h0Zm11.93-12.31l-2.17,1.82,1.85,2.09,2.17-1.82-1.85-2.09Zm3.3,15.15l-.79,.52-1.08-1.08v-7.17l.91-.72-.12-.15-.76,.59-1.8-2.14-2.96,2.07,.1,.17,.74-.49,.99,1.23v6.61l-1.33,1.01,.12,.12,.67-.46,2.32,2.12,3.11-2.07-.1-.15h0Zm16.63-.1l-.74,.49-1.16-1.11v-7.03l.94-.72-.12-.15-.84,.64-2.47-2.2-2.78,2.17-2.44-2.17-2.74,2.14-1.85-2.14-2.96,2.07,.1,.17,.74-.49,1.06,1.21v6.61l-.81,.81,2.36,2,2.29-2.07-.94-.88v-7.04l.61-.45,1.7,1.48v6.16l-.79,.81,2.39,2,2.24-2.07-.94-.88v-7.04l.59-.47,1.72,1.5v6.06l-.69,.72,2.41,2.2,3.18-2.17-.1-.15h.02Zm8.6-1.5l-2.36,1.87-2.71-2.14v-1.33l4.68-3.3-2.36-3.67-5.2,2.86v6.93l3.57,2.59,4.51-3.62-.12-.17h0Zm-5.08-1.88v-5.15l2.27,3.55-2.27,1.6Zm14.12-.97l-2-1.53c1.33-1.16,1.8-2.63,1.8-3.69,0-.15-.03-.42-.05-.67h-.08c-.19,.54-.72,1.01-1.53,1.01s-1.26-.45-1.75-.99l-4.58,2.54v3.72l1.75,1.38c-1.75,1.55-2.09,2.51-2.09,3.4s.52,1.67,1.41,2.02l.07-.12c-.22-.19-.42-.32-.42-.79,0-.34,.35-.88,1.14-.88,1.01,0,1.63,.69,1.95,1.06,0-.03,4.38-2.69,4.38-2.69v-3.77h0Zm-1.03-3.05c-.69,1.23-2.21,2.44-3.11,3.13l-1.11-.94v-3.62c.45,.99,1.36,1.82,2.54,1.82,.69,0,1.14-.12,1.67-.39m-1.9,8.13c-.52-1.16-1.63-2-2.86-2-.3,0-1.21-.03-2,.46,.47-.79,1.87-2.21,3.65-3.28l1.21,1.01v3.8Z"></path></svg></a></div><div class="css-8pe5zk"><ul class="css-42kif7" data-testid="masthead-edition-menu"><li class="css-1shpxcj"><a aria-current="page" class="css-1baq5tz" href="/" lang="en-US">U.S.</a></li><li class="css-1nrdlje" data-testid="edition-menu-international"><a class="css-1baq5tz" href="/international/" lang="en">International</a></li><li class="css-1nrdlje" data-testid="edition-menu-canada"><a class="css-1baq5tz" href="/ca/" lang="en-CA">Canada</a></li><li class="css-1nrdlje" data-testid="edition-menu-spanish"><a class="css-1baq5tz" href="https://www.nytimes.com/es/" lang="es-ES">Español</a></li><li class="css-1nrdlje" data-testid="edition-menu-chinese"><a class="css-1baq5tz" href="https://cn.nytimes.com" lang="zh-hans">中文</a></li></ul></div><div class="css-50gbj e1j3jvdr1"></div></section><section class="hasLinks css-15el443 e1pjtsj62" id="masthead-bar-one"><div><div class="css-e2a84x e1pjtsj60"><span> </span></div><div class="css-bfvq22 e1pjtsj61"><a class="css-j184jz" href="https://www.nytimes.com/section/todayspaper">Today’s Paper</a></div></div><div class="css-vryaoq" id="masthead-bar-one-widgets"><div class="css-77hcv e55wwux2"></div></div><div class="css-qebcue"></div></section><div class="css-stscvm"><div class="css-158f1cv" data-testid="masthead-desktop-logo"><a aria-label="New York Times homepage" class="css-1qcrlqu eoab3xr0" href="/"><svg aria-hidden="true" fill="#000000" viewbox="0 0 184 25"><path d="M14.57,2.57C14.57,.55,12.65-.06,11.04,.01V.19c.96,.07,1.7,.46,1.7,1.11,0,.45-.32,1.01-1.28,1.01-.76,0-2.02-.45-3.2-.84-1.3-.45-2.54-.87-3.57-.87-2.02,0-3.55,1.5-3.55,3.36,0,1.5,1.16,2.02,1.63,2.21l.03-.07c-.3-.2-.49-.42-.49-1.06,0-.54,.39-1.26,1.43-1.26,.94,0,2.17,.42,3.8,.88,1.4,.39,2.91,.76,3.75,.87v3.28l-1.58,1.3,1.58,1.36v4.49c-.81,.46-1.75,.61-2.56,.61-1.5,0-2.88-.42-4.02-1.68l4.26-2.07V5.73l-5.2,2.32c.54-1.38,1.55-2.41,2.66-3.08l-.03-.08C3.31,5.73,.5,8.56,.5,12.06c0,4.19,3.35,7.3,7.22,7.3,4.19,0,6.65-3.28,6.61-6.75h-.08c-.61,1.33-1.63,2.59-2.78,3.25v-4.38l1.65-1.33-1.65-1.33v-3.28c1.53,0,3.11-1.01,3.11-2.96M5.8,14.13l-1.21,.61c-.74-.96-1.23-2.32-1.23-4.07,0-.72,.08-1.7,.32-2.39l2.14-.96-.03,6.8h0Zm19.47-5.76l-.81,.64-2.47-2.2-2.86,2.21V.48l-3.89,2.69c.45,.15,.99,.39,.99,1.43v11.81l-1.33,1.01,.12,.12,.67-.46,2.32,2.12,3.11-2.07-.1-.15-.79,.52-1.08-1.08v-7.12l.74-.54,1.7,1.48v6.19c0,3.92-.87,4.73-2.63,5.37v.1c2.93,.12,5.57-.87,5.57-5.89v-6.75l.88-.72-.12-.15h0Zm5.22,10.8l4.51-3.62-.12-.17-2.36,1.87-2.71-2.14v-1.33l4.68-3.3-2.36-3.67-5.2,2.86v6.8l-1.01,.79,.12,.15,.96-.76,3.5,2.54h-.01Zm-.69-5.67v-5.15l2.27,3.55-2.27,1.6ZM53.65,1.61c0-.32-.08-.59-.2-.96h-.07c-.32,.87-.67,1.33-1.68,1.33-.88,0-1.58-.54-1.95-.94,0,.03-2.96,3.42-2.96,3.42l.15,.12,.84-.96c.64,.49,1.21,1.06,2.63,1.08V13.34l-6.06-10.5c-.47-.79-1.28-1.97-2.66-1.97-1.63,0-2.86,1.4-2.66,3.77h.1c.12-.59,.47-1.33,1.18-1.33,.57,0,1.03,.54,1.3,1.03v3.38c-1.87,0-2.93,.87-2.93,2.34,0,.61,.45,1.94,1.72,2.17v-.07c-.17-.17-.34-.32-.34-.67,0-.57,.42-.88,1.18-.88,.12,0,.3,.03,.37,.05v4.38c-2.2,.03-3.89,1.23-3.89,3.31s1.7,2.88,3.47,2.78v-.07c-1.11-.12-1.68-.69-1.68-1.5,0-.88,.64-1.36,1.45-1.36s1.43,.52,1.95,1.11l2.96-3.33-.12-.12-.76,.87c-1.14-1.01-1.87-1.48-3.18-1.68V4.67l8.36,14.57h.45V4.72c1.6-.1,3.03-1.3,3.03-3.11m2.81,17.54l4.51-3.62-.12-.17-2.36,1.87-2.71-2.14v-1.33l4.68-3.3-2.36-3.67-5.2,2.86v6.8l-1.01,.79,.12,.15,.96-.76,3.5,2.54h0Zm-.69-5.67v-5.15l2.27,3.55-2.27,1.6Zm21.22-5.52l-.69,.52-1.97-1.68-2.29,2.07,.94,.88v7.72l-2.34-1.6v-6.26l.81-.57-2.41-2.24-2.24,2.07,.94,.88v7.46l-.15,.1-2.2-1.6v-6.13c0-1.43-.72-1.85-1.63-2.41-.76-.47-1.16-.91-1.16-1.63,0-.79,.69-1.11,.91-1.23-.79-.03-2.98,.76-3.03,2.76-.03,1.03,.47,1.48,.99,1.97,.52,.49,1.01,.96,1.01,1.83v6.01l-1.06,.84,.12,.12,1.01-.79,2.63,2.14,2.51-1.75,2.76,1.75,5.42-3.2v-6.95l1.21-.94-.1-.15h0Zm18.15-5.84l-1.03,.94-2.32-2.02-3.13,2.51V1.19h-.19V18.12c-.34-.05-1.06-.25-1.85-.37V3.58c0-1.03-.74-2.47-2.59-2.47s-3.01,1.56-3.01,2.91h.08c.1-.61,.52-1.16,1.13-1.16s1.18,.39,1.18,1.78v4.04c-1.75,.07-2.81,1.16-2.81,2.34,0,.67,.42,1.92,1.75,1.97v-.1c-.45-.19-.54-.42-.54-.67,0-.59,.57-.79,1.36-.79h.19v6.51c-1.5,.52-2.2,1.53-2.2,2.78,0,1.72,1.38,3.05,3.4,3.05,1.43,0,2.44-.25,3.75-.54,1.06-.22,2.21-.47,2.83-.47,.79,0,1.14,.35,1.14,.91,0,.72-.27,1.08-.69,1.21v.1c1.7-.32,2.69-1.3,2.69-2.83s-1.5-2.54-3.18-2.54c-.87,0-2.44,.27-3.72,.57-1.43,.32-2.66,.47-3.11,.47-.72,0-1.6-.32-1.6-1.28,0-.87,.72-1.56,2.49-1.56,.96,0,1.9,.15,3.08,.42,1.26,.27,2.12,.64,3.2,.64,1.5,0,2.71-.54,2.71-2.74V3.29l1.11-1.01-.12-.15h0Zm-4.24,6.78c-.27,.3-.59,.54-1.11,.54-.57,0-.87-.3-1.14-.54V3.81l.74-.59,1.5,1.28v4.41h0Zm0,2.41c-.25-.25-.57-.47-1.11-.47s-.91,.27-1.14,.47v-2.17c.22,.19,.59,.49,1.14,.49s.87-.25,1.11-.49v2.17Zm0,5.1c0,.84-.42,1.78-1.5,1.78-.17,0-.57-.03-.74-.05v-6.58c.25-.22,.57-.52,1.14-.52,.52,0,.81,.25,1.11,.52v4.86h0Zm8.78,2.74l5.03-3.13v-6.85l-3.25-2.39-5.03,2.88v6.78l-.99,.79,.1,.15,.81-.67,3.33,2.44h0Zm-.37-3.55v-7.3l2.51,1.87v7.3l-2.51-1.87Zm15.01-8.65c-.39,.27-.74,.42-1.11,.42-.39,0-.88-.25-1.14-.57,0,.03-1.87,2.02-1.87,2.02l-1.87-2.02-3.05,2.12,.1,.17,.81-.54,1.11,1.21v6.63l-1.33,1.01,.12,.12,.67-.46,2.49,2.12,3.15-2.09-.1-.15-.81,.49-1.28-1.16v-7.28c.52,.57,1.11,1.06,1.82,1.06,1.28,0,2.14-1.53,2.29-3.11m11.88,9.81l-.94,.59-5.2-7.76,.27-.37c.57,.34,1.08,.81,2.17,.81s2.47-1.14,2.59-3.23c-.27,.37-.81,.81-1.7,.81-.64,0-1.28-.42-1.67-.81l-3.55,5.22,4.71,7.17,3.42-2.27-.1-.17h0Zm-6.31,.19l-.79,.52-1.08-1.08V.48l-3.89,2.69c.45,.15,.99,.39,.99,1.43v11.81l-1.33,1.01,.12,.12,.67-.46,2.32,2.12,3.11-2.07-.1-.15h0Zm22.89-14.39c0-2.02-1.92-2.63-3.53-2.56V.19c.96,.07,1.7,.46,1.7,1.11,0,.45-.32,1.01-1.28,1.01-.76,0-2.02-.45-3.2-.84-1.3-.45-2.54-.87-3.57-.87-2.02,0-3.55,1.5-3.55,3.35,0,1.5,1.16,2.02,1.63,2.21l.03-.07c-.3-.2-.49-.42-.49-1.06,0-.54,.39-1.26,1.43-1.26,.94,0,2.17,.42,3.8,.88,1.4,.39,2.91,.76,3.75,.87v3.28l-1.58,1.3,1.58,1.36v4.49c-.81,.46-1.75,.61-2.56,.61-1.5,0-2.89-.42-4.02-1.68l4.26-2.07V5.73l-5.2,2.32c.54-1.38,1.55-2.41,2.66-3.08l-.03-.08c-3.08,.84-5.89,3.67-5.89,7.17,0,4.19,3.35,7.3,7.22,7.3,4.19,0,6.65-3.28,6.61-6.75h-.07c-.61,1.33-1.63,2.59-2.78,3.25v-4.38l1.65-1.33-1.65-1.33v-3.28c1.53,0,3.11-1.01,3.11-2.96m-8.78,11.56l-1.21,.61c-.74-.96-1.23-2.32-1.23-4.07,0-.72,.07-1.7,.32-2.39l2.14-.96-.03,6.8h0Zm11.93-12.31l-2.17,1.82,1.85,2.09,2.17-1.82-1.85-2.09Zm3.3,15.15l-.79,.52-1.08-1.08v-7.17l.91-.72-.12-.15-.76,.59-1.8-2.14-2.96,2.07,.1,.17,.74-.49,.99,1.23v6.61l-1.33,1.01,.12,.12,.67-.46,2.32,2.12,3.11-2.07-.1-.15h0Zm16.63-.1l-.74,.49-1.16-1.11v-7.03l.94-.72-.12-.15-.84,.64-2.47-2.2-2.78,2.17-2.44-2.17-2.74,2.14-1.85-2.14-2.96,2.07,.1,.17,.74-.49,1.06,1.21v6.61l-.81,.81,2.36,2,2.29-2.07-.94-.88v-7.04l.61-.45,1.7,1.48v6.16l-.79,.81,2.39,2,2.24-2.07-.94-.88v-7.04l.59-.47,1.72,1.5v6.06l-.69,.72,2.41,2.2,3.18-2.17-.1-.15h.02Zm8.6-1.5l-2.36,1.87-2.71-2.14v-1.33l4.68-3.3-2.36-3.67-5.2,2.86v6.93l3.57,2.59,4.51-3.62-.12-.17h0Zm-5.08-1.88v-5.15l2.27,3.55-2.27,1.6Zm14.12-.97l-2-1.53c1.33-1.16,1.8-2.63,1.8-3.69,0-.15-.03-.42-.05-.67h-.08c-.19,.54-.72,1.01-1.53,1.01s-1.26-.45-1.75-.99l-4.58,2.54v3.72l1.75,1.38c-1.75,1.55-2.09,2.51-2.09,3.4s.52,1.67,1.41,2.02l.07-.12c-.22-.19-.42-.32-.42-.79,0-.34,.35-.88,1.14-.88,1.01,0,1.63,.69,1.95,1.06,0-.03,4.38-2.69,4.38-2.69v-3.77h0Zm-1.03-3.05c-.69,1.23-2.21,2.44-3.11,3.13l-1.11-.94v-3.62c.45,.99,1.36,1.82,2.54,1.82,.69,0,1.14-.12,1.67-.39m-1.9,8.13c-.52-1.16-1.63-2-2.86-2-.3,0-1.21-.03-2,.46,.47-.79,1.87-2.21,3.65-3.28l1.21,1.01v3.8Z"></path></svg></a></div></div><div class="css-1x8fy0n"></div><div aria-hidden="true" class="css-v21ltd" style="visibility:hidden"><div class="css-1llhclm"><nav aria-label="Main" data-testid="floating-desktop-nested-nav"><ul class="css-14m0cem"><li class="css-1qtaxzf" data-navid="U.S." data-testid="nav-item-U.S."><a class="css-u90q6n" data-navid="U.S." href="https://www.nytimes.com/section/us">U.S.</a><button aria-label="open U.S. submenu" class="css-1bp1dzo" data-navid="U.S." type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="U.S. submenu" class="css-ljayxv" data-testid="nav-dropdown-U.S."><div class="css-13qj3r5"><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="U.S.-links-column-header">Sections</h3><ul aria-labelledby="U.S.-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/us">U.S.</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/politics">Politics</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/nyregion">New York</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/california-news">California</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/education">Education</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/health">Health</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/obituaries">Obituaries</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/science">Science</a></li></ul></div></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="U.S.-links-column-header"></h3><ul aria-labelledby="U.S.-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/climate">Climate</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/weather">Weather</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/sports">Sports</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business">Business</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/technology">Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/upshot">The Upshot</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/magazine">The Magazine</a></li></ul></div></div><div class="css-132p2yg"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="U.S.-links-column-header">Top Stories</h3><ul aria-labelledby="U.S.-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/trump-transition">Trump Transition</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/topic/organization/us-supreme-court">Supreme Court</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/topic/organization/us-congress">Congress</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/immigration-us">Immigration</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/abortion-news">Abortion</a></li></ul></div></div><div class="css-132p2yg"><div id="U.S.-thumbnail-column"><h3 class="css-1nudrh3" id="U.S.-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="U.S.-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/morning-briefing"><img alt="The Morning Logo" class="" src="/vi-assets/static-assets/icon-the-morning_144x144-b12a6923b6ad9102b766352261b1a847.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Morning</div><p>Make sense of the day’s news and ideas.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/upshot"><img alt="The Upshot Logo" class="" src="/vi-assets/static-assets/icon-the-upshot_144x144-0b1553ff703bbd07ac8fe73e6d215888.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Upshot</div><p>Analysis that explains politics, policy and everyday life.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div><div class="css-1didtc4"></div><div class="css-132p2yg"><div id="U.S.-thumbnail-column"><h3 class="css-1nudrh3" id="U.S.-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="U.S.-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/the-daily"><img alt="The Daily Logo" class="" src="https://static01.nyt.com/images/2017/01/29/podcasts/the-daily-album-art/the-daily-album-art-mediumSquare149-v3.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">The Daily</div><p>The biggest stories of our time, in 20 minutes a day.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/column/election-run-up-podcast"><img alt="The Run-Up Logo" class="css-hqhlyo" src="https://static01.nyt.com/images/2022/08/29/podcasts/the-run-up-album-art/the-run-up-album-art-thumbLarge.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">The Run-Up</div><p>On the campaign trail with Astead Herndon.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="World" data-testid="nav-item-World"><a class="css-u90q6n" data-navid="World" href="https://www.nytimes.com/section/world">World</a><button aria-label="open World submenu" class="css-1bp1dzo" data-navid="World" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="World submenu" class="css-ljayxv" data-testid="nav-dropdown-World"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="World-links-column-header">Sections</h3><ul aria-labelledby="World-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world">World</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/africa">Africa</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/americas">Americas</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/asia">Asia</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/australia">Australia</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/canada">Canada</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/europe">Europe</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/middleeast">Middle East</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/science">Science</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/climate">Climate</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/weather">Weather</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/health">Health</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/obituaries">Obituaries</a></li></ul></div></div><div class="css-167b3qw"></div><div class="css-r6g2pn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="World-links-column-header">Top Stories</h3><ul aria-labelledby="World-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/israel-hamas-gaza">Middle East Crisis</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/ukraine-russia">Russia-Ukraine War</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/china-relations">China International Relations</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-global-profile">The Global Profile</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/es/">Leer en Español</a></li></ul></div></div><div class="css-132p2yg"><div id="World-thumbnail-column"><h3 class="css-1nudrh3" id="World-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="World-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/morning-briefing-europe"><img alt="Morning Briefing: Europe Logo" class="" src="/vi-assets/static-assets/icon-europe-morning-briefing_144x144-f0a330cb12ba0c31f81f13e25f6d0d18.webp"/><div><div class="css-1xpp3bj e10k0zts0">Morning Briefing: Europe</div><p>Get what you need to know to start your day.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/the-interpreter"><img alt="The Interpreter Logo" class="" src="/vi-assets/static-assets/icon-the-interpreter_144x144-b29b74b2ebedb8e74823f33b16fb8167.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Interpreter</div><p>Original analysis on the week’s biggest global stories.</p></div></a></li></ul></div></div><div class="css-132p2yg"><div id="World-thumbnail-column"><h3 class="css-1nudrh3" id="World-thumbnail-column-header"></h3><ul aria-labelledby="World-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/your-places-global-update"><img alt="Your Places: Global Update Logo" class="" src="/vi-assets/static-assets/icon-yourplaces-globalupdate_144x144-c25aba1c2904f301a08ad33183f723c6.webp"/><div><div class="css-1xpp3bj e10k0zts0">Your Places: Global Update</div><p>The latest news for any part of the world you select.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/canada-letter"><img alt="Canada Letter Logo" class="" src="/vi-assets/static-assets/icon-canada-letter_144x144-65d899377edbcce9773d31fd03a77e8d.webp"/><div><div class="css-1xpp3bj e10k0zts0">Canada Letter</div><p>Backstories and analysis from our Canadian correspondents.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Business" data-testid="nav-item-Business"><a class="css-u90q6n" data-navid="Business" href="https://www.nytimes.com/section/business">Business</a><button aria-label="open Business submenu" class="css-1bp1dzo" data-navid="Business" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Business submenu" class="css-ljayxv" data-testid="nav-dropdown-Business"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Business-links-column-header">Sections</h3><ul aria-labelledby="Business-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business">Business</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/technology">Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business/economy">Economy</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business/media">Media</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/markets-overview">Finance and Markets</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Business-links-column-header"></h3><ul aria-labelledby="Business-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business/dealbook">DealBook</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/technology/personaltech">Personal Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business/energy-environment">Energy Transition</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/your-money">Your Money</a></li></ul></div></div><div class="css-r6g2pn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Business-links-column-header">Top Stories</h3><ul aria-labelledby="Business-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/economy-business-us">U.S. Economy</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/markets-overview">Stock Market</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/artificial-intelligence">Artificial Intelligence</a></li></ul></div></div><div class="css-132p2yg"><div id="Business-thumbnail-column"><h3 class="css-1nudrh3" id="Business-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Business-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/dealbook"><img alt="DealBook Logo" class="" src="/vi-assets/static-assets/icon-dealbook_144x144-28e8f71aafff426804c3a92b1b176e07.webp"/><div><div class="css-1xpp3bj e10k0zts0">DealBook</div><p>The most crucial business and policy news you need to know.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div><div class="css-132p2yg"><div id="Business-thumbnail-column"><h3 class="css-1nudrh3" id="Business-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="Business-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/hard-fork"><img alt="Hard Fork Logo" class="" src="https://static01.nyt.com/images/2022/09/28/podcasts/hard-fork-album-art/hard-fork-album-art-mediumSquare149-v2.png?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Hard Fork</div><p>Our tech journalists help you make sense of the rapidly changing tech world.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Arts" data-testid="nav-item-Arts"><a class="css-u90q6n" data-navid="Arts" href="https://www.nytimes.com/section/arts">Arts</a><button aria-label="open Arts submenu" class="css-1bp1dzo" data-navid="Arts" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Arts submenu" class="css-ljayxv" data-testid="nav-dropdown-Arts"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Arts-links-column-header">Sections</h3><ul aria-labelledby="Arts-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts">Today's Arts</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/books">Book Review</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/books/best-sellers">Best Sellers</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts/dance">Dance</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/movies">Movies</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts/music">Music</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Arts-links-column-header"></h3><ul aria-labelledby="Arts-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts/television">Television</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/theater">Theater</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/pop-culture">Pop Culture</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/t-magazine">T Magazine</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts/design">Visual Arts</a></li></ul></div></div><div class="css-r6g2pn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Arts-links-column-header">Recommendations</h3><ul aria-labelledby="Arts-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/interactive/2024/books/best-books-21st-century.html">100 Best Books of the 21st Century</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/critics-picks">Critic’s Picks</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/books-to-read">What to Read</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/what-to-watch">What to Watch</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/playlist">What to Listen To</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/interactive/2023/10/27/arts/music/music-fivemins-collection.html">5 Minutes to Make You Love Music</a></li></ul></div></div><div class="css-132p2yg"><div id="Arts-thumbnail-column"><h3 class="css-1nudrh3" id="Arts-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Arts-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/read-like-the-wind"><img alt="Read Like the Wind Logo" class="" src="/vi-assets/static-assets/icon-read-like-the-wind_144x144-5bcf9faf41d0b49df1df29e59a868b36.webp"/><div><div class="css-1xpp3bj e10k0zts0">Read Like the Wind</div><p>Book recommendations from our critics.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/watching"><img alt="Watching Logo" class="" src="/vi-assets/static-assets/icon-watching_144x144-631a1da177f9fda1a7f4614ad8e607bd.webp"/><div><div class="css-1xpp3bj e10k0zts0">Watching</div><p>Streaming TV and movie recommendations.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div><div class="css-132p2yg"><div id="Arts-thumbnail-column"><h3 class="css-1nudrh3" id="Arts-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="Arts-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/book-review-podcast"><img alt="Book Review Logo" class="" src="https://static01.nyt.com/images/2018/03/27/books/book-review-album-art-v2/book-review-album-art-v2-thumbLarge-v3.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Book Review</div><p>The podcast that takes you inside the literary world.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/column/popcast-pop-music-podcast"><img alt="Popcast Logo" class="" src="https://static01.nyt.com/images/2011/05/20/multimedia/music-popcast/music-popcast-thumbLarge-v3.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Popcast</div><p>Pop music news, new songs and albums, and artists of note.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Lifestyle" data-testid="nav-item-Lifestyle"><a class="css-u90q6n" data-navid="Lifestyle" href="https://www.nytimes.com/spotlight/lifestyle">Lifestyle</a><button aria-label="open Lifestyle submenu" class="css-1bp1dzo" data-navid="Lifestyle" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Lifestyle submenu" class="css-ljayxv" data-testid="nav-dropdown-Lifestyle"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Lifestyle-links-column-header">Sections</h3><ul aria-labelledby="Lifestyle-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/lifestyle">All Lifestyle</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/well">Well</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/travel">Travel</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/style">Style</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/realestate">Real Estate</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Lifestyle-links-column-header"></h3><ul aria-labelledby="Lifestyle-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/food">Food</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/fashion/weddings">Love</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/your-money">Your Money</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/technology/personaltech">Personal Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/t-magazine">T Magazine</a></li></ul></div></div><div class="css-r6g2pn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Lifestyle-links-column-header">Columns</h3><ul aria-labelledby="Lifestyle-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/36-hours">36 Hours</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/ask-well">Ask Well</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-hunt">The Hunt</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/modern-love">Modern Love</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/best-restaurants">Where to Eat</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/vows">Vows</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/social-qs">Social Q’s</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-ethicist">The Ethicist</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/ask-the-therapist">Ask the Therapist</a></li></ul></div></div><div class="css-132p2yg"><div id="Lifestyle-thumbnail-column"><h3 class="css-1nudrh3" id="Lifestyle-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Lifestyle-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/open-thread-fashion"><img alt="Open Thread Logo" class="" src="/vi-assets/static-assets/icon-open-thread-fashion_144x144-8e1b4b3fd68c2f333faa63097da2249b.webp"/><div><div class="css-1xpp3bj e10k0zts0">Open Thread</div><p>The latest news on what we wear, by our chief fashion critic.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/well"><img alt="Well Logo" class="" src="/vi-assets/static-assets/icon-well_144x144-433c9d15dc985dded9b705942592c6fb.webp"/><div><div class="css-1xpp3bj e10k0zts0">Well</div><p>Essential news and guidance to live your healthiest life.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div><div class="css-132p2yg"><div id="Lifestyle-thumbnail-column"><h3 class="css-1nudrh3" id="Lifestyle-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="Lifestyle-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/modern-love-podcast"><img alt="Modern Love Logo" class="" src="https://static01.nyt.com/images/2020/09/21/podcasts/modernlove-logo/modernlove-logo-thumbLarge-v3.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Modern Love</div><p>The complicated love lives of real people.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Opinion" data-testid="nav-item-Opinion"><a class="css-u90q6n" data-navid="Opinion" href="https://www.nytimes.com/section/opinion">Opinion</a><button aria-label="open Opinion submenu" class="css-1bp1dzo" data-navid="Opinion" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Opinion submenu" class="css-ljayxv" data-testid="nav-dropdown-Opinion"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Opinion-links-column-header">Sections</h3><ul aria-labelledby="Opinion-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion">Opinion</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/contributors">Guest Essays</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/editorials">Editorials</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/op-docs">Op-Docs</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/opinion-video">Videos</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/letters">Letters</a></li></ul></div></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Opinion-links-column-header">Topics</h3><ul aria-labelledby="Opinion-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/politics">Politics</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/international-world">World</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/business-economics">Business</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/technology">Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/environment">Climate</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/health">Health</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/culture">Culture</a></li></ul></div></div><div class="css-167b3qw"></div><div class="css-9jpfr0"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Opinion-links-column-header">Columnists</h3><ul aria-labelledby="Opinion-links-column-header" class="css-34zueq"><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/jamelle-bouie">Jamelle Bouie</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/david-brooks">David Brooks</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/gail-collins">Gail Collins</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/ross-douthat">Ross Douthat</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/maureen-dowd">Maureen Dowd</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/david-french">David French</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/thomas-l-friedman">Thomas L. Friedman</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/m-gessen">M. Gessen</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/michelle-goldberg">Michelle Goldberg</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/ezra-klein">Ezra Klein</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/nicholas-kristof">Nicholas Kristof</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/carlos-lozada">Carlos Lozada</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/tressie-mcmillan-cottom">Tressie McMillan Cottom</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/pamela-paul">Pamela Paul</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/lydia-polgreen">Lydia Polgreen</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/bret-stephens">Bret Stephens</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/zeynep-tufekci">Zeynep Tufekci</a></li></ul></div></div><div class="css-132p2yg"><div id="Opinion-thumbnail-column"><h3 class="css-1nudrh3" id="Opinion-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="Opinion-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/matter-of-opinion"><img alt="Matter of Opinion Logo" class="" src="https://static01.nyt.com/images/2023/05/08/podcasts/matter-of-opinion-album-art/matter-of-opinion-album-art-thumbLarge-v2.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Matter of Opinion</div><p>Thoughts, aloud. With Michelle Cottle, Ross Douthat, Carlos Lozada and Lydia Polgreen.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/column/ezra-klein-podcast"><img alt="The Ezra Klein Show Logo" class="" src="https://static01.nyt.com/images/2023/04/05/podcasts/ezra-klein-album-art/ezra-klein-album-art-thumbLarge-v3.png"/><div><div class="css-1xpp3bj e10k0zts0">The Ezra Klein Show</div><p>Discussions of ideas that matter, plus book recommendations.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Audio" data-testid="nav-item-Audio"><a class="css-u90q6n" data-navid="Audio" href="https://www.nytimes.com/spotlight/podcasts">Audio</a><button aria-label="open Audio submenu" class="css-1bp1dzo" data-navid="Audio" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Audio submenu" class="css-ljayxv" data-testid="nav-dropdown-Audio"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="Audio-brand-column"><a href="https://www.nytimes.com/spotlight/podcasts"><h3>Audio</h3><p>Podcasts and narrated articles covering news, tech, culture and more.</p></a><a class="css-1q7rq5u" href="https://www.nytimes.com/audio/app">Download the Audio app on iOS.</a></div></div><div class="css-15iksc8"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Audio-links-column-header">Listen</h3><ul aria-labelledby="Audio-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-headlines">The Headlines</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-daily">The Daily</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/hard-fork">Hard Fork</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/ezra-klein-podcast">The Ezra Klein Show</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/matter-of-opinion">Matter of Opinion</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/interactive/2022/podcasts/serial-productions.html">Serial Productions</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/book-review-podcast">The Book Review Podcast</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/modern-love-podcast">Modern Love</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/election-run-up-podcast">The Run-Up</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/popcast-pop-music-podcast">Popcast</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/reporter-reads">Reporter Reads</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/the-sunday-read">The Sunday Read</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-culture-desk">The Culture Desk</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-interview">The Interview</a></li></ul><a class="css-c38y3b" href="https://www.nytimes.com/spotlight/podcasts">See all audio</a></div></div><div class="css-132p2yg"><div id="Audio-thumbnail-column"><h3 class="css-1nudrh3" id="Audio-thumbnail-column-header">Featured</h3><ul aria-labelledby="Audio-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/the-interview"><img alt="The Interview Logo" class="" src="/vi-assets/static-assets/NYT-TheInterview-0232c6c95d42d77941fd3d8e5d2776cb.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Interview</div><p>Conversations with the world’s most fascinating people.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/column/the-headlines"><img alt="The Headlines Logo" class="" src="https://static01.nyt.com/images/2022/10/12/podcasts/headlines-albumartwork-audioapp-2/headlines-albumartwork-audioapp-2-thumbLarge.png?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">The Headlines</div><p>Your morning listen. Top stories, in 10 minutes.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/interactive/2024/podcasts/serial-good-whale.html"><img alt="Serial: The Good Whale Logo" class="" src="/vi-assets/static-assets/TheGoodWhale_144x144-f0eb13463973a1da8814328998990640.webp"/><div><div class="css-1xpp3bj e10k0zts0">Serial: The Good Whale</div><p>A Hollywood orca's real-life odyssey back to the ocean.</p></div></a></li></ul></div></div><div class="css-132p2yg"><div id="Audio-thumbnail-column"><h3 class="css-1nudrh3" id="Audio-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Audio-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/audio"><img alt="Audio Logo" class="" src="/vi-assets/static-assets/icon-audio_144x144-dc00c6581be29065cbd19ec7a83a3767.webp"/><div><div class="css-1xpp3bj e10k0zts0">Audio</div><p>Our editors share their favorite listens from the New York Times Audio app.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div><div class="css-1qt2b4r">Audio<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li><li class="css-1qtaxzf" data-navid="Games" data-testid="nav-item-Games"><a class="css-u90q6n" data-navid="Games" href="https://www.nytimes.com/crosswords">Games</a><button aria-label="open Games submenu" class="css-1bp1dzo" data-navid="Games" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Games submenu" class="css-ljayxv" data-testid="nav-dropdown-Games"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="Games-brand-column"><a href="https://www.nytimes.com/crosswords"><h3>Games</h3><p>Word games, logic puzzles and crosswords, including an extensive archive.</p></a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs" id="Games-links-icons-column"><h3 class="css-1nudrh3" id="Games-links-icons-column-header">Play</h3><ul aria-labelledby="Games-links-icons-column-header"><li><a class="css-ed0o7b" href="https://www.nytimes.com/puzzles/spelling-bee"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/spelling-bee.svg)"></i><p><span>Spelling Bee</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/crosswords/game/mini"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/mini.svg)"></i><p><span>The Mini Crossword</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/games/wordle/index.html"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/wordle.svg)"></i><p><span>Wordle</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/crosswords/game/daily/"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/daily.svg)"></i><p><span>The Crossword</span> </p></a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs" id="Games-links-icons-column"><h3 class="css-1nudrh3" id="Games-links-icons-column-header"></h3><ul aria-labelledby="Games-links-icons-column-header"><li><a class="css-ed0o7b" href="https://www.nytimes.com/games/strands"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/strands.svg)"></i><p><span>Strands</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/games/connections"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/connections.svg)"></i><p><span>Connections</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/puzzles/sudoku"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/expansion-games/sudoku-card-icon.svg)"></i><p><span>Sudoku</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/puzzles/letter-boxed"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/letter-boxed.svg)"></i><p><span>Letter Boxed</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/puzzles/tiles"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/tiles.svg)"></i><p><span>Tiles</span> </p></a></li></ul></div></div><div class="css-132p2yg"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Games-links-column-header">Community</h3><ul aria-labelledby="Games-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/spelling-bee-forum">Spelling Bee Forum</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/daily-crossword-column">Wordplay Column</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/wordle-review">Wordle Review</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/article/submit-crossword-puzzles-the-new-york-times.html">Submit a Crossword</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/puzzle-making">Meet Our Crossword Constructors</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/2022/09/19/crosswords/mini-to-maestro-part-1.html">Mini to Maestro</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/interactive/2022/upshot/wordle-bot.html">Wordlebot</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/puzzle-personality">Take the Puzzle Personality Quiz</a></li></ul></div></div><div class="css-132p2yg"><div id="Games-thumbnail-column"><h3 class="css-1nudrh3" id="Games-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Games-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/gameplay"><img alt="Gameplay Logo" class="" src="/vi-assets/static-assets/icon-gameplay_144x144-b6cc5e2a7cc27a43096274a02921329c.webp"/><div><div class="css-1xpp3bj e10k0zts0">Gameplay</div><p>Puzzles, brain teasers, solving tips and more.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/easy-mode"><img alt="Easy Mode Logo" class="" src="/vi-assets/static-assets/icon-games-easymode_144x144-307b8f657d987516abff44220313daae.webp"/><div><div class="css-1xpp3bj e10k0zts0">Easy Mode</div><p>Get an easy version of one of the hardest crossword puzzles of the week.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div><div class="css-1qt2b4r">Games<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li><li class="css-1qtaxzf" data-navid="Cooking" data-testid="nav-item-Cooking"><a class="css-u90q6n" data-navid="Cooking" href="https://cooking.nytimes.com/">Cooking</a><button aria-label="open Cooking submenu" class="css-1bp1dzo" data-navid="Cooking" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Cooking submenu" class="css-ljayxv" data-testid="nav-dropdown-Cooking"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="Cooking-brand-column"><a href="https://cooking.nytimes.com/"><h3>Cooking</h3><p>Recipes, advice and inspiration for everyday cooking, special occasions and more.</p></a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Cooking-links-column-header">Recipes</h3><ul aria-labelledby="Cooking-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/easy-recipes">Easy</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/dinner-recipes">Dinner</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/43843372-quick-recipes">Quick</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/healthy-recipes">Healthy</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/breakfast">Breakfast</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/vegetarian">Vegetarian</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/vegan-recipes">Vegan</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/our-best-chicken-recipes">Chicken</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/our-best-pasta-recipes">Pasta</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/desserts">Dessert</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Cooking-links-column-header">Editors' Picks</h3><ul aria-labelledby="Cooking-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/1334836-easy-salmon-recipes">Easy Salmon Recipes</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/638891-best-soup-stew-recipes">Soups and Stews</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/easy-weeknight">Easy Weeknight</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/32998034-our-newest-recipes">Newest Recipes</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/41238156-cheap-and-easy-dinner-ideas">Cheap and Easy Dinner Ideas</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/950138-amazing-slow-cooker-recipes">Slow Cooker Recipes</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/16596-healthy-breakfast-ideas">Healthy Breakfast Ideas</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/22792-best-tofu-recipes">Best Tofu Recipes</a></li></ul></div></div><div class="css-132p2yg"><div id="Cooking-thumbnail-column"><h3 class="css-1nudrh3" id="Cooking-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Cooking-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/cooking"><img alt="The Cooking Newsletter Logo" class="" src="/vi-assets/static-assets/icon-cooking_144x144-5a8be1ef711d4ba5e66b0be7a2ca8bfe.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Cooking Newsletter</div><p>New recipes, easy dinner ideas and smart kitchen tips from Melissa Clark, Sam Sifton and our New York Times Cooking editors.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/the-veggie"><img alt="The Veggie Logo" class="" src="/vi-assets/static-assets/icon-the-veggie_144x144-f99606e1ca100f88cdfd8d763bf442c5.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Veggie</div><p>Delicious vegetarian recipes and tips from Tanya Sichynsky.</p></div></a></li></ul></div></div><div class="css-132p2yg"><div id="Cooking-thumbnail-column"><h3 class="css-1nudrh3" id="Cooking-thumbnail-column-header"></h3><ul aria-labelledby="Cooking-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/five-weeknight-dishes"><img alt="Five Weeknight Dishes Logo" class="" src="/vi-assets/static-assets/icon-five-weeknight-dishes_144x144-97d51c5d4ba98233667b4057e3d852ab.webp"/><div><div class="css-1xpp3bj e10k0zts0">Five Weeknight Dishes</div><p>Dinner ideas for busy people from Emily Weinstein.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div><div class="css-1qt2b4r">Cooking<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li><li class="css-1qtaxzf" data-navid="Wirecutter" data-testid="nav-item-Wirecutter"><a class="css-u90q6n" data-navid="Wirecutter" href="https://www.nytimes.com/wirecutter/">Wirecutter</a><button aria-label="open Wirecutter submenu" class="css-1bp1dzo" data-navid="Wirecutter" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Wirecutter submenu" class="css-ljayxv" data-testid="nav-dropdown-Wirecutter"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="Wirecutter-brand-column"><a href="https://www.nytimes.com/wirecutter"><h3>Wirecutter</h3><p>Reviews and recommendations for thousands of products.</p></a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Wirecutter-links-column-header">Reviews</h3><ul aria-labelledby="Wirecutter-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/kitchen-dining/">Kitchen</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/electronics/">Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/sleep/">Sleep</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/appliances/">Appliances</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/home-garden/">Home and Garden</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/make-a-plan/moving/">Moving</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Wirecutter-links-column-header"></h3><ul aria-labelledby="Wirecutter-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/travel/">Travel</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/gifts/">Gifts</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/deals/">Deals</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/baby-kid/">Baby and Kid</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/health-fitness/">Health and Fitness</a></li></ul></div></div><div class="css-132p2yg"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Wirecutter-links-column-header">The Best...</h3><ul aria-labelledby="Wirecutter-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-air-purifier/">Air Purifier</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-electric-toothbrush/">Electric Toothbrush</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-pressure-washer/">Pressure Washer</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-cordless-stick-vacuum/">Cordless Stick Vacuum</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-office-chair/">Office Chair</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-robot-vacuum/">Robot Vacuum</a></li></ul></div></div><div class="css-132p2yg"><div id="Wirecutter-thumbnail-column"><h3 class="css-1nudrh3" id="Wirecutter-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Wirecutter-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/the-recommendation"><img alt="The Recommendation Logo" class="" src="/vi-assets/static-assets/icon-the-recommendation_144x144-3e66bd6cc82013bd511c31a8f04d4ff7.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Recommendation</div><p>The best independent reviews, expert advice and intensively researched deals.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/clean-everything"><img alt="Clean Everything Logo" class="" src="/vi-assets/static-assets/icon-clean-everything_144x144-97312e349d7284039a2153cb541b7fda.webp"/><div><div class="css-1xpp3bj e10k0zts0">Clean Everything</div><p>Step-by-step advice on how to keep everything in your home squeaky clean.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div><div class="css-1qt2b4r">Wirecutter<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li><li class="css-1qtaxzf" data-navid="The Athletic" data-testid="nav-item-The Athletic"><a class="css-u90q6n" data-navid="The Athletic" href="https://www.nytimes.com/athletic/">The Athletic</a><button aria-label="open The Athletic submenu" class="css-1bp1dzo" data-navid="The Athletic" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="The Athletic submenu" class="css-ljayxv" data-testid="nav-dropdown-The Athletic"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="The Athletic-brand-column"><a href="https://www.nytimes.com/athletic/"><h3>The Athletic</h3><p>Personalized coverage of your sports teams and leagues.</p></a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="The Athletic-links-column-header">Leagues</h3><ul aria-labelledby="The Athletic-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/nfl/">NFL</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/nba/">NBA</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/nhl/">NHL</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/football/premier-league/">Premier League</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/mlb/">MLB</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/college-football/">College Football</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/college-basketball/">NCAAM</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/womens-college-basketball/">NCAAW</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/tennis/">Tennis</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/formula-1/">F1</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/sports-betting/">Fantasy & Betting</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/wnba/">WNBA</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="The Athletic-links-column-header">Top Stories</h3><ul aria-labelledby="The Athletic-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/tag/bracketcentral/">March Madness</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/nfl/draft/">NFL Draft</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/tag/transfer-news/">Soccer Transfers</a></li></ul></div></div><div class="css-132p2yg"><div id="The Athletic-thumbnail-column"><h3 class="css-1nudrh3" id="The Athletic-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="The Athletic-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/athletic/newsletters/the-pulse/"><img alt="The Pulse Logo" class="" src="/vi-assets/static-assets/icon-athletic-pulse_144x144-393cbda91e2678278456723b62a9b21f.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Pulse</div><p>Delivering the top stories in sports, Sunday to Friday.</p></div></a></li><li><a class="css-hcfrni" href="https://www.nytimes.com/athletic/newsletters/scoop-city/"><img alt="Scoop City Logo" class="" src="/vi-assets/static-assets/icon_scoop_city_144x144-d0fc86b7506a3e8c0558a4e72a29a963.webp"/><div><div class="css-1xpp3bj e10k0zts0">Scoop City</div><p>The top stories in the NFL, from Jacob Robinson with Dianna Russini.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/athletic/5803046/2024/09/30/the-athletic-newsletters-sign-up/">See all newsletters</a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs" id="The Athletic-links-icons-column"><h3 class="css-1nudrh3" id="The Athletic-links-icons-column-header">Play</h3><ul aria-labelledby="The Athletic-links-icons-column-header"><li><a class="css-ed0o7b" href="https://www.nytimes.com/athletic/connections-sports-edition" style="white-space:pre-wrap"><i class="css-1n92pmf" style="background-image:url(/vi-assets/static-assets/icon_connections-d36a17a7babda7caf1b04cd9df95ba0d.svg)"></i><p><span>Connections:
Sports Edition</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/athletic/tag/connections-sports-edition-coach/" style="white-space:pre-wrap"><i class="css-1n92pmf"></i><p><span>Connections Coach</span> </p></a></li></ul></div></div></div><div class="css-1qt2b4r">The Athletic<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li></ul></nav></div></div><div class="css-oi0jtw" data-testid="masthead-nested-nav"><nav aria-label="Main" data-testid="desktop-nested-nav"><ul class="css-17j7fe1"><li class="css-1qtaxzf" data-navid="U.S." data-testid="nav-item-U.S."><a class="css-u90q6n" data-navid="U.S." href="https://www.nytimes.com/section/us">U.S.</a><button aria-label="open U.S. submenu" class="css-1bp1dzo" data-navid="U.S." type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="U.S. submenu" class="css-1qalmak" data-testid="nav-dropdown-U.S."><div class="css-13qj3r5"><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="U.S.-links-column-header">Sections</h3><ul aria-labelledby="U.S.-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/us">U.S.</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/politics">Politics</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/nyregion">New York</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/california-news">California</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/education">Education</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/health">Health</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/obituaries">Obituaries</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/science">Science</a></li></ul></div></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="U.S.-links-column-header"></h3><ul aria-labelledby="U.S.-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/climate">Climate</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/weather">Weather</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/sports">Sports</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business">Business</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/technology">Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/upshot">The Upshot</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/magazine">The Magazine</a></li></ul></div></div><div class="css-132p2yg"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="U.S.-links-column-header">Top Stories</h3><ul aria-labelledby="U.S.-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/trump-transition">Trump Transition</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/topic/organization/us-supreme-court">Supreme Court</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/topic/organization/us-congress">Congress</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/immigration-us">Immigration</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/abortion-news">Abortion</a></li></ul></div></div><div class="css-132p2yg"><div id="U.S.-thumbnail-column"><h3 class="css-1nudrh3" id="U.S.-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="U.S.-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/morning-briefing"><img alt="The Morning Logo" class="" src="/vi-assets/static-assets/icon-the-morning_144x144-b12a6923b6ad9102b766352261b1a847.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Morning</div><p>Make sense of the day’s news and ideas.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/upshot"><img alt="The Upshot Logo" class="" src="/vi-assets/static-assets/icon-the-upshot_144x144-0b1553ff703bbd07ac8fe73e6d215888.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Upshot</div><p>Analysis that explains politics, policy and everyday life.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div><div class="css-1didtc4"></div><div class="css-132p2yg"><div id="U.S.-thumbnail-column"><h3 class="css-1nudrh3" id="U.S.-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="U.S.-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/the-daily"><img alt="The Daily Logo" class="" src="https://static01.nyt.com/images/2017/01/29/podcasts/the-daily-album-art/the-daily-album-art-mediumSquare149-v3.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">The Daily</div><p>The biggest stories of our time, in 20 minutes a day.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/column/election-run-up-podcast"><img alt="The Run-Up Logo" class="css-hqhlyo" src="https://static01.nyt.com/images/2022/08/29/podcasts/the-run-up-album-art/the-run-up-album-art-thumbLarge.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">The Run-Up</div><p>On the campaign trail with Astead Herndon.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="World" data-testid="nav-item-World"><a class="css-u90q6n" data-navid="World" href="https://www.nytimes.com/section/world">World</a><button aria-label="open World submenu" class="css-1bp1dzo" data-navid="World" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="World submenu" class="css-1qalmak" data-testid="nav-dropdown-World"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="World-links-column-header">Sections</h3><ul aria-labelledby="World-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world">World</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/africa">Africa</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/americas">Americas</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/asia">Asia</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/australia">Australia</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/canada">Canada</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/europe">Europe</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/world/middleeast">Middle East</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/science">Science</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/climate">Climate</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/weather">Weather</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/health">Health</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/obituaries">Obituaries</a></li></ul></div></div><div class="css-167b3qw"></div><div class="css-r6g2pn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="World-links-column-header">Top Stories</h3><ul aria-labelledby="World-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/israel-hamas-gaza">Middle East Crisis</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/ukraine-russia">Russia-Ukraine War</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/china-relations">China International Relations</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-global-profile">The Global Profile</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/es/">Leer en Español</a></li></ul></div></div><div class="css-132p2yg"><div id="World-thumbnail-column"><h3 class="css-1nudrh3" id="World-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="World-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/morning-briefing-europe"><img alt="Morning Briefing: Europe Logo" class="" src="/vi-assets/static-assets/icon-europe-morning-briefing_144x144-f0a330cb12ba0c31f81f13e25f6d0d18.webp"/><div><div class="css-1xpp3bj e10k0zts0">Morning Briefing: Europe</div><p>Get what you need to know to start your day.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/the-interpreter"><img alt="The Interpreter Logo" class="" src="/vi-assets/static-assets/icon-the-interpreter_144x144-b29b74b2ebedb8e74823f33b16fb8167.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Interpreter</div><p>Original analysis on the week’s biggest global stories.</p></div></a></li></ul></div></div><div class="css-132p2yg"><div id="World-thumbnail-column"><h3 class="css-1nudrh3" id="World-thumbnail-column-header"></h3><ul aria-labelledby="World-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/your-places-global-update"><img alt="Your Places: Global Update Logo" class="" src="/vi-assets/static-assets/icon-yourplaces-globalupdate_144x144-c25aba1c2904f301a08ad33183f723c6.webp"/><div><div class="css-1xpp3bj e10k0zts0">Your Places: Global Update</div><p>The latest news for any part of the world you select.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/canada-letter"><img alt="Canada Letter Logo" class="" src="/vi-assets/static-assets/icon-canada-letter_144x144-65d899377edbcce9773d31fd03a77e8d.webp"/><div><div class="css-1xpp3bj e10k0zts0">Canada Letter</div><p>Backstories and analysis from our Canadian correspondents.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Business" data-testid="nav-item-Business"><a class="css-u90q6n" data-navid="Business" href="https://www.nytimes.com/section/business">Business</a><button aria-label="open Business submenu" class="css-1bp1dzo" data-navid="Business" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Business submenu" class="css-1qalmak" data-testid="nav-dropdown-Business"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Business-links-column-header">Sections</h3><ul aria-labelledby="Business-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business">Business</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/technology">Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business/economy">Economy</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business/media">Media</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/markets-overview">Finance and Markets</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Business-links-column-header"></h3><ul aria-labelledby="Business-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business/dealbook">DealBook</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/technology/personaltech">Personal Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/business/energy-environment">Energy Transition</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/your-money">Your Money</a></li></ul></div></div><div class="css-r6g2pn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Business-links-column-header">Top Stories</h3><ul aria-labelledby="Business-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/news-event/economy-business-us">U.S. Economy</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/markets-overview">Stock Market</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/artificial-intelligence">Artificial Intelligence</a></li></ul></div></div><div class="css-132p2yg"><div id="Business-thumbnail-column"><h3 class="css-1nudrh3" id="Business-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Business-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/dealbook"><img alt="DealBook Logo" class="" src="/vi-assets/static-assets/icon-dealbook_144x144-28e8f71aafff426804c3a92b1b176e07.webp"/><div><div class="css-1xpp3bj e10k0zts0">DealBook</div><p>The most crucial business and policy news you need to know.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div><div class="css-132p2yg"><div id="Business-thumbnail-column"><h3 class="css-1nudrh3" id="Business-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="Business-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/hard-fork"><img alt="Hard Fork Logo" class="" src="https://static01.nyt.com/images/2022/09/28/podcasts/hard-fork-album-art/hard-fork-album-art-mediumSquare149-v2.png?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Hard Fork</div><p>Our tech journalists help you make sense of the rapidly changing tech world.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Arts" data-testid="nav-item-Arts"><a class="css-u90q6n" data-navid="Arts" href="https://www.nytimes.com/section/arts">Arts</a><button aria-label="open Arts submenu" class="css-1bp1dzo" data-navid="Arts" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Arts submenu" class="css-1qalmak" data-testid="nav-dropdown-Arts"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Arts-links-column-header">Sections</h3><ul aria-labelledby="Arts-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts">Today's Arts</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/books">Book Review</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/books/best-sellers">Best Sellers</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts/dance">Dance</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/movies">Movies</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts/music">Music</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Arts-links-column-header"></h3><ul aria-labelledby="Arts-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts/television">Television</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/theater">Theater</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/pop-culture">Pop Culture</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/t-magazine">T Magazine</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/arts/design">Visual Arts</a></li></ul></div></div><div class="css-r6g2pn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Arts-links-column-header">Recommendations</h3><ul aria-labelledby="Arts-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/interactive/2024/books/best-books-21st-century.html">100 Best Books of the 21st Century</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/critics-picks">Critic’s Picks</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/books-to-read">What to Read</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/what-to-watch">What to Watch</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/playlist">What to Listen To</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/interactive/2023/10/27/arts/music/music-fivemins-collection.html">5 Minutes to Make You Love Music</a></li></ul></div></div><div class="css-132p2yg"><div id="Arts-thumbnail-column"><h3 class="css-1nudrh3" id="Arts-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Arts-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/read-like-the-wind"><img alt="Read Like the Wind Logo" class="" src="/vi-assets/static-assets/icon-read-like-the-wind_144x144-5bcf9faf41d0b49df1df29e59a868b36.webp"/><div><div class="css-1xpp3bj e10k0zts0">Read Like the Wind</div><p>Book recommendations from our critics.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/watching"><img alt="Watching Logo" class="" src="/vi-assets/static-assets/icon-watching_144x144-631a1da177f9fda1a7f4614ad8e607bd.webp"/><div><div class="css-1xpp3bj e10k0zts0">Watching</div><p>Streaming TV and movie recommendations.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div><div class="css-132p2yg"><div id="Arts-thumbnail-column"><h3 class="css-1nudrh3" id="Arts-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="Arts-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/book-review-podcast"><img alt="Book Review Logo" class="" src="https://static01.nyt.com/images/2018/03/27/books/book-review-album-art-v2/book-review-album-art-v2-thumbLarge-v3.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Book Review</div><p>The podcast that takes you inside the literary world.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/column/popcast-pop-music-podcast"><img alt="Popcast Logo" class="" src="https://static01.nyt.com/images/2011/05/20/multimedia/music-popcast/music-popcast-thumbLarge-v3.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Popcast</div><p>Pop music news, new songs and albums, and artists of note.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Lifestyle" data-testid="nav-item-Lifestyle"><a class="css-u90q6n" data-navid="Lifestyle" href="https://www.nytimes.com/spotlight/lifestyle">Lifestyle</a><button aria-label="open Lifestyle submenu" class="css-1bp1dzo" data-navid="Lifestyle" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Lifestyle submenu" class="css-1qalmak" data-testid="nav-dropdown-Lifestyle"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Lifestyle-links-column-header">Sections</h3><ul aria-labelledby="Lifestyle-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/lifestyle">All Lifestyle</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/well">Well</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/travel">Travel</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/style">Style</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/realestate">Real Estate</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Lifestyle-links-column-header"></h3><ul aria-labelledby="Lifestyle-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/food">Food</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/fashion/weddings">Love</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/your-money">Your Money</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/technology/personaltech">Personal Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/t-magazine">T Magazine</a></li></ul></div></div><div class="css-r6g2pn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Lifestyle-links-column-header">Columns</h3><ul aria-labelledby="Lifestyle-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/36-hours">36 Hours</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/ask-well">Ask Well</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-hunt">The Hunt</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/modern-love">Modern Love</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/best-restaurants">Where to Eat</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/vows">Vows</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/social-qs">Social Q’s</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-ethicist">The Ethicist</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/ask-the-therapist">Ask the Therapist</a></li></ul></div></div><div class="css-132p2yg"><div id="Lifestyle-thumbnail-column"><h3 class="css-1nudrh3" id="Lifestyle-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Lifestyle-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/open-thread-fashion"><img alt="Open Thread Logo" class="" src="/vi-assets/static-assets/icon-open-thread-fashion_144x144-8e1b4b3fd68c2f333faa63097da2249b.webp"/><div><div class="css-1xpp3bj e10k0zts0">Open Thread</div><p>The latest news on what we wear, by our chief fashion critic.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/well"><img alt="Well Logo" class="" src="/vi-assets/static-assets/icon-well_144x144-433c9d15dc985dded9b705942592c6fb.webp"/><div><div class="css-1xpp3bj e10k0zts0">Well</div><p>Essential news and guidance to live your healthiest life.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div><div class="css-132p2yg"><div id="Lifestyle-thumbnail-column"><h3 class="css-1nudrh3" id="Lifestyle-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="Lifestyle-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/modern-love-podcast"><img alt="Modern Love Logo" class="" src="https://static01.nyt.com/images/2020/09/21/podcasts/modernlove-logo/modernlove-logo-thumbLarge-v3.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Modern Love</div><p>The complicated love lives of real people.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Opinion" data-testid="nav-item-Opinion"><a class="css-u90q6n" data-navid="Opinion" href="https://www.nytimes.com/section/opinion">Opinion</a><button aria-label="open Opinion submenu" class="css-1bp1dzo" data-navid="Opinion" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Opinion submenu" class="css-1qalmak" data-testid="nav-dropdown-Opinion"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Opinion-links-column-header">Sections</h3><ul aria-labelledby="Opinion-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion">Opinion</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/contributors">Guest Essays</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/editorials">Editorials</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/op-docs">Op-Docs</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/opinion-video">Videos</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/letters">Letters</a></li></ul></div></div><div class="css-r7t1h4"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Opinion-links-column-header">Topics</h3><ul aria-labelledby="Opinion-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/politics">Politics</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/international-world">World</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/business-economics">Business</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/technology">Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/environment">Climate</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/health">Health</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/section/opinion/culture">Culture</a></li></ul></div></div><div class="css-167b3qw"></div><div class="css-9jpfr0"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Opinion-links-column-header">Columnists</h3><ul aria-labelledby="Opinion-links-column-header" class="css-34zueq"><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/jamelle-bouie">Jamelle Bouie</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/david-brooks">David Brooks</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/gail-collins">Gail Collins</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/ross-douthat">Ross Douthat</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/maureen-dowd">Maureen Dowd</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/david-french">David French</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/thomas-l-friedman">Thomas L. Friedman</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/m-gessen">M. Gessen</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/michelle-goldberg">Michelle Goldberg</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/ezra-klein">Ezra Klein</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/nicholas-kristof">Nicholas Kristof</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/carlos-lozada">Carlos Lozada</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/tressie-mcmillan-cottom">Tressie McMillan Cottom</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/pamela-paul">Pamela Paul</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/lydia-polgreen">Lydia Polgreen</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/bret-stephens">Bret Stephens</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/by/zeynep-tufekci">Zeynep Tufekci</a></li></ul></div></div><div class="css-132p2yg"><div id="Opinion-thumbnail-column"><h3 class="css-1nudrh3" id="Opinion-thumbnail-column-header">Podcasts</h3><ul aria-labelledby="Opinion-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/matter-of-opinion"><img alt="Matter of Opinion Logo" class="" src="https://static01.nyt.com/images/2023/05/08/podcasts/matter-of-opinion-album-art/matter-of-opinion-album-art-thumbLarge-v2.jpg?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">Matter of Opinion</div><p>Thoughts, aloud. With Michelle Cottle, Ross Douthat, Carlos Lozada and Lydia Polgreen.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/column/ezra-klein-podcast"><img alt="The Ezra Klein Show Logo" class="" src="https://static01.nyt.com/images/2023/04/05/podcasts/ezra-klein-album-art/ezra-klein-album-art-thumbLarge-v3.png"/><div><div class="css-1xpp3bj e10k0zts0">The Ezra Klein Show</div><p>Discussions of ideas that matter, plus book recommendations.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/spotlight/podcasts">See all podcasts</a></div></div></div></div></li><li class="css-1qtaxzf" data-navid="Audio" data-testid="nav-item-Audio"><a class="css-u90q6n" data-navid="Audio" href="https://www.nytimes.com/spotlight/podcasts">Audio</a><button aria-label="open Audio submenu" class="css-1bp1dzo" data-navid="Audio" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Audio submenu" class="css-1qalmak" data-testid="nav-dropdown-Audio"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="Audio-brand-column"><a href="https://www.nytimes.com/spotlight/podcasts"><h3>Audio</h3><p>Podcasts and narrated articles covering news, tech, culture and more.</p></a><a class="css-1q7rq5u" href="https://www.nytimes.com/audio/app">Download the Audio app on iOS.</a></div></div><div class="css-15iksc8"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Audio-links-column-header">Listen</h3><ul aria-labelledby="Audio-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-headlines">The Headlines</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-daily">The Daily</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/hard-fork">Hard Fork</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/ezra-klein-podcast">The Ezra Klein Show</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/matter-of-opinion">Matter of Opinion</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/interactive/2022/podcasts/serial-productions.html">Serial Productions</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/book-review-podcast">The Book Review Podcast</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/modern-love-podcast">Modern Love</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/election-run-up-podcast">The Run-Up</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/popcast-pop-music-podcast">Popcast</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/reporter-reads">Reporter Reads</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/the-sunday-read">The Sunday Read</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-culture-desk">The Culture Desk</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/column/the-interview">The Interview</a></li></ul><a class="css-c38y3b" href="https://www.nytimes.com/spotlight/podcasts">See all audio</a></div></div><div class="css-132p2yg"><div id="Audio-thumbnail-column"><h3 class="css-1nudrh3" id="Audio-thumbnail-column-header">Featured</h3><ul aria-labelledby="Audio-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/column/the-interview"><img alt="The Interview Logo" class="" src="/vi-assets/static-assets/NYT-TheInterview-0232c6c95d42d77941fd3d8e5d2776cb.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Interview</div><p>Conversations with the world’s most fascinating people.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/column/the-headlines"><img alt="The Headlines Logo" class="" src="https://static01.nyt.com/images/2022/10/12/podcasts/headlines-albumartwork-audioapp-2/headlines-albumartwork-audioapp-2-thumbLarge.png?quality=75&auto=webp&disable=upscale"/><div><div class="css-1xpp3bj e10k0zts0">The Headlines</div><p>Your morning listen. Top stories, in 10 minutes.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/interactive/2024/podcasts/serial-good-whale.html"><img alt="Serial: The Good Whale Logo" class="" src="/vi-assets/static-assets/TheGoodWhale_144x144-f0eb13463973a1da8814328998990640.webp"/><div><div class="css-1xpp3bj e10k0zts0">Serial: The Good Whale</div><p>A Hollywood orca's real-life odyssey back to the ocean.</p></div></a></li></ul></div></div><div class="css-132p2yg"><div id="Audio-thumbnail-column"><h3 class="css-1nudrh3" id="Audio-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Audio-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/audio"><img alt="Audio Logo" class="" src="/vi-assets/static-assets/icon-audio_144x144-dc00c6581be29065cbd19ec7a83a3767.webp"/><div><div class="css-1xpp3bj e10k0zts0">Audio</div><p>Our editors share their favorite listens from the New York Times Audio app.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div><div class="css-1qt2b4r">Audio<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li><li class="css-1qtaxzf" data-navid="Games" data-testid="nav-item-Games"><a class="css-u90q6n" data-navid="Games" href="https://www.nytimes.com/crosswords">Games</a><button aria-label="open Games submenu" class="css-1bp1dzo" data-navid="Games" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Games submenu" class="css-1qalmak" data-testid="nav-dropdown-Games"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="Games-brand-column"><a href="https://www.nytimes.com/crosswords"><h3>Games</h3><p>Word games, logic puzzles and crosswords, including an extensive archive.</p></a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs" id="Games-links-icons-column"><h3 class="css-1nudrh3" id="Games-links-icons-column-header">Play</h3><ul aria-labelledby="Games-links-icons-column-header"><li><a class="css-ed0o7b" href="https://www.nytimes.com/puzzles/spelling-bee"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/spelling-bee.svg)"></i><p><span>Spelling Bee</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/crosswords/game/mini"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/mini.svg)"></i><p><span>The Mini Crossword</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/games/wordle/index.html"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/wordle.svg)"></i><p><span>Wordle</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/crosswords/game/daily/"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/daily.svg)"></i><p><span>The Crossword</span> </p></a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs" id="Games-links-icons-column"><h3 class="css-1nudrh3" id="Games-links-icons-column-header"></h3><ul aria-labelledby="Games-links-icons-column-header"><li><a class="css-ed0o7b" href="https://www.nytimes.com/games/strands"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/strands.svg)"></i><p><span>Strands</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/games/connections"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/connections.svg)"></i><p><span>Connections</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/puzzles/sudoku"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/expansion-games/sudoku-card-icon.svg)"></i><p><span>Sudoku</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/puzzles/letter-boxed"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/letter-boxed.svg)"></i><p><span>Letter Boxed</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/puzzles/tiles"><i class="css-1n92pmf" style="background-image:url(https://www.nytimes.com/games-assets/v2/assets/icons/tiles.svg)"></i><p><span>Tiles</span> </p></a></li></ul></div></div><div class="css-132p2yg"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Games-links-column-header">Community</h3><ul aria-labelledby="Games-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/spelling-bee-forum">Spelling Bee Forum</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/daily-crossword-column">Wordplay Column</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/wordle-review">Wordle Review</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/article/submit-crossword-puzzles-the-new-york-times.html">Submit a Crossword</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/spotlight/puzzle-making">Meet Our Crossword Constructors</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/2022/09/19/crosswords/mini-to-maestro-part-1.html">Mini to Maestro</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/interactive/2022/upshot/wordle-bot.html">Wordlebot</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/puzzle-personality">Take the Puzzle Personality Quiz</a></li></ul></div></div><div class="css-132p2yg"><div id="Games-thumbnail-column"><h3 class="css-1nudrh3" id="Games-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Games-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/gameplay"><img alt="Gameplay Logo" class="" src="/vi-assets/static-assets/icon-gameplay_144x144-b6cc5e2a7cc27a43096274a02921329c.webp"/><div><div class="css-1xpp3bj e10k0zts0">Gameplay</div><p>Puzzles, brain teasers, solving tips and more.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/easy-mode"><img alt="Easy Mode Logo" class="" src="/vi-assets/static-assets/icon-games-easymode_144x144-307b8f657d987516abff44220313daae.webp"/><div><div class="css-1xpp3bj e10k0zts0">Easy Mode</div><p>Get an easy version of one of the hardest crossword puzzles of the week.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div><div class="css-1qt2b4r">Games<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li><li class="css-1qtaxzf" data-navid="Cooking" data-testid="nav-item-Cooking"><a class="css-u90q6n" data-navid="Cooking" href="https://cooking.nytimes.com/">Cooking</a><button aria-label="open Cooking submenu" class="css-1bp1dzo" data-navid="Cooking" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Cooking submenu" class="css-1qalmak" data-testid="nav-dropdown-Cooking"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="Cooking-brand-column"><a href="https://cooking.nytimes.com/"><h3>Cooking</h3><p>Recipes, advice and inspiration for everyday cooking, special occasions and more.</p></a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Cooking-links-column-header">Recipes</h3><ul aria-labelledby="Cooking-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/easy-recipes">Easy</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/dinner-recipes">Dinner</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/43843372-quick-recipes">Quick</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/healthy-recipes">Healthy</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/breakfast">Breakfast</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/vegetarian">Vegetarian</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/vegan-recipes">Vegan</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/our-best-chicken-recipes">Chicken</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/our-best-pasta-recipes">Pasta</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/desserts">Dessert</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Cooking-links-column-header">Editors' Picks</h3><ul aria-labelledby="Cooking-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/1334836-easy-salmon-recipes">Easy Salmon Recipes</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/638891-best-soup-stew-recipes">Soups and Stews</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/topics/easy-weeknight">Easy Weeknight</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/32998034-our-newest-recipes">Newest Recipes</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/41238156-cheap-and-easy-dinner-ideas">Cheap and Easy Dinner Ideas</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/950138-amazing-slow-cooker-recipes">Slow Cooker Recipes</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/16596-healthy-breakfast-ideas">Healthy Breakfast Ideas</a></li><li><a class="css-kz1pwz" href="https://cooking.nytimes.com/68861692-nyt-cooking/22792-best-tofu-recipes">Best Tofu Recipes</a></li></ul></div></div><div class="css-132p2yg"><div id="Cooking-thumbnail-column"><h3 class="css-1nudrh3" id="Cooking-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Cooking-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/cooking"><img alt="The Cooking Newsletter Logo" class="" src="/vi-assets/static-assets/icon-cooking_144x144-5a8be1ef711d4ba5e66b0be7a2ca8bfe.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Cooking Newsletter</div><p>New recipes, easy dinner ideas and smart kitchen tips from Melissa Clark, Sam Sifton and our New York Times Cooking editors.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/the-veggie"><img alt="The Veggie Logo" class="" src="/vi-assets/static-assets/icon-the-veggie_144x144-f99606e1ca100f88cdfd8d763bf442c5.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Veggie</div><p>Delicious vegetarian recipes and tips from Tanya Sichynsky.</p></div></a></li></ul></div></div><div class="css-132p2yg"><div id="Cooking-thumbnail-column"><h3 class="css-1nudrh3" id="Cooking-thumbnail-column-header"></h3><ul aria-labelledby="Cooking-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/five-weeknight-dishes"><img alt="Five Weeknight Dishes Logo" class="" src="/vi-assets/static-assets/icon-five-weeknight-dishes_144x144-97d51c5d4ba98233667b4057e3d852ab.webp"/><div><div class="css-1xpp3bj e10k0zts0">Five Weeknight Dishes</div><p>Dinner ideas for busy people from Emily Weinstein.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div><div class="css-1qt2b4r">Cooking<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li><li class="css-1qtaxzf" data-navid="Wirecutter" data-testid="nav-item-Wirecutter"><a class="css-u90q6n" data-navid="Wirecutter" href="https://www.nytimes.com/wirecutter/">Wirecutter</a><button aria-label="open Wirecutter submenu" class="css-1bp1dzo" data-navid="Wirecutter" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="Wirecutter submenu" class="css-1qalmak" data-testid="nav-dropdown-Wirecutter"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="Wirecutter-brand-column"><a href="https://www.nytimes.com/wirecutter"><h3>Wirecutter</h3><p>Reviews and recommendations for thousands of products.</p></a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Wirecutter-links-column-header">Reviews</h3><ul aria-labelledby="Wirecutter-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/kitchen-dining/">Kitchen</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/electronics/">Tech</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/sleep/">Sleep</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/appliances/">Appliances</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/home-garden/">Home and Garden</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/make-a-plan/moving/">Moving</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Wirecutter-links-column-header"></h3><ul aria-labelledby="Wirecutter-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/travel/">Travel</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/gifts/">Gifts</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/deals/">Deals</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/baby-kid/">Baby and Kid</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/health-fitness/">Health and Fitness</a></li></ul></div></div><div class="css-132p2yg"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="Wirecutter-links-column-header">The Best...</h3><ul aria-labelledby="Wirecutter-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-air-purifier/">Air Purifier</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-electric-toothbrush/">Electric Toothbrush</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-pressure-washer/">Pressure Washer</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-cordless-stick-vacuum/">Cordless Stick Vacuum</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-office-chair/">Office Chair</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/wirecutter/reviews/best-robot-vacuum/">Robot Vacuum</a></li></ul></div></div><div class="css-132p2yg"><div id="Wirecutter-thumbnail-column"><h3 class="css-1nudrh3" id="Wirecutter-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="Wirecutter-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/the-recommendation"><img alt="The Recommendation Logo" class="" src="/vi-assets/static-assets/icon-the-recommendation_144x144-3e66bd6cc82013bd511c31a8f04d4ff7.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Recommendation</div><p>The best independent reviews, expert advice and intensively researched deals.</p></div></a></li><li><a class="css-11kdrny" href="https://www.nytimes.com/newsletters/clean-everything"><img alt="Clean Everything Logo" class="" src="/vi-assets/static-assets/icon-clean-everything_144x144-97312e349d7284039a2153cb541b7fda.webp"/><div><div class="css-1xpp3bj e10k0zts0">Clean Everything</div><p>Step-by-step advice on how to keep everything in your home squeaky clean.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/newsletters">See all newsletters</a></div></div></div><div class="css-1qt2b4r">Wirecutter<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li><li class="css-1qtaxzf" data-navid="The Athletic" data-testid="nav-item-The Athletic"><a class="css-u90q6n" data-navid="The Athletic" href="https://www.nytimes.com/athletic/">The Athletic</a><button aria-label="open The Athletic submenu" class="css-1bp1dzo" data-navid="The Athletic" type="button"><svg class="css-1gq8co7" viewbox="0 0 13 8"><polygon fill="#979797" points="6.5,8 0,1.4 1.4,0 6.5,5.2 11.6,0 13,1.4"></polygon></svg></button><div aria-hidden="true" aria-label="The Athletic submenu" class="css-1qalmak" data-testid="nav-dropdown-The Athletic"><div class="css-13qj3r5"><div class="css-167b3qw"></div><div class="css-132p2yg"><div class="css-7s6arl" id="The Athletic-brand-column"><a href="https://www.nytimes.com/athletic/"><h3>The Athletic</h3><p>Personalized coverage of your sports teams and leagues.</p></a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="The Athletic-links-column-header">Leagues</h3><ul aria-labelledby="The Athletic-links-column-header" class="css-1qlpcok"><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/nfl/">NFL</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/nba/">NBA</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/nhl/">NHL</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/football/premier-league/">Premier League</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/mlb/">MLB</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/college-football/">College Football</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/college-basketball/">NCAAM</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/womens-college-basketball/">NCAAW</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/tennis/">Tennis</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/formula-1/">F1</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/sports-betting/">Fantasy & Betting</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/wnba/">WNBA</a></li></ul></div></div><div class="css-dd9fkn"><div class="css-j7qwjs"><h3 class="css-1nudrh3" id="The Athletic-links-column-header">Top Stories</h3><ul aria-labelledby="The Athletic-links-column-header" class="css-j7qwjs"><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/tag/bracketcentral/">March Madness</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/nfl/draft/">NFL Draft</a></li><li><a class="css-kz1pwz" href="https://www.nytimes.com/athletic/tag/transfer-news/">Soccer Transfers</a></li></ul></div></div><div class="css-132p2yg"><div id="The Athletic-thumbnail-column"><h3 class="css-1nudrh3" id="The Athletic-thumbnail-column-header">Newsletters</h3><ul aria-labelledby="The Athletic-thumbnail-column-header"><li><a class="css-11kdrny" href="https://www.nytimes.com/athletic/newsletters/the-pulse/"><img alt="The Pulse Logo" class="" src="/vi-assets/static-assets/icon-athletic-pulse_144x144-393cbda91e2678278456723b62a9b21f.webp"/><div><div class="css-1xpp3bj e10k0zts0">The Pulse</div><p>Delivering the top stories in sports, Sunday to Friday.</p></div></a></li><li><a class="css-hcfrni" href="https://www.nytimes.com/athletic/newsletters/scoop-city/"><img alt="Scoop City Logo" class="" src="/vi-assets/static-assets/icon_scoop_city_144x144-d0fc86b7506a3e8c0558a4e72a29a963.webp"/><div><div class="css-1xpp3bj e10k0zts0">Scoop City</div><p>The top stories in the NFL, from Jacob Robinson with Dianna Russini.</p></div></a></li></ul><a class="css-b3grxh" href="https://www.nytimes.com/athletic/5803046/2024/09/30/the-athletic-newsletters-sign-up/">See all newsletters</a></div></div><div class="css-dd9fkn"><div class="css-j7qwjs" id="The Athletic-links-icons-column"><h3 class="css-1nudrh3" id="The Athletic-links-icons-column-header">Play</h3><ul aria-labelledby="The Athletic-links-icons-column-header"><li><a class="css-ed0o7b" href="https://www.nytimes.com/athletic/connections-sports-edition" style="white-space:pre-wrap"><i class="css-1n92pmf" style="background-image:url(/vi-assets/static-assets/icon_connections-d36a17a7babda7caf1b04cd9df95ba0d.svg)"></i><p><span>Connections:
Sports Edition</span> </p></a></li><li><a class="css-ed0o7b" href="https://www.nytimes.com/athletic/tag/connections-sports-edition-coach/" style="white-space:pre-wrap"><i class="css-1n92pmf"></i><p><span>Connections Coach</span> </p></a></li></ul></div></div></div><div class="css-1qt2b4r">The Athletic<!-- --> is included in an All Access subscription.<!-- --> <a href="https://www.nytimes.com/subscription/all-access">Learn more.</a></div></div></li></ul></nav></div></header></div></div><div><main id="site-content"><div class="css-pqd7q9 eu3y8st0"><div class="smartphone tablet desktop"><h1 class="css-1dv1kvn">New York Times - Top Stories</h1><div data-hierarchy="feed" data-testid="programming-node"><div class="css-hmsvwu e1ppw5w20"><div class="css-m5ahyg"><div class="css-1l10c03 e17qa79g0" span="14"><div><div data-hierarchy="zone" data-testid="programming-node"><div class="css-1w1paqe e1ppw5w20"><div class="css-1u1zmuv e1ppw5w20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/95773dee-1b8a-5f08-b78a-29ed1903e90c" href="https://www.nytimes.com/2025/02/28/us/politics/musk-federal-bureaucracy-takeover.html"><div class="css-xdandi"><p class="indicate-hover css-1gg6cw2">How Elon Musk Executed His Takeover of the Federal Bureaucracy</p></div><p class="summary-class css-ofqxyv">The operation was driven with a frenetic focus by the billionaire, who channeled his resentment of regulatory oversight into a government overhaul.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">18 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/89c13edf-2ccb-5223-91c7-a96b2e7b0998" href="https://www.nytimes.com/2025/02/28/us/politics/musk-doge-timeline-takeaways.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">The Building of DOGE: A Timeline and Key Takeaways</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">2 min read</p></div></div></a></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><a aria-hidden="true" class="css-9mylee" data-uri="nyt://article/95773dee-1b8a-5f08-b78a-29ed1903e90c" href="https://www.nytimes.com/2025/02/28/us/politics/musk-federal-bureaucracy-takeover.html" tabindex="-1"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)" srcset="https://static01.nyt.com/images/2025/02/25/multimedia/dc-doge-origins-promo/dc-doge-origins-promo-threeByTwoSmallAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale 1x, https://static01.nyt.com/images/2025/02/25/multimedia/dc-doge-origins-promo/dc-doge-origins-promo-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale 2x"/><img alt="Mr. Musk now finds himself spearheading a radical takeover of the federal bureaucracy, empowered by President Trump in ways that have no historical parallel for an unelected adviser." class="css-hdqqnp" fetchpriority="high" src="https://static01.nyt.com/images/2025/02/25/multimedia/dc-doge-origins-promo/dc-doge-origins-promo-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/><noscript><img alt="Mr. Musk now finds himself spearheading a radical takeover of the federal bureaucracy, empowered by President Trump in ways that have no historical parallel for an unelected adviser." class="css-122y91a" src="https://static01.nyt.com/images/2025/02/25/multimedia/dc-doge-origins-promo/dc-doge-origins-promo-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Eric Lee/The New York Times</span></figcaption></a></figure><figure class="container-margin css-hurk9l"><div class="reporter-thread-carddeck"><div class="css-1lc519a"><div class="css-10avpiw" data-key="1873857.1960980943"><a class="css-1pwpj83" href="https://www.nytimes.com/2025/02/28/us/politics/musk-federal-bureaucracy-takeover.html"><div class="css-shshzh" data-testid="live-blog-byline"><span class="css-15zbqwq"><img alt="Kate Conger" height="40" loading="lazy" sizes="40px" src="https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbLarge-v5.jpg?quality=75&auto=webp" srcset="https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbStandard-v5.jpg?quality=75&auto=webp 75w, https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbLarge-v5.jpg?quality=75&auto=webp 150w" width="40"/></span><div class="css-1ppjtba"><div><p class="css-kzazds e1jsehar1"><span class="css-1baulvz last-byline" itemprop="name">Kate Conger</span></p></div></div></div><p class="css-1ja5hfe">It started as banter at a fund-raising dinner for Vivek Ramaswamy’s presidential campaign in 2023. Elon Musk indicated to guests that he believed that technology was the key to gutting the federal bureaucracy →</p></a></div><div class="css-10avpiw" data-key="6377542.584128635"><a class="css-1pwpj83" href="https://www.nytimes.com/2025/02/28/us/politics/musk-federal-bureaucracy-takeover.html"><div class="css-shshzh" data-testid="live-blog-byline"><span class="css-15zbqwq"><img alt="Nicholas Nehamas" height="40" loading="lazy" sizes="40px" src="https://static01.nyt.com/images/2023/04/21/reader-center/author-nicholas-nehamas/author-nicholas-nehamas-thumbLarge.png?quality=75&auto=webp" srcset="https://static01.nyt.com/images/2023/04/21/reader-center/author-nicholas-nehamas/author-nicholas-nehamas-thumbStandard.png?quality=75&auto=webp 75w, https://static01.nyt.com/images/2023/04/21/reader-center/author-nicholas-nehamas/author-nicholas-nehamas-thumbLarge.png?quality=75&auto=webp 150w" width="40"/></span><div class="css-1ppjtba"><div><p class="css-kzazds e1jsehar1"><span class="css-1baulvz last-byline" itemprop="name">Nicholas Nehamas</span></p></div></div></div><p class="css-1ja5hfe">Musk’s interest in reshaping the government grew after he endorsed Donald J. Trump, pouring millions into his campaign. After Trump won, seasoned conservative operatives helped educate Musk about the executive branch.</p></a></div><div class="css-10avpiw" data-key="1962504.0820107586"><a class="css-1pwpj83" href="https://www.nytimes.com/2025/02/28/us/politics/musk-federal-bureaucracy-takeover.html"><div class="css-shshzh" data-testid="live-blog-byline"><span class="css-15zbqwq"><img alt="Kate Conger" height="40" loading="lazy" sizes="40px" src="https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbLarge-v5.jpg?quality=75&auto=webp" srcset="https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbStandard-v5.jpg?quality=75&auto=webp 75w, https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbLarge-v5.jpg?quality=75&auto=webp 150w" width="40"/></span><div class="css-1ppjtba"><div><p class="css-kzazds e1jsehar1"><span class="css-1baulvz last-byline" itemprop="name">Kate Conger</span></p></div></div></div><p class="css-1ja5hfe">Trump said that the Department of Government Efficiency, or DOGE, would operate outside the government. But Musk’s team wanted to get inside the bureaucracy and decided to take over an existing White House digital services office.</p></a></div><div class="css-10avpiw" data-key="8598760.920657681"><a class="css-1pwpj83" href="https://www.nytimes.com/2025/02/28/us/politics/musk-federal-bureaucracy-takeover.html"><div class="css-shshzh" data-testid="live-blog-byline"><span class="css-15zbqwq"><img alt="Nicholas Nehamas" height="40" loading="lazy" sizes="40px" src="https://static01.nyt.com/images/2023/04/21/reader-center/author-nicholas-nehamas/author-nicholas-nehamas-thumbLarge.png?quality=75&auto=webp" srcset="https://static01.nyt.com/images/2023/04/21/reader-center/author-nicholas-nehamas/author-nicholas-nehamas-thumbStandard.png?quality=75&auto=webp 75w, https://static01.nyt.com/images/2023/04/21/reader-center/author-nicholas-nehamas/author-nicholas-nehamas-thumbLarge.png?quality=75&auto=webp 150w" width="40"/></span><div class="css-1ppjtba"><div><p class="css-kzazds e1jsehar1"><span class="css-1baulvz last-byline" itemprop="name">Nicholas Nehamas</span></p></div></div></div><p class="css-1ja5hfe">The Trump transition had an ally in the digital unit: Amy Gleason, a career employee who rejoined it late last year. She referred several job applicants who were later hired by DOGE after the inauguration.</p></a></div><div class="css-10avpiw" data-key="1525946.9699402817"><a class="css-1pwpj83" href="https://www.nytimes.com/2025/02/28/us/politics/musk-federal-bureaucracy-takeover.html"><div class="css-shshzh" data-testid="live-blog-byline"><span class="css-15zbqwq"><img alt="Kate Conger" height="40" loading="lazy" sizes="40px" src="https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbLarge-v5.jpg?quality=75&auto=webp" srcset="https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbStandard-v5.jpg?quality=75&auto=webp 75w, https://static01.nyt.com/images/2022/02/26/business/author-kate-conger/author-kate-conger-thumbLarge-v5.jpg?quality=75&auto=webp 150w" width="40"/></span><div class="css-1ppjtba"><div><p class="css-kzazds e1jsehar1"><span class="css-1baulvz last-byline" itemprop="name">Kate Conger</span></p></div></div></div><p class="css-1ja5hfe">After Trump was sworn in, Musk’s team swiftly inserted itself into more than 20 agencies, pushing out career employees and freezing grants. In his White House office, Musk installed a gaming computer and put a sign on his desk that read “DOGE.”</p></a></div></div><div class="css-r1a9vb"><div class="css-pnomtl"><div class="css-dbnm2s"></div><div class="css-b66c96"></div><div class="css-h1qsyw"></div><div class="css-sb29ax"></div><div class="css-1t9uiwm"></div></div><div class="css-1pqiz9q"><button aria-label="Previous" class="css-13ug63a" data-testid="Disappearing-Next" disabled="" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button><button aria-label="Next" class="css-1rh6kpg" data-testid="Disappearing-Next" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button></div></div></div></figure></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><section class="story-wrapper css-5rwu3a"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/8b200636-8df5-5caa-8daa-308efea287b0" href="https://www.nytimes.com/2025/02/28/podcasts/the-headlines/iowa-trans-rights-zelensky-trump.html"><div class="css-k008qs"><span class="css-nn5e6h"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 1px)"/><img alt="People gather to protest an Iowa bill to strip civil rights protections from transgender people at the Iowa Capitol in Des Moines." class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="People gather to protest an Iowa bill to strip civil rights protections from transgender people at the Iowa Capitol in Des Moines." class="css-122y91a" src="https://static01.nyt.com/images/2025/02/28/multimedia/28theheadlines-app-header-mvjq/28theheadlines-app-header-mvjq-square640.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></span><div><div class="css-xdandi"><div class="css-1a3ibh4"><p class="css-tdd4a3"><span class="css-1p26664">The Headlines</span><span class="css-inu6q5">Audio</span></p></div><p class="indicate-hover css-1a5fuvt">Iowa Moves to Eliminate Trans Rights, Volodymyr Zelensky Heads to the White House, and More</p></div><div class="css-zsumg4"><div class="css-1vmm8al"><svg fill="none" height="24" viewbox="0 0 28 28" width="24" xmlns="http://www.w3.org/2000/svg"><circle cx="14" cy="14" r="13.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M20.125 14L10.9375 19.3044L10.9375 8.69559L20.125 14Z" fill="var(--color-stroke-primary,#121212)"></path></svg></div><p class="css-fdpae6">9<!-- --> min listen</p></div></div></div></a></section></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-15rwwo"><div class="css-y7g724"><div><figure class="container-margin css-hurk9l"><div class="container-size-large"><section class="css-l08pwh interactive-content interactive-size-medium" data-id="100000010015190" data-source-id="100000010015190" data-testid="inline-interactive" id="prism-styln-us-ukraine-minerals-homepageMenu-1740606871165"><div class="css-17ih8de interactive-body" data-sourceid="100000010015190" id="embed-id-100000010015190" slug="prism-styln-us-ukraine-minerals-homepageMenu-1740606871165">
<script>window.registerInteractive && window.registerInteractive("100000010015190");</script>
<nav aria-labelledby="styln-us-ukraine-minerals-hp-menu" class="css-qvlk0g"><p id="styln-us-ukraine-minerals-hp-menu"><span class="css-14sajpe">U.S.-Ukraine Mineral Deal</span></p><ul class="css-11xr2bo"><li class="css-wc84xp"><a href="https://www.nytimes.com/article/ukraine-mineral-deal-trump.html">What to Know</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/12/world/europe/trump-ukraine-rare-earth-minerals.html">How the Deal Began</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/25/world/europe/trump-us-ukraine-mineral-deal.html">‘Protection Racket’ Diplomacy</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/26/us/politics/trump-biden-minerals-ukraine.html">U.S.’s Core Focus on Minerals</a></li></ul><script>"use strict";(function(){// prevent menus from overflowing into right rail on desktop
function a(){var a=document.querySelector("nav[aria-labelledby=\"styln-us-ukraine-minerals-hp-menu\"]"),b=document.querySelectorAll("[data-hierarchy=\"zone\"]"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth>c.clientWidth)// remove the last item from the menu if it is overflowing
{var d=a.querySelectorAll("li"),e=d[d.length-1];e.remove()}}// only check for overflow if the user is on desktop or tablet
// remove links that match ones already shown in the live band
var b=document.querySelector("#hp-live-band-list");if(b){var c=Array.from(b.querySelectorAll("a")).map(function(b){return b.href}),d=document.querySelectorAll("nav[aria-labelledby=\"styln-us-ukraine-minerals-hp-menu\"] li");d.forEach(function(a){var b,d=null===(b=a.querySelector("a"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia("(pointer: coarse) and (max-width: 739px)").matches){a();var e;window.addEventListener("resize",function(){clearTimeout(e),e=setTimeout(a,250)})}})();</script></nav></div></section></div></figure></div></div></div><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://legacycollection/452b1d37-7b45-5f33-86f9-23ba2244d6b3" href="https://www.nytimes.com/live/2025/02/28/us/trump-news"><div class="css-xdandi"><div class="css-1a3ibh4"><p class="css-ae0yjg"><span class="css-12tlih8">LIVE</span></p><span class="css-1ufpbe9"><time class="css-16lxk39" datetime="2025-02-28T13:10:16.130Z"><div class="css-ki347z"><span aria-hidden="true" class="css-1stvlmo" data-time="abs">Feb. 28, 2025, 8:10 a.m. ET</span><span class="css-kpxlkr" data-time="rel"></span></div></time></span></div><p class="indicate-hover css-91bpc3">Zelensky to Visit White House to Sign Minerals Deal</p></div><p class="summary-class css-1l5zmz6">President Trump is to meet with President Volodymyr Zelensky at the White House today after falsely asserting that Ukraine started the war with Russia.</p><p class="css-ih99h"> </p></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/e5314031-2e3d-5d39-83d9-210e3f501e95" href="https://www.nytimes.com/2025/02/28/world/europe/trump-starmer-king-charles.html"><div class="css-xdandi"><div class="css-1a3ibh4"><p class="css-tdd4a3"><span class="css-wt2ynm">Analysis</span></p></div><p class="indicate-hover css-91bpc3">With a Letter From King Charles, Keir Starmer Was Welcomed Into Trump’s Court</p></div><p class="summary-class css-1l5zmz6">Britain’s prime minister gave President Trump a royal invitation and scored several political wins. But his top goal — a security guarantee for Ukraine — remained elusive.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/3ab13ed6-8bfc-561a-926e-13bc18df7495" href="https://www.nytimes.com/2025/02/27/us/politics/starmer-ukraine-trump.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Trump Says He Believes Putin Would Abide by Any Ukraine Peace Deal</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">4 min read</p></div></div></a></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><figure class="css-1cv5kz9" data-slug="28trump-zelensky-header"><div class="css-ha69tt"><div><div><svg height="100%" version="1.1" width="100%" xmlns="http://www.w3.org/2000/svg"></svg></div></div><div><div><svg height="100%" version="1.1" width="100%" xmlns="http://www.w3.org/2000/svg"></svg></div></div></div><figcaption class="css-tm2y8a">Anna Rose Layden for The New York Times; Eric Lee/The New York Times</figcaption></figure></figure></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-15rwwo"><div class="css-y7g724"><div><figure class="container-margin css-hurk9l"><div class="container-size-large"><section class="css-l08pwh interactive-content interactive-size-medium" data-id="100000009939007" data-source-id="100000009939007" data-testid="inline-interactive" id="prism-styln-trump-administration-homepageMenu-1737426444607"><div class="css-17ih8de interactive-body" data-sourceid="100000009939007" id="embed-id-100000009939007" slug="prism-styln-trump-administration-homepageMenu-1737426444607">
<script>window.registerInteractive && window.registerInteractive("100000009939007");</script>
<nav aria-labelledby="styln-trump-administration-hp-menu" class="css-qvlk0g"><p id="styln-trump-administration-hp-menu"><span class="css-14sajpe">Trump Administration</span></p><ul class="css-11xr2bo"><li class="css-wc84xp"><a href="https://www.nytimes.com/interactive/2025/us/trump-agenda-2025.html">Tracking Major Moves</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/interactive/2025/us/trump-administration-lawsuits.html">Lawsuits Tracker</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/20/upshot/trump-executive-orders-legality.html">Is That Legal?</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/interactive/2025/01/29/us/politics/trump-cabinet-confirmations-tracker.html">Confirmations Tracker</a></li></ul><script>"use strict";(function(){// prevent menus from overflowing into right rail on desktop
function a(){var a=document.querySelector("nav[aria-labelledby=\"styln-trump-administration-hp-menu\"]"),b=document.querySelectorAll("[data-hierarchy=\"zone\"]"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth>c.clientWidth)// remove the last item from the menu if it is overflowing
{var d=a.querySelectorAll("li"),e=d[d.length-1];e.remove()}}// only check for overflow if the user is on desktop or tablet
// remove links that match ones already shown in the live band
var b=document.querySelector("#hp-live-band-list");if(b){var c=Array.from(b.querySelectorAll("a")).map(function(b){return b.href}),d=document.querySelectorAll("nav[aria-labelledby=\"styln-trump-administration-hp-menu\"] li");d.forEach(function(a){var b,d=null===(b=a.querySelector("a"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia("(pointer: coarse) and (max-width: 739px)").matches){a();var e;window.addEventListener("resize",function(){clearTimeout(e),e=setTimeout(a,250)})}})();</script></nav></div></section></div></figure></div></div></div><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/fe1a7c93-0a1a-5d69-9592-73c1b4faa68b" href="https://www.nytimes.com/2025/02/28/business/economy/trump-tariff-goals-contradictions.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">How Trump’s Tariffs Could Undermine Each Other</p></div><p class="summary-class css-1l5zmz6">President Trump has promised big results, from raising revenue to reviving domestic manufacturing. But many of his goals contradict one another.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">6 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/40788135-75c9-5cdc-bbf8-f46ef5b6d02d" href="https://www.nytimes.com/2025/02/28/business/dealbook/inflation-trump-tariffs.html"><div class="css-xdandi"><div class="css-1a3ibh4"><p class="css-tdd4a3"><span class="css-1p26664">DealBook</span><span class="css-inu6q5">Newsletter</span></p></div><p class="indicate-hover css-1a5fuvt">Inflation Is Stalking Trump</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">8 min read</p></div></div></a></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><a aria-hidden="true" class="css-9mylee" data-uri="nyt://article/fe1a7c93-0a1a-5d69-9592-73c1b4faa68b" href="https://www.nytimes.com/2025/02/28/business/economy/trump-tariff-goals-contradictions.html" tabindex="-1"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt='A woman, shown from behind, wearing a yellow jacket and holding a sign reading “Public Service is a Badge of Honor!" over her head.' class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt='A woman, shown from behind, wearing a yellow jacket and holding a sign reading “Public Service is a Badge of Honor!" over her head.' class="css-122y91a" src="https://static01.nyt.com/images/2025/02/27/multimedia/27dc-federal-cuts1-gvhj-hp/27dc-federal-cuts1-gvhj-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Anna Rose Layden for The New York Times</span></figcaption></a></figure></div></div><div class="css-1lvvmm"><div class="css-10kglqe e17qa79g0" span="7"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/9a41ce77-0343-5ae2-81c8-56cb5cb081cb" href="https://www.nytimes.com/2025/02/27/us/politics/trump-federal-worker-layoffs.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Office Closures and Relocations Part of Trump’s Plan for Large-Scale Layoffs</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section></div><div class="css-10kglqe e17qa79g0" span="7"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/d32ab414-1972-50fe-8c0a-208302f972b0" href="https://www.nytimes.com/2025/02/27/us/politics/federal-layoffs-trump-opm.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Memos Directing Mass Firings of Federal Workers Were Illegal, Judge Rules</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">3 min read</p></div></div></a></section></div></div></div></div></div><hr aria-hidden="true" class="css-e3mkx1"/><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/b0b07911-d060-5a9a-a3e6-e0db0d473d84" href="https://www.nytimes.com/2025/02/28/podcasts/the-daily/trump-minerals-ukraine-republican-budget.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">Trump 2.0: The Art of the Deal</p></div><p class="summary-class css-1l5zmz6">Watch or listen to our political round table about President Trump, the Republican budget and Ukraine.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">2 min read</p></div></div></a></section></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div><div class="css-ho9dtj"></div></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">The New York Times</span></figcaption></figure></div></div></div></div></div><hr aria-hidden="true" class="css-e3mkx1"/><div class="isPersonalizedAddOn"><div><div><div class="css-79elbk"><div class="css-12ilnc8" data-testid="carouselOuterClass"><div class="css-cn25ca"><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-0 css-1njmbkd"><div class="css-o0xbcl e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div><div class="css-gd99i5 e17qa79g0" span="5"><section class="story-wrapper css-1om0j5j"></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-1 css-1njmbkd"><div class="css-o0xbcl e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div><div class="css-gd99i5 e17qa79g0" span="5"><section class="story-wrapper css-1om0j5j"></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-2 css-1njmbkd"><div class="css-o0xbcl e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div><div class="css-gd99i5 e17qa79g0" span="5"><section class="story-wrapper css-1om0j5j"></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-3 css-1njmbkd"><div class="css-o0xbcl e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div><div class="css-gd99i5 e17qa79g0" span="5"><section class="story-wrapper css-1om0j5j"></section></div></div></div></div></div><div role="group"><div class="css-uf1mhr"><button aria-label="Previous" class="css-u9thtu" data-testid="Disappearing-Next" disabled="" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button><button aria-label="Next" class="css-1pvdjg1" data-testid="Disappearing-Next" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button></div></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><section><section class="story-wrapper"><figure class="container-margin css-160v3a8"><div class="container-size-large"><section class="css-l08pwh interactive-content interactive-size-medium" data-id="100000009942400" data-source-id="100000009942400" data-testid="inline-interactive" id="2025-hp-tip-strip"><div class="css-17ih8de interactive-body" data-sourceid="100000009942400" id="embed-id-100000009942400" slug="2025-hp-tip-strip">
<script>window.registerInteractive && window.registerInteractive("100000009942400");</script>
<p class="g-demo-sentence"> <a href="https://www.nytimes.com/tips"><b>Got a Tip?</b> The Times offers several ways to send important information confidentially ›</a></p>
</div></section></div></figure></section></section></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/dcdfc485-364a-5a09-bb3d-79f645a6f129" href="https://www.nytimes.com/2025/02/28/world/americas/argentina-crypto-scandal-president.html"><div class="css-1lvvmm"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">A Crypto Scam Swindled Argentines. What Did the President Know?</p></div><p class="summary-class css-1l5zmz6">A new cryptocurrency called $Libra and promoted by President Javier Milei bilked investors out of $250 million.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">9 min read</p></div></div></section></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="Javier Milei, the president of Argentina, stands in the middle of a crowd of supporters. " class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="Javier Milei, the president of Argentina, stands in the middle of a crowd of supporters. " class="css-122y91a" src="https://static01.nyt.com/images/2025/03/01/multimedia/22argentina-milei-crypto-hpmk/22argentina-milei-crypto-hpmk-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Magali Druscovich for The New York Times</span></figcaption></figure></div></div></a></section></div></div></div></div></div></div><div class="css-12qvnte e1ppw5w20"><div class="css-15rwwo"><div class="css-y7g724"><div><figure class="container-margin css-hurk9l"><div class="container-size-large"><section class="css-l08pwh interactive-content interactive-size-medium" data-id="100000010016286" data-source-id="100000010016286" data-testid="inline-interactive" id="prism-styln-gene-hackman-homepageMenu-1740663283666"><div class="css-17ih8de interactive-body" data-sourceid="100000010016286" id="embed-id-100000010016286" slug="prism-styln-gene-hackman-homepageMenu-1740663283666">
<script>window.registerInteractive && window.registerInteractive("100000010016286");</script>
<nav aria-labelledby="styln-gene-hackman-hp-menu" class="css-qvlk0g"><p id="styln-gene-hackman-hp-menu"><span class="css-14sajpe">Gene Hackman (1930-2025)</span></p><ul class="css-11xr2bo"><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/27/obituaries/gene-hackman-dead.html">Obituary</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/27/movies/gene-hackman-appraisal.html">Appraisal</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/27/movies/gene-hackman-hollywood-reaction.html">Luminaries Mourn</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/27/movies/gene-hackman-movies-streaming.html">Movies to Stream</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2001/12/16/movies/gene-hackman-hollywood-s-every-angry-man.html">2001 Profile</a></li></ul><script>"use strict";(function(){// prevent menus from overflowing into right rail on desktop
function a(){var a=document.querySelector("nav[aria-labelledby=\"styln-gene-hackman-hp-menu\"]"),b=document.querySelectorAll("[data-hierarchy=\"zone\"]"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth>c.clientWidth)// remove the last item from the menu if it is overflowing
{var d=a.querySelectorAll("li"),e=d[d.length-1];e.remove()}}// only check for overflow if the user is on desktop or tablet
// remove links that match ones already shown in the live band
var b=document.querySelector("#hp-live-band-list");if(b){var c=Array.from(b.querySelectorAll("a")).map(function(b){return b.href}),d=document.querySelectorAll("nav[aria-labelledby=\"styln-gene-hackman-hp-menu\"] li");d.forEach(function(a){var b,d=null===(b=a.querySelector("a"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia("(pointer: coarse) and (max-width: 739px)").matches){a();var e;window.addEventListener("resize",function(){clearTimeout(e),e=setTimeout(a,250)})}})();</script></nav></div></section></div></figure></div></div></div><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/85713c47-6ee2-52cc-84eb-02be4ce1ee1f" href="https://www.nytimes.com/2025/02/28/movies/gene-hackman-death-new-mexico.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">For Gene Hackman, a Jarring End to a Quiet, Art-Filled Life in Santa Fe</p></div><p class="summary-class css-1l5zmz6">The actor, who was found dead with his wife and one of their dogs, had written novels and painted since leaving Hollywood for retirement in New Mexico.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/cd0c66fd-7767-5885-b4d3-597efd65d1a0" href="https://www.nytimes.com/2025/02/28/us/gene-hackman-death-updates.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">What We Know About Hackman’s Death</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">3 min read</p></div></div></a></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><a aria-hidden="true" class="css-9mylee" data-uri="nyt://article/85713c47-6ee2-52cc-84eb-02be4ce1ee1f" href="https://www.nytimes.com/2025/02/28/movies/gene-hackman-death-new-mexico.html" tabindex="-1"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2025/02/28/multimedia/28hackman-hp-01-lqtz/28hackman-hp-01-lqtz-threeByTwoMediumAt2X-v2.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">George Brich/Associated Press</span></figcaption></a></figure></div></div><div class="css-1lvvmm"><div class="css-10kglqe e17qa79g0" span="7"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://interactive/d41992cc-6b6c-544d-a568-309e7846c9c9" href="https://www.nytimes.com/interactive/2025/02/27/movies/hackman-affidavit.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Read the Search Warrant Affidavit in the Death Inquiry</p></div></a></section></div><div class="css-10kglqe e17qa79g0" span="7"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/cb0fd188-e910-5caf-b97f-9959fe27aad8" href="https://www.nytimes.com/2025/02/27/movies/gene-hackman-unforgiven-bonnie-clyde.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">An Appraisal: A Smile That Could Give You Shivers</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">4 min read</p></div></div></a></section></div></div></div></div></div></div></div><div class="css-1w1paqe e1ppw5w20"><div class="css-1u1zmuv e1ppw5w20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/720399f2-c1ec-5608-9963-cfaa03abd183" href="https://www.nytimes.com/2025/02/28/world/asia/china-military-drills-pacific.html"><div class="css-1lvvmm"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">China’s Military Puts Pacific on Notice as U.S. Priorities Shift</p></div><p class="summary-class css-1l5zmz6">China is flexing its military muscle in the region to show that it will not wait for the Trump administration to decide how hard it wants to counter Beijing.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></section></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2025/02/28/multimedia/28china-military-promo/28china-military-clhj-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Hogp/Royal Australian Navy/ADF, via Associated Press</span></figcaption></figure></div></div></a></section></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-15rwwo"><div class="css-y7g724"><div><figure class="container-margin css-hurk9l"><div class="container-size-large"><section class="css-l08pwh interactive-content interactive-size-medium" data-id="100000009319548" data-source-id="100000009319548" data-testid="inline-interactive" id="prism-styln-iran-bombings-homepageMenu-1708267852451"><div class="css-17ih8de interactive-body" data-sourceid="100000009319548" id="embed-id-100000009319548" slug="prism-styln-iran-bombings-homepageMenu-1708267852451">
<script>window.registerInteractive && window.registerInteractive("100000009319548");</script>
<nav aria-labelledby="styln-middle-east-crisis-hp-menu" class="css-qvlk0g"><p id="styln-middle-east-crisis-hp-menu"><span class="css-14sajpe">Middle East Tensions</span></p><ul class="css-11xr2bo"><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/26/world/middleeast/who-are-the-israeli-hostages-hamas.html">4 Hostages’ Bodies Returned</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/24/world/middleeast/israel-west-bank-what-why.html">Rising West Bank Tensions</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/22/world/middleeast/syria-assad-israel-raids.html">Israeli Incursions Into Syria</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/14/world/middleeast/gaza-rubble-missing-bodies.html">Searching for Gaza’s Dead</a></li></ul><script>"use strict";(function(){// prevent menus from overflowing into right rail on desktop
function a(){var a=document.querySelector("nav[aria-labelledby=\"styln-middle-east-crisis-hp-menu\"]"),b=document.querySelectorAll("[data-hierarchy=\"zone\"]"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth>c.clientWidth)// remove the last item from the menu if it is overflowing
{var d=a.querySelectorAll("li"),e=d[d.length-1];e.remove()}}// only check for overflow if the user is on desktop or tablet
// remove links that match ones already shown in the live band
var b=document.querySelector("#hp-live-band-list");if(b){var c=Array.from(b.querySelectorAll("a")).map(function(b){return b.href}),d=document.querySelectorAll("nav[aria-labelledby=\"styln-middle-east-crisis-hp-menu\"] li");d.forEach(function(a){var b,d=null===(b=a.querySelector("a"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia("(pointer: coarse) and (max-width: 739px)").matches){a();var e;window.addEventListener("resize",function(){clearTimeout(e),e=setTimeout(a,250)})}})();</script></nav></div></section></div></figure></div></div></div><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/f86860c9-bc29-598c-bc53-5b81b1def8a5" href="https://www.nytimes.com/2025/02/28/world/middleeast/west-bank-jenin-palestinian-authority-israel.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">West Bank Operation Tests Palestinian Leaders’ Ability to Root Out Militants</p></div><p class="summary-class css-1l5zmz6">The Palestinian Authority wants to prove it can handle security in Gaza, even if it means working in parallel with a major Israeli military raid.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/eed2db6f-2fad-5bf8-b96f-b8ad776652ff" href="https://www.nytimes.com/2025/02/28/world/middleeast/syria-ramadan-economy-cash-shortage.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">As Ramadan Nears, Syrians Feel the Pinch of a Cash Shortage</p></div><p class="summary-class css-1l5zmz6">The Assad dictatorship is out, but Syria’s economy is in chaos after a civil war and recent policy shifts. The situation is dampening a typically festive season.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><a aria-hidden="true" class="css-9mylee" data-uri="nyt://article/f86860c9-bc29-598c-bc53-5b81b1def8a5" href="https://www.nytimes.com/2025/02/28/world/middleeast/west-bank-jenin-palestinian-authority-israel.html" tabindex="-1"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="Men in camouflage carrying guns step out of a military-style vehicle." class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="Men in camouflage carrying guns step out of a military-style vehicle." class="css-122y91a" src="https://static01.nyt.com/images/2025/02/06/multimedia/00jenin-01-gkcf/00jenin-01-gkcf-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Jaafar Ashtiyeh/Agence France-Presse — Getty Images</span></figcaption></a></figure></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/2734f206-c837-5724-a971-94aedb7c0cc5" href="https://www.nytimes.com/2025/02/27/us/iowa-transgender-civil-rights-bill.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">Iowa Lawmakers Pass Bill to Eliminate Transgender Civil Rights Protections</p></div><p class="summary-class css-1l5zmz6">If signed by Gov. Kim Reynolds, the Republican-backed measure would eliminate state civil rights protections for transgender Iowans.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">3 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/2b8e7341-5edb-508e-81d3-079dc1d8f638" href="https://www.nytimes.com/2025/02/27/us/politics/trans-troops-pentagon-figures.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Number of Trans Troops Is Far Lower Than Estimated, Pentagon Figures Show</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">2 min read</p></div></div></a></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><a aria-hidden="true" class="css-9mylee" data-uri="nyt://article/2734f206-c837-5724-a971-94aedb7c0cc5" href="https://www.nytimes.com/2025/02/27/us/iowa-transgender-civil-rights-bill.html" tabindex="-1"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="People holding signs in protest. " class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="People holding signs in protest. " class="css-122y91a" src="https://static01.nyt.com/images/2025/02/27/multimedia/27nat-iowa-transgender-01-htgp/27nat-iowa-transgender-01-htgp-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Rachel Mummey for The New York Times</span></figcaption></a></figure></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://interactive/1e284d42-0f2f-5814-a3d3-ad7fe8a1f4a1" href="https://www.nytimes.com/interactive/2025/02/27/us/politics/medicaid-enrollment.html"><div class="css-1lvvmm"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">More Than 70 Million Americans Are on Medicaid. This Is Where They Live.</p></div><p class="summary-class css-1l5zmz6">As Republicans weigh deep cuts, these congressional districts — some red, some blue — have the most to lose.</p></section></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2025/02/27/multimedia/2025-02-25-medicaid-enrollment-index/2025-02-25-medicaid-enrollment-index-threeByTwoMediumAt2X-v4.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">The New York Times</span></figcaption></figure></div></div></a></section></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-15rwwo"><div class="css-y7g724"><div><figure class="container-margin css-hurk9l"><div class="container-size-large"><section class="css-l08pwh interactive-content interactive-size-medium" data-id="100000008804928" data-source-id="100000008804928" data-testid="inline-interactive" id="prism-styln-academy-awards-2023-homepageMenu-1678397502869"><div class="css-17ih8de interactive-body" data-sourceid="100000008804928" id="embed-id-100000008804928" slug="prism-styln-academy-awards-2023-homepageMenu-1678397502869">
<script>window.registerInteractive && window.registerInteractive("100000008804928");</script>
<nav aria-labelledby="styln-academy-awards-hp-menu" class="css-qvlk0g"><p id="styln-academy-awards-hp-menu"><span class="css-14sajpe">Oscars 2025</span></p><ul class="css-11xr2bo"><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/01/23/movies/oscar-nominees-full-list.html">Nominees List</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/26/movies/oscar-nominees-dinner-photos.html">Photos: Nominees Dinner</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/12/movies/oscars-race-emilia-perez-anora.html">Where the Race Stands</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/02/26/magazine/timothee-chalamet-oscar-campaign.html">Timothée Chalamet’s Campaign</a></li><li class="css-wc84xp"><a href="https://www.nytimes.com/2025/01/23/movies/oscar-nominees-movies-streaming.html">Where to Stream</a></li></ul><script>"use strict";(function(){// prevent menus from overflowing into right rail on desktop
function a(){var a=document.querySelector("nav[aria-labelledby=\"styln-academy-awards-hp-menu\"]"),b=document.querySelectorAll("[data-hierarchy=\"zone\"]"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth>c.clientWidth)// remove the last item from the menu if it is overflowing
{var d=a.querySelectorAll("li"),e=d[d.length-1];e.remove()}}// only check for overflow if the user is on desktop or tablet
// remove links that match ones already shown in the live band
var b=document.querySelector("#hp-live-band-list");if(b){var c=Array.from(b.querySelectorAll("a")).map(function(b){return b.href}),d=document.querySelectorAll("nav[aria-labelledby=\"styln-academy-awards-hp-menu\"] li");d.forEach(function(a){var b,d=null===(b=a.querySelector("a"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia("(pointer: coarse) and (max-width: 739px)").matches){a();var e;window.addEventListener("resize",function(){clearTimeout(e),e=setTimeout(a,250)})}})();</script></nav></div></section></div></figure></div></div></div><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/61bfc8df-d91b-50a6-aecc-b9a82d7da8d9" href="https://www.nytimes.com/2025/02/28/movies/best-picture-nominees-oscars-conclave-wicked.html"><div class="css-xdandi"><p class="indicate-hover css-4blv3u">There’s Something Weird About This Year’s Oscars</p></div><p class="summary-class css-ofqxyv">Or with the 10 best picture nominees, at least, a critic writes. But what seems clear is that Hollywood is celebrating the kinds of movies it doesn’t make.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">9 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/dd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6" href="https://www.nytimes.com/2025/02/28/arts/dance/zoe-saldana-emilia-perez-dance.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">In Zoe Saldaña, a Choreographer for ‘Emilia Pérez’ Finds His Dream Dancer</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">4 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/b0813f6c-840e-5ae7-a88c-d5d430d3c564" href="https://www.nytimes.com/2025/02/28/world/americas/emilia-perez-mexico-oscars.html"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Why ‘Emilia Pérez,’ a Film About Mexico, Flopped in Mexico</p></div><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><a aria-hidden="true" class="css-9mylee" data-uri="nyt://article/61bfc8df-d91b-50a6-aecc-b9a82d7da8d9" href="https://www.nytimes.com/2025/02/28/movies/best-picture-nominees-oscars-conclave-wicked.html" tabindex="-1"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2025/02/27/multimedia/00morris-hp-promo/00morris-hp-promo-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Searchlight Pictures; Mubi; Netflix</span></figcaption></a></figure></div></div></div></div></div></div><div class="css-1u1zmuv e1ppw5w20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/eae58626-ae12-58cf-a801-1c30238d52ed" href="https://www.nytimes.com/2025/02/28/nyregion/adams-corruption-new-charge.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">Amid Furor Over Adams Case, a Glimpse of a Charge Never Brought</p></div><p class="summary-class css-1l5zmz6">Prosecutors said they had proposed a new charge against Mayor Eric Adams of New York. Court filings suggest it related to the conduct of an aide.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/2f9390e8-607b-51a0-b3ea-47be2f5821dc" href="https://www.nytimes.com/2025/02/28/nyregion/andrew-cuomo-new-york-mayor.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">If Cuomo Became Mayor, Would He Really Stand Up to Trump?</p></div><p class="summary-class css-1l5zmz6">Behind the perception of Andrew Cuomo as “Mr. Tough Guy” is the reality: The former governor has rarely criticized President Trump by name, our columnist writes.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">4 min read</p></div></div></a></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><a aria-hidden="true" class="css-9mylee" data-uri="nyt://article/eae58626-ae12-58cf-a801-1c30238d52ed" href="https://www.nytimes.com/2025/02/28/nyregion/adams-corruption-new-charge.html" tabindex="-1"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="A close-up of the face of Mayor Eric Adams." class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="A close-up of the face of Mayor Eric Adams." class="css-122y91a" src="https://static01.nyt.com/images/2025/02/26/multimedia/adams-obstruction-lbkc/adams-obstruction-lbkc-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Graham Dickie/The New York Times</span></figcaption></a></figure></div></div></div></div></div></div><div class="css-12qvnte e1ppw5w20"><section><section class="story-wrapper"><div class="css-79elbk"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/f04154fe-aaa0-5b58-bdd7-66b4439e06e5" href="https://www.nytimes.com/2025/02/28/business/shopping-addiction-no-buy-2025.html"><div class="css-1lvvmm"><div class="css-kuzbnk e17qa79g0" span="5"><div class="css-gx0lhm"><p class="indicate-hover css-6l658f">What Does It Take to Quit Shopping? Mute, Delete and Unsubscribe.</p><p class="summary-class css-17sbsu2 indicate-hover">Marketers followed consumers to social media and their phones. “Low Buy 2025” influencers are sharing tips for how to resist them.</p><p class="css-11uabb1" data-ttr="1">7 min read</p></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="Cassandra Orakpo in a denim dress holds a phone in front of four shelves filled with perfume bottles and a rack of clothes behind her." class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="Cassandra Orakpo in a denim dress holds a phone in front of four shelves filled with perfume bottles and a rack of clothes behind her." class="css-122y91a" src="https://static01.nyt.com/images/2025/02/18/multimedia/00shopaholics-orakpo-kbqp/00shopaholics-orakpo-kbqp-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Michael Stravato for The New York Times</span></figcaption></figure></div></div></a></div></section></section></div></div><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1l10c03 e17qa79g0" span="14"><section class="story-wrapper"><figure class="container-margin css-160v3a8"><div class="container-size-large"><section class="css-l08pwh interactive-content interactive-size-medium" data-id="100000009592393" data-source-id="100000009592393" data-testid="inline-interactive" id="weather-hp-strip"><div class="css-17ih8de interactive-body" data-sourceid="100000009592393" id="embed-id-100000009592393" slug="weather-hp-strip">
<script>window.registerInteractive && window.registerInteractive("100000009592393");</script>
<!-- birdkit: do not modify this file -->
<head>
</head>
<div class="birdkit-body g-weather-hp-strip" data-birdkit-hydrate="b1b90d249ea4f59d" data-preview-slug="weather-hp-strip" id="g-weather-hp-strip">
<div class="weather-hp-strip-grid s-zxpogMpJrieb"><div class="g-screenreader-only s-zxpogMpJrieb"><h2 class="g-screenreader" data-svelte-h="svelte-yjtbeg">Weather</h2></div> <div class="modules_wrapper modules_mobile s-IKV1CDMPALxt"><div class="weather-card-container s-IKV1CDMPALxt"><article class="loading-card s-wKKTKMWUBRLJ"> <div class="loading-header s-wKKTKMWUBRLJ"></div> <div class="loading-body s-wKKTKMWUBRLJ"><div class="loading-row"></div><div class="loading-row"></div><div class="loading-row"></div></div> <div class="loading-credit s-wKKTKMWUBRLJ"></div> </article> </div><div class="weather-card-container s-IKV1CDMPALxt"><article class="loading-card s-wKKTKMWUBRLJ"> <div class="loading-header s-wKKTKMWUBRLJ"></div> <div class="loading-body s-wKKTKMWUBRLJ"><div class="loading-row"></div><div class="loading-row"></div><div class="loading-row"></div></div> <div class="loading-credit s-wKKTKMWUBRLJ"></div> </article> </div><div class="weather-card-container s-IKV1CDMPALxt"><article class="loading-card s-wKKTKMWUBRLJ"> <div class="loading-header s-wKKTKMWUBRLJ"></div> <div class="loading-body s-wKKTKMWUBRLJ"><div class="loading-row"></div><div class="loading-row"></div><div class="loading-row"></div></div> <div class="loading-credit s-wKKTKMWUBRLJ"></div> </article> </div></div> <div class="wea-nav-container s-IKV1CDMPALxt"> </div> </div>
<script>
{
__sveltekit_1r4ocwm = {
base: new URL(".", location).pathname.slice(0, -1),
env: {"PUBLIC_BIRDKIT_CSS_SCOPED_CLASS":"g-weather-hp-strip","PUBLIC_BIRDKIT_PROJECT_SLUG":"weather-hp-strip"}
};
const element = document.querySelector('[data-birdkit-hydrate="b1b90d249ea4f59d"]');
const data = [null,{"type":"data","data":{body:[{type:"svelte",value:{component:"WeatherModules",marginInline:false,maxWidth:"1000px",wrapper:false,theme:"v1",modules:[{module:"WeatherCardToday",use_overrides:false,props:{credit:"Source: Tomorrow.io"}},{module:"WeatherCardWindChill",use_overrides:false,props:{}},{module:"WeatherCardRisk",use_overrides:false,hazard_type:"wssi",props:{}},{module:"WeatherCardAirQuality",use_overrides:false,props:{}}]}}],theme:"news",sheets:{},scoopProps:{uri:"nyt://embeddedinteractive/557659e5-f688-5fe8-bdc0-ed9d03c1fa1e",slug:"weather-hp-strip-hp-strip"}},"uses":{"url":1}}];
Promise.all([
import("https://static01.nytimes.com/newsgraphics/weather-hp-strip/cb02c00a-e5f5-41fb-b9ab-85ea8382b647/_assets/_app/immutable/entry/start.6f861287.js"),
import("https://static01.nytimes.com/newsgraphics/weather-hp-strip/cb02c00a-e5f5-41fb-b9ab-85ea8382b647/_assets/_app/immutable/entry/app.21f3107c.js")
]).then(([kit, app]) => {
kit.start(app, element, {
node_ids: [0, 3],
data,
form: null,
error: null,
params: {},
route: {"id":"/hp-strip"}
});
});
}
</script>
</div>
</div></section></div></figure></section></div></div></div></div></div></div></div></div></div></div><div class="css-1bjokvb e17qa79g0" span="6"><div><div data-hierarchy="zone" data-testid="programming-node"><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><div class="isPersonalizedPackage"><div><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-ho9dtj"><picture class="css-hdqqnp"></picture></div></figure></section><hr aria-hidden="true" class="css-e3mkx1"/><div class="isPersonalizedAddOn"><div><div class="css-79elbk"><div class="css-12ilnc8" data-testid="carouselOuterClass"><div class="css-ztw7vs"><div class="css-1as87w5 e17qa79g0" span="1"><div class="css-17jnd0c"><div class="css-1qpxn73 e17qa79g0" span="6"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="css-17jnd0c"><div class="css-1qpxn73 e17qa79g0" span="6"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="css-17jnd0c"><div class="css-1qpxn73 e17qa79g0" span="6"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="css-17jnd0c"><div class="css-1qpxn73 e17qa79g0" span="6"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div></div></div><div role="group"><div class="css-m3y3ka"><button aria-label="Previous" class="css-6gfk0j" data-testid="Disappearing-Next" disabled="" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button><button aria-label="Next" class="css-1qle2ps" data-testid="Disappearing-Next" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button></div></div></div></div></div></div></div></div></div><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><div class="css-15rwwo"><div class="css-y7g724"><div><figure class="container-margin css-hurk9l"><div class="container-size-small"><section class="css-l08pwh interactive-content interactive-size-medium" data-id="100000009780651" data-source-id="100000009780651" data-testid="inline-interactive" id="large-opinion-label"><div class="css-17ih8de interactive-body" data-sourceid="100000009780651" id="embed-id-100000009780651" slug="large-opinion-label">
<script>window.registerInteractive && window.registerInteractive("100000009780651");</script>
<div class="g-large-opinion-label">
<a href="https://www.nytimes.com/section/opinion">
Opinion
</a>
</div></div></section></div></figure></div></div></div><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/351b30ec-12d0-5d59-9f63-5d81a895ded1" href="https://www.nytimes.com/2025/02/28/opinion/free-speech-trump-maga.html"><p class="css-hr63kf">The Editorial Board</p><p class="indicate-hover css-uzitgk">Language Police Have Taken Over the White House </p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">8 min read</p></div></div></a></section><hr aria-hidden="true" class="css-e3mkx1"/><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/6717e682-229e-51b8-9947-2b735defd735" href="https://www.nytimes.com/2025/02/28/opinion/horse-racing-government-subsidies.html"><p class="css-hr63kf">Noah Shachtman</p><p class="indicate-hover css-uzitgk">Why Does the Government Pay Billions for This Deadly, Unpopular Sport?</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">17 min read</p></div></div><figure class="container-margin css-1debogi"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="A brown racehorse in mid gallop, with a jockey perched atop. " class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="A brown racehorse in mid gallop, with a jockey perched atop. " class="css-122y91a" src="https://static01.nyt.com/images/2025/03/02/multimedia/02cover1-kphj/02cover1-kphj-threeByTwoMediumAt2X-v2.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Justin Kaneps for The New York Times</span></figcaption></figure></a></section><hr aria-hidden="true" class="css-e3mkx1"/><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/f08fbb32-8818-558e-8473-71631754acea" href="https://www.nytimes.com/2025/02/28/opinion/donald-trump-birthright-citizenship.html"><p class="css-hr63kf">Frank Bruni, Nicole Hemmer and Tim Miller</p><p class="indicate-hover css-uzitgk">‘Like Raptors Flinging Themselves Against an Electric Fence’: 3 Writers on Trump’s First Month</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">13 min read</p></div></div></a></section><hr aria-hidden="true" class="css-e3mkx1"/><section class="story-wrapper css-szc2m3"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/96f9592e-54a6-5dea-845b-785346ba26b1" href="https://www.nytimes.com/2025/02/28/opinion/andrew-tate-donald-trump.html"><div class="css-cephuo"><div class="css-4dgob3 e17qa79g0" span="3"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (max-width: 600px)"/><img alt="Andrew Tate’s head partly obscuring Tristan Tate’s head." class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="Andrew Tate’s head partly obscuring Tristan Tate’s head." class="css-122y91a" src="https://static01.nyt.com/images/2025/02/28/multimedia/28goldberg-wczt/28goldberg-wczt-smallSquare252.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></div></div><p class="css-hr63kf">Michelle Goldberg</p><p class="indicate-hover css-uzitgk">Andrew Tate, Accused Sex Trafficker, Is Trump’s Kind of Guy</p><p class="css-hf5jg6" data-ttr="1">4 min read</p></a></section><hr aria-hidden="true" class="css-e3mkx1"/><section><section aria-label="More Stories" class="css-1jke4yk"><div class="css-i6bazn"><div class="css-fyro28"><div aria-roledescription="carousel" class="css-6w67yl" role="tablist" style="transform:translateX(0%)
translateX(0px)"><div class="css-jsjvin" role="tab"><div class="css-1rr4qq7"><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/896e3424-8c01-5485-bb02-1560953263ba" href="https://www.nytimes.com/2025/02/27/opinion/government-great-progressive-abundance.html"><p class="css-hr63kf">David Brooks</p><p class="indicate-hover css-kaomn7">We Can Achieve Great Things</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">6 min read</p></div></div></a></section></div><div class="css-1rr4qq7"><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/5452cb92-2c7c-5c19-b4c5-863234fce6ab" href="https://www.nytimes.com/2025/02/28/opinion/elon-musk-south-africa.html"><p class="css-hr63kf">William Shoki</p><p class="indicate-hover css-kaomn7">Elon Musk Is South African. We Shouldn’t Forget It.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section></div></div><div class="css-jsjvin" role="tab"><div class="css-1rr4qq7"><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a" href="https://www.nytimes.com/2025/02/28/opinion/texas-measles-vaccine.html"><p class="css-hr63kf">Zeynep Tufekci</p><p class="indicate-hover css-kaomn7">The Texas Measles Outbreak Is Even Scarier Than It Looks</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section></div><div class="css-1rr4qq7"><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/f716ba76-77d6-558b-9a94-bf1f5f1718b1" href="https://www.nytimes.com/2025/02/28/opinion/medicaid-republicans.html"><p class="css-hr63kf">Michael Kinnucan</p><p class="indicate-hover css-kaomn7">Republicans Want to Gut Medicaid. They Might Regret It.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section></div></div><div class="css-jsjvin" role="tab"><div class="css-1rr4qq7"><section class="story-wrapper css-5rwu3a"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/e4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c" href="https://www.nytimes.com/2025/02/28/opinion/matter-of-opinion-final-episode.html"><div class="css-1szm689"><div><div class="css-xdandi"><div class="css-1a3ibh4"><p class="css-tdd4a3"><span class="css-1p26664">Matter of Opinion</span><span class="css-inu6q5">Audio</span></p></div><p class="indicate-hover css-kaomn7">Your Questions Answered, and a Big Announcement</p></div><div class="css-zsumg4"><div class="css-1vmm8al"><svg fill="none" height="24" viewbox="0 0 28 28" width="24" xmlns="http://www.w3.org/2000/svg"><circle cx="14" cy="14" r="13.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M20.125 14L10.9375 19.3044L10.9375 8.69559L20.125 14Z" fill="var(--color-stroke-primary,#121212)"></path></svg></div><p class="css-fdpae6">46<!-- --> min listen</p></div></div></div></a></section></div><div class="css-1rr4qq7"><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/a3f3b0d1-5608-54dd-a08b-83d185b5db46" href="https://www.nytimes.com/live/2025/02/25/opinion/thepoint/barnard-college-sit-in-palestinian-israel-protest"><p class="css-hr63kf">Bret Stephens</p><p class="indicate-hover css-kaomn7">Earth to Barnard College: Enough</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">2 min read</p></div></div></a></section></div></div><div class="css-jsjvin" role="tab"><div class="css-1rr4qq7"><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/e5fb45b5-1082-5fcc-ba9a-5bcc56a75975" href="https://www.nytimes.com/2025/02/27/opinion/trump-hegseth-pentagon.html"><p class="css-hr63kf">David French</p><p class="indicate-hover css-kaomn7">Trump’s Decision to Fire the JAG Generals Gives the Game Away</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">9 min read</p></div></div></a></section></div><div class="css-1rr4qq7"><section class="story-wrapper"><a aria-hidden="false" class="css-rgq5s4" data-uri="nyt://article/1b78bf9c-210e-5ae9-99a4-980a3696891d" href="https://www.nytimes.com/2025/02/27/opinion/trump-republicans-masculinity-gender-traditional.html"><p class="css-hr63kf">Michael Tesler, John Sides and Colette Marcellin</p><p class="indicate-hover css-kaomn7">Republican Men and Women Are Changing Their Minds About How Women Should Behave</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section></div></div></div></div></div><div role="group"><div class="css-m3y3ka"><button aria-label="Previous" class="css-6gfk0j" data-testid="Disappearing-Next" disabled="" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button><button aria-label="Next" class="css-1qle2ps" data-testid="Disappearing-Next" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button></div></div></section></section></div></div><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><div><div class=""><div class="css-hep49k e1yccyp20"><div class="css-b6n3ve"><div class="css-1d18fn2 e17qa79g0" span="6"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/fe3aa929-085b-529c-9598-e16fc54c363b" href="https://www.nytimes.com/2025/02/28/arts/television/stephen-colbert-trump-voters-remorse.html"><div class="css-b6n3ve"><div class="css-kuec6r e17qa79g0" span="4"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Late Night: Buyer’s Remorse</p></div><p class="summary-class css-1l5zmz6">Stephen Colbert discussed a new poll that indicated that some Trump voters were regretting their decision.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">2 min read</p></div></div></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (max-width: 600px)"/><img alt="Stephen Colbert, in a suit, stands onstage." class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="Stephen Colbert, in a suit, stands onstage." class="css-122y91a" src="https://static01.nyt.com/images/2025/02/28/arts/28latenight/28latenight-smallSquare252.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></div></div></a></section></div></div></div></div></div></div></div><div class="css-1w1paqe e1ppw5w20"><div><div class="css-1u1zmuv e1ppw5w20"><section class="story-wrapper css-1om0j5j"><figure class="container-margin css-1debogi"><div class="css-ho9dtj"><picture class="css-hdqqnp"></picture></div></figure></section><hr aria-hidden="true" class="css-e3mkx1"/><section class="story-wrapper css-1om0j5j"></section></div></div></div></div></div></div></div></div><div class="css-1r9ysjz e1ppw5w20"><div><div class="css-rpp6l6 e1xxpj0j0"><div class="css-tt22rr e1xxpj0j1"><div class="css-aljv3" data-testid="StandardAd"><p class="css-le4k3i">Advertisement</p><a class="css-777zgl" href="#after-dfp-ad-mid1">SKIP ADVERTISEMENT</a><div class="ad dfp-ad-mid1-wrapper css-rfqw0c" id="dfp-ad-mid1"></div><div id="after-dfp-ad-mid1"></div></div></div></div></div></div><div class="css-12qvnte e1ppw5w20"><div data-hierarchy="container" data-testid="programming-node"><div data-hierarchy="zone" data-testid="programming-node"><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><div class="css-v7w2uh"><span>More News</span></div></h2></div></div><div class="css-m5ahyg"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-1lvvmm"><div class="css-f52tr7 e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><div class="css-tlxejj"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/7cd260c2-752a-5a3c-9978-3e999d9b5dfa" href="https://www.nytimes.com/2025/02/27/us/politics/mexico-cartel-sheinbaum-trump.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">Mexico Transfers Dozens of Cartel Operatives to U.S. Custody</p></div><p class="summary-class css-1l5zmz6">The traffickers will face charges in U.S. courts. Among them is Rafael Caro Quintero, a Sinaloa cartel founder whom U.S. officials have been after for 40 years.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">5 min read</p></div></div></a></section></div></div><div class="css-tlxejj"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/aff5b023-b154-5fd7-ac1a-bc207716b35c" href="https://www.nytimes.com/2025/02/27/business/jeffrey-epstein-files-pam-bondi.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">‘Epstein Files’ Release, Hyped by Pam Bondi, Falls Short of Expectations</p></div><p class="summary-class css-1l5zmz6">The release of flight logs and Jeffrey Epstein’s contact list by the attorney general was met with criticism from those who had expected new information.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">4 min read</p></div></div></a></section></div></div><div class="css-tlxejj"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/d1966252-a51e-5c6f-8d72-f5b7f05eb56d" href="https://www.nytimes.com/2025/02/28/nyregion/citigroup-81-trillion-error.html"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">Citigroup Accidentally Credits Customer’s Account With $81 Trillion</p></div><p class="summary-class css-1l5zmz6">The bank temporarily credited a customer’s account with trillions of dollars, adding to scrutiny of risk management systems after a series of errors.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">2 min read</p></div></div></a></section></div></div></div></div><div class="css-18c0apr e17qa79g0" span="9"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/adab900a-3ca2-5afe-b1d4-477fc6bb909e" href="https://www.nytimes.com/2025/02/28/world/canada/canada-us-trump-tariffs-reactions.html"><div class="css-f6ozty e1yccyp20"><figure class="container-margin css-170wooj"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="A sign in a store says, “Buy Canadian Instead.’’" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="A sign in a store says, “Buy Canadian Instead.’’" class="css-122y91a" src="https://static01.nyt.com/images/2025/02/28/multimedia/28canada-angry-explainer-1-vgkz/28canada-angry-explainer-1-vgkz-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Chris Helgren/Reuters</span></figcaption></figure><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-91bpc3">How Canadians Are Making Their Anger Toward the U.S. Loud and Clear</p></div><p class="summary-class css-1l5zmz6">Canadians are shunning U.S. products and abandoning trips to America to protest the economic punishment President Trump has threatened to impose with tariffs.</p><div class="css-1tic89u"><div><p class="css-1a0ymrn" data-ttr="1">4 min read</p></div></div></section></div></a></section></div></div></div><div class="css-1d18fn2 e17qa79g0" span="6"><div class="isPersonalizedAddOn"><div><div><div class="css-b6n3ve"><div class="css-1d18fn2 e17qa79g0" span="6"><div class="css-hep49k e1yccyp20"><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section></div></div></div></div></div></div></div></div></div></div></div></div></div><div class="css-12qvnte e1ppw5w20"><div data-hierarchy="container" data-testid="programming-node"><div data-hierarchy="zone" data-testid="programming-node"><div class="css-17jkqqy e1ppw5w20"><div class="css-h753dc e1ppw5w20"><div class="isPersonalizedPackage"><div><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><div class="css-v7w2uh"><span>Well</span></div></h2></div></div><div><div class="css-79elbk"><div class="css-12ilnc8" data-testid="carouselOuterClass"><div class="css-n3ncu4"><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-0 css-fi9dry"><div class="css-cw4snp e17qa79g0" span="4"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-1 css-fi9dry"><div class="css-cw4snp e17qa79g0" span="4"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-2 css-fi9dry"><div class="css-cw4snp e17qa79g0" span="4"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-3 css-fi9dry"><div class="css-cw4snp e17qa79g0" span="4"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div><div class="css-1as87w5 e17qa79g0" span="1"><div class="carousel-story-4 css-fi9dry"><div class="css-cw4snp e17qa79g0" span="4"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></div></div></div><div role="group"><div class="css-uf1mhr"><button aria-label="Previous" class="css-u9thtu" data-testid="Disappearing-Next" disabled="" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button><button aria-label="Next" class="css-1pvdjg1" data-testid="Disappearing-Next" disabled="" tabindex="-1" type="button"><svg viewbox="0 0 26 26"><circle cx="13" cy="13" r="12.5" stroke="var(--color-stroke-quaternary,#DFDFDF)"></circle><path d="M10.3984 7.7998L15.5984 12.9998L10.3984 18.1998" fill="none" stroke-width="1.5"></path></svg></button></div></div></div></div></div></div></div><div class="css-1r9ysjz e1ppw5w20"></div></div><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><section><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><div class="css-v7w2uh"><span>Culture and Lifestyle</span></div></h2></div></div><div class="css-m5ahyg"><div class="css-1l10c03 e17qa79g0" span="14"><section class="story-wrapper"><div class="css-79elbk"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://article/911b0155-1b65-5576-91a0-215b59fb3215" href="https://www.nytimes.com/2025/02/27/style/sharon-van-etten-album.html"><div class="css-1lvvmm"><div class="css-kuzbnk e17qa79g0" span="5"><div class="css-gx0lhm"><p class="indicate-hover css-6l658f">Sharon Van Etten Finds Her Way Home</p><p class="summary-class css-17sbsu2 indicate-hover">For her seventh album, the indie singer-songwriter comes to New Jersey to play on her old turf and process the past.</p><p class="css-11uabb1" data-ttr="1">7 min read</p></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div class="css-ho9dtj"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (min-width: 601px)"/><img alt="A woman in a black coat sitting on the porch of a house, there are two doors behind her." class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="A woman in a black coat sitting on the porch of a house, there are two doors behind her." class="css-122y91a" src="https://static01.nyt.com/images/2025/02/27/multimedia/25SHARON-VAN-ETTEN-05-pkgm/25SHARON-VAN-ETTEN-05-pkgm-threeByTwoMediumAt2X.jpg?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div><figcaption aria-hidden="true" class="css-1p5yz2j"><span class="css-1yg7u8y">Sabrina Santiago for The New York Times</span></figcaption></figure></div></div></a></div></section></div><div class="css-1d18fn2 e17qa79g0" span="6"><div class="isPersonalizedAddOn"><div><div><div class=""><div class="css-hep49k e1yccyp20"><div class="css-b6n3ve"><div class="css-1d18fn2 e17qa79g0" span="6"><section class="story-wrapper"><div class="css-b6n3ve"><div class="css-kuec6r e17qa79g0" span="4"><section class="story-wrapper css-1om0j5j"></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div></div></section></div></div><div class="css-b6n3ve"><div class="css-1d18fn2 e17qa79g0" span="6"><section class="story-wrapper"><div class="css-b6n3ve"><div class="css-kuec6r e17qa79g0" span="4"><section class="story-wrapper css-1om0j5j"></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div></div></section></div></div><div class="css-b6n3ve"><div class="css-1d18fn2 e17qa79g0" span="6"><section class="story-wrapper"><div class="css-b6n3ve"><div class="css-kuec6r e17qa79g0" span="4"><section class="story-wrapper css-1om0j5j"></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div></div></section></div></div></div></div></div></div></div></div></div></section></div></div><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><div class="isPersonalizedPackage"><div><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><a aria-hidden="false" class="css-9mylee" data-uri="" href="https://www.nytimes.com/athletic/"><div class="css-1lel406"><p class="css-2z4gz0"><span>The Athletic</span></p><span class="css-wne7fp">Sports coverage</span></div></a></h2></div></div><div class="css-m5ahyg"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div class="css-ho9dtj"><picture class="css-hdqqnp"></picture></div></figure></div></div></div></div><div class="css-1d18fn2 e17qa79g0" span="6"><div class="isPersonalizedAddOn"><div><div><div class="css-b6n3ve"><div class="css-1d18fn2 e17qa79g0" span="6"><div class="css-hep49k e1yccyp20"><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section></div></div></div></div></div></div><hr aria-hidden="true" class="css-e3mkx1"/></div></div></div></div></div></div><div class="css-17jkqqy e1ppw5w20"><div class="css-h753dc e1ppw5w20"><div class="isPersonalizedPackage"><div><div><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><div class="css-1lel406"><p class="css-2z4gz0"><span>Audio</span></p><span class="css-wne7fp">Podcasts and narrated articles</span></div></h2></div></div><div class=""><div class="css-hep49k e1yccyp20"><div class="css-m5ahyg"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><div class="css-tlxejj"><div class="css-50ivt8 e17qa79g0" span="3"><section class="story-wrapper css-1om0j5j"></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div></div></section></div><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><div class="css-tlxejj"><div class="css-50ivt8 e17qa79g0" span="3"><section class="story-wrapper css-1om0j5j"></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div></div></section></div><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><div class="css-tlxejj"><div class="css-50ivt8 e17qa79g0" span="3"><section class="story-wrapper css-1om0j5j"></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div></div></section></div><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper"><div class="css-tlxejj"><div class="css-50ivt8 e17qa79g0" span="3"><section class="story-wrapper css-1om0j5j"></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></div></div></section></div></div></div></div></div></div></div></div><div class="css-1r9ysjz e1ppw5w20"></div></div><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><div class="isPersonalizedPackage"><div><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><a aria-hidden="false" class="css-9mylee" data-uri="" href="https://cooking.nytimes.com/"><div class="css-1lel406"><p class="css-2z4gz0"><span>Cooking</span></p><span class="css-wne7fp">Recipes and guides</span></div></a></h2></div></div><div class="css-m5ahyg"><div class="css-1l10c03 e17qa79g0" span="14"><div class="css-hep49k e1yccyp20"><div class="css-1lvvmm"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><section class="story-wrapper css-1om0j5j"></section><section class="story-wrapper css-1om0j5j"></section></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div class="css-ho9dtj"><picture class="css-hdqqnp"></picture></div></figure></div></div></div></div><div class="css-1d18fn2 e17qa79g0" span="6"><div class="isPersonalizedAddOn"><div><div><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><div class="css-v7w2uh"><span>Most Popular This Week</span></div></h2></div></div><div class=""><div class="css-hep49k e1yccyp20"><div class="css-b6n3ve"><div class="css-remrbt e17qa79g0" span="3"><section class="story-wrapper"><div class="css-oz0kdv"><div class="css-remrbt e17qa79g0" span="3"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></section></div><div class="css-remrbt e17qa79g0" span="3"><section class="story-wrapper"><div class="css-oz0kdv"><div class="css-remrbt e17qa79g0" span="3"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></section></div></div><div class="css-b6n3ve"><div class="css-remrbt e17qa79g0" span="3"><section class="story-wrapper"><div class="css-oz0kdv"><div class="css-remrbt e17qa79g0" span="3"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></section></div><div class="css-remrbt e17qa79g0" span="3"><section class="story-wrapper"><div class="css-oz0kdv"><div class="css-remrbt e17qa79g0" span="3"><section class="story-wrapper css-3o0db8"><figure class="container-margin css-125baf0"><div class="css-wne2ji"><picture class="css-hdqqnp"></picture></div></figure></section></div></div></section></div></div></div></div></div></div></div></div></div></div></div></div></div><div class="css-1w1paqe e1ppw5w20"><div class="css-12qvnte e1ppw5w20"><div class="isPersonalizedPackage"><div><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><a aria-hidden="false" class="css-9mylee" data-uri="" href="https://www.nytimes.com/wirecutter/"><div class="css-1lel406"><p class="css-2z4gz0"><span>Wirecutter</span></p><span class="css-wne7fp">Product recommendations</span></div></a></h2></div></div><div class="css-m5ahyg"><div class="css-14ywp0y e17qa79g0" span="20"><div class="css-m5ahyg"><div class="css-1432v6n e17qa79g0" span="5"><div class="css-hep49k e1yccyp20"><div class="css-tlxejj"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper css-1om0j5j"></section></div></div><div class="css-tlxejj"><div class="css-f52tr7 e17qa79g0" span="5"><section class="story-wrapper css-1om0j5j"></section></div></div></div></div><div class="css-1e7omub e17qa79g0" span="9"><figure class="container-margin css-hurk9l"><div class="css-ho9dtj"><picture class="css-hdqqnp"></picture></div></figure></div><div class="css-1d18fn2 e17qa79g0" span="6"><div class="css-hep49k e1yccyp20"><div class="css-b6n3ve"><div class="css-1d18fn2 e17qa79g0" span="6"><section class="story-wrapper css-1om0j5j"></section></div></div><div class="css-b6n3ve"><div class="css-1d18fn2 e17qa79g0" span="6"><section class="story-wrapper css-1om0j5j"></section></div></div></div></div></div></div></div></div></div></div></div><div class="css-17jkqqy e1ppw5w20"><div class="css-h753dc e1ppw5w20"><div><div class="css-15rwwo"><div class="package-title-wrapper css-1wd5atx"><h2><div class="css-1lel406"><p class="css-2z4gz0"><span>Games</span></p><span class="css-wne7fp">Daily puzzles</span></div></h2></div></div><div class=""><div class="css-hep49k e1yccyp20"><div class="css-m5ahyg"><div class="css-10kglqe e17qa79g0" span="7"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://promo/c526680a-46b9-5c63-8313-c3aefb0c2df3" href="https://www.nytimes.com/games/wordle/index.html"><div class="css-vrywmf"><div class="css-1432v6n e17qa79g0" span="5"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Wordle</p></div><p class="summary-class css-1l5zmz6">Guess the 5-letter word with 6 chances.</p></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (max-width: 600px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2022/03/02/crosswords/alpha-wordle-icon-new/alpha-wordle-icon-new-smallSquare252-v3.png?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></div></div></a></section></div><div class="css-10kglqe e17qa79g0" span="7"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://promo/03b461e3-31bb-5fd0-be52-8fa3e27c8e6b" href="https://www.nytimes.com/games/connections?GAMES_connectionsRollout_1130=1_ConnectionsV2"><div class="css-vrywmf"><div class="css-1432v6n e17qa79g0" span="5"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Connections</p></div><p class="summary-class css-1l5zmz6">Group words that share a common thread.</p></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (max-width: 600px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2023/08/25/crosswords/alpha-connections-icon-original/alpha-connections-icon-original-smallSquare252.png?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></div></div></a></section></div><div class="css-1d18fn2 e17qa79g0" span="6"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://promo/b2a56bdd-40da-54e0-9c71-42cc74c71765" href="https://www.nytimes.com/games/strands"><div class="css-b6n3ve"><div class="css-kuec6r e17qa79g0" span="4"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Strands</p></div><p class="summary-class css-1l5zmz6">Uncover hidden words and reveal the theme.</p></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (max-width: 600px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2024/01/16/crosswords/alpha-strands-icon/alpha-strands-icon-smallSquare252.png?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></div></div></a></section></div></div><div class="css-m5ahyg"><div class="css-10kglqe e17qa79g0" span="7"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://promo/8788a6e9-0db1-5c7c-a8e8-d82c37ccfdd0" href="https://www.nytimes.com/puzzles/spelling-bee"><div class="css-vrywmf"><div class="css-1432v6n e17qa79g0" span="5"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">Spelling Bee</p></div><p class="summary-class css-1l5zmz6">How many words can you make with 7 letters?</p></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (max-width: 600px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2020/03/23/crosswords/spelling-bee-logo-nytgames-hi-res/spelling-bee-logo-nytgames-hi-res-smallSquare252-v4.png?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></div></div></a></section></div><div class="css-10kglqe e17qa79g0" span="7"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://promo/f7f1225d-6bba-5864-ad80-682122a9fbbf" href="https://www.nytimes.com/crosswords"><div class="css-vrywmf"><div class="css-1432v6n e17qa79g0" span="5"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">The Crossword</p></div><p class="summary-class css-1l5zmz6">Get clued in with wordplay, every day.</p></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (max-width: 600px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2020/03/23/crosswords/crossword-logo-nytgames-hires/crossword-logo-nytgames-hires-smallSquare252-v3.png?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></div></div></a></section></div><div class="css-1d18fn2 e17qa79g0" span="6"><section class="story-wrapper"><a aria-hidden="false" class="css-9mylee" data-uri="nyt://promo/c397d2d2-758a-5a3a-a897-2add6cf9ae78" href="http://www.nytimes.com/crosswords/game/mini"><div class="css-b6n3ve"><div class="css-kuec6r e17qa79g0" span="4"><section class="story-wrapper"><div class="css-xdandi"><p class="indicate-hover css-1a5fuvt">The Mini Crossword</p></div><p class="summary-class css-1l5zmz6">Solve this bite-sized puzzle in just a few minutes.</p></section></div><div class="css-1fvhbq8 e17qa79g0" span="2"><figure class="container-margin css-hurk9l"><div class="css-wne2ji"><picture class="css-hdqqnp"><source class="css-hdqqnp" media="screen and (max-width: 600px)"/><img alt="" class="css-dzl7b5" fetchpriority="auto" loading="lazy"/><noscript><img alt="" class="css-122y91a" src="https://static01.nyt.com/images/2021/03/23/multimedia/alpha-mini-promo-1616527576800/alpha-mini-promo-1616527576800-smallSquare252-v4.png?format=pjpg&quality=75&auto=webp&disable=upscale"/></noscript></picture></div></figure></div></div></a></section></div></div></div></div></div></div><div class="css-1r9ysjz e1ppw5w20"></div></div></div></div></div></div></div><div class="css-19pyzy9"><div><div class="css-1vzzb6i"><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div></div><div class="css-1vzzb6i"><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div></div><div class="css-1vzzb6i"><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div></div><div class="css-1vzzb6i"><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div></div><div class="css-1vzzb6i"><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div></div><div class="css-1vzzb6i"><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div></div><div class="css-1vzzb6i"><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div></div><div class="css-1vzzb6i"><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div><div class="css-1nkoa58"><div class="css-1x6dw1b"></div><div class="css-kvmiee"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div><div class="css-gf25qg"></div></div></div></div></div></div></main><nav aria-labelledby="site-index-label" class="css-1jmk4jh" data-testid="site-index" id="site-index"><h2 class="css-1dv1kvn" id="site-index-label">Site Index</h2><div class="css-uc4bdz"></div></nav><footer class="css-1e1s8k7" role="contentinfo"><nav class="css-1qa4qp6" data-testid="footer"><h2 class="css-1dv1kvn">Site Information Navigation</h2><ul class="css-1ho5u4o edvi3so0"><li data-testid="copyright"><a class="css-13mf4b4" href="https://help.nytimes.com/hc/en-us/articles/115014792127-Copyright-notice">© <span>2025</span> <span>The New York Times Company</span></a></li></ul><ul class="css-t8x4fj edvi3so1"><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://www.nytco.com/">NYTCo</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://help.nytimes.com/hc/en-us/articles/115015385887-Contact-Us">Contact Us</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://help.nytimes.com/hc/en-us/articles/115015727108-Accessibility">Accessibility</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://www.nytco.com/careers/">Work with us</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://advertising.nytimes.com/">Advertise</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://www.tbrandstudio.com/">T Brand Studio</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://www.nytimes.com/privacy/cookie-policy#how-do-i-manage-trackers">Your Ad Choices</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://www.nytimes.com/privacy/privacy-policy">Privacy Policy</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://help.nytimes.com/hc/en-us/articles/115014893428-Terms-of-service">Terms of Service</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://help.nytimes.com/hc/en-us/articles/115014893968-Terms-of-sale">Terms of Sale</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="/sitemap/">Site Map</a></li><li class="mobileOnly css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://www.nytimes.com/ca/">Canada</a></li><li class="mobileOnly css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://www.nytimes.com/international/">International</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://help.nytimes.com/hc/en-us">Help</a></li><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="footer-link" href="https://www.nytimes.com/subscription?campaignId=37WXW" rel="nofollow">Subscriptions</a></li></ul><ul class="css-t8x4fj edvi3so1"><li class="css-a7htku edvi3so2"><a class="css-13mf4b4" data-testid="privacy-preferences-link" href="/privacy/manage-settings" rel="noreferrer noopener" target="_blank">Manage Privacy Preferences</a></li></ul></nav></footer></div></div></div>
<script>window.__preloadedData = {"initialData":{"data":{"home":{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNodeEmbedded","children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"UnstructuredBlock"},{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"CapsuleBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"InteractiveBlock"},{"__typename":"Heading2Block"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Jonathan Swan","id":"UGVyc29uOm55dDovL3BlcnNvbi8wYWMzOTMzMS1kZTBkLTU0NjItODhlZi05NjZmOWM4NmQ3Yzg=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F02\u002F22\u002Freader-center\u002Fauthor-jonathan-swan\u002Fauthor-jonathan-swan-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjlkZDhhM2MtNzkwZi01MzBkLTk3YTQtMmNkZDg1MjNkYWZl"}},{"__typename":"Person","displayName":"Theodore Schleifer","id":"UGVyc29uOm55dDovL3BlcnNvbi9kMWUyNDQ5Yi04YTY5LTUzMDUtODYzYi02MzYwMjk1ZmRiZGQ=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F06\u002F04\u002Freader-center\u002Fauthor-teddy-schleifer\u002Fauthor-teddy-schleifer-thumbLarge-v3.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjFiOTAzZGMtNjdlMC01MWYyLTk3NmItZGIzNTdmZGJmMTU4"}},{"__typename":"Person","displayName":"Maggie Haberman","id":"UGVyc29uOm55dDovL3BlcnNvbi81M2U0ZmU5OC0wNzBjLTU3ZTYtOWEzMy1lZmY4MmViMTkwZjk=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F07\u002F12\u002Fmultimedia\u002Fauthor-maggie-haberman\u002Fauthor-maggie-haberman-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTE2MjI2OTYtYWRhMC01MjRmLTk0Y2ItOWEwMGRjNzI5YTNj"}},{"__typename":"Person","displayName":"Ryan Mac","id":"UGVyc29uOm55dDovL3BlcnNvbi85YjQyNDIzZi1mNTk5LTVhM2YtOTYyNi02ZGQ5ODUzZGM0Y2I=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F09\u002F10\u002Freader-center\u002Fauthor-ryan-mac\u002Fauthor-ryan-mac-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjQzODQ1OTEtYTlhYi01ZjU1LTk0NmUtMTgxODI5NTIzYzEz"}},{"__typename":"Person","displayName":"Kate Conger","id":"UGVyc29uOm55dDovL3BlcnNvbi82MWM0ZDBkOS1iNTA2LTU1NzAtOTQ1Zi03N2Q0OGMwMzgyYjE=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-thumbLarge-v5.jpg","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjYxN2EzZjctNTkwMC01ZWRmLWE1ZmUtOGM4MmVmMTc4YzNm"}},{"__typename":"Person","displayName":"Nicholas Nehamas","id":"UGVyc29uOm55dDovL3BlcnNvbi80MTA2NjZjZi0zZmFkLTU4NWQtYTc5ZS1kNmNiYzdlNmIyYzc=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F04\u002F21\u002Freader-center\u002Fauthor-nicholas-nehamas\u002Fauthor-nicholas-nehamas-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMzE5YjI1NDctYTRlMS01MTQzLTg2NDctZGU1NTcyNDQ4Nzgw"}},{"__typename":"Person","displayName":"Madeleine Ngo","id":"UGVyc29uOm55dDovL3BlcnNvbi84ZjkwNTVlZC0yNTNjLTUxNDMtOTg0MC1hMWFkNTAyYzI5Y2M=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F09\u002F30\u002Fus\u002Fpolitics\u002Fauthor-madeleine-ngo\u002Fauthor-madeleine-ngo-thumbLarge-v3.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNGQyMDBkZGUtYjdhYi01MmY0LWJjYmMtODgyMmY0YzA3ZjZi"}}],"renderedRepresentation":"By Jonathan Swan, Theodore Schleifer, Maggie Haberman, Ryan Mac, Kate Conger, Nicholas Nehamas and Madeleine Ngo"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-95773dee-1b8a-5f08-b78a-29ed1903e90c\u002Fjob-1740736971771\u002Farticle-95773dee-1b8a-5f08-b78a-29ed1903e90c-job-1740736971771.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for How Elon Musk Executed His Takeover of the Federal Bureaucracy"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vMjZmOTRkZWQtOTE5MC01NjZmLTg1YmMtNzViZjUyMGUxYTEy","length":1708,"sourceId":"100000010019031","uri":"nyt:\u002F\u002Faudio\u002F26f94ded-9190-566f-85bc-75bf520e1a12"}},"firstPublished":"2025-02-28T10:02:50.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"How Elon Musk Executed His Takeover of the Federal Bureaucracy"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzk1NzczZGVlLTFiOGEtNWYwOC1iNzhhLTI5ZWQxOTAzZTkwYw==","lastModified":"2025-02-28T11:37:15.494Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-promo-square640-v2.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-promo-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvY2Y0MGM2NzUtNWQ5Yi01NjUyLTljY2EtZGViN2YxOTA5NTAy"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010012063","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F95773dee-1b8a-5f08-b78a-29ed1903e90c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-federal-bureaucracy-takeover.html","wordCount":4671},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"CapsuleBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"InteractiveBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"The New York Times","id":"UGVyc29uOm55dDovL3BlcnNvbi8wZjFhNWIxOC03ZTM4LTU5ZmUtODEzMi1iNTAxNWNkMzcxNDg=","promotionalMedia":null}],"renderedRepresentation":"By The New York Times"}],"featuredAudio":null,"firstPublished":"2025-02-28T10:04:16.382Z","headline":{"__typename":"CreativeWorkHeadline","default":"How Musk Built DOGE: Timeline and Key Takeaways"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzg5YzEzZWRmLTJjY2ItNTIyMy05MWM3LWE5NmIyZTdiMDk5OA==","lastModified":"2025-02-28T10:04:16.382Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjQ4YmI3YjUtYjZjNC01NDVjLWJhYzktMDBlZWVmMDNiNDhi"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010016948","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F89c13edf-2ccb-5223-91c7-a96b2e7b0998","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-doge-timeline-takeaways.html","wordCount":490}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:37:15.494Z","uri":"nyt:\u002F\u002Farticle\u002F95773dee-1b8a-5f08-b78a-29ed1903e90c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-federal-bureaucracy-takeover.html"},{"__typename":"Article","lastModified":"2025-02-28T10:04:16.382Z","uri":"nyt:\u002F\u002Farticle\u002F89c13edf-2ccb-5223-91c7-a96b2e7b0998","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-doge-timeline-takeaways.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F6bbdb3077ab64b69b4b5cbf86dad2a61","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Mr. Musk now finds himself spearheading a radical takeover of the federal bureaucracy, empowered by President Trump in ways that have no historical parallel for an unelected adviser."},"captionOverride":null,"credit":"Eric Lee\u002FThe New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-promo-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-promo-square640-v2.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-promo-mediumSquareAt3X-v2.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-promo-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-promo-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-header1-tgvm-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F25\u002Fmultimedia\u002Fdc-doge-origins-promo\u002Fdc-doge-origins-promo-verticalTwoByThree735-v2.jpg","width":735}]}],"firstPublished":"2025-02-27T22:11:03.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvY2Y0MGM2NzUtNWQ5Yi01NjUyLTljY2EtZGViN2YxOTA5NTAy","lastModified":"2025-02-28T09:35:47.324Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017855","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fcf40c675-5d9b-5652-9cca-deb7f1909502","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002Fdc-doge-origins-promo.html"},{"__typename":"CardDeck","asset":{"__typename":"ReporterThread","cards":[{"__typename":"ReporterThreadCard","bylines":[{"__typename":"BylineKV","byline":{"__typename":"Byline","creators":[{"__typename":"PersonSnapshot","displayName":"Kate Conger","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":75,"name":"thumbStandard","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-thumbStandard-v5.jpg","width":75},{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-thumbLarge-v5.jpg","width":150}]},{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":489,"name":"mobileMasterAt3x","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-mobileMasterAt3x-v5.jpg","width":489}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjYxN2EzZjctNTkwMC01ZWRmLWE1ZmUtOGM4MmVmMTc4YzNm"}}],"prefix":"By"},"key":"byline"}],"data":"{}","key":"1873857.1960980943","text":[{"__typename":"TextRunKV","content":[{"__typename":"TextInline","text":"It started as banter at a fund-raising dinner for Vivek Ramaswamy’s presidential campaign in 2023. Elon Musk indicated to guests that he believed that technology was the key to gutting the federal bureaucracy →"}],"key":"body"}]},{"__typename":"ReporterThreadCard","bylines":[{"__typename":"BylineKV","byline":{"__typename":"Byline","creators":[{"__typename":"PersonSnapshot","displayName":"Nicholas Nehamas","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":75,"name":"thumbStandard","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F04\u002F21\u002Freader-center\u002Fauthor-nicholas-nehamas\u002Fauthor-nicholas-nehamas-thumbStandard.png","width":75},{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F04\u002F21\u002Freader-center\u002Fauthor-nicholas-nehamas\u002Fauthor-nicholas-nehamas-thumbLarge.png","width":150}]},{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":1000,"name":"mobileMasterAt3x","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F04\u002F21\u002Freader-center\u002Fauthor-nicholas-nehamas\u002Fauthor-nicholas-nehamas-mobileMasterAt3x.png","width":1000}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMzE5YjI1NDctYTRlMS01MTQzLTg2NDctZGU1NTcyNDQ4Nzgw"}}],"prefix":"By"},"key":"byline"}],"data":"{}","key":"6377542.584128635","text":[{"__typename":"TextRunKV","content":[{"__typename":"TextInline","text":"Musk’s interest in reshaping the government grew after he endorsed Donald J. Trump, pouring millions into his campaign. After Trump won, seasoned conservative operatives helped educate Musk about the executive branch."}],"key":"body"}]},{"__typename":"ReporterThreadCard","bylines":[{"__typename":"BylineKV","byline":{"__typename":"Byline","creators":[{"__typename":"PersonSnapshot","displayName":"Kate Conger","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":75,"name":"thumbStandard","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-thumbStandard-v5.jpg","width":75},{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-thumbLarge-v5.jpg","width":150}]},{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":489,"name":"mobileMasterAt3x","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-mobileMasterAt3x-v5.jpg","width":489}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjYxN2EzZjctNTkwMC01ZWRmLWE1ZmUtOGM4MmVmMTc4YzNm"}}],"prefix":"By"},"key":"byline"}],"data":"{}","key":"1962504.0820107586","text":[{"__typename":"TextRunKV","content":[{"__typename":"TextInline","text":"Trump said that the Department of Government Efficiency, or DOGE, would operate outside the government. But Musk’s team wanted to get inside the bureaucracy and decided to take over an existing White House digital services office."}],"key":"body"}]},{"__typename":"ReporterThreadCard","bylines":[{"__typename":"BylineKV","byline":{"__typename":"Byline","creators":[{"__typename":"PersonSnapshot","displayName":"Nicholas Nehamas","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":75,"name":"thumbStandard","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F04\u002F21\u002Freader-center\u002Fauthor-nicholas-nehamas\u002Fauthor-nicholas-nehamas-thumbStandard.png","width":75},{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F04\u002F21\u002Freader-center\u002Fauthor-nicholas-nehamas\u002Fauthor-nicholas-nehamas-thumbLarge.png","width":150}]},{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":1000,"name":"mobileMasterAt3x","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F04\u002F21\u002Freader-center\u002Fauthor-nicholas-nehamas\u002Fauthor-nicholas-nehamas-mobileMasterAt3x.png","width":1000}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMzE5YjI1NDctYTRlMS01MTQzLTg2NDctZGU1NTcyNDQ4Nzgw"}}],"prefix":"By"},"key":"byline"}],"data":"{}","key":"8598760.920657681","text":[{"__typename":"TextRunKV","content":[{"__typename":"TextInline","text":"The Trump transition had an ally in the digital unit: Amy Gleason, a career employee who rejoined it late last year. She referred several job applicants who were later hired by DOGE after the inauguration."}],"key":"body"}]},{"__typename":"ReporterThreadCard","bylines":[{"__typename":"BylineKV","byline":{"__typename":"Byline","creators":[{"__typename":"PersonSnapshot","displayName":"Kate Conger","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":75,"name":"thumbStandard","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-thumbStandard-v5.jpg","width":75},{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-thumbLarge-v5.jpg","width":150}]},{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","height":489,"name":"mobileMasterAt3x","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F02\u002F26\u002Fbusiness\u002Fauthor-kate-conger\u002Fauthor-kate-conger-mobileMasterAt3x-v5.jpg","width":489}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjYxN2EzZjctNTkwMC01ZWRmLWE1ZmUtOGM4MmVmMTc4YzNm"}}],"prefix":"By"},"key":"byline"}],"data":"{}","key":"1525946.9699402817","text":[{"__typename":"TextRunKV","content":[{"__typename":"TextInline","text":"After Trump was sworn in, Musk’s team swiftly inserted itself into more than 20 agencies, pushing out career employees and freezing grants. In his White House office, Musk installed a gaming computer and put a sign on his desk that read “DOGE.”"}],"key":"body"}]}],"data":"{\"uppercase\":false,\"kickerURL\":\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-federal-bureaucracy-takeover.html\"}"},"bylines":[],"firstPublished":"2025-02-28T10:01:02.996Z","id":"Q2FyZERlY2s6bnl0Oi8vY2FyZGRlY2svYmRjZDc2YjEtMGMwZS00MTk2LTg5YTYtNTJkMTkzMjAyOTI1","lastModified":"2025-02-28T11:13:08.851Z","promotionalImage":null,"section":null,"slug":"dc-doge-origins-thread","sourceId":"bdcd76b1-0c0e-4196-89a6-52d193202925","tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fcarddeck\u002Fbdcd76b1-0c0e-4196-89a6-52d193202925","url":""},{"__typename":"Image","altText":"Elon Musk standing in the background during a meeting with cabinet officials, including Russell Vought, Robert F. Kennedy Jr. and Doug Burgum, and President Trump.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Doug Mills\u002FThe New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz\u002F28dc-doge-origins-takeaways-hpqz-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T10:04:14.292Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjQ4YmI3YjUtYjZjNC01NDVjLWJhYzktMDBlZWVmMDNiNDhi","lastModified":"2025-02-28T10:04:14.292Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017391","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fb48bb7b5-b6c4-545c-bac9-00eeef03b48b","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28dc-doge-origins-takeaways-hpqz.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fcf40c675-5d9b-5652-9cca-deb7f1909502\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":\"nyt:\u002F\u002Fcarddeck\u002Fbdcd76b1-0c0e-4196-89a6-52d193202925\",\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"How Elon Musk Executed His Takeover of the Federal Bureaucracy\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The operation was driven with a frenetic focus by the billionaire, who channeled his resentment of regulatory oversight into a government overhaul.\",\"thread\":null,\"sentence\":\"How Elon Musk Executed His Takeover of the Federal Bureaucracy\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F95773dee-1b8a-5f08-b78a-29ed1903e90c\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fb48bb7b5-b6c4-545c-bac9-00eeef03b48b\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SENTENCE\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"How Musk Built DOGE: Timeline and Key Takeaways\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The New York Times interviewed more than 60 people familiar with Mr. Musk’s effort to piece together new details about it.\",\"thread\":null,\"sentence\":\"The Building of DOGE: A Timeline and Key Takeaways\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F89c13edf-2ccb-5223-91c7-a96b2e7b0998\"}]}","sourceId":"1f7476f2-79c8-4468-87ca-e98233a57ec5","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fb2ef26af-8177-599a-b6fc-a4a98c32f270","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderMultimediaBlock","media":{"__typename":"AudioBlock","media":{"__typename":"Audio","fileUrl":"https:\u002F\u002Fnyt.simplecastaudio.com\u002F6be9f004-3e81-4fb7-9ff7-a70c01563962\u002Fepisodes\u002F35f6f7cb-b73d-46a8-b758-2e3ebc8937de\u002Faudio\u002F128\u002Fdefault.mp3?awCollectionId=6be9f004-3e81-4fb7-9ff7-a70c01563962&awEpisodeId=35f6f7cb-b73d-46a8-b758-2e3ebc8937de&nocache","headline":{"__typename":"CreativeWorkHeadline","default":"The Headlines"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vYmQ3MzFkMGYtMTZhNy01ZGUyLTgyZTYtOTBkNDViZDRhMDEw","length":556,"podcastSeries":{"__typename":"AudioPodcastSeries","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F10\u002F12\u002Fpodcasts\u002Fheadlines-albumartwork-audioapp-2\u002Fheadlines-albumartwork-audioapp-2-square640-v2.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTNiYTgzOGEtNTRhZC01ODljLTgxZDQtNzc3NTRkYTZjYzVi"},"title":"The Headlines"},"promotionalImage":null,"sourceId":"100000010008921","uri":"nyt:\u002F\u002Faudio\u002Fbd731d0f-16a7-5de2-82e6-90d45bd4a010"}}},{"__typename":"Heading3Block"},{"__typename":"RuleBlock"},{"__typename":"BylineBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ListBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RuleBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Tracy Mumford","id":"UGVyc29uOm55dDovL3BlcnNvbi8wMWRiMGI2NC0wNTM0LTVkYmItODU5Ni1mYjAwOWQ3OGRkZjI=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F08\u002F06\u002Freader-center\u002Fauthor-tracy-mumford\u002Fauthor-tracy-mumford-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMTczMGUzY2ItYWY4NS01ODdhLThkMTctODlhZDQxMGRjNjMy"}},{"__typename":"Person","displayName":"Will Jarvis","id":"UGVyc29uOm55dDovL3BlcnNvbi80NzllMjRjNy1iYTZjLTVjM2UtOTNlYi0zNzgyODdkZTIwN2Q=","promotionalMedia":null},{"__typename":"Person","displayName":"Ian Stewart","id":"UGVyc29uOm55dDovL3BlcnNvbi9jZWM5MDVhZS02YTY3LTVmYzAtYWE3Ni1jMWFlNTAyNWNlYWI=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F12\u002F05\u002Freader-center\u002Fauthor-ian-stewart\u002Fauthor-ian-stewart-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvM2Q1YjllZmItYWJkZC01MTFmLTk0MDktZmJkY2IxNDRhZjIz"}},{"__typename":"Person","displayName":"Jessica Metzger","id":"UGVyc29uOm55dDovL3BlcnNvbi9mYWVkM2FjNi04NjU3LTUzYjUtODAzMS01YWE1NWEwNTVkZjk=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F05\u002F29\u002Freader-center\u002Fauthor-jessica-metzger\u002Fauthor-jessica-metzger-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNmM2NzYyODQtOTBmMC01Yjg4LWFkNjktM2VjNzkxOWRiNmU3"}},{"__typename":"Person","displayName":"Raja Abdulrahim","id":"UGVyc29uOm55dDovL3BlcnNvbi82YTMxYjMzNy0yNjE4LTVjZTMtOTZhZi03ZDIzZTVhY2UyNDg=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F04\u002F06\u002Freader-center\u002Fauthor-raja-abdulrahim\u002Fauthor-raja-abdulrahim-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMmRjMzMyYTYtMDA1YS01ZjhiLWFhM2EtNjFkYmY1YjczODA2"}}],"renderedRepresentation":"By Tracy Mumford, Will Jarvis, Ian Stewart, Jessica Metzger and Raja Abdulrahim"}],"featuredAudio":null,"firstPublished":"2025-02-28T11:00:09.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Iowa Moves to Eliminate Trans Rights, and Zelensky Heads to the White House"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzhiMjAwNjM2LThkZjUtNWNhYS04ZGFhLTMwOGVmZWEyODdiMA==","lastModified":"2025-02-28T11:20:12.055Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-threeByTwoLargeAt2X-v2.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvODUzYmMxZGEtMjg5ZC01NGU5LWFmYmYtMDUyNzBkN2JmYzBh"}},"section":{"__typename":"Section","displayName":"Podcasts","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzExMDc5OTg3LWFlOGQtNWY1Zi04YzJlLThjNjZkMzM3ZDJiYQ=="},"sourceId":"100000010008969","tone":"FEATURE","uri":"nyt:\u002F\u002Farticle\u002F8b200636-8df5-5caa-8daa-308efea287b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-headlines\u002Fiowa-trans-rights-zelensky-trump.html","wordCount":159}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:20:12.055Z","uri":"nyt:\u002F\u002Farticle\u002F8b200636-8df5-5caa-8daa-308efea287b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-headlines\u002Fiowa-trans-rights-zelensky-trump.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Ffce5d264465448fd804b496107b7be24","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"Image","altText":"People gather to protest an Iowa bill to strip civil rights protections from transgender people at the Iowa Capitol in Des Moines.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Advocates for L.G.B.T.Q. rights said Iowa would become the first state to remove broad protections for transgender people if the governor signed the bill."},"captionOverride":null,"credit":"Rachel Mummey for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-square640.jpg","width":640},{"__typename":"ImageRendition","height":1801,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-threeByTwoMediumAt2X-v2.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-threeByTwoSmallAt2X-v2.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq\u002F28theheadlines-app-header-mvjq-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T08:42:52.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvODUzYmMxZGEtMjg5ZC01NGU5LWFmYmYtMDUyNzBkN2JmYzBh","lastModified":"2025-02-28T09:30:28.694Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010018665","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F853bc1da-289d-54e9-afbf-05270d7bfc0a","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28theheadlines-app-header-mvjq.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F853bc1da-289d-54e9-afbf-05270d7bfc0a\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Iowa Moves to Eliminate Trans Rights, Volodymyr Zelensky Heads to the White House, and More\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Plus, Conan O’Brien on hosting the Oscars.\",\"thread\":null,\"sentence\":\"Iowa Moves to Eliminate Trans Rights, and Zelensky Heads to the White House\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F8b200636-8df5-5caa-8daa-308efea287b0\"}]}","sourceId":"97125bb4-de95-4925-97c7-d6be04fc2dc9","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F01ae2935-20f3-5dc3-8d2a-ed4757b7a55a","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"LegacyCollection","active":true,"assets":{"__typename":"AssetsConnection","edges":[{"__typename":"AssetsEdge","node":{"__typename":"Article","firstPublished":"2025-02-28T10:04:30.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"After Insults and False Claims, Trump to Host Zelensky for Minerals Deal","sentence":"After insults and false claims, Trump will host Zelensky."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzJlZTFkOTFiLTMyMGYtNTNlNy04MTdmLWY5OTI1ZjJjNjNlZQ==","lastModified":"2025-02-28T13:00:42.851Z","sourceId":"100000010017974","uri":"nyt:\u002F\u002Farticle\u002F2ee1d91b-320f-53e7-817f-f9925f2c63ee","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Ftrump-zelensky-ukraine-russia-putin.html"}},{"__typename":"AssetsEdge","node":{"__typename":"Article","firstPublished":"2025-02-28T02:54:46.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Judge Says Trump Administration Memos Directing Mass Firings Were Illegal","sentence":"A federal judge says Trump administration memos directing mass firings were illegal."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2QzMmFiNDE0LTE5NzItNTBmZS04YzBhLTIwODMwMmY5NzJiMA==","lastModified":"2025-02-28T13:00:42.943Z","sourceId":"100000010017975","uri":"nyt:\u002F\u002Farticle\u002Fd32ab414-1972-50fe-8c0a-208302f972b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ffederal-layoffs-trump-opm.html"}},{"__typename":"AssetsEdge","node":{"__typename":"Article","firstPublished":"2025-02-28T02:26:03.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Office Closures and Relocations Part of Trump’s Plan for Large-Scale Layoffs","sentence":"Office closures and relocations are part of Trump’s layoff plan."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzlhNDFjZTc3LTAzNDMtNWFlMi04MWM4LTU2Y2I1Y2IwODFjYg==","lastModified":"2025-02-28T13:00:43.134Z","sourceId":"100000010016329","uri":"nyt:\u002F\u002Farticle\u002F9a41ce77-0343-5ae2-81c8-56cb5cb081cb","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrump-federal-worker-layoffs.html"}},{"__typename":"AssetsEdge","node":{"__typename":"Article","firstPublished":"2025-02-27T23:44:22.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Mass Layoffs Begin at NOAA, With Hundreds Said to Be Fired in One Day","sentence":"Mass layoffs begin at NOAA, with hundreds said to be fired."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2RmNTFlY2E3LWI5YTYtNTM5Zi04NjVlLWU3ZjNmMjMyZTg5NA==","lastModified":"2025-02-28T13:00:43.439Z","sourceId":"100000010017686","uri":"nyt:\u002F\u002Farticle\u002Fdf51eca7-b9a6-539f-865e-e7f3f232e894","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fclimate\u002Fnoaa-layoffs-trump.html"}},{"__typename":"AssetsEdge","node":{"__typename":"Article","firstPublished":"2025-02-27T23:08:48.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"With First Weekly Call, Patel Begins Serious Business of Running F.B.I.","sentence":"With his first weekly call, Patel begins the serious business of running the F.B.I."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzAwZDM2YTk3LWM5NDAtNWE2ZC05NjRiLWZlOGFiMDQ2ZWVjNA==","lastModified":"2025-02-28T13:00:43.115Z","sourceId":"100000010017418","uri":"nyt:\u002F\u002Farticle\u002F00d36a97-c940-5a6d-964b-fe8ab046eec4","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fkash-patel-fbi-director-ufc.html"}},{"__typename":"AssetsEdge","node":{"__typename":"Article","firstPublished":"2025-02-27T21:45:05.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Joe Gebbia, a billionaire on Musk’s team, reveals his role.","sentence":""},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzdhZmUyYzFiLTA3MjAtNWZjMS04OTcwLTQ0NTc4NzFmYjUxZg==","lastModified":"2025-02-28T13:00:43.109Z","sourceId":"100000010016936","uri":"nyt:\u002F\u002Farticle\u002F7afe2c1b-0720-5fc1-8970-4457871fb51f","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F28\u002Fus\u002Ftrump-news\u002Fjoe-gebbia-airbnb-trump-elon-musk-doge"}},{"__typename":"AssetsEdge","node":{"__typename":"Article","firstPublished":"2025-02-27T18:57:06.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Trump Says He Believes Putin Would Abide by Any Ukraine Peace Deal","sentence":"Hosting Britain’s leader, Trump brushes aside worries about Putin."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzNhYjEzZWQ2LThiZmMtNTYxYS05MjZlLTEzYmMxOGRmNzQ5NQ==","lastModified":"2025-02-28T13:00:43.670Z","sourceId":"100000010017108","uri":"nyt:\u002F\u002Farticle\u002F3ab13ed6-8bfc-561a-926e-13bc18df7495","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fstarmer-ukraine-trump.html"}},{"__typename":"AssetsEdge","node":{"__typename":"Article","firstPublished":"2025-02-27T18:49:12.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"U.S. Canceled Work to Contain a Serious Ebola Outbreak","sentence":"Musk said funding to fight Ebola was paused ‘very briefly.’ Much of it was canceled, officials said."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2Y5MzFlNDEzLTRiYmUtNWZhMC1hMjdiLTAyOGVlMzlhMThmMg==","lastModified":"2025-02-28T13:00:43.196Z","sourceId":"100000010016774","uri":"nyt:\u002F\u002Farticle\u002Ff931e413-4bbe-5fa0-a27b-028ee39a18f2","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fhealth\u002Fmusk-ebola-funding.html"}}]},"bylines":[],"eventId":"pubp:\u002F\u002Fevent\u002Fede885bd568840aa9e5b4c83d749d913","firstPublished":"2025-02-28T13:00:38.000Z","groupings":[{"__typename":"LegacyCollectionGrouping","containers":[{"__typename":"LegacyCollectionContainer","label":"highlights","name":"highlights","relations":[]},{"__typename":"LegacyCollectionContainer","label":"promos","name":"promos","relations":[]},{"__typename":"LegacyCollectionContainer","label":"footer","name":"footer","relations":[]},{"__typename":"LegacyCollectionContainer","label":"feed lede","name":"feed lede","relations":[{"__typename":"LegacyCollectionRelation","asset":{"__typename":"Capsule"}}]},{"__typename":"LegacyCollectionContainer","label":"additional content","name":"additional content","relations":[{"__typename":"LegacyCollectionRelation","asset":{"__typename":"LegacyCollection"}}]}]}],"id":"TGVnYWN5Q29sbGVjdGlvbjpueXQ6Ly9sZWdhY3ljb2xsZWN0aW9uLzQ1MmIxZDM3LTdiNDUtNWYzMy04NmY5LTIzYmEyMjQ0ZDZiMw==","lastModified":"2025-02-28T13:10:16.130Z","name":"28trump-news","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-live-zelensky-gqfm-promo\u002F28trump-live-zelensky-gqfm-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-live-zelensky-gqfm-promo\u002F28trump-live-zelensky-gqfm-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjM1NzZjYTktODlkNi01YWQzLWI1NzEtMmJmOTBlNzAxMDA0"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010017638","tone":"NEWS","unstructuredData":"{\"sectionPromoId\":100000010017639,\"typeOfBlog\":\"BLOG\",\"supportsFirestore\":{\"removedUpdates\":true,\"labels\":true},\"topicLabel\":\"\",\"messagingConfig\":{\"push\":{\"method\":\"MEMO\",\"segment\":\"22trump-news-0363\",\"enabled\":true}},\"newsEvent\":\"Trump Administration News\"}","uri":"nyt:\u002F\u002Flegacycollection\u002F452b1d37-7b45-5f33-86f9-23ba2244d6b3","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F28\u002Fus\u002Ftrump-news"},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Mark Landler","id":"UGVyc29uOm55dDovL3BlcnNvbi8zMDA4MmFjNi0wMmQyLTViYTYtYTcwZC1iNjgzZjE3NWJhNTU=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2019\u002F10\u002F22\u002Freader-center\u002Fauthor-mark-landler\u002Fauthor-mark-landler-thumbLarge-v7.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMmJmZGNkYWUtNWMwZS01MWFiLWEwZGUtMTcxM2JkMTNjMjY1"}}],"renderedRepresentation":"By Mark Landler"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-e5314031-2e3d-5d39-83d9-210e3f501e95\u002Fjob-1740744183323\u002Farticle-e5314031-2e3d-5d39-83d9-210e3f501e95-job-1740744183323.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for With a Letter From King Charles, Starmer Was Welcomed Into Trump’s Court"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vYmU4NDBiZTItMmNhZS01NTQxLTllOTUtNjYxYjVhODU1YTUz","length":427,"sourceId":"100000010019123","uri":"nyt:\u002F\u002Faudio\u002Fbe840be2-2cae-5541-9e95-661b5a855a53"}},"firstPublished":"2025-02-28T12:02:45.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"With a Letter From King Charles, Starmer Was Welcomed Into Trump’s Court"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2U1MzE0MDMxLTJlM2QtNWQzOS04M2Q5LTIxMGUzZjUwMWU5NQ==","lastModified":"2025-02-28T12:13:45.314Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTExOTk5NTctNDBmOS01ZTg0LTlkNGItODVjNTg4ZGJjYjVk"}},"section":{"__typename":"Section","displayName":"World","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzcwZTg2NWI2LWNjNzAtNTE4MS04NGM5LTgzNjhiM2E1YzM0Yg=="},"sourceId":"100000010018994","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Fe5314031-2e3d-5d39-83d9-210e3f501e95","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Feurope\u002Ftrump-starmer-king-charles.html","wordCount":1213},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Michael D. Shear","id":"UGVyc29uOm55dDovL3BlcnNvbi8yN2I0YzE4NC1iYWU5LTUyMmYtYjUwZS03NjBjOWYzMWE5Yzk=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F06\u002F13\u002Fmultimedia\u002Fauthor-michael-d-shear\u002Fauthor-michael-d-shear-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNzY4YzBhOTMtZTBiYS01MmRmLWIyNGMtYjE4YTU0MDgzODdj"}},{"__typename":"Person","displayName":"Shawn McCreesh","id":"UGVyc29uOm55dDovL3BlcnNvbi9lZTViMmQxNy00MjNlLTU4YjctOGU2Ny05ZjY0ZjFmN2RlNjI=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2019\u002F12\u002F13\u002Freader-center\u002Fauthor-shawn-mccreesh\u002Fauthor-shawn-mccreesh-thumbLarge-v3.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvY2E1YTUwYWItMDYwMS01NTlhLThhM2QtZDJhMDcxZTBkZjc1"}}],"renderedRepresentation":"By Michael D. Shear and Shawn McCreesh"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-3ab13ed6-8bfc-561a-926e-13bc18df7495\u002Fjob-1740703591694\u002Farticle-3ab13ed6-8bfc-561a-926e-13bc18df7495-job-1740703591694.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for British Leader Comes With a Plea and a Promise for Trump Over Ukraine"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vOWZkN2VmZWMtZGVlNi01YmJmLWJmZDktNjdiZjIzNmE2YjM3","length":294,"sourceId":"100000010017230","uri":"nyt:\u002F\u002Faudio\u002F9fd7efec-dee6-5bbf-bfd9-67bf236a6b37"}},"firstPublished":"2025-02-27T18:57:06.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Trump Says He Believes Putin Would Abide by Any Ukraine Peace Deal"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzNhYjEzZWQ2LThiZmMtNTYxYS05MjZlLTEzYmMxOGRmNzQ5NQ==","lastModified":"2025-02-28T13:00:43.670Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTBiYjgxNjQtZDU3ZS01MDc4LWJhOTUtYTBjMTI4ZGRmM2Mz"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010017108","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F3ab13ed6-8bfc-561a-926e-13bc18df7495","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fstarmer-ukraine-trump.html","wordCount":821}],"children":[],"descendantAssets":[{"__typename":"LegacyCollection","lastModified":"2025-02-28T13:10:16.130Z","uri":"nyt:\u002F\u002Flegacycollection\u002F452b1d37-7b45-5f33-86f9-23ba2244d6b3","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F28\u002Fus\u002Ftrump-news"},{"__typename":"Article","lastModified":"2025-02-28T12:13:45.314Z","uri":"nyt:\u002F\u002Farticle\u002Fe5314031-2e3d-5d39-83d9-210e3f501e95","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Feurope\u002Ftrump-starmer-king-charles.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:43.670Z","uri":"nyt:\u002F\u002Farticle\u002F3ab13ed6-8bfc-561a-926e-13bc18df7495","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fstarmer-ukraine-trump.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fe512852a2bd84ff3a7e7be8773b64ca2","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"EmbeddedInteractive","bylines":[],"compatibility":"INLINE","credit":"","displayProperties":{"__typename":"CreativeWorkDisplayProperties","displayOverrides":["DARK_MODE_COMPATIBLE"]},"firstPublished":"2025-02-26T21:55:00.000Z","html":"\u003Cnav aria-labelledby=\"styln-us-ukraine-minerals-hp-menu\" class=\"css-qvlk0g\"\u003E\u003Cp id=\"styln-us-ukraine-minerals-hp-menu\"\u003E\u003Cspan class=\"css-14sajpe\"\u003EU.S.-Ukraine Mineral Deal\u003C\u002Fspan\u003E\u003C\u002Fp\u003E\u003Cul class=\"css-11xr2bo\"\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002Farticle\u002Fukraine-mineral-deal-trump.html\"\u003EWhat to Know\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F12\u002Fworld\u002Feurope\u002Ftrump-ukraine-rare-earth-minerals.html\"\u003EHow the Deal Began\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F25\u002Fworld\u002Feurope\u002Ftrump-us-ukraine-mineral-deal.html\"\u003E‘Protection Racket’ Diplomacy\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F26\u002Fus\u002Fpolitics\u002Ftrump-biden-minerals-ukraine.html\"\u003EU.S.’s Core Focus on Minerals\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003C\u002Ful\u003E\u003Cscript\u003E\"use strict\";(function(){\u002F\u002F prevent menus from overflowing into right rail on desktop\nfunction a(){var a=document.querySelector(\"nav[aria-labelledby=\\\"styln-us-ukraine-minerals-hp-menu\\\"]\"),b=document.querySelectorAll(\"[data-hierarchy=\\\"zone\\\"]\"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth\u003Ec.clientWidth)\u002F\u002F remove the last item from the menu if it is overflowing\n{var d=a.querySelectorAll(\"li\"),e=d[d.length-1];e.remove()}}\u002F\u002F only check for overflow if the user is on desktop or tablet\n\u002F\u002F remove links that match ones already shown in the live band\nvar b=document.querySelector(\"#hp-live-band-list\");if(b){var c=Array.from(b.querySelectorAll(\"a\")).map(function(b){return b.href}),d=document.querySelectorAll(\"nav[aria-labelledby=\\\"styln-us-ukraine-minerals-hp-menu\\\"] li\");d.forEach(function(a){var b,d=null===(b=a.querySelector(\"a\"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia(\"(pointer: coarse) and (max-width: 739px)\").matches){a();var e;window.addEventListener(\"resize\",function(){clearTimeout(e),e=setTimeout(a,250)})}})();\u003C\u002Fscript\u003E\u003C\u002Fnav\u003E","id":"RW1iZWRkZWRJbnRlcmFjdGl2ZTpueXQ6Ly9lbWJlZGRlZGludGVyYWN0aXZlLzI5NGMzOGE2LTFhODMtNTczZi05YTg2LWM3MDRiNThhYzdjNA==","lastModified":"2025-02-27T03:44:16.968Z","promotionalImage":null,"promotionalMedia":null,"section":{"__typename":"Section","displayName":"Admin","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q0MmQzYTNmLTAyYTgtNWRlNy1hOTEyLTUyMGZhNmI0NGY0ZQ=="},"slug":"prism-styln-us-ukraine-minerals-homepageMenu-1740606871165","sourceId":"100000010015190","storyFormat":null,"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F294c38a6-1a83-573f-9a86-c704b58ac7c4","url":"null"},{"__typename":"CardDeck","asset":{"__typename":"Grid","cards":[{"__typename":"GridCard","assets":[{"__typename":"Image","altText":"","credit":"Anna Rose Layden for The New York Times","crops":[{"__typename":"ImageCrop","name":"MASTER","renditions":[{"__typename":"ImageRendition","height":900,"name":"articleLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-articleLarge-v2.jpg","width":600},{"__typename":"ImageRendition","height":888,"name":"tmagArticle","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-tmagArticle-v2.jpg","width":592},{"__typename":"ImageRendition","height":1024,"name":"jumbo","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-jumbo-v2.jpg","width":683},{"__typename":"ImageRendition","height":1575,"name":"master1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-master1050-v2.jpg","width":1050},{"__typename":"ImageRendition","height":1012,"name":"master675","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-master675-v2.jpg","width":675},{"__typename":"ImageRendition","height":1152,"name":"master768","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-master768-v2.jpg","width":768}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-square640.jpg","width":640}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-threeByTwoMediumAt2X.jpg","width":1500}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":338,"name":"videoSixteenByNine600","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-videoSixteenByNine600.jpg","width":600},{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-verticalTwoByThree735-v2.jpg","width":735}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjAzY2Y5ZjQtNTNlMi01ODI0LWFhOTEtNTdmZTg5ZWYyZWIy","sourceId":"100000010018655","uri":"nyt:\u002F\u002Fimage\u002Fb03cf9f4-53e2-5824-aa91-57fe89ef2eb2","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm.html"}],"data":"{\"credit\":\"Anna Rose Layden for The New York Times\",\"altText\":\"\",\"backgroundMedia\":\"nyt:\u002F\u002Fimage\u002Fb03cf9f4-53e2-5824-aa91-57fe89ef2eb2\"}","key":"3061790.3584565176","text":[]},{"__typename":"GridCard","assets":[{"__typename":"Image","altText":"","credit":"Eric Lee\u002FThe New York Times","crops":[{"__typename":"ImageCrop","name":"MASTER","renditions":[{"__typename":"ImageRendition","height":900,"name":"articleLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-articleLarge-v5.jpg","width":600},{"__typename":"ImageRendition","height":888,"name":"tmagArticle","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-tmagArticle-v5.jpg","width":592},{"__typename":"ImageRendition","height":1024,"name":"jumbo","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-jumbo-v5.jpg","width":683},{"__typename":"ImageRendition","height":1575,"name":"master1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-master1050-v5.jpg","width":1050},{"__typename":"ImageRendition","height":1013,"name":"master675","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-master675-v5.jpg","width":675},{"__typename":"ImageRendition","height":1152,"name":"master768","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-master768-v5.jpg","width":768}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-square640-v5.jpg","width":640}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-threeByTwoMediumAt2X.jpg","width":1500}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":338,"name":"videoSixteenByNine600","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-videoSixteenByNine600.jpg","width":600},{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk\u002F28trump-header-grid-3-bmwk-verticalTwoByThree735-v5.jpg","width":735}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjBlZDZiY2EtYjFmZC01YTY2LWEzZWYtOGU3MWFkMWZiZTRk","sourceId":"100000010018657","uri":"nyt:\u002F\u002Fimage\u002F20ed6bca-b1fd-5a66-a3ef-8e71ad1fbe4d","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-3-bmwk.html"}],"data":"{\"credit\":\"Eric Lee\u002FThe New York Times\",\"altText\":\"\",\"backgroundMedia\":\"nyt:\u002F\u002Fimage\u002F20ed6bca-b1fd-5a66-a3ef-8e71ad1fbe4d\"}","key":"4876769.520212882","text":[]}],"data":"{\"caption\":\"Anna Rose Layden for The New York Times; Eric Lee\u002FThe New York Times\"}"},"bylines":[],"firstPublished":"2025-02-28T08:21:51.431Z","id":"Q2FyZERlY2s6bnl0Oi8vY2FyZGRlY2svOGQzZjJhNDEtYTBkNS00NGVkLTk1MjktNjUwMDIyMGQ3Nzky","lastModified":"2025-02-28T08:22:34.291Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28trump-header-grid-1-kfvm\u002F28trump-header-grid-1-kfvm-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjAzY2Y5ZjQtNTNlMi01ODI0LWFhOTEtNTdmZTg5ZWYyZWIy"}},"section":null,"slug":"28trump-zelensky-header","sourceId":"8d3f2a41-a0d5-44ed-9529-6500220d7792","tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fcarddeck\u002F8d3f2a41-a0d5-44ed-9529-6500220d7792","url":""},{"__typename":"Video","aspectRatio":"3:2","autoplay":false,"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"The New York Times","id":"UGVyc29uOm55dDovL3BlcnNvbi8wZjFhNWIxOC03ZTM4LTU5ZmUtODEzMi1iNTAxNWNkMzcxNDg=","promotionalMedia":null}],"renderedRepresentation":"By The New York Times"}],"credits":[{"__typename":"Credit","creator":{"__typename":"Person","displayName":"The New York Times","id":"UGVyc29uOm55dDovL3BlcnNvbi8wZjFhNWIxOC03ZTM4LTU5ZmUtODEzMi1iNTAxNWNkMzcxNDg="},"role":"author"},{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Nader Ibrahim","id":"UGVyc29uOm55dDovL3BlcnNvbi9jMzcxYTg1ZC0zNzE5LTVjMDMtOWNmOC03ZDE0NTAxOWE3ZGI="},"role":"credited_producer"},{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Nader Ibrahim","id":"UGVyc29uOm55dDovL3BlcnNvbi9jMzcxYTg1ZC0zNzE5LTVjMDMtOWNmOC03ZDE0NTAxOWE3ZGI="},"role":"processing_producer"},{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Esther Bintliff","id":"UGVyc29uOm55dDovL3BlcnNvbi8wYzM0NzIxZi02MTViLTUyMDUtOTMyNS1lNWEyM2UzOTA3MjE="},"role":"senior_producer"}],"duration":74000,"firstPublished":"2025-02-28T12:02:44.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"President Trump Is Invited for a ‘Historic’ Second State Visit to U.K."},"id":"VmlkZW86bnl0Oi8vdmlkZW8vM2M5MzM4NTItYWUzMS01OTI3LThmYTctOTIwNmQxZWFlZjc0","isCinemagraph":false,"isLive":false,"lastModified":"2025-02-28T12:48:01.855Z","liveUrls":[],"promotionalHeadline":"President Trump Is Invited for a ‘Historic’ Second State Visit to U.K.","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTExOTk5NTctNDBmOS01ZTg0LTlkNGItODVjNTg4ZGJjYjVk"}},"promotionalMedia":{"__typename":"Image","altText":"Prime Minister Keir Starmer shaking hands with President Trump, standing in front of the flags of their countries and two lecterns.","caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Haiyun Jiang for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-square640.jpg","width":640},{"__typename":"ImageRendition","height":1799,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28uk-starmer-01-gthq\u002F28uk-starmer-01-gthq-verticalTwoByThree735.jpg","width":735}]}],"hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTExOTk5NTctNDBmOS01ZTg0LTlkNGItODVjNTg4ZGJjYjVk","sourceId":"100000010019083","summary":"","timesTags":[],"uri":"nyt:\u002F\u002Fimage\u002F91199957-40f9-5e84-9d4b-85c588dbcb5d"},"promotionalSummary":"During his visit to Washington, Prime Minister Keir Starmer of Britain handed President Trump a letter from King Charles.","renditions":[{"__typename":"VideoRendition","aspectRatio":"3:2","height":1080,"type":"video_1080p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_1080p.mp4","width":1620},{"__typename":"VideoRendition","aspectRatio":"3:2","height":1080,"type":"syndication","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_hd_synd.mov","width":1620},{"__typename":"VideoRendition","aspectRatio":"3:2","height":720,"type":"video_720p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_720p.mp4","width":1080},{"__typename":"VideoRendition","aspectRatio":"3:2","height":480,"type":"video_480p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_480p.mp4","width":720},{"__typename":"VideoRendition","aspectRatio":"3:2","height":480,"type":"video_480p_webm","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_480p.webm","width":720},{"__typename":"VideoRendition","aspectRatio":"3:2","height":360,"type":"video_360p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_360p.mp4","width":540},{"__typename":"VideoRendition","aspectRatio":"3:2","height":240,"type":"video_240p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_240p.mp4","width":360},{"__typename":"VideoRendition","aspectRatio":"3:2","height":0,"type":"hls","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhls\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"3:2","height":0,"type":"hlsfmp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhlsfmp4\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"9:16","height":1080,"type":"video_1080p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile_1080p.mp4","width":608},{"__typename":"VideoRendition","aspectRatio":"9:16","height":1080,"type":"syndication_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile_hd_synd.mov","width":608},{"__typename":"VideoRendition","aspectRatio":"9:16","height":720,"type":"video_720p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile_720p.mp4","width":404},{"__typename":"VideoRendition","aspectRatio":"9:16","height":480,"type":"video_480p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile_480p.mp4","width":270},{"__typename":"VideoRendition","aspectRatio":"9:16","height":480,"type":"video_480p_webm_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile_480p.webm","width":270},{"__typename":"VideoRendition","aspectRatio":"9:16","height":360,"type":"video_360p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile_360p.mp4","width":202},{"__typename":"VideoRendition","aspectRatio":"9:16","height":240,"type":"video_240p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile_240p.mp4","width":134},{"__typename":"VideoRendition","aspectRatio":"9:16","height":0,"type":"hls_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhls\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"9:16","height":0,"type":"hlsfmp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhlsfmp4\u002F2025\u002F02\u002F28\u002F134836_1_28vid-starmer-trump-kings-letter_wg_mobile\u002Fmaster.m3u8","width":0}],"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010019067","summary":"During his visit to Washington, Prime Minister Keir Starmer of Britain handed President Trump a letter from King Charles.","tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fvideo\u002F3c933852-ae31-5927-8fa7-9206d1eaef74","url":"https:\u002F\u002Fwww.nytimes.com\u002Fvideo\u002Fus\u002F100000010019067\u002Fstarmer-trump-king-invitation-letter-.html","videoAssetSummary":"During his visit to Washington, Prime Minister Keir Starmer of Britain handed President Trump a letter from King Charles."},{"__typename":"Video","aspectRatio":"3:2","autoplay":false,"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"The New York Times","id":"UGVyc29uOm55dDovL3BlcnNvbi8wZjFhNWIxOC03ZTM4LTU5ZmUtODEzMi1iNTAxNWNkMzcxNDg=","promotionalMedia":null}],"renderedRepresentation":"By The New York Times"}],"credits":[{"__typename":"Credit","creator":{"__typename":"Person","displayName":"The New York Times","id":"UGVyc29uOm55dDovL3BlcnNvbi8wZjFhNWIxOC03ZTM4LTU5ZmUtODEzMi1iNTAxNWNkMzcxNDg="},"role":"author"},{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Ang Li","id":"UGVyc29uOm55dDovL3BlcnNvbi9iYTEyOTBkYi04ODY3LTU4ZDMtYmM4ZS05ZDM2ZjY2NTJjNjM="},"role":"credited_producer"},{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Ang Li","id":"UGVyc29uOm55dDovL3BlcnNvbi9iYTEyOTBkYi04ODY3LTU4ZDMtYmM4ZS05ZDM2ZjY2NTJjNjM="},"role":"processing_producer"},{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Rex Sakamoto","id":"UGVyc29uOm55dDovL3BlcnNvbi9iMDZiOTY5Mi04ZjllLTU3MjctODBiYS1hZDY1MzI5YjBjNTk="},"role":"senior_producer"}],"duration":69000,"firstPublished":"2025-02-27T23:51:18.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Trump Says He Believes Putin Will Not Violate Any Ukraine Peace Deal"},"id":"VmlkZW86bnl0Oi8vdmlkZW8vMzM4NmMyNDItODUxNC01NWFhLThiZTMtZGEyN2U5ODM2ZDAw","isCinemagraph":false,"isLive":false,"lastModified":"2025-02-28T00:37:29.876Z","liveUrls":[],"promotionalHeadline":"Trump Says He Believes Putin Will Not Violate Any Ukraine Peace Deal","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTBiYjgxNjQtZDU3ZS01MDc4LWJhOTUtYTBjMTI4ZGRmM2Mz"}},"promotionalMedia":{"__typename":"Image","altText":"","caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Haiyun Jiang for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27vid-ukraine-trump-full-cover-qljc\u002F27vid-ukraine-trump-full-cover-qljc-verticalTwoByThree735.jpg","width":735}]}],"hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTBiYjgxNjQtZDU3ZS01MDc4LWJhOTUtYTBjMTI4ZGRmM2Mz","sourceId":"100000010017948","summary":"","timesTags":[],"uri":"nyt:\u002F\u002Fimage\u002F90bb8164-d57e-5078-ba95-a0c128ddf3c3"},"promotionalSummary":"During a meeting with Prime Minister Keir Starmer of Britain, President Trump said repeatedly that he trusted President Vladimir V. Putin of Russia not to violate the terms of whatever peace deal that might soon be reached to end the war in Ukraine.","renditions":[{"__typename":"VideoRendition","aspectRatio":"3:2","height":1080,"type":"video_1080p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_1080p.mp4","width":1620},{"__typename":"VideoRendition","aspectRatio":"3:2","height":1080,"type":"syndication","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_hd_synd.mov","width":1620},{"__typename":"VideoRendition","aspectRatio":"3:2","height":720,"type":"video_720p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_720p.mp4","width":1080},{"__typename":"VideoRendition","aspectRatio":"3:2","height":480,"type":"video_480p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_480p.mp4","width":720},{"__typename":"VideoRendition","aspectRatio":"3:2","height":480,"type":"video_480p_webm","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_480p.webm","width":720},{"__typename":"VideoRendition","aspectRatio":"3:2","height":360,"type":"video_360p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_360p.mp4","width":540},{"__typename":"VideoRendition","aspectRatio":"3:2","height":240,"type":"video_240p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_240p.mp4","width":360},{"__typename":"VideoRendition","aspectRatio":"3:2","height":0,"type":"hls","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhls\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"3:2","height":0,"type":"hlsfmp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhlsfmp4\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"9:16","height":1080,"type":"video_1080p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile_1080p.mp4","width":608},{"__typename":"VideoRendition","aspectRatio":"9:16","height":1080,"type":"syndication_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile_hd_synd.mov","width":608},{"__typename":"VideoRendition","aspectRatio":"9:16","height":720,"type":"video_720p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile_720p.mp4","width":404},{"__typename":"VideoRendition","aspectRatio":"9:16","height":480,"type":"video_480p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile_480p.mp4","width":270},{"__typename":"VideoRendition","aspectRatio":"9:16","height":480,"type":"video_480p_webm_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile_480p.webm","width":270},{"__typename":"VideoRendition","aspectRatio":"9:16","height":360,"type":"video_360p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile_360p.mp4","width":202},{"__typename":"VideoRendition","aspectRatio":"9:16","height":240,"type":"video_240p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile_240p.mp4","width":134},{"__typename":"VideoRendition","aspectRatio":"9:16","height":0,"type":"hls_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhls\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"9:16","height":0,"type":"hlsfmp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhlsfmp4\u002F2025\u002F02\u002F27\u002F134819_1_27vid-ukraine-trump-full_wg_mobile\u002Fmaster.m3u8","width":0}],"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010017883","summary":"During a meeting with Prime Minister Keir Starmer of Britain, President Trump said repeatedly that he trusted President Vladimir V. Putin of Russia not to violate the terms of whatever peace deal that might soon be reached to end the war in Ukraine.","tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fvideo\u002F3386c242-8514-55aa-8be3-da27e9836d00","url":"https:\u002F\u002Fwww.nytimes.com\u002Fvideo\u002Fus\u002Fpolitics\u002F100000010017883\u002Ftrump-starmer-ukraine-putin.html","videoAssetSummary":"During a meeting with Prime Minister Keir Starmer of Britain, President Trump said repeatedly that he trusted President Vladimir V. Putin of Russia not to violate the terms of whatever peace deal that might soon be reached to end the war in Ukraine."}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"\",\"packageTitleDescription\":null,\"packageTitleOverride\":\"nyt:\u002F\u002Fembeddedinteractive\u002F294c38a6-1a83-573f-9a86-c704b58ac7c4\",\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"showCaption\":false,\"mutedAutoplayOverlay\":false,\"headline\":\"28trump-zelensky-header\",\"mobileAspectRatioCrop\":\"SQUARE\",\"uri\":\"nyt:\u002F\u002Fcarddeck\u002F8d3f2a41-a0d5-44ed-9529-6500220d7792\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"LIVE\",\"kicker\":null,\"headline\":\"Zelensky to Visit White House to Sign Minerals Deal\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"President Trump is to meet with President Volodymyr Zelensky at the White House today after falsely asserting that Ukraine started the war with Russia.\",\"thread\":null,\"sentence\":\"Trump Administration Live Updates: Zelensky to Visit White House to Sign Minerals Deal\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Flegacycollection\u002F452b1d37-7b45-5f33-86f9-23ba2244d6b3\"},{\"showMedia\":false,\"media\":{\"headline\":\"President Trump Is Invited for a ‘Historic’ Second State Visit to U.K.\",\"uri\":\"nyt:\u002F\u002Fvideo\u002F3c933852-ae31-5927-8fa7-9206d1eaef74\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":\"Analysis\",\"headline\":\"With a Letter From King Charles, Keir Starmer Was Welcomed Into Trump’s Court\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Britain’s prime minister gave President Trump a royal invitation and scored several political wins. But his top goal — a security guarantee for Ukraine — remained elusive.\",\"thread\":null,\"sentence\":\"With a Letter From King Charles, Starmer Was Welcomed Into Trump’s Court\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fe5314031-2e3d-5d39-83d9-210e3f501e95\"},{\"showMedia\":false,\"media\":{\"mutedAutoplayOverlay\":true,\"headline\":\"Trump Says He Believes Putin Will Not Violate Any Ukraine Peace Deal\",\"uri\":\"nyt:\u002F\u002Fvideo\u002F3386c242-8514-55aa-8be3-da27e9836d00\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Trump Says He Believes Putin Would Abide by Any Ukraine Peace Deal\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"During a visit to the White House by the British prime minister, President Trump refused to pledge U.S. military support for a peacekeeping force for Ukraine.\",\"thread\":null,\"sentence\":\"Trump Says He Believes Putin Would Abide by Any Ukraine Peace Deal\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F3ab13ed6-8bfc-561a-926e-13bc18df7495\"}]}","sourceId":"f486edca-2a38-4aaf-8ad6-ab9838b67548","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F507e8cc4-1281-5c5e-8df5-79f26dd64b0b","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Ana Swanson","id":"UGVyc29uOm55dDovL3BlcnNvbi9mNmFiNDg4Yy0xODNjLTVkMTMtYjFlMC01YmRmY2ZiYmQyNWE=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F12\u002F10\u002Fmultimedia\u002Fauthor-ana-swanson\u002Fauthor-ana-swanson-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNzIzZmYzMDctODU0Yy01MjQ3LWJiODktNDc3YjZmNDU4YmMw"}},{"__typename":"Person","displayName":"Andrew Duehren","id":"UGVyc29uOm55dDovL3BlcnNvbi9iZDljNjFjZC1mOTRiLTUxZDItYTk3NS03ODM4ZGJhNzcwZjM=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F08\u002F06\u002Freader-center\u002Fauthor-andrew-duehren\u002Fauthor-andrew-duehren-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNDQwZmUzODgtMTJiNy01MDExLWFiYzEtYWEwZjg5NGEzNTk4"}},{"__typename":"Person","displayName":"Colby Smith","id":"UGVyc29uOm55dDovL3BlcnNvbi81NzkxMGFkNy0yZjI5LTU2NDYtOTNlOS1hOGMzMWY0M2Q5Mjc=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F01\u002F29\u002Fbusiness\u002Fauthor_colby_smith\u002Fauthor_colby_smith-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNzA3NWQ1NGMtZDFjOS01ZTQyLWEwNTAtNzhlMmVkZGU4ODQ3"}}],"renderedRepresentation":"By Ana Swanson, Andrew Duehren and Colby Smith"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-fe1a7c93-0a1a-5d69-9592-73c1b4faa68b\u002Fjob-1740747672733\u002Farticle-fe1a7c93-0a1a-5d69-9592-73c1b4faa68b-job-1740747672733.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for When It Comes to Tariffs, Trump Can’t Have It All"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vYjFkOTExZGMtYzQzNi01ZDE1LWExYWYtNjExOWM3OGY4ZjRk","length":541,"sourceId":"100000010019013","uri":"nyt:\u002F\u002Faudio\u002Fb1d911dc-c436-5d15-a1af-6119c78f8f4d"}},"firstPublished":"2025-02-28T10:01:03.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"When It Comes to Tariffs, Trump Can’t Have It All"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2ZlMWE3YzkzLTBhMWEtNWQ2OS05NTkyLTczYzFiNGZhYTY4Yg==","lastModified":"2025-02-28T13:00:49.168Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002F00dc-tariff-logic01\u002F00dc-trump-conflict-ctbl-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002F00dc-tariff-logic01\u002F00dc-trump-conflict-ctbl-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvY2VmNjU2YjYtNzEzOC01MjUwLWFkNDMtY2JhNDQ4M2Q2MjZh"}},"section":{"__typename":"Section","displayName":"Business","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzA0MTViMmIwLTUxM2EtNWU3OC04MGRhLTIxYWI3NzBjYjc1Mw=="},"sourceId":"100000009994458","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Ffe1a7c93-0a1a-5d69-9592-73c1b4faa68b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Feconomy\u002Ftrump-tariff-goals-contradictions.html","wordCount":1445},{"__typename":"Article","associatedNewsletter":{"__typename":"AssociatedNewsletter","newsletterProduct":{"__typename":"HelixNewsletterProduct","id":"SGVsaXhOZXdzbGV0dGVyUHJvZHVjdDpueXQ6Ly9uZXdzbGV0dGVycHJvZHVjdC84Mzk0MjFjZS1lY2M5LTQwOWItODZkMy1hMTgzNDRkZThmYWQ=","name":"DealBook"}},"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ImageBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading3Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RuleBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RuleBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"BlockquoteBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading3Block"},{"__typename":"ParagraphBlock"},{"__typename":"ListBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ListBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ListBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Andrew Ross Sorkin","id":"UGVyc29uOm55dDovL3BlcnNvbi9iMmZkY2E4ZC05NDk0LTUwNTEtYWI1NC1kZmI3MmM5MzE0MGU=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F07\u002F16\u002Fmultimedia\u002Fauthor-andrew-ross-sorkin\u002Fauthor-andrew-ross-sorkin-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMzQwMDc2ZDItZjFlYS01YjEwLWI0MWYtNjM4NzhlZjljZGU0"}},{"__typename":"Person","displayName":"Ravi Mattu","id":"UGVyc29uOm55dDovL3BlcnNvbi8wOTk0YWRhZi05NDc0LTVlOGQtYTU2YS1iNTdlODY3NzU3Y2M=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F09\u002F22\u002Freader-center\u002Fauthor-ravi-mattu\u002Fauthor-ravi-mattu-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMGU1NjVlYWUtOGViNy01MWMzLTlhY2QtOGRiNGY2MDUwZjJl"}},{"__typename":"Person","displayName":"Bernhard Warner","id":"UGVyc29uOm55dDovL3BlcnNvbi81N2ZjOTI1Yi02ZjY4LTU2YjctYTRkMC1hYzIzMGQ2MzZmNTg=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F09\u002F26\u002Freader-center\u002Fauthor-bernhard-warner\u002Fauthor-bernhard-warner-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMWQ2MWI3OWEtNjc0MS01MDQwLWIwM2EtMDhiNTJkMTgyMjE4"}},{"__typename":"Person","displayName":"Sarah Kessler","id":"UGVyc29uOm55dDovL3BlcnNvbi81Y2FhNzJiNy0yZTVmLTVhNjYtOGY4NC1iN2I1M2QzNzVmN2Q=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F06\u002F13\u002Fmultimedia\u002Fauthor-sarah-kessler\u002Fauthor-sarah-kessler-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTcwODA5NzQtYTMxYS01OWUxLTlmMDMtNGM3ZTdlZGQzY2Rk"}},{"__typename":"Person","displayName":"Michael J. de la Merced","id":"UGVyc29uOm55dDovL3BlcnNvbi8wNzUxMjE2Ni0xYTYzLTVlNmUtOWExOS1mYjNhYzIzM2I2ODg=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2019\u002F10\u002F07\u002Freader-center\u002Fauthor-michael-j-de-la-merced\u002Fauthor-michael-j-de-la-merced-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNzRkNmI0NGEtZTNiNS01ODIzLTk3YTMtZWVjMDJmNGMyNmI5"}},{"__typename":"Person","displayName":"Lauren Hirsch","id":"UGVyc29uOm55dDovL3BlcnNvbi84NmUyMjgwNy02NjA4LTVlNTctODRiZS1iNjU0YWI0MDc4NDA=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F08\u002F10\u002Fbusiness\u002Fauthor-lauren-hirsch\u002Fauthor-lauren-hirsch-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTVlY2Y2ZGUtMzJmNi01MjA2LWFjNGEtMWRjYmMyMzJjOGU4"}},{"__typename":"Person","displayName":"Edmund Lee","id":"UGVyc29uOm55dDovL3BlcnNvbi9kOGZkNTUwYS02NTUyLTVhNmMtYTM5MS0zZGJiMDRmMGE5ZjQ=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F10\u002F11\u002Finsider\u002Fedleebiophoto\u002Fedleebiophoto-thumbLarge.jpg","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOGY4MzdiY2MtNGRmNS01MzIyLThkZmMtY2U0NTE1YjMwZjYy"}},{"__typename":"Person","displayName":"Benjamin Mullin","id":"UGVyc29uOm55dDovL3BlcnNvbi8wZGQ2MTgyOS0wNmE3LTVhMDYtOWU5Ny1mNzYzNGQyNDJiMzM=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F02\u002F09\u002Fmultimedia\u002Fauthor-benjamin-mullin\u002Fauthor-benjamin-mullin-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTk1NzU0ODEtMTg0NC01ODliLTk2ZGQtMDM3NTBhMDgwMTRh"}},{"__typename":"Person","displayName":"Danielle Kaye","id":"UGVyc29uOm55dDovL3BlcnNvbi9lOWZiYzM3OC1hMTQxLTVkMWQtOWZkYS03NzRkY2VkMTM0ZDY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F06\u002F14\u002Freader-center\u002Fauthor-danielle-kaye\u002Fauthor-danielle-kaye-thumbLarge-v4.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjU5MThmMWItOWJlZS01NzA3LThmYzMtM2U0ODI4ZGNiYWY0"}}],"renderedRepresentation":"By Andrew Ross Sorkin, Ravi Mattu, Bernhard Warner, Sarah Kessler, Michael J. de la Merced, Lauren Hirsch, Edmund Lee, Benjamin Mullin and Danielle Kaye"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-40788135-75c9-5cdc-bbf8-f46ef5b6d02d\u002Fjob-1740746412117\u002Farticle-40788135-75c9-5cdc-bbf8-f46ef5b6d02d-job-1740746412117.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Inflation Is Stalking Trump"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZDc4MWJiOTUtODg5Ny01Y2IxLWJhZjAtY2RjODk2ZTc0Y2Rm","length":792,"sourceId":"100000010019129","uri":"nyt:\u002F\u002Faudio\u002Fd781bb95-8897-5cb1-baf0-cdc896e74cdf"}},"firstPublished":"2025-02-28T12:14:17.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Inflation Is Stalking Trump"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzQwNzg4MTM1LTc1YzktNWNkYy1iYmY4LWY0NmVmNWI2ZDAyZA==","lastModified":"2025-02-28T12:39:54.210Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZjM3YjE5YTYtMjlmMi01MGE4LTk5YmItMDdmZjUxNDdiMDM3"}},"section":{"__typename":"Section","displayName":"Business","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzA0MTViMmIwLTUxM2EtNWU3OC04MGRhLTIxYWI3NzBjYjc1Mw=="},"sourceId":"100000010019081","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F40788135-75c9-5cdc-bbf8-f46ef5b6d02d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fdealbook\u002Finflation-trump-tariffs.html","wordCount":2051},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Eileen Sullivan","id":"UGVyc29uOm55dDovL3BlcnNvbi8wYTM5M2NhNi0xY2ViLTUxYWEtOWUxYS1hYzJmNjZkMzAzZmM=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2019\u002F12\u002F13\u002Freader-center\u002Fauthor-eileen-sullivan\u002Fauthor-eileen-sullivan-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTgyMjQ5MjAtZGQ0Mi01MTYyLWJhZDctMGQzMThkYTk1MjBm"}}],"renderedRepresentation":"By Eileen Sullivan"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-9a41ce77-0343-5ae2-81c8-56cb5cb081cb\u002Fjob-1740737150137\u002Farticle-9a41ce77-0343-5ae2-81c8-56cb5cb081cb-job-1740737150137.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Office Closures and Relocations Part of Trump’s Plan for Large-Scale Layoffs"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vMWYxYTMyN2QtMjJiYS01MDk1LTkxYWItMDJkZTk4MmQ1YjM5","length":408,"sourceId":"100000010018360","uri":"nyt:\u002F\u002Faudio\u002F1f1a327d-22ba-5095-91ab-02de982d5b39"}},"firstPublished":"2025-02-28T02:26:03.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Office Closures and Relocations Part of Trump’s Plan for Large-Scale Layoffs"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzlhNDFjZTc3LTAzNDMtNWFlMi04MWM4LTU2Y2I1Y2IwODFjYg==","lastModified":"2025-02-28T13:00:43.134Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDU1M2U2MDMtODJjNi01NzdjLTk2NTAtMjJhZDY4OTA5ZmI2"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010016329","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F9a41ce77-0343-5ae2-81c8-56cb5cb081cb","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrump-federal-worker-layoffs.html","wordCount":1107},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Zach Montague","id":"UGVyc29uOm55dDovL3BlcnNvbi8zZDI0YzgyYS0wNGJmLTVlNmItYWE1MC04ZGRjOWJmMGFiNzY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F03\u002F01\u002Freader-center\u002Fauthor-zachary-montague\u002Fauthor-zachary-montague-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOWFkOGQ3MzItZGY4YS01MDZiLTg0MmQtMDVmMmM2YzExMjU4"}}],"renderedRepresentation":"By Zach Montague"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-d32ab414-1972-50fe-8c0a-208302f972b0\u002Fjob-1740711286699\u002Farticle-d32ab414-1972-50fe-8c0a-208302f972b0-job-1740711286699.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Judge Says Trump Administration Memos Directing Mass Firings Were Illegal"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZWZlMDhmODMtZDVlNS01ZjRkLTg3ODQtNDZmNjQ3MmYxOTM1","length":257,"sourceId":"100000010018403","uri":"nyt:\u002F\u002Faudio\u002Fefe08f83-d5e5-5f4d-8784-46f6472f1935"}},"firstPublished":"2025-02-28T02:54:46.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Judge Says Trump Administration Memos Directing Mass Firings Were Illegal"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2QzMmFiNDE0LTE5NzItNTBmZS04YzBhLTIwODMwMmY5NzJiMA==","lastModified":"2025-02-28T13:00:42.943Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjA4NmY1M2EtM2I5OS01MTE2LTg0OTQtNjNjZjIyODcyODc4"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010017975","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Fd32ab414-1972-50fe-8c0a-208302f972b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ffederal-layoffs-trump-opm.html","wordCount":667}],"children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"BylineBlock"},{"__typename":"Heading3Block"},{"__typename":"EmailSignupBlock"},{"__typename":"RuleBlock"},{"__typename":"AudioBlock","media":{"__typename":"Audio","fileUrl":"https:\u002F\u002Fnyt.simplecastaudio.com\u002F03d8b493-87fc-4bd1-931f-8a8e9b945d8a\u002Fepisodes\u002F938e7235-c089-4ab6-a45e-112e48735ef6\u002Faudio\u002F128\u002Fdefault.mp3?awCollectionId=03d8b493-87fc-4bd1-931f-8a8e9b945d8a&awEpisodeId=938e7235-c089-4ab6-a45e-112e48735ef6&nocache","headline":{"__typename":"CreativeWorkHeadline","default":"Listen to ‘The Daily’: The Art of the Deal"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZWY2NDkzYmYtYjVlYy01MGMwLTk3N2QtOWY4ZmY1YzllM2Ey","length":1672,"podcastSeries":{"__typename":"AudioPodcastSeries","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2017\u002F01\u002F29\u002Fpodcasts\u002Fthe-daily-album-art\u002Fthe-daily-album-art-square640-v5.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjQyM2YwN2EtNmY5ZC01MzE0LTgzZDktYTQzOWU1ZTEyYzRk"},"title":"The Daily"},"promotionalImage":null,"sourceId":"100000010004745","uri":"nyt:\u002F\u002Faudio\u002Fef6493bf-b5ec-50c0-977d-9f8ff5c9e3a2"}},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DetailBlock"},{"__typename":"RuleBlock"},{"__typename":"Heading3Block"},{"__typename":"UnstructuredBlock"},{"__typename":"UnstructuredBlock"},{"__typename":"UnstructuredBlock"},{"__typename":"Heading3Block"},{"__typename":"ListBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"RuleBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Michael Barbaro","id":"UGVyc29uOm55dDovL3BlcnNvbi9jZjdmNjdlYS0zMWYxLTVjYjUtODE2OC1hMTk3NWE3ODA1NjA=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2019\u002F01\u002F25\u002Fmultimedia\u002Fauthor-michael-barbaro\u002Fauthor-michael-barbaro-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYWE2NWNhNzItNzAxYi01NGYyLTkyMTgtODBlMDRmYzRhNWNh"}},{"__typename":"Person","displayName":"Catie Edmondson","id":"UGVyc29uOm55dDovL3BlcnNvbi83MWJjMzljNy0zOGVhLTU4M2ItYjFkYi1kYmRjMDM3YjI4N2M=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F04\u002F11\u002Freader-center\u002Fauthor-catie-edmonson\u002Fauthor-catie-edmonson-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMGI2YzMzMjQtOWM2Ny01NGVmLThlNmEtNTMxYjNjZmJiMjcz"}},{"__typename":"Person","displayName":"Maggie Haberman","id":"UGVyc29uOm55dDovL3BlcnNvbi81M2U0ZmU5OC0wNzBjLTU3ZTYtOWEzMy1lZmY4MmViMTkwZjk=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F07\u002F12\u002Fmultimedia\u002Fauthor-maggie-haberman\u002Fauthor-maggie-haberman-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTE2MjI2OTYtYWRhMC01MjRmLTk0Y2ItOWEwMGRjNzI5YTNj"}},{"__typename":"Person","displayName":"Zolan Kanno-Youngs","id":"UGVyc29uOm55dDovL3BlcnNvbi80NzQ1YzNlNy0wNTM3LTUyNjktOThjMC05ZTJiOWJkZjA4YjQ=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2019\u002F12\u002F13\u002Freader-center\u002Fauthor-zolan-kanno-youngs\u002Fauthor-zolan-kanno-youngs-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNDRiOGQyOGEtNzY5OC01ZDRlLWFjNTYtMjVkYTliZDAyNzEw"}},{"__typename":"Person","displayName":"Carlos Prieto","id":"UGVyc29uOm55dDovL3BlcnNvbi80MzFhMGI3Yi1lZmU0LTUzNmYtOGU4Yi02NjU0M2IzMTdkNzI=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F05\u002F23\u002Freader-center\u002Fauthor-carlos-prieto\u002Fauthor-carlos-prieto-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMDc5NWM3MjQtZDQxMy01MmE0LTgxYmMtZWM0MGE3MWY2MjQ1"}},{"__typename":"Person","displayName":"Eric Krupke","id":"UGVyc29uOm55dDovL3BlcnNvbi80YjNmNmEyYS00M2U4LTU4ZjEtODViYS05YzIwYzk3NGJhZGY=","promotionalMedia":null},{"__typename":"Person","displayName":"Rachel Quester","id":"UGVyc29uOm55dDovL3BlcnNvbi9kZmJhYjhiOS1kYTMxLTU1NDMtODhlYy0wOTdhMzQzNGNlNGY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F05\u002F29\u002Freader-center\u002Fauthor-rachel-quester\u002Fauthor-rachel-quester-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTdiYWU2ZTUtZjQ2OS01NjI2LTk3ODAtMDUyY2EwOTVjY2Ey"}},{"__typename":"Person","displayName":"M.J. Davis Lin","id":"UGVyc29uOm55dDovL3BlcnNvbi9jYzI0ZDczYy0xZjczLTVjM2EtOTU4My05NGEwOWEwNTYyM2I=","promotionalMedia":null},{"__typename":"Person","displayName":"Diane Wong","id":"UGVyc29uOm55dDovL3BlcnNvbi9kOGI3OGE4NC04ZmMzLTUxM2QtOWVmMC02MDNlNWJiOTgwZWM=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F04\u002F24\u002Freader-center\u002Fauthor-diane-wong\u002Fauthor-diane-wong-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMTgxMmUyYjYtNzI3OS01YzQ2LTlhYmUtMGQ0ZmYxNzliYTM5"}},{"__typename":"Person","displayName":"Rowan Niemisto","id":"UGVyc29uOm55dDovL3BlcnNvbi8zMDJiNzY5My1hNmY1LTVhMWYtYTIyMC0wN2RhNGJkMTA5ZGY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F05\u002F23\u002Freader-center\u002Fauthor-rowan-niemisto\u002Fauthor-rowan-niemisto-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvODYxZGYzNTEtZjU0Ni01MmMzLTg3MWMtZDUzZDM3Yzk0Mjg4"}},{"__typename":"Person","displayName":"Elisheba Ittoop","id":"UGVyc29uOm55dDovL3BlcnNvbi9hNDJhZjJhNi02NjBhLTVkN2EtOGFlMi1lNzM1NzhiNmY3Mzk=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F05\u002F23\u002Freader-center\u002Fauthor-elisheba-ittoop\u002Fauthor-elisheba-ittoop-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNzY0NGFlZTEtZWY0Yi01YTYxLWFkYzktYzFiN2Q1NzNmNTBh"}},{"__typename":"Person","displayName":"Chris Wood","id":"UGVyc29uOm55dDovL3BlcnNvbi84MDNiMjk2Ny0wMzU3LTU3ZDYtODRiNi1mMDY3ZWY5ZDNkMjY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F09\u002F22\u002Freader-center\u002Fauthor-chris-wood\u002Fauthor-chris-wood-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjRjMjAwNjYtZGViYi01MjVhLTkzODItNGRjMTE2MTEyM2Fk"}}],"renderedRepresentation":"By Michael Barbaro, Catie Edmondson, Maggie Haberman, Zolan Kanno-Youngs, Carlos Prieto, Eric Krupke, Rachel Quester, M.J. Davis Lin, Diane Wong, Rowan Niemisto, Elisheba Ittoop and Chris Wood"}],"featuredAudio":null,"firstPublished":"2025-02-28T11:00:06.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Trump 2.0: The Art of the Deal"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2IwYjA3OTExLWQwNjAtNWE5YS1hM2U2LWUwZGIwZDQ3M2Q4NA==","lastModified":"2025-02-28T11:36:27.694Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002F28TheDaily\u002F28TheDaily-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002F28TheDaily\u002F28TheDaily-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDEzYmQyMzYtODUyZi01NjcwLWI0YWItZjJiMDBmYTA5MDA5"}},"section":{"__typename":"Section","displayName":"Podcasts","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzExMDc5OTg3LWFlOGQtNWY1Zi04YzJlLThjNjZkMzM3ZDJiYQ=="},"sourceId":"100000010004750","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Fb0b07911-d060-5a9a-a3e6-e0db0d473d84","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002Ftrump-minerals-ukraine-republican-budget.html","wordCount":347}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:36:27.694Z","uri":"nyt:\u002F\u002Farticle\u002Fb0b07911-d060-5a9a-a3e6-e0db0d473d84","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002Ftrump-minerals-ukraine-republican-budget.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F701e0c629b8e486bb2f4671061afc860","hierarchyType":"addOn","isPersonalized":false,"layoutType":"StandardAddon","media":[{"__typename":"Video","aspectRatio":"3:2","autoplay":false,"bylines":[],"credits":[{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Sophie Erickson","id":"UGVyc29uOm55dDovL3BlcnNvbi9hMTk0MzQyYi04NjliLTVkN2EtYmM4Mi1jYTNlNjYxMDUxMTE="},"role":"credited_producer"},{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Sophie Erickson","id":"UGVyc29uOm55dDovL3BlcnNvbi9hMTk0MzQyYi04NjliLTVkN2EtYmM4Mi1jYTNlNjYxMDUxMTE="},"role":"processing_producer"},{"__typename":"Credit","creator":{"__typename":"Person","displayName":"Sophie Erickson","id":"UGVyc29uOm55dDovL3BlcnNvbi9hMTk0MzQyYi04NjliLTVkN2EtYmM4Mi1jYTNlNjYxMDUxMTE="},"role":"senior_producer"}],"duration":284000,"firstPublished":"2025-02-28T10:49:01.981Z","headline":{"__typename":"CreativeWorkHeadline","default":"Trump’s Deal Making and Breaking"},"id":"VmlkZW86bnl0Oi8vdmlkZW8vYmZmMmI2ZGQtMDQxZi01NTUwLTlkZTYtMzEzMTI0MDU5NjVl","isCinemagraph":false,"isLive":false,"lastModified":"2025-02-28T10:49:01.981Z","liveUrls":[],"promotionalHeadline":"Trump’s Deal Making and Breaking","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-square640-v2.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-threeByTwoLargeAt2X-v2.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZGUyMTg4ODYtYzQ4Yy01OTYxLWIzYjItYTYwMzlmMDdhOTc5"}},"promotionalMedia":{"__typename":"Image","altText":"","caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-smallSquare252-v2.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-square640-v2.jpg","width":640},{"__typename":"ImageRendition","height":1484,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-mediumSquareAt3X-v2.jpg","width":1484}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-threeByTwoMediumAt2X-v2.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-threeByTwoSmallAt2X-v2.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-videoSixteenByNine1050-v2.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fpodcasts\u002F28daily-core\u002F28daily-core-verticalTwoByThree735-v3.jpg","width":735}]}],"hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZGUyMTg4ODYtYzQ4Yy01OTYxLWIzYjItYTYwMzlmMDdhOTc5","sourceId":"100000010018054","summary":"","timesTags":[],"uri":"nyt:\u002F\u002Fimage\u002Fde218886-c48c-5961-b3b2-a6039f07a979"},"promotionalSummary":"Catie Edmondson, Maggie Haberman and Zolan Kanno-Youngs discuss how this week, President Trump proposed two deals — one at home and the other abroad — that would require allies to put his needs ahead of their own.","renditions":[{"__typename":"VideoRendition","aspectRatio":"3:2","height":1080,"type":"video_1080p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_1080p.mp4","width":1620},{"__typename":"VideoRendition","aspectRatio":"3:2","height":1080,"type":"syndication","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_hd_synd.mov","width":1620},{"__typename":"VideoRendition","aspectRatio":"3:2","height":720,"type":"video_720p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_720p.mp4","width":1080},{"__typename":"VideoRendition","aspectRatio":"3:2","height":480,"type":"video_480p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_480p.mp4","width":720},{"__typename":"VideoRendition","aspectRatio":"3:2","height":480,"type":"video_480p_webm","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_480p.webm","width":720},{"__typename":"VideoRendition","aspectRatio":"3:2","height":360,"type":"video_360p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_360p.mp4","width":540},{"__typename":"VideoRendition","aspectRatio":"3:2","height":240,"type":"video_240p_mp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_240p.mp4","width":360},{"__typename":"VideoRendition","aspectRatio":"3:2","height":0,"type":"hls","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhls\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"3:2","height":0,"type":"hlsfmp4","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhlsfmp4\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"9:16","height":1080,"type":"video_1080p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile_1080p.mp4","width":608},{"__typename":"VideoRendition","aspectRatio":"9:16","height":1080,"type":"syndication_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile_hd_synd.mov","width":608},{"__typename":"VideoRendition","aspectRatio":"9:16","height":720,"type":"video_720p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile_720p.mp4","width":404},{"__typename":"VideoRendition","aspectRatio":"9:16","height":480,"type":"video_480p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile_480p.mp4","width":270},{"__typename":"VideoRendition","aspectRatio":"9:16","height":480,"type":"video_480p_webm_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile_480p.webm","width":270},{"__typename":"VideoRendition","aspectRatio":"9:16","height":360,"type":"video_360p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile_360p.mp4","width":202},{"__typename":"VideoRendition","aspectRatio":"9:16","height":240,"type":"video_240p_mp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile_240p.mp4","width":134},{"__typename":"VideoRendition","aspectRatio":"9:16","height":0,"type":"hls_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhls\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile\u002Fmaster.m3u8","width":0},{"__typename":"VideoRendition","aspectRatio":"9:16","height":0,"type":"hlsfmp4_mobile","url":"https:\u002F\u002Fvp.nyt.com\u002Fvideo\u002Fhlsfmp4\u002F2025\u002F02\u002F26\u002F134680_1_28-thedaily-roundtable-clip_wg_mobile\u002Fmaster.m3u8","width":0}],"section":{"__typename":"Section","displayName":"Podcasts","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzExMDc5OTg3LWFlOGQtNWY1Zi04YzJlLThjNjZkMzM3ZDJiYQ=="},"sourceId":"100000010013747","summary":"Catie Edmondson, Maggie Haberman and Zolan Kanno-Youngs discuss how this week, President Trump proposed two deals — one at home and the other abroad — that would require allies to put his needs ahead of their own.","tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fvideo\u002Fbff2b6dd-041f-5550-9de6-31312405965e","url":"https:\u002F\u002Fwww.nytimes.com\u002Fvideo\u002Fpodcasts\u002Fthe-daily\u002F100000010013747\u002Ftrumps-deal-making-and-breaking.html","videoAssetSummary":"Catie Edmondson, Maggie Haberman and Zolan Kanno-Youngs discuss how this week, President Trump proposed two deals — one at home and the other abroad — that would require allies to put his needs ahead of their own."}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"kickersDisplay\":\"DISPLAY_ALL\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"showCaption\":false,\"mutedAutoplayOverlay\":true,\"headline\":\"\",\"mobileAspectRatioCrop\":\"SQUARE\",\"uri\":\"nyt:\u002F\u002Fvideo\u002Fbff2b6dd-041f-5550-9de6-31312405965e\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Trump 2.0: The Art of the Deal\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Watch or listen to our political round table about President Trump, the Republican budget and Ukraine.\",\"thread\":null,\"sentence\":null,\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fb0b07911-d060-5a9a-a3e6-e0db0d473d84\"}]}","sourceId":"50684f32-3108-4215-b115-efa66835fb25","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F1d5ac077-43d6-5951-a299-a499b22bf806","url":"","variantAllocation":null,"children":[]},{"__typename":"ProgrammingNode","assets":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F081935f75ee64106862c627439fdc5d0","hierarchyType":"addOn","isPersonalized":true,"layoutType":"Carousel2","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":4,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002Fd27b52e8-e2d8-5227-8d56-8b1f88e16b80","surface":"home-packages-GE1_EI-EngBandit_Fixed"},"properties":"{\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"HEADLINE\",\"mediaEmphasis\":\"THUMB\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[]}","sourceId":"90286f2a-f7db-4520-a7d7-a7ddae5be7ec","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Ff056d60c-90d9-5ce4-aea0-1cb731923f80","url":"","variantAllocation":null,"children":[]}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:36:27.694Z","uri":"nyt:\u002F\u002Farticle\u002Fb0b07911-d060-5a9a-a3e6-e0db0d473d84","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002Ftrump-minerals-ukraine-republican-budget.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Ff5ca9dbe6d104286b9ea02f3475bf1fb","hierarchyType":"dropzone","sourceId":"8b2ff081-c23b-4b9b-8c33-8f5b8b1a065c65feb920-aaea-475e-9401-57d6a3a432e7belowPackage","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F9d4ee97d-2b67-5321-a1fe-9e66a4223f2b","url":"","variantAllocation":null,"zoneKey":"belowPackage","assets":undefined,"media":undefined}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:36:27.694Z","uri":"nyt:\u002F\u002Farticle\u002Fb0b07911-d060-5a9a-a3e6-e0db0d473d84","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002Ftrump-minerals-ukraine-republican-budget.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:49.168Z","uri":"nyt:\u002F\u002Farticle\u002Ffe1a7c93-0a1a-5d69-9592-73c1b4faa68b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Feconomy\u002Ftrump-tariff-goals-contradictions.html"},{"__typename":"Article","lastModified":"2025-02-28T12:39:54.210Z","uri":"nyt:\u002F\u002Farticle\u002F40788135-75c9-5cdc-bbf8-f46ef5b6d02d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fdealbook\u002Finflation-trump-tariffs.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:43.134Z","uri":"nyt:\u002F\u002Farticle\u002F9a41ce77-0343-5ae2-81c8-56cb5cb081cb","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrump-federal-worker-layoffs.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:42.943Z","uri":"nyt:\u002F\u002Farticle\u002Fd32ab414-1972-50fe-8c0a-208302f972b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ffederal-layoffs-trump-opm.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F4de2ff955aed41118d76498313f3f226","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"EmbeddedInteractive","bylines":[],"compatibility":"INLINE","credit":"","displayProperties":{"__typename":"CreativeWorkDisplayProperties","displayOverrides":["DARK_MODE_COMPATIBLE"]},"firstPublished":"2025-01-21T02:27:47.000Z","html":"\u003Cnav aria-labelledby=\"styln-trump-administration-hp-menu\" class=\"css-qvlk0g\"\u003E\u003Cp id=\"styln-trump-administration-hp-menu\"\u003E\u003Cspan class=\"css-14sajpe\"\u003ETrump Administration\u003C\u002Fspan\u003E\u003C\u002Fp\u003E\u003Cul class=\"css-11xr2bo\"\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002Fus\u002Ftrump-agenda-2025.html\"\u003ETracking Major Moves\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002Fus\u002Ftrump-administration-lawsuits.html\"\u003ELawsuits Tracker\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F20\u002Fupshot\u002Ftrump-executive-orders-legality.html\"\u003EIs That Legal?\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F01\u002F29\u002Fus\u002Fpolitics\u002Ftrump-cabinet-confirmations-tracker.html\"\u003EConfirmations Tracker\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003C\u002Ful\u003E\u003Cscript\u003E\"use strict\";(function(){\u002F\u002F prevent menus from overflowing into right rail on desktop\nfunction a(){var a=document.querySelector(\"nav[aria-labelledby=\\\"styln-trump-administration-hp-menu\\\"]\"),b=document.querySelectorAll(\"[data-hierarchy=\\\"zone\\\"]\"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth\u003Ec.clientWidth)\u002F\u002F remove the last item from the menu if it is overflowing\n{var d=a.querySelectorAll(\"li\"),e=d[d.length-1];e.remove()}}\u002F\u002F only check for overflow if the user is on desktop or tablet\n\u002F\u002F remove links that match ones already shown in the live band\nvar b=document.querySelector(\"#hp-live-band-list\");if(b){var c=Array.from(b.querySelectorAll(\"a\")).map(function(b){return b.href}),d=document.querySelectorAll(\"nav[aria-labelledby=\\\"styln-trump-administration-hp-menu\\\"] li\");d.forEach(function(a){var b,d=null===(b=a.querySelector(\"a\"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia(\"(pointer: coarse) and (max-width: 739px)\").matches){a();var e;window.addEventListener(\"resize\",function(){clearTimeout(e),e=setTimeout(a,250)})}})();\u003C\u002Fscript\u003E\u003C\u002Fnav\u003E","id":"RW1iZWRkZWRJbnRlcmFjdGl2ZTpueXQ6Ly9lbWJlZGRlZGludGVyYWN0aXZlL2VhMTY4ZTQzLWQxNjQtNWQ2ZC1hZjg5LWJkNDg0NWE3ZjFlZg==","lastModified":"2025-02-27T17:48:49.394Z","promotionalImage":null,"promotionalMedia":null,"section":{"__typename":"Section","displayName":"Admin","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q0MmQzYTNmLTAyYTgtNWRlNy1hOTEyLTUyMGZhNmI0NGY0ZQ=="},"slug":"prism-styln-trump-administration-homepageMenu-1737426444607","sourceId":"100000009939007","storyFormat":null,"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fembeddedinteractive\u002Fea168e43-d164-5d6d-af89-bd4845a7f1ef","url":"null"},{"__typename":"Image","altText":"A woman, shown from behind, wearing a yellow jacket and holding a sign reading “Public Service is a Badge of Honor!\" over her head.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Supporters sent off former employees of the U.S. Agency for International Development as they gathered their personal belongings from the Reagan Building in Washington on Thursday."},"captionOverride":null,"credit":"Anna Rose Layden for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj-hp\u002F27dc-federal-cuts1-gvhj-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj-hp\u002F27dc-federal-cuts1-gvhj-square640.jpg","width":640},{"__typename":"ImageRendition","height":1802,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj-hp\u002F27dc-federal-cuts1-gvhj-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj-hp\u002F27dc-federal-cuts1-gvhj-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj-hp\u002F27dc-federal-cuts1-gvhj-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj-hp\u002F27dc-federal-cuts1-gvhj-hp-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj-hp\u002F27dc-federal-cuts1-gvhj-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T09:53:28.413Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMTE1M2RkY2ItNDY4Ni01MjE0LThiM2UtYmI3NTEyZjQ0MzY0","lastModified":"2025-02-28T09:53:28.413Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010019003","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F1153ddcb-4686-5214-8b3e-bb7512f44364","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj-hp.html"},{"__typename":"Image","altText":"Two shoppers look at a wall of products including butter at a grocery store.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Stubbornly high inflation is beginning to weigh on households, with sentiment souring fast, economists note."},"captionOverride":null,"credit":"Brandon Bell\u002FGetty Images","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv\u002F28db-inflation-kclv-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T11:03:37.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZjM3YjE5YTYtMjlmMi01MGE4LTk5YmItMDdmZjUxNDdiMDM3","lastModified":"2025-02-28T12:09:08.330Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010019082","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Ff37b19a6-29f2-50a8-99bb-07ff5147b037","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28db-inflation-kclv.html"},{"__typename":"Image","altText":"A woman, shown from behind, wearing a yellow jacket and holding a sign reading “Public Service is a Badge of Honor!\" over her head.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Supporters sent off former employees of the U.S. Agency for International Development as they gathered their personal belongings from the Reagan Building in Washington on Thursday."},"captionOverride":null,"credit":"Anna Rose Layden for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-square640.jpg","width":640},{"__typename":"ImageRendition","height":1802,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj\u002F27dc-federal-cuts1-gvhj-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T02:26:01.460Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDU1M2U2MDMtODJjNi01NzdjLTk2NTAtMjJhZDY4OTA5ZmI2","lastModified":"2025-02-28T02:26:01.460Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017562","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fd553e603-82c6-577c-9650-22ad68909fb6","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-federal-cuts1-gvhj.html"},{"__typename":"Image","altText":"A building with a sign reading “Theodore Roosevelt Federal Building” above its doors. Shrubbery is in the foreground.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"The Office of Personnel Management cannot order agencies to cut staff, the judge ruled."},"captionOverride":null,"credit":"Valerie Plesch for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-square640.jpg","width":640},{"__typename":"ImageRendition","height":1801,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq\u002F27dc-opm-halt1-lkcq-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T02:54:44.125Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjA4NmY1M2EtM2I5OS01MTE2LTg0OTQtNjNjZjIyODcyODc4","lastModified":"2025-02-28T02:54:44.125Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010018085","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fb086f53a-3b99-5116-8494-63cf22872878","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27dc-opm-halt1-lkcq.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":\"nyt:\u002F\u002Fembeddedinteractive\u002Fea168e43-d164-5d6d-af89-bd4845a7f1ef\",\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"showCaption\":false,\"mutedAutoplayOverlay\":false,\"headline\":\"\",\"mobileAspectRatioCrop\":\"SQUARE\",\"uri\":\"nyt:\u002F\u002Fimage\u002F1153ddcb-4686-5214-8b3e-bb7512f44364\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"How Trump’s Tariffs Could Undermine Each Other\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"President Trump has promised big results, from raising revenue to reviving domestic manufacturing. But many of his goals contradict one another.\",\"thread\":null,\"sentence\":\"When It Comes to Tariffs, Trump Can’t Have It All\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Ffe1a7c93-0a1a-5d69-9592-73c1b4faa68b\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Ff37b19a6-29f2-50a8-99bb-07ff5147b037\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Inflation Is Stalking Trump\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Consumer sentiment has turned south as high prices weigh on households. Could that crimp big pieces of the president’s economic agenda, including tariffs?\",\"thread\":null,\"sentence\":\"Inflation Is Stalking Trump\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F40788135-75c9-5cdc-bbf8-f46ef5b6d02d\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fd553e603-82c6-577c-9650-22ad68909fb6\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Office Closures and Relocations Part of Trump’s Plan for Large-Scale Layoffs\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The Trump administration’s new requirements for agencies to move forward with mass cuts to the federal work force have employees even more on edge.\",\"thread\":null,\"sentence\":\"Office Closures and Relocations Part of Trump’s Plan for Large-Scale Layoffs\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F9a41ce77-0343-5ae2-81c8-56cb5cb081cb\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fb086f53a-3b99-5116-8494-63cf22872878\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Memos Directing Mass Firings of Federal Workers Were Illegal, Judge Rules\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"A federal judge in California ordered the retraction of the memos and suggested, but did not order, that the layoffs be stopped.\",\"thread\":null,\"sentence\":\"Judge Says Trump Administration Memos Directing Mass Firings Were Illegal\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fd32ab414-1972-50fe-8c0a-208302f972b0\"}]}","sourceId":"65feb920-aaea-475e-9401-57d6a3a432e7","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F4e51f90b-2533-5148-b6ce-9c3598e7f45e","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"EmbeddedInteractive","bylines":[],"compatibility":"INLINE","credit":"","displayProperties":{"__typename":"CreativeWorkDisplayProperties","displayOverrides":["DARK_MODE_COMPATIBLE"]},"firstPublished":"2025-01-22T16:28:41.000Z","html":"\u003Cp class=\"g-demo-sentence\"\u003E \u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002Ftips\"\u003E\u003Cb\u003EGot a Tip?\u003C\u002Fb\u003E The Times offers several ways to send important information confidentially ›\u003C\u002Fa\u003E\u003C\u002Fp\u003E\r\n\r\n","id":"RW1iZWRkZWRJbnRlcmFjdGl2ZTpueXQ6Ly9lbWJlZGRlZGludGVyYWN0aXZlLzBkZjk0NTVhLTFjNTItNWE1NC05NDAwLTc0NDNjNDNkOWE2OA==","lastModified":"2025-01-22T20:54:52.365Z","promotionalImage":null,"promotionalMedia":null,"section":{"__typename":"Section","displayName":"Admin","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q0MmQzYTNmLTAyYTgtNWRlNy1hOTEyLTUyMGZhNmI0NGY0ZQ=="},"slug":"2025-hp-tip-strip","sourceId":"100000009942400","storyFormat":null,"tone":"NEWS","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F0df9455a-1c52-5a54-9400-7443c43d9a68","url":"null"}],"children":[],"descendantAssets":[{"__typename":"EmbeddedInteractive","lastModified":"2025-01-22T20:54:52.365Z","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F0df9455a-1c52-5a54-9400-7443c43d9a68","url":"null"}],"eventId":"pubp:\u002F\u002Fevent\u002F333af249148f43b9af7ac49d8ff50eb8","hierarchyType":"package","isPersonalized":false,"layoutType":"RichMedia","media":[],"personalizationConfig":null,"properties":"{\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"bannerNewsStatus\":\"NONE\",\"italicizeHeadline\":false,\"deepLink\":null,\"assets\":[{\"uri\":\"nyt:\u002F\u002Fembeddedinteractive\u002F0df9455a-1c52-5a54-9400-7443c43d9a68\"}]}","sourceId":"341cb0ed-d987-44b6-8c67-0a70692b7a18","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F7e8295b8-6f1f-54e4-a676-9be9a7d184e1","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"TwitterEmbedBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"TwitterEmbedBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"TwitterEmbedBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Jack Nicas","id":"UGVyc29uOm55dDovL3BlcnNvbi80ZmRmZjA4ZC00ZGI2LTVhNWQtOGU4Zi1kYTBmNmZlODg4MjY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F11\u002F26\u002Fmultimedia\u002Fauthor-jack-nicas\u002Fauthor-jack-nicas-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZjYyNWFiZDktMDE0Zi01YjU1LWI5NTQtNmY3MDBkOTkzYmJm"}},{"__typename":"Person","displayName":"David Yaffe-Bellany","id":"UGVyc29uOm55dDovL3BlcnNvbi8yZThiMzc2YS1lOGQ0LTUxMDctYjk4Ny1hOGEzMmQ0MTM2NjA=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F01\u002F25\u002Freader-center\u002Fauthor-david-yaffe-bellany\u002Fauthor-david-yaffe-bellany-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNDJkYjJlYjAtZTNiNC01OTgxLWFkNzgtN2VhODQ4MGY5OTU4"}}],"renderedRepresentation":"By Jack Nicas and David Yaffe-Bellany"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-dcdfc485-364a-5a09-bb3d-79f645a6f129\u002Fjob-1740736925834\u002Farticle-dcdfc485-364a-5a09-bb3d-79f645a6f129-job-1740736925834.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for A Crypto Scam Swindled Argentines. What Did the President Know?"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZGQ1YWRiMmQtMDRmNC01MzFlLWE0NjYtNzNjN2E2M2MxY2Yx","length":941,"sourceId":"100000010019025","uri":"nyt:\u002F\u002Faudio\u002Fdd5adb2d-04f4-531e-a466-73c7a63c1cf1"}},"firstPublished":"2025-02-28T10:02:05.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"A Crypto Scam Swindled Argentines. What Did the President Know?"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2RjZGZjNDg1LTM2NGEtNWEwOS1iYjNkLTc5ZjY0NWE2ZjEyOQ==","lastModified":"2025-02-28T11:45:26.642Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjJkNmE1MmEtZWJjMS01YzhiLWFlNzMtOTk5ZTY2NjA0MThk"}},"section":{"__typename":"Section","displayName":"World","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzcwZTg2NWI2LWNjNzAtNTE4MS04NGM5LTgzNjhiM2E1YzM0Yg=="},"sourceId":"100000010002142","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Fdcdfc485-364a-5a09-bb3d-79f645a6f129","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Fargentina-crypto-scandal-president.html","wordCount":2390}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:45:26.642Z","uri":"nyt:\u002F\u002Farticle\u002Fdcdfc485-364a-5a09-bb3d-79f645a6f129","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Fargentina-crypto-scandal-president.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F3e26ba5e9f7648e0ab0010cfb44955e2","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"Image","altText":"Javier Milei, the president of Argentina, stands in the middle of a crowd of supporters. ","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Mr. Milei greeted his followers after giving a speech during the Conservative Political Action Conference in Buenos Aires, in December."},"captionOverride":null,"credit":"Magali Druscovich for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F01\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk\u002F22argentina-milei-crypto-hpmk-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T10:02:00.710Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjJkNmE1MmEtZWJjMS01YzhiLWFlNzMtOTk5ZTY2NjA0MThk","lastModified":"2025-02-28T10:02:00.710Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010004146","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fb2d6a52a-ebc1-5c8b-ae73-999e6660418d","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F22argentina-milei-crypto-hpmk.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fb2d6a52a-ebc1-5c8b-ae73-999e6660418d\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"A Crypto Scam Swindled Argentines. What Did the President Know?\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"A new cryptocurrency called $Libra and promoted by President Javier Milei bilked investors out of $250 million.\",\"thread\":null,\"sentence\":\"A Crypto Scam Swindled Argentines. What Did the President Know?\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fdcdfc485-364a-5a09-bb3d-79f645a6f129\"}]}","sourceId":"9024695d-76eb-4a98-8a9e-2e6529acc1b6","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F78df4001-cf6c-534a-8bc8-172c741f3bc5","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"VideoBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Julia Jacobs","id":"UGVyc29uOm55dDovL3BlcnNvbi84NmZkNjNiNi0yMTkzLTVlYjgtYTg3Mi1lNzQyMzMwNDkyODQ=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F12\u002F06\u002Freader-center\u002Fauthor-julia-jacobs\u002Fauthor-julia-jacobs-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMzE5NmZmZjAtMDlhMS01Y2Y2LWI5MzQtNzFhNmU5MWYyMjIz"}}],"renderedRepresentation":"By Julia Jacobs"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-85713c47-6ee2-52cc-84eb-02be4ce1ee1f\u002Fjob-1740737066504\u002Farticle-85713c47-6ee2-52cc-84eb-02be4ce1ee1f-job-1740737066504.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for For Gene Hackman, a Jarring End to a Quiet, Art-Filled Life in Santa Fe"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vMmMxYTU0ZmYtYjk5YS01YjJjLTg1M2UtNjQ1YTBmOGY4NTJm","length":381,"sourceId":"100000010019044","uri":"nyt:\u002F\u002Faudio\u002F2c1a54ff-b99a-5b2c-853e-645a0f8f852f"}},"firstPublished":"2025-02-28T10:04:26.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"For Gene Hackman, a Jarring End to a Quiet, Art-Filled Life in Santa Fe"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzg1NzEzYzQ3LTZlZTItNTJjYy04NGViLTAyYmU0Y2UxZWUxZg==","lastModified":"2025-02-28T11:25:49.237Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-santa-fe-1-gzbq\u002F28hackman-santa-fe-1-gzbq-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-santa-fe-1-gzbq\u002F28hackman-santa-fe-1-gzbq-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZjQwNGM5MzYtMzdlMC01ZGFkLTk0YTYtOTM2YjNjNDIxMjZi"}},"section":{"__typename":"Section","displayName":"Movies","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzYyYjNkNDcxLTRhZTUtNWFjMi04MzZmLWNiN2FkNTMxYzRjYg=="},"sourceId":"100000010017517","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F85713c47-6ee2-52cc-84eb-02be4ce1ee1f","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fgene-hackman-death-new-mexico.html","wordCount":1098},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"VideoBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"InteractiveBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"The New York Times","id":"UGVyc29uOm55dDovL3BlcnNvbi8wZjFhNWIxOC03ZTM4LTU5ZmUtODEzMi1iNTAxNWNkMzcxNDg=","promotionalMedia":null}],"renderedRepresentation":"By The New York Times"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-cd0c66fd-7767-5885-b4d3-597efd65d1a0\u002Fjob-1740735343094\u002Farticle-cd0c66fd-7767-5885-b4d3-597efd65d1a0-job-1740735343094.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for What We Know About Gene Hackman’s Death"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vM2JhYjQ4ZTMtOGUyZC01MjE0LWFjNTMtNjU2NmNhODU2OWVj","length":216,"sourceId":"100000010018990","uri":"nyt:\u002F\u002Faudio\u002F3bab48e3-8e2d-5214-ac53-6566ca8569ec"}},"firstPublished":"2025-02-28T09:35:25.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"What We Know About Gene Hackman’s Death"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2NkMGM2NmZkLTc3NjctNTg4NS1iNGQzLTU5N2VmZDY1ZDFhMA==","lastModified":"2025-02-28T12:44:37.741Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28xp-hackman-wwk-htpg\u002F28xp-hackman-wwk-htpg-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28xp-hackman-wwk-htpg\u002F28xp-hackman-wwk-htpg-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDAzYzY3YmYtNDU3MC01NWVhLThhZjMtNDBmZjAwNjMyMzMy"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010018175","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Fcd0c66fd-7767-5885-b4d3-597efd65d1a0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fgene-hackman-death-updates.html","wordCount":594},{"__typename":"Interactive","bylines":[],"firstPublished":"2025-02-27T20:38:05.000Z","id":"SW50ZXJhY3RpdmU6bnl0Oi8vaW50ZXJhY3RpdmUvZDQxOTkyY2MtNmI2Yy01NDRkLWE1NjgtMzA5ZTc4NDZjOWM5","interactiveWordCount":null,"lastModified":"2025-02-28T00:40:02.920Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-square640.png"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-threeByTwoLargeAt2X.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZTg4YTQ3Y2ItOTk4NC01YjMwLWI0YWEtZGQ4OTk4NjU0ZjU3"}},"section":{"__typename":"Section","displayName":"Movies","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzYyYjNkNDcxLTRhZTUtNWFjMi04MzZmLWNiN2FkNTMxYzRjYg=="},"sourceId":"100000010017393","tone":"NEWS","uri":"nyt:\u002F\u002Finteractive\u002Fd41992cc-6b6c-544d-a568-309e7846c9c9","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fmovies\u002Fhackman-affidavit.html"},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Manohla Dargis","id":"UGVyc29uOm55dDovL3BlcnNvbi9jYzNlZjBmNS05MWFmLTU2MjctYjAxMC02Y2UxYTcxMDM1NzI=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F02\u002F15\u002Freader-center\u002Fauthor-manohla-dargis\u002Fauthor-manohla-dargis-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOThlNTZhZDItNjM1Mi01OTBkLWIxZmEtNTRkZWQxMjc1Mjdi"}}],"renderedRepresentation":"By Manohla Dargis"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-cb0fd188-e910-5caf-b97f-9959fe27aad8\u002Fjob-1740708751426\u002Farticle-cb0fd188-e910-5caf-b97f-9959fe27aad8-job-1740708751426.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Gene Hackman’s Smile Could Give You Shivers"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vMWY0YWU1MmMtYWU4NC01MmRjLTg5YTEtN2M0NjJiOTVjNmNi","length":347,"sourceId":"100000010018079","uri":"nyt:\u002F\u002Faudio\u002F1f4ae52c-ae84-52dc-89a1-7c462b95c6cb"}},"firstPublished":"2025-02-27T23:30:35.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Gene Hackman’s Smile Could Give You Shivers"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2NiMGZkMTg4LWU5MTAtNWNhZi1iOTdmLTk5NTlmZTI3YWFkOA==","lastModified":"2025-02-28T02:12:14.299Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMTNhM2FiNjYtYjM5YS01ZmMzLWIwY2YtM2RiM2Q5MjFhMjMx"}},"section":{"__typename":"Section","displayName":"Movies","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzYyYjNkNDcxLTRhZTUtNWFjMi04MzZmLWNiN2FkNTMxYzRjYg=="},"sourceId":"100000010017255","tone":"FEATURE","uri":"nyt:\u002F\u002Farticle\u002Fcb0fd188-e910-5caf-b97f-9959fe27aad8","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fmovies\u002Fgene-hackman-unforgiven-bonnie-clyde.html","wordCount":987}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:25:49.237Z","uri":"nyt:\u002F\u002Farticle\u002F85713c47-6ee2-52cc-84eb-02be4ce1ee1f","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fgene-hackman-death-new-mexico.html"},{"__typename":"Article","lastModified":"2025-02-28T12:44:37.741Z","uri":"nyt:\u002F\u002Farticle\u002Fcd0c66fd-7767-5885-b4d3-597efd65d1a0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fgene-hackman-death-updates.html"},{"__typename":"Interactive","lastModified":"2025-02-28T00:40:02.920Z","uri":"nyt:\u002F\u002Finteractive\u002Fd41992cc-6b6c-544d-a568-309e7846c9c9","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fmovies\u002Fhackman-affidavit.html"},{"__typename":"Article","lastModified":"2025-02-28T02:12:14.299Z","uri":"nyt:\u002F\u002Farticle\u002Fcb0fd188-e910-5caf-b97f-9959fe27aad8","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fmovies\u002Fgene-hackman-unforgiven-bonnie-clyde.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F75effbf0596f42aab9609151e5440fb7","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"EmbeddedInteractive","bylines":[],"compatibility":"INLINE","credit":"","displayProperties":{"__typename":"CreativeWorkDisplayProperties","displayOverrides":["DARK_MODE_COMPATIBLE"]},"firstPublished":"2025-02-27T13:34:59.000Z","html":"\u003Cnav aria-labelledby=\"styln-gene-hackman-hp-menu\" class=\"css-qvlk0g\"\u003E\u003Cp id=\"styln-gene-hackman-hp-menu\"\u003E\u003Cspan class=\"css-14sajpe\"\u003EGene Hackman (1930-2025)\u003C\u002Fspan\u003E\u003C\u002Fp\u003E\u003Cul class=\"css-11xr2bo\"\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fobituaries\u002Fgene-hackman-dead.html\"\u003EObituary\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fmovies\u002Fgene-hackman-appraisal.html\"\u003EAppraisal\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fmovies\u002Fgene-hackman-hollywood-reaction.html\"\u003ELuminaries Mourn\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fmovies\u002Fgene-hackman-movies-streaming.html\"\u003EMovies to Stream\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2001\u002F12\u002F16\u002Fmovies\u002Fgene-hackman-hollywood-s-every-angry-man.html\"\u003E2001 Profile\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003C\u002Ful\u003E\u003Cscript\u003E\"use strict\";(function(){\u002F\u002F prevent menus from overflowing into right rail on desktop\nfunction a(){var a=document.querySelector(\"nav[aria-labelledby=\\\"styln-gene-hackman-hp-menu\\\"]\"),b=document.querySelectorAll(\"[data-hierarchy=\\\"zone\\\"]\"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth\u003Ec.clientWidth)\u002F\u002F remove the last item from the menu if it is overflowing\n{var d=a.querySelectorAll(\"li\"),e=d[d.length-1];e.remove()}}\u002F\u002F only check for overflow if the user is on desktop or tablet\n\u002F\u002F remove links that match ones already shown in the live band\nvar b=document.querySelector(\"#hp-live-band-list\");if(b){var c=Array.from(b.querySelectorAll(\"a\")).map(function(b){return b.href}),d=document.querySelectorAll(\"nav[aria-labelledby=\\\"styln-gene-hackman-hp-menu\\\"] li\");d.forEach(function(a){var b,d=null===(b=a.querySelector(\"a\"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia(\"(pointer: coarse) and (max-width: 739px)\").matches){a();var e;window.addEventListener(\"resize\",function(){clearTimeout(e),e=setTimeout(a,250)})}})();\u003C\u002Fscript\u003E\u003C\u002Fnav\u003E","id":"RW1iZWRkZWRJbnRlcmFjdGl2ZTpueXQ6Ly9lbWJlZGRlZGludGVyYWN0aXZlL2ZlNTA2ZWQ1LTRkOGMtNTJjOC04NzA5LTBmNzBjMDU1ZGVjZg==","lastModified":"2025-02-27T21:48:45.785Z","promotionalImage":null,"promotionalMedia":null,"section":{"__typename":"Section","displayName":"Admin","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q0MmQzYTNmLTAyYTgtNWRlNy1hOTEyLTUyMGZhNmI0NGY0ZQ=="},"slug":"prism-styln-gene-hackman-homepageMenu-1740663283666","sourceId":"100000010016286","storyFormat":null,"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fembeddedinteractive\u002Ffe506ed5-4d8c-52c8-8709-0f70c055decf","url":"null"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"George Brich\u002FAssociated Press","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-hp-01-lqtz\u002F28hackman-hp-01-lqtz-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-hp-01-lqtz\u002F28hackman-hp-01-lqtz-square640-v2.jpg","width":640},{"__typename":"ImageRendition","height":1741,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-hp-01-lqtz\u002F28hackman-hp-01-lqtz-mediumSquareAt3X-v2.jpg","width":1739}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1001,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-hp-01-lqtz\u002F28hackman-hp-01-lqtz-threeByTwoMediumAt2X-v2.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-hp-01-lqtz\u002F28hackman-hp-01-lqtz-threeByTwoSmallAt2X-v2.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-hp-01-lqtz\u002F28hackman-hp-01-lqtz-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1104,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-hp-01-lqtz\u002F28hackman-hp-01-lqtz-verticalTwoByThree735-v2.jpg","width":735}]}],"firstPublished":"2025-02-28T10:04:57.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvN2M0ZmMzNmEtZWNiMi01NjliLTk0MTUtMjQ0OTA1YmYxZTZk","lastModified":"2025-02-28T13:12:35.662Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010019008","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F7c4fc36a-ecb2-569b-9415-244905bf1e6d","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28hackman-hp-01-lqtz.html"},{"__typename":"Image","altText":"The exterior of a home with many trees surrounding the property. ","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"The house owned by actor Gene Hackman and his wife, Betsy Arakawa, on Thursday."},"captionOverride":null,"credit":"Roberto E. Rosales\u002FAssociated Press","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28xp-hackman-wwk-htpg\u002F28xp-hackman-wwk-htpg-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28xp-hackman-wwk-htpg\u002F28xp-hackman-wwk-htpg-square640.jpg","width":640},{"__typename":"ImageRendition","height":986,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28xp-hackman-wwk-htpg\u002F28xp-hackman-wwk-htpg-mediumSquareAt3X.jpg","width":986}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":986,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28xp-hackman-wwk-htpg\u002F28xp-hackman-wwk-htpg-threeByTwoMediumAt2X.jpg","width":1478},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28xp-hackman-wwk-htpg\u002F28xp-hackman-wwk-htpg-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28xp-hackman-wwk-htpg\u002F28xp-hackman-wwk-htpg-videoSixteenByNine1050.jpg","width":1050}]}],"firstPublished":"2025-02-28T00:30:32.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDAzYzY3YmYtNDU3MC01NWVhLThhZjMtNDBmZjAwNjMyMzMy","lastModified":"2025-02-28T10:59:05.913Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010018184","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fd03c67bf-4570-55ea-8af3-40ff00632332","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F28xp-hackman-wwk-htpg.html"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-smallSquare252.png","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-square640.png","width":640},{"__typename":"ImageRendition","height":1224,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-mediumSquareAt3X.png","width":1224}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":816,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-threeByTwoMediumAt2X.png","width":1224},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-threeByTwoSmallAt2X.png","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-videoSixteenByNine1050.png","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo\u002Fdoc-75218578-e541-465c-9bbd-3-promo-verticalTwoByThree735.png","width":735}]}],"firstPublished":"2025-02-27T20:38:03.677Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZTg4YTQ3Y2ItOTk4NC01YjMwLWI0YWEtZGQ4OTk4NjU0ZjU3","lastModified":"2025-02-27T20:38:03.677Z","promotionalImage":null,"section":null,"sourceId":"100000010017392","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fe88a47cb-9984-5b30-b4aa-dd8998654f57","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fdoc-75218578-e541-465c-9bbd-3-promo.html"},{"__typename":"Image","altText":"A man in a cowboy hat smiles at a terrified-looking woman. ","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Gene Hackman, with Frances Fisher, in the 1992 western “Unforgiven.”"},"captionOverride":null,"credit":"Warner Bros., via Everett Collection","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis\u002F27hackman-dargis-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-27T23:30:32.760Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMTNhM2FiNjYtYjM5YS01ZmMzLWIwY2YtM2RiM2Q5MjFhMjMx","lastModified":"2025-02-27T23:30:32.760Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Arts","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzZlNmVlMjkyLWI0YmQtNTAwNi1hNjE5LTljZWFiMDM1MjRmMg=="},"sourceId":"100000010017817","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F13a3ab66-b39a-5fc3-b0cf-3db3d921a231","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Farts\u002F27hackman-dargis.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"\",\"packageTitleDescription\":null,\"packageTitleOverride\":\"nyt:\u002F\u002Fembeddedinteractive\u002Ffe506ed5-4d8c-52c8-8709-0f70c055decf\",\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"showCaption\":false,\"mutedAutoplayOverlay\":false,\"headline\":\"\",\"mobileAspectRatioCrop\":\"SQUARE\",\"uri\":\"nyt:\u002F\u002Fimage\u002F7c4fc36a-ecb2-569b-9415-244905bf1e6d\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"For Gene Hackman, a Jarring End to a Quiet, Art-Filled Life in Santa Fe\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The actor, who was found dead with his wife and one of their dogs, had written novels and painted since leaving Hollywood for retirement in New Mexico.\",\"thread\":null,\"sentence\":\"For Gene Hackman, a Jarring End to a Quiet, Art-Filled Life in Santa Fe\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F85713c47-6ee2-52cc-84eb-02be4ce1ee1f\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fd03c67bf-4570-55ea-8af3-40ff00632332\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"What We Know About Hackman’s Death\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Mr. Hackman was found dead inside his home, along with his wife and a dog. The sheriff’s office in Santa Fe, N.M., is investigating.\",\"thread\":null,\"sentence\":\"What We Know About Gene Hackman’s Death\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fcd0c66fd-7767-5885-b4d3-597efd65d1a0\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fe88a47cb-9984-5b30-b4aa-dd8998654f57\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Read the Search Warrant Affidavit in the Death Inquiry\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"An affidavit from a Santa Fe County detective described how deputies found the bodies of Gene Hackman and his wife, Betsy Arakawa, on Wednesday.\",\"thread\":null,\"sentence\":\"Read the Search Warrant Affidavit in the Gene Hackman Death Inquiry\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Finteractive\u002Fd41992cc-6b6c-544d-a568-309e7846c9c9\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F13a3ab66-b39a-5fc3-b0cf-3db3d921a231\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"An Appraisal: A Smile That Could Give You Shivers\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"In “Bonnie and Clyde” and “Unforgiven,” the actor used his charm to great disarming effect, flashing a smile before abruptly shifting to a sneer.\",\"thread\":null,\"sentence\":\"Gene Hackman’s Smile Could Give You Shivers\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fcb0fd188-e910-5caf-b97f-9959fe27aad8\"}]}","sourceId":"5685e8a3-da0c-4bab-8f62-e8a48a67d335","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fef1e432a-05c0-5907-9f30-c379727535b0","url":"","variantAllocation":null}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:37:15.494Z","uri":"nyt:\u002F\u002Farticle\u002F95773dee-1b8a-5f08-b78a-29ed1903e90c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-federal-bureaucracy-takeover.html"},{"__typename":"Article","lastModified":"2025-02-28T10:04:16.382Z","uri":"nyt:\u002F\u002Farticle\u002F89c13edf-2ccb-5223-91c7-a96b2e7b0998","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-doge-timeline-takeaways.html"},{"__typename":"Article","lastModified":"2025-02-28T11:20:12.055Z","uri":"nyt:\u002F\u002Farticle\u002F8b200636-8df5-5caa-8daa-308efea287b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-headlines\u002Fiowa-trans-rights-zelensky-trump.html"},{"__typename":"LegacyCollection","lastModified":"2025-02-28T13:10:16.130Z","uri":"nyt:\u002F\u002Flegacycollection\u002F452b1d37-7b45-5f33-86f9-23ba2244d6b3","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F28\u002Fus\u002Ftrump-news"},{"__typename":"Article","lastModified":"2025-02-28T12:13:45.314Z","uri":"nyt:\u002F\u002Farticle\u002Fe5314031-2e3d-5d39-83d9-210e3f501e95","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Feurope\u002Ftrump-starmer-king-charles.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:43.670Z","uri":"nyt:\u002F\u002Farticle\u002F3ab13ed6-8bfc-561a-926e-13bc18df7495","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fstarmer-ukraine-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T11:36:27.694Z","uri":"nyt:\u002F\u002Farticle\u002Fb0b07911-d060-5a9a-a3e6-e0db0d473d84","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002Ftrump-minerals-ukraine-republican-budget.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:49.168Z","uri":"nyt:\u002F\u002Farticle\u002Ffe1a7c93-0a1a-5d69-9592-73c1b4faa68b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Feconomy\u002Ftrump-tariff-goals-contradictions.html"},{"__typename":"Article","lastModified":"2025-02-28T12:39:54.210Z","uri":"nyt:\u002F\u002Farticle\u002F40788135-75c9-5cdc-bbf8-f46ef5b6d02d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fdealbook\u002Finflation-trump-tariffs.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:43.134Z","uri":"nyt:\u002F\u002Farticle\u002F9a41ce77-0343-5ae2-81c8-56cb5cb081cb","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrump-federal-worker-layoffs.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:42.943Z","uri":"nyt:\u002F\u002Farticle\u002Fd32ab414-1972-50fe-8c0a-208302f972b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ffederal-layoffs-trump-opm.html"},{"__typename":"EmbeddedInteractive","lastModified":"2025-01-22T20:54:52.365Z","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F0df9455a-1c52-5a54-9400-7443c43d9a68","url":"null"},{"__typename":"Article","lastModified":"2025-02-28T11:45:26.642Z","uri":"nyt:\u002F\u002Farticle\u002Fdcdfc485-364a-5a09-bb3d-79f645a6f129","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Fargentina-crypto-scandal-president.html"},{"__typename":"Article","lastModified":"2025-02-28T11:25:49.237Z","uri":"nyt:\u002F\u002Farticle\u002F85713c47-6ee2-52cc-84eb-02be4ce1ee1f","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fgene-hackman-death-new-mexico.html"},{"__typename":"Article","lastModified":"2025-02-28T12:44:37.741Z","uri":"nyt:\u002F\u002Farticle\u002Fcd0c66fd-7767-5885-b4d3-597efd65d1a0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fgene-hackman-death-updates.html"},{"__typename":"Interactive","lastModified":"2025-02-28T00:40:02.920Z","uri":"nyt:\u002F\u002Finteractive\u002Fd41992cc-6b6c-544d-a568-309e7846c9c9","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fmovies\u002Fhackman-affidavit.html"},{"__typename":"Article","lastModified":"2025-02-28T02:12:14.299Z","uri":"nyt:\u002F\u002Farticle\u002Fcb0fd188-e910-5caf-b97f-9959fe27aad8","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fmovies\u002Fgene-hackman-unforgiven-bonnie-clyde.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F3e5c2b7f5dd44514b390d7fa4fd07916","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"d694d76f-c3e3-46c2-9cd2-cc8d42b71461","trackingName":"Top Stories","uri":"nyt:\u002F\u002Fprogrammingnode\u002Fc43bf195-3ac0-5dfd-815f-475d2771b288","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fec46991c4d534c5ba31d39f4248c27b9","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"848b7d83-2760-4d04-8237-93dd320a5a9d","trackingName":"Opinion Mobile","uri":"nyt:\u002F\u002Fprogrammingnode\u002F83240f6b-1a84-5115-94d6-4266546e19ad","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Chris Buckley","id":"UGVyc29uOm55dDovL3BlcnNvbi8yNGZjZDk0OC1jZDY5LTU5ZmMtOWI5NS00ZTJlZjIwYzExNTY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F10\u002F08\u002Fmultimedia\u002Fauthor-chris-buckley\u002Fauthor-chris-buckley-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTg1NDg2NDEtNTI4Yi01YmNjLWFhOTktNDBlMjg2ZWQ1MGVk"}},{"__typename":"Person","displayName":"Damien Cave","id":"UGVyc29uOm55dDovL3BlcnNvbi83Y2NiNzQ4Ny1mZDViLTVhNWEtYTdhNi0wZGI3OTAyZjg5Yzg=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F10\u002F08\u002Fmultimedia\u002Fauthor-damien-cave\u002Fauthor-damien-cave-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMDRhZGU2ZjYtYmRkMC01MDU5LWFmNDAtZmI4ZTk1ZGIzM2Iy"}}],"renderedRepresentation":"By Chris Buckley and Damien Cave"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-720399f2-c1ec-5608-9963-cfaa03abd183\u002Fjob-1740728317409\u002Farticle-720399f2-c1ec-5608-9963-cfaa03abd183-job-1740728317409.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for China’s Military Puts Pacific on Notice as U.S. Priorities Shift"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vM2RiYzFiZGQtYjVkMC01ZjhkLWJkMDUtZWEzZDJmZTM2MGJj","length":495,"sourceId":"100000010018636","uri":"nyt:\u002F\u002Faudio\u002F3dbc1bdd-b5d0-5f8d-bd05-ea3d2fe360bc"}},"firstPublished":"2025-02-28T07:38:21.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"China’s Military Puts Pacific on Notice as U.S. Priorities Shift"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzcyMDM5OWYyLWMxZWMtNTYwOC05OTYzLWNmYWEwM2FiZDE4Mw==","lastModified":"2025-02-28T11:30:16.929Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMTM0MDYxYzQtOTRkNi01NTg3LTlmYjMtOWMzYTdiM2I3MGI5"}},"section":{"__typename":"Section","displayName":"World","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzcwZTg2NWI2LWNjNzAtNTE4MS04NGM5LTgzNjhiM2E1YzM0Yg=="},"sourceId":"100000010015671","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F720399f2-c1ec-5608-9963-cfaa03abd183","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fasia\u002Fchina-military-drills-pacific.html","wordCount":1298}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:30:16.929Z","uri":"nyt:\u002F\u002Farticle\u002F720399f2-c1ec-5608-9963-cfaa03abd183","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fasia\u002Fchina-military-drills-pacific.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fad07623c2b31441f99f10871235bf5ba","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Hogp\u002FRoyal Australian Navy\u002FADF, via Associated Press","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo\u002F28china-military-clhj-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T05:32:48.361Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMTM0MDYxYzQtOTRkNi01NTg3LTlmYjMtOWMzYTdiM2I3MGI5","lastModified":"2025-02-28T05:32:48.361Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010018553","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F134061c4-94d6-5587-9fb3-9c3a7b3b70b9","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28china-military-promo.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"headline\":\"\",\"uri\":\"nyt:\u002F\u002Fimage\u002F134061c4-94d6-5587-9fb3-9c3a7b3b70b9\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"China’s Military Puts Pacific on Notice as U.S. Priorities Shift\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"China is flexing its military muscle in the region to show that it will not wait for the Trump administration to decide how hard it wants to counter Beijing.\",\"thread\":null,\"sentence\":\"With Military Displays Across the Pacific, China’s Assertiveness\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F720399f2-c1ec-5608-9963-cfaa03abd183\"}]}","sourceId":"64399eef-a631-4f4c-b54f-8db5129f76c9","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Ff63c8563-2fb0-5086-9595-c7151c0e6661","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Adam Rasgon","id":"UGVyc29uOm55dDovL3BlcnNvbi9lNWRhMzZiYi04NmQwLTVjYzktODdlOS0xODZjYjM4NzRlZWY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F05\u002F14\u002Freader-center\u002Fauthor-adam-rasgon\u002Fauthor-adam-rasgon-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTA2ODM1ZTQtZTJmYi01Y2U5LTg1M2YtZGNhNTE0YWQ2YzE2"}},{"__typename":"Person","displayName":"Fatima AbdulKarim","id":"UGVyc29uOm55dDovL3BlcnNvbi8zN2NjNmRmNi1hODNmLTU3ODYtYWZhNS04OWI2ZmY4NDY2Yjg=","promotionalMedia":null}],"renderedRepresentation":"By Adam Rasgon and Fatima AbdulKarim"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-f86860c9-bc29-598c-bc53-5b81b1def8a5\u002Fjob-1740738777235\u002Farticle-f86860c9-bc29-598c-bc53-5b81b1def8a5-job-1740738777235.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for West Bank Operation Tests Palestinian Leaders’ Ability to Root Out Militants"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZGEwZThmOTgtYTBmMC01ODM5LWJkZDgtYzdmMmJlMjNkZmEz","length":472,"sourceId":"100000010019074","uri":"nyt:\u002F\u002Faudio\u002Fda0e8f98-a0f0-5839-bdd8-c7f2be23dfa3"}},"firstPublished":"2025-02-28T10:32:41.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"West Bank Operation Tests Palestinian Leaders’ Ability to Root Out Militants"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2Y4Njg2MGM5LWJjMjktNTk4Yy1iYzUzLTViODFiMWRlZjhhNQ==","lastModified":"2025-02-28T10:33:26.206Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOGNjMzRlZTMtMzhhMi01Y2Q4LTllY2ItMjFmNjU4NGFlNDE3"}},"section":{"__typename":"Section","displayName":"World","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzcwZTg2NWI2LWNjNzAtNTE4MS04NGM5LTgzNjhiM2E1YzM0Yg=="},"sourceId":"100000009918506","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Ff86860c9-bc29-598c-bc53-5b81b1def8a5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fwest-bank-jenin-palestinian-authority-israel.html","wordCount":1187},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderFullBleedHorizontalBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Raja Abdulrahim","id":"UGVyc29uOm55dDovL3BlcnNvbi82YTMxYjMzNy0yNjE4LTVjZTMtOTZhZi03ZDIzZTVhY2UyNDg=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F04\u002F06\u002Freader-center\u002Fauthor-raja-abdulrahim\u002Fauthor-raja-abdulrahim-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMmRjMzMyYTYtMDA1YS01ZjhiLWFhM2EtNjFkYmY1YjczODA2"}},{"__typename":"Person","displayName":"Kiana Hayeri","id":"UGVyc29uOm55dDovL3BlcnNvbi85NjFlY2VjYS01Y2QwLTUwNDMtODlhZC0wNTNkODcxYjc2YzM=","promotionalMedia":null}],"renderedRepresentation":"By Raja Abdulrahim and Kiana Hayeri"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-eed2db6f-2fad-5bf8-b96f-b8ad776652ff\u002Fjob-1740737032631\u002Farticle-eed2db6f-2fad-5bf8-b96f-b8ad776652ff-job-1740737032631.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for As Ramadan Nears, Syrians Feel the Pinch of a Cash Shortage"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vNGVkZTQzYjYtMTZmMC01YjlmLWE4YjYtYThiMjJkZDIwMDdj","length":417,"sourceId":"100000010019036","uri":"nyt:\u002F\u002Faudio\u002F4ede43b6-16f0-5b9f-a8b6-a8b22dd2007c"}},"firstPublished":"2025-02-28T10:03:52.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"As Ramadan Nears, Syrians Feel the Pinch of a Cash Shortage"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2VlZDJkYjZmLTJmYWQtNWJmOC1iOTZmLWI4YWQ3NzY2NTJmZg==","lastModified":"2025-02-28T11:02:22.739Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNmZhZGQ4ZGItZDBhMS01NDE0LThlYmQtNmU5NmY1MGU2MjQ3"}},"section":{"__typename":"Section","displayName":"World","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzcwZTg2NWI2LWNjNzAtNTE4MS04NGM5LTgzNjhiM2E1YzM0Yg=="},"sourceId":"100000010012898","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Feed2db6f-2fad-5bf8-b96f-b8ad776652ff","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fsyria-ramadan-economy-cash-shortage.html","wordCount":1152}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T10:33:26.206Z","uri":"nyt:\u002F\u002Farticle\u002Ff86860c9-bc29-598c-bc53-5b81b1def8a5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fwest-bank-jenin-palestinian-authority-israel.html"},{"__typename":"Article","lastModified":"2025-02-28T11:02:22.739Z","uri":"nyt:\u002F\u002Farticle\u002Feed2db6f-2fad-5bf8-b96f-b8ad776652ff","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fsyria-ramadan-economy-cash-shortage.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fb1eb4a74a44640769e3fd23204d4550d","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"EmbeddedInteractive","bylines":[],"compatibility":"INLINE","credit":"","displayProperties":{"__typename":"CreativeWorkDisplayProperties","displayOverrides":["DARK_MODE_COMPATIBLE"]},"firstPublished":"2024-02-19T15:07:48.000Z","html":"\u003Cnav aria-labelledby=\"styln-middle-east-crisis-hp-menu\" class=\"css-qvlk0g\"\u003E\u003Cp id=\"styln-middle-east-crisis-hp-menu\"\u003E\u003Cspan class=\"css-14sajpe\"\u003EMiddle East Tensions\u003C\u002Fspan\u003E\u003C\u002Fp\u003E\u003Cul class=\"css-11xr2bo\"\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F26\u002Fworld\u002Fmiddleeast\u002Fwho-are-the-israeli-hostages-hamas.html\"\u003E4 Hostages’ Bodies Returned\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F24\u002Fworld\u002Fmiddleeast\u002Fisrael-west-bank-what-why.html\"\u003ERising West Bank Tensions\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F22\u002Fworld\u002Fmiddleeast\u002Fsyria-assad-israel-raids.html\"\u003EIsraeli Incursions Into Syria\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F14\u002Fworld\u002Fmiddleeast\u002Fgaza-rubble-missing-bodies.html\"\u003ESearching for Gaza’s Dead\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003C\u002Ful\u003E\u003Cscript\u003E\"use strict\";(function(){\u002F\u002F prevent menus from overflowing into right rail on desktop\nfunction a(){var a=document.querySelector(\"nav[aria-labelledby=\\\"styln-middle-east-crisis-hp-menu\\\"]\"),b=document.querySelectorAll(\"[data-hierarchy=\\\"zone\\\"]\"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth\u003Ec.clientWidth)\u002F\u002F remove the last item from the menu if it is overflowing\n{var d=a.querySelectorAll(\"li\"),e=d[d.length-1];e.remove()}}\u002F\u002F only check for overflow if the user is on desktop or tablet\n\u002F\u002F remove links that match ones already shown in the live band\nvar b=document.querySelector(\"#hp-live-band-list\");if(b){var c=Array.from(b.querySelectorAll(\"a\")).map(function(b){return b.href}),d=document.querySelectorAll(\"nav[aria-labelledby=\\\"styln-middle-east-crisis-hp-menu\\\"] li\");d.forEach(function(a){var b,d=null===(b=a.querySelector(\"a\"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia(\"(pointer: coarse) and (max-width: 739px)\").matches){a();var e;window.addEventListener(\"resize\",function(){clearTimeout(e),e=setTimeout(a,250)})}})();\u003C\u002Fscript\u003E\u003C\u002Fnav\u003E","id":"RW1iZWRkZWRJbnRlcmFjdGl2ZTpueXQ6Ly9lbWJlZGRlZGludGVyYWN0aXZlLzNjYTNkMzhlLTRiYmItNTg0OS1hMTJkLTIzMmRiYmI1ZmYyZQ==","lastModified":"2025-02-27T21:51:58.986Z","promotionalImage":null,"promotionalMedia":null,"section":{"__typename":"Section","displayName":"Admin","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q0MmQzYTNmLTAyYTgtNWRlNy1hOTEyLTUyMGZhNmI0NGY0ZQ=="},"slug":"prism-styln-iran-bombings-homepageMenu-1708267852451","sourceId":"100000009319548","storyFormat":null,"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F3ca3d38e-4bbb-5849-a12d-232dbbb5ff2e","url":"null"},{"__typename":"Image","altText":"Men in camouflage carrying guns step out of a military-style vehicle.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Security forces from the Palestinian Authority deploying in the Jenin camp neighborhood of the West Bank in January. "},"captionOverride":null,"credit":"Jaafar Ashtiyeh\u002FAgence France-Presse — Getty Images","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F06\u002Fmultimedia\u002F00jenin-01-gkcf\u002F00jenin-01-gkcf-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T10:32:37.944Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOGNjMzRlZTMtMzhhMi01Y2Q4LTllY2ItMjFmNjU4NGFlNDE3","lastModified":"2025-02-28T10:32:37.944Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000009970924","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F8cc34ee3-38a2-5cd8-9ecb-21f6584ae417","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F00jenin-01-gkcf.html"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Kiana Hayeri for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F27\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo\u002F27syria-ramadan-01-lhpc-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T10:03:49.781Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNmZhZGQ4ZGItZDBhMS01NDE0LThlYmQtNmU5NmY1MGU2MjQ3","lastModified":"2025-02-28T10:03:49.781Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010018986","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F6fadd8db-d0a1-5414-8ebd-6e96f50e6247","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F27syria-ramadan-01-lhpc-promo.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":\"nyt:\u002F\u002Fembeddedinteractive\u002F3ca3d38e-4bbb-5849-a12d-232dbbb5ff2e\",\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F8cc34ee3-38a2-5cd8-9ecb-21f6584ae417\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"West Bank Operation Tests Palestinian Leaders’ Ability to Root Out Militants\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The Palestinian Authority wants to prove it can handle security in Gaza, even if it means working in parallel with a major Israeli military raid.\",\"thread\":null,\"sentence\":\"West Bank Operation Tests Palestinian Leaders’ Ability to Root Out Militants\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Ff86860c9-bc29-598c-bc53-5b81b1def8a5\"},{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F6fadd8db-d0a1-5414-8ebd-6e96f50e6247\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"As Ramadan Nears, Syrians Feel the Pinch of a Cash Shortage\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The Assad dictatorship is out, but Syria’s economy is in chaos after a civil war and recent policy shifts. The situation is dampening a typically festive season.\",\"thread\":null,\"sentence\":\"As Ramadan Nears, Syrians Feel the Pinch of a Cash Shortage\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Feed2db6f-2fad-5bf8-b96f-b8ad776652ff\"}]}","sourceId":"a0836577-4f71-4204-a3d2-3cc580e24a46","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F8a566e7d-ee8b-595a-97b5-504396af97f0","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"VideoBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Mitch Smith","id":"UGVyc29uOm55dDovL3BlcnNvbi9lMTBkYTYxZC0xY2Q4LTU5ZGUtODczMi0zNmVhMDc2Y2NlZTQ=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F09\u002F10\u002Fmultimedia\u002Fauthor-mitch-smith\u002Fauthor-mitch-smith-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvN2NhYTk3NDMtZjBmMC01MTY5LTg1MTktNzhlZmQ2OTVkYmQz"}}],"renderedRepresentation":"By Mitch Smith"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-2734f206-c837-5724-a971-94aedb7c0cc5\u002Fjob-1740698419195\u002Farticle-2734f206-c837-5724-a971-94aedb7c0cc5-job-1740698419195.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Iowa Lawmakers Pass Bill to Eliminate Transgender Civil Rights Protections"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vMzU1YTMyMjUtYmZjNC01M2JlLThkNGYtMTQ4MDA3NDg3ZWM3","length":302,"sourceId":"100000010017913","uri":"nyt:\u002F\u002Faudio\u002F355a3225-bfc4-53be-8d4f-148007487ec7"}},"firstPublished":"2025-02-27T22:27:36.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Iowa Lawmakers Pass Bill to Eliminate Transgender Civil Rights Protections"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzI3MzRmMjA2LWM4MzctNTcyNC1hOTcxLTk0YWVkYjdjMGNjNQ==","lastModified":"2025-02-28T02:57:42.743Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMDMxYmIxYWUtYzY4Ny01OGViLTg4YWUtMjU1ZmEyMDU4ZDFj"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010014562","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F2734f206-c837-5724-a971-94aedb7c0cc5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fiowa-transgender-civil-rights-bill.html","wordCount":764},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Dave Philipps","id":"UGVyc29uOm55dDovL3BlcnNvbi84MjA4MjQ0Yy1hNTkyLTU2MDctOWJhNi1mZjFiZmY0MzdmYzQ=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2019\u002F10\u002F04\u002Freader-center\u002Fauthor-dave-philipps\u002Fauthor-dave-philipps-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjg4MzViZWItYWQ3ZS01MmU2LWJhYTQtYTk4NzljYjFiMWI3"}}],"renderedRepresentation":"By Dave Philipps"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-2b8e7341-5edb-508e-81d3-079dc1d8f638\u002Fjob-1740708265750\u002Farticle-2b8e7341-5edb-508e-81d3-079dc1d8f638-job-1740708265750.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Number of Trans Troops Far Lower Than Estimated, Pentagon Figures Show"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vNmU5NmFmMWItNjUxNC01ZTg2LTllNmItNzY1ZWJhMzdmYTVi","length":127,"sourceId":"100000010018189","uri":"nyt:\u002F\u002Faudio\u002F6e96af1b-6514-5e86-9e6b-765eba37fa5b"}},"firstPublished":"2025-02-28T00:35:12.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Number of Trans Troops Far Lower Than Estimated, Pentagon Figures Show"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzJiOGU3MzQxLTVlZGItNTA4ZS04MWQzLTA3OWRjMWQ4ZjYzOA==","lastModified":"2025-02-28T02:03:23.518Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNWJmYTZhYmQtNGJkYS01OWM5LWFkYzQtMDhlNjNmZjJmZGE3"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010017949","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F2b8e7341-5edb-508e-81d3-079dc1d8f638","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrans-troops-pentagon-figures.html","wordCount":301}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T02:57:42.743Z","uri":"nyt:\u002F\u002Farticle\u002F2734f206-c837-5724-a971-94aedb7c0cc5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fiowa-transgender-civil-rights-bill.html"},{"__typename":"Article","lastModified":"2025-02-28T02:03:23.518Z","uri":"nyt:\u002F\u002Farticle\u002F2b8e7341-5edb-508e-81d3-079dc1d8f638","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrans-troops-pentagon-figures.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fbb2cc2fdac1f474e8fb28aa210c0478a","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"Image","altText":"People holding signs in protest. ","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Advocates for L.G.B.T.Q. rights said Iowa would become the first state to remove broad protections for transgender people if the governor signed the bill."},"captionOverride":null,"credit":"Rachel Mummey for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-square640.jpg","width":640},{"__typename":"ImageRendition","height":1801,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp\u002F27nat-iowa-transgender-01-htgp-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-27T22:27:33.044Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMDMxYmIxYWUtYzY4Ny01OGViLTg4YWUtMjU1ZmEyMDU4ZDFj","lastModified":"2025-02-27T22:27:33.044Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017809","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F031bb1ae-c687-58eb-88ae-255fa2058d1c","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F27nat-iowa-transgender-01-htgp.html"},{"__typename":"Image","altText":"Troops, with their backs turned to the camera, sit in chairs.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"The Trump administration has cited the cost of medical care as one of the reasons for banning transgender soldiers from serving."},"captionOverride":null,"credit":"Kaylee Greenlee for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F21\u002Fmultimedia\u002F21trump-news-trans-military-zqgf\u002F21trump-news-trans-military-zqgf-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T00:35:09.825Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNWJmYTZhYmQtNGJkYS01OWM5LWFkYzQtMDhlNjNmZjJmZGE3","lastModified":"2025-02-28T00:35:09.825Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010018109","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F5bfa6abd-4bda-59c9-adc4-08e63ff2fda7","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F21trump-news-trans-military-zqgf.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F031bb1ae-c687-58eb-88ae-255fa2058d1c\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Iowa Lawmakers Pass Bill to Eliminate Transgender Civil Rights Protections\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"If signed by Gov. Kim Reynolds, the Republican-backed measure would eliminate state civil rights protections for transgender Iowans.\",\"thread\":null,\"sentence\":\"Iowa Lawmakers Pass Bill to Eliminate Transgender Civil Rights Protections\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F2734f206-c837-5724-a971-94aedb7c0cc5\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F5bfa6abd-4bda-59c9-adc4-08e63ff2fda7\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Number of Trans Troops Is Far Lower Than Estimated, Pentagon Figures Show\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The Defense Department said 4,240 service members, or about 0.2 percent of those in uniform, have a diagnosis of gender dysphoria. Previous estimates had put the number at triple that figure.\",\"thread\":null,\"sentence\":\"Number of Trans Troops Far Lower Than Estimated, Pentagon Figures Show\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F2b8e7341-5edb-508e-81d3-079dc1d8f638\"}]}","sourceId":"a5fad8ff-1d6a-4a7b-aeb1-cea6c167225d","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fd5e3a1b6-b846-5cc8-9cc0-a51c1ddc12ee","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Interactive","bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Sarah Kliff","id":"UGVyc29uOm55dDovL3BlcnNvbi9lYzU1NDhlYS1kYjU4LTUxYWQtODA1Ny02ZjNjY2EzZGY5ODk=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F08\u002F25\u002Freader-center\u002Fauthor-sarah-kliff\u002Fauthor-sarah-kliff-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMzk0YzMzMzctZjg4Yi01YWFlLWFjZmQtMGM4MDMwNmQwNGVm"}},{"__typename":"Person","displayName":"Martín González Gómez","id":"UGVyc29uOm55dDovL3BlcnNvbi9kMjczNGFkMy1jMjE0LTUwZGItYjUyNy0zMmJkOTM5ODE0OWI=","promotionalMedia":null}],"renderedRepresentation":"By Sarah Kliff and Martín González Gómez"}],"firstPublished":"2025-02-28T02:28:13.000Z","id":"SW50ZXJhY3RpdmU6bnl0Oi8vaW50ZXJhY3RpdmUvMWUyODRkNDItMGYyZi01ODE0LWEzZDMtYWQ3ZmU4YTFmNGEx","interactiveWordCount":null,"lastModified":"2025-02-28T06:21:51.581Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-square640-v4.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-threeByTwoLargeAt2X-v3.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDM0MzgwZjgtZTRkYS01NTZiLTllODktNGQ1MmYyMmU5Mzdj"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010017384","tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Finteractive\u002F1e284d42-0f2f-5814-a3d3-ad7fe8a1f4a1","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmedicaid-enrollment.html"}],"children":[],"descendantAssets":[{"__typename":"Interactive","lastModified":"2025-02-28T06:21:51.581Z","uri":"nyt:\u002F\u002Finteractive\u002F1e284d42-0f2f-5814-a3d3-ad7fe8a1f4a1","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmedicaid-enrollment.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F69a999965c2546d1b04ba73cec514e8e","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-smallSquare252-v3.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-square640-v4.jpg","width":640},{"__typename":"ImageRendition","height":1000,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-mediumSquareAt3X-v4.jpg","width":1000}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-threeByTwoMediumAt2X-v4.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-threeByTwoSmallAt2X-v4.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-videoSixteenByNine1050-v3.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index\u002F2025-02-25-medicaid-enrollment-index-verticalTwoByThree735-v4.jpg","width":735}]}],"firstPublished":"2025-02-27T22:22:42.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDM0MzgwZjgtZTRkYS01NTZiLTllODktNGQ1MmYyMmU5Mzdj","lastModified":"2025-02-27T22:55:23.028Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017898","summary":"","timesTags":[{"__typename":"Subject","vernacular":"internal-dark-mode-incompatible-image"}],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fd34380f8-e4da-556b-9e89-4d52f22e937c","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F2025-02-25-medicaid-enrollment-index.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fd34380f8-e4da-556b-9e89-4d52f22e937c\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"More Than 70 Million Americans Are on Medicaid. This Is Where They Live.\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"As Republicans weigh deep cuts, these congressional districts — some red, some blue — have the most to lose.\",\"thread\":null,\"sentence\":\"More than 70 Million Americans Are on Medicaid. This Is Where They Live.\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Finteractive\u002F1e284d42-0f2f-5814-a3d3-ad7fe8a1f4a1\"}]}","sourceId":"0663bec9-b84a-4944-a0ff-4735a60228e7","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fcf153b12-9490-5e92-b4a2-19f2c0d16ab8","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderFullBleedVerticalBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Wesley Morris","id":"UGVyc29uOm55dDovL3BlcnNvbi84YWRlNjkwYS1jMGNkLTU1MDgtOTEyZi03OGRkOTBmODk5ZDA=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F06\u002F13\u002Fmultimedia\u002Fauthor-wesley-morris\u002Fauthor-wesley-morris-thumbLarge-v3.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjgzMjIzNGMtZTNhMi01OThjLTgyNWUtYWUyNDc5MTY3M2Fm"}}],"renderedRepresentation":"By Wesley Morris"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-61bfc8df-d91b-50a6-aecc-b9a82d7da8d9\u002Fjob-1740737023239\u002Farticle-61bfc8df-d91b-50a6-aecc-b9a82d7da8d9-job-1740737023239.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for There’s Something Weird About This Year’s Oscars"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vMDJjNDJjMDAtNzA2NC01NTZiLTk1MGMtNThlYWRkYzIxNTA1","length":759,"sourceId":"100000010019035","uri":"nyt:\u002F\u002Faudio\u002F02c42c00-7064-556b-950c-58eaddc21505"}},"firstPublished":"2025-02-28T10:03:42.174Z","headline":{"__typename":"CreativeWorkHeadline","default":"There’s Something Weird About This Year’s Oscars"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzYxYmZjOGRmLWQ5MWItNTBhNi1hZWNjLWI5YTgyZDdkYThkOQ==","lastModified":"2025-02-28T10:03:42.174Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmovies\u002Fmorris-oscars-lead\u002FoakImage-1740592246218-square640.png"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmovies\u002Fmorris-oscars-lead\u002FoakImage-1740592246218-threeByTwoLargeAt2X.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvODQ1YTliNzctYmIwMi01Y2QyLTk5MGQtZTQ0NGE2YjhjZjZm"}},"section":{"__typename":"Section","displayName":"Movies","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzYyYjNkNDcxLTRhZTUtNWFjMi04MzZmLWNiN2FkNTMxYzRjYg=="},"sourceId":"100000010012162","tone":"FEATURE","uri":"nyt:\u002F\u002Farticle\u002F61bfc8df-d91b-50a6-aecc-b9a82d7da8d9","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fbest-picture-nominees-oscars-conclave-wicked.html","wordCount":2202},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Gia Kourlas","id":"UGVyc29uOm55dDovL3BlcnNvbi9kMmY1ZWI3ZC02MzU1LTVhYTEtYTgwMC1lYTEzOWI5OGZkZmU=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F03\u002F21\u002Freader-center\u002Fauthor-gia-kourlas\u002Fauthor-gia-kourlas-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMWI3MGUxYWEtMDhmNi01MmIwLTg0OGQtNmQ4NGFkNzI3OWY0"}}],"renderedRepresentation":"By Gia Kourlas"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-dd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6\u002Fjob-1740736907837\u002Farticle-dd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6-job-1740736907837.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for In Zoe Saldaña, a Choreographer Finds His Dream Dancer"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vNjk2ZmJmNDItNTU1OS01NmEwLWIyODEtZjk3YjlmNTlmMTY0","length":300,"sourceId":"100000010019018","uri":"nyt:\u002F\u002Faudio\u002F696fbf42-5559-56a0-b281-f97b9f59f164"}},"firstPublished":"2025-02-28T10:01:46.736Z","headline":{"__typename":"CreativeWorkHeadline","default":"In Zoe Saldaña, a Choreographer Finds His Dream Dancer"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2RkOWU2ZjNjLThjM2MtNWMxZC04YjJjLWFiYTQwNGNlNWNkNg==","lastModified":"2025-02-28T10:01:46.736Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOWIxMGRmNWItOGU4NC01YTA0LThjOTAtOThmMzY3OWIyMGEx"}},"section":{"__typename":"Section","displayName":"Arts","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzZlNmVlMjkyLWI0YmQtNTAwNi1hNjE5LTljZWFiMDM1MjRmMg=="},"sourceId":"100000009998145","tone":"FEATURE","uri":"nyt:\u002F\u002Farticle\u002Fdd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Fdance\u002Fzoe-saldana-emilia-perez-dance.html","wordCount":839},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"James Wagner","id":"UGVyc29uOm55dDovL3BlcnNvbi9kMWNjY2Y5Mi1jMDM2LTU3NWQtYTUxMy1kMTFmMWJhMTBkMTA=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F06\u002F13\u002Fmultimedia\u002Fauthor-james-wagner\u002Fauthor-james-wagner-thumbLarge-v4.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDhhNzA1MDUtMWQyZC01YTFjLTg5NjYtZmNjYWJlYWI2YzBi"}}],"renderedRepresentation":"By James Wagner"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-b0813f6c-840e-5ae7-a88c-d5d430d3c564\u002Fjob-1740742763087\u002Farticle-b0813f6c-840e-5ae7-a88c-d5d430d3c564-job-1740742763087.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Why ‘Emilia Pérez,’ a Film About Mexico, Flopped in Mexico"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZTFhNTI0ZDAtZTk4My01YzA2LTk1NGMtNGIzODQ3NmUxYmQ1","length":529,"sourceId":"100000010019038","uri":"nyt:\u002F\u002Faudio\u002Fe1a524d0-e983-5c06-954c-4b38476e1bd5"}},"firstPublished":"2025-02-28T10:03:59.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Why ‘Emilia Pérez,’ a Film About Mexico, Flopped in Mexico"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2IwODEzZjZjLTg0MGUtNWFlNy1hODhjLWQ1ZDQzMGQzYzU2NA==","lastModified":"2025-02-28T11:39:21.985Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZjQxNTJhN2EtYjZhZC01YjFiLWE4YWMtZTM0NTUwZjE3NmYw"}},"section":{"__typename":"Section","displayName":"World","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzcwZTg2NWI2LWNjNzAtNTE4MS04NGM5LTgzNjhiM2E1YzM0Yg=="},"sourceId":"100000010013690","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Fb0813f6c-840e-5ae7-a88c-d5d430d3c564","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Femilia-perez-mexico-oscars.html","wordCount":1348}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T10:03:42.174Z","uri":"nyt:\u002F\u002Farticle\u002F61bfc8df-d91b-50a6-aecc-b9a82d7da8d9","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fbest-picture-nominees-oscars-conclave-wicked.html"},{"__typename":"Article","lastModified":"2025-02-28T10:01:46.736Z","uri":"nyt:\u002F\u002Farticle\u002Fdd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Fdance\u002Fzoe-saldana-emilia-perez-dance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:39:21.985Z","uri":"nyt:\u002F\u002Farticle\u002Fb0813f6c-840e-5ae7-a88c-d5d430d3c564","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Femilia-perez-mexico-oscars.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F83ddc54a1441482683de0b656ee9bd71","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"EmbeddedInteractive","bylines":[],"compatibility":"INLINE","credit":"","displayProperties":{"__typename":"CreativeWorkDisplayProperties","displayOverrides":["DARK_MODE_COMPATIBLE"]},"firstPublished":"2023-03-09T21:32:39.000Z","html":"\u003Cnav aria-labelledby=\"styln-academy-awards-hp-menu\" class=\"css-qvlk0g\"\u003E\u003Cp id=\"styln-academy-awards-hp-menu\"\u003E\u003Cspan class=\"css-14sajpe\"\u003EOscars 2025\u003C\u002Fspan\u003E\u003C\u002Fp\u003E\u003Cul class=\"css-11xr2bo\"\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F01\u002F23\u002Fmovies\u002Foscar-nominees-full-list.html\"\u003ENominees List\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F26\u002Fmovies\u002Foscar-nominees-dinner-photos.html\"\u003EPhotos: Nominees Dinner\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F12\u002Fmovies\u002Foscars-race-emilia-perez-anora.html\"\u003EWhere the Race Stands\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F26\u002Fmagazine\u002Ftimothee-chalamet-oscar-campaign.html\"\u003ETimothée Chalamet’s Campaign\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003Cli class=\"css-wc84xp\"\u003E\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F01\u002F23\u002Fmovies\u002Foscar-nominees-movies-streaming.html\"\u003EWhere to Stream\u003C\u002Fa\u003E\u003C\u002Fli\u003E\u003C\u002Ful\u003E\u003Cscript\u003E\"use strict\";(function(){\u002F\u002F prevent menus from overflowing into right rail on desktop\nfunction a(){var a=document.querySelector(\"nav[aria-labelledby=\\\"styln-academy-awards-hp-menu\\\"]\"),b=document.querySelectorAll(\"[data-hierarchy=\\\"zone\\\"]\"),c=Array.from(b).filter(function(b){return b.contains(a)})[0];if(c&&a.clientWidth\u003Ec.clientWidth)\u002F\u002F remove the last item from the menu if it is overflowing\n{var d=a.querySelectorAll(\"li\"),e=d[d.length-1];e.remove()}}\u002F\u002F only check for overflow if the user is on desktop or tablet\n\u002F\u002F remove links that match ones already shown in the live band\nvar b=document.querySelector(\"#hp-live-band-list\");if(b){var c=Array.from(b.querySelectorAll(\"a\")).map(function(b){return b.href}),d=document.querySelectorAll(\"nav[aria-labelledby=\\\"styln-academy-awards-hp-menu\\\"] li\");d.forEach(function(a){var b,d=null===(b=a.querySelector(\"a\"))||void 0===b?void 0:b.pathname;d&&c.forEach(function(b){b.includes(d)&&a.remove()})})}if(!window.matchMedia(\"(pointer: coarse) and (max-width: 739px)\").matches){a();var e;window.addEventListener(\"resize\",function(){clearTimeout(e),e=setTimeout(a,250)})}})();\u003C\u002Fscript\u003E\u003C\u002Fnav\u003E","id":"RW1iZWRkZWRJbnRlcmFjdGl2ZTpueXQ6Ly9lbWJlZGRlZGludGVyYWN0aXZlL2QwNGI3MjZhLTY5MzktNTkxMS04YmVmLTYzMDQ4NjVhZTE3Yg==","lastModified":"2025-02-27T12:55:20.914Z","promotionalImage":null,"promotionalMedia":null,"section":{"__typename":"Section","displayName":"Admin","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q0MmQzYTNmLTAyYTgtNWRlNy1hOTEyLTUyMGZhNmI0NGY0ZQ=="},"slug":"prism-styln-academy-awards-2023-homepageMenu-1678397502869","sourceId":"100000008804928","storyFormat":null,"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fembeddedinteractive\u002Fd04b726a-6939-5911-8bef-6304865ae17b","url":"null"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Searchlight Pictures; Mubi; Netflix","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00morris-hp-promo\u002F00morris-hp-promo-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00morris-hp-promo\u002F00morris-hp-promo-square640.jpg","width":640},{"__typename":"ImageRendition","height":1080,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00morris-hp-promo\u002F00morris-hp-promo-mediumSquareAt3X.jpg","width":1080}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00morris-hp-promo\u002F00morris-hp-promo-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00morris-hp-promo\u002F00morris-hp-promo-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00morris-hp-promo\u002F00morris-hp-promo-videoSixteenByNine1050.jpg","width":1050}]}],"firstPublished":"2025-02-27T22:03:05.978Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOGFlZWM3YjItNGI3MS01MjA0LWFiMDMtOTJmNTY2ODc0Y2M4","lastModified":"2025-02-27T22:03:05.978Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017834","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F8aeec7b2-4b71-5204-ab03-92f566874cc8","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00morris-hp-promo.html"},{"__typename":"Image","altText":"A woman in jeans and a T-shirt dances on a table, her hair flying. Choreographers watch.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Zoe Saldaña rehearsing “Emilia Pérez” with the choreographer Damien Jalet, left, and Gabriela Ceceña, a choreographic assistant."},"captionOverride":null,"credit":"Netflix","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl\u002F28saldana-spotlight-01-bmzl-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T10:01:44.161Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOWIxMGRmNWItOGU4NC01YTA0LThjOTAtOThmMzY3OWIyMGEx","lastModified":"2025-02-28T10:01:44.161Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017707","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F9b10df5b-8e84-5a04-8c90-98f3679b20a1","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28saldana-spotlight-01-bmzl.html"},{"__typename":"Image","altText":"The inside of a movie theater with a poster for the film “Emilia Perez” at left.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"“Emilia Pérez” wasn’t theatrically released in Mexico until Jan. 23 — five months after its debut in France and two months after its U.S. release."},"captionOverride":null,"credit":"Alfredo Estrella\u002FAgence France-Presse — Getty Images","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-square640.jpg","width":640},{"__typename":"ImageRendition","height":1340,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-mediumSquareAt3X.jpg","width":1340}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz\u002F28mexico-emiliaperez-mbtz-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T10:03:56.246Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZjQxNTJhN2EtYjZhZC01YjFiLWE4YWMtZTM0NTUwZjE3NmYw","lastModified":"2025-02-28T10:03:56.246Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010016831","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Ff4152a7a-b6ad-5b1b-a8ac-e34550f176f0","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28mexico-emiliaperez-mbtz.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":\"nyt:\u002F\u002Fembeddedinteractive\u002Fd04b726a-6939-5911-8bef-6304865ae17b\",\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"showCaption\":false,\"mutedAutoplayOverlay\":false,\"headline\":\"\",\"mobileAspectRatioCrop\":\"SQUARE\",\"uri\":\"nyt:\u002F\u002Fimage\u002F8aeec7b2-4b71-5204-ab03-92f566874cc8\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"There’s Something Weird About This Year’s Oscars\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Or with the 10 best picture nominees, at least, a critic writes. But what seems clear is that Hollywood is celebrating the kinds of movies it doesn’t make.\",\"thread\":null,\"sentence\":\"There’s Something Weird About This Year’s Oscars\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F61bfc8df-d91b-50a6-aecc-b9a82d7da8d9\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F9b10df5b-8e84-5a04-8c90-98f3679b20a1\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"In Zoe Saldaña, a Choreographer for ‘Emilia Pérez’ Finds His Dream Dancer\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The Academy Award-nominated actress discovers her inner dancer in “Emilia Pérez” with the help of the choreographer Damien Jalet.\",\"thread\":null,\"sentence\":\"In Zoe Saldaña, a Choreographer Finds His Dream Dancer\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fdd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Ff4152a7a-b6ad-5b1b-a8ac-e34550f176f0\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Why ‘Emilia Pérez,’ a Film About Mexico, Flopped in Mexico\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The polarizing movie is up for 13 Academy Awards on Sunday. But in Mexico, it has been widely criticized for its depiction of the country.\",\"thread\":null,\"sentence\":\"Why ‘Emilia Pérez,’ a Film About Mexico, Flopped in Mexico\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fb0813f6c-840e-5ae7-a88c-d5d430d3c564\"}]}","sourceId":"dbde0cbc-6254-4c07-a4b9-50c418e2b596","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fff9781ea-e8ce-5bd0-b845-5ee4c0ada044","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"William K. Rashbaum","id":"UGVyc29uOm55dDovL3BlcnNvbi85OGM2Mzg2ZC0wMDRmLTVjNmItYTkzZC1jMGQxNzI5ZTY4ODY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F06\u002F13\u002Fmultimedia\u002Fauthor-william-k-rashbaum\u002Fauthor-william-k-rashbaum-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvODJiY2RjN2MtNTY4Ni01NTkzLWFmM2ItNTJkOWExOGMxMjcx"}},{"__typename":"Person","displayName":"Michael Rothfeld","id":"UGVyc29uOm55dDovL3BlcnNvbi8wNDc0MzkwYS1lNzk4LTU1YmUtOGM4Mi1kMjM2YjkxNWFjNTU=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F05\u002F11\u002Freader-center\u002Fauthor-michael-rothfeld\u002Fauthor-michael-rothfeld-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvODljYWE0NGMtZjJiZS01NzQyLTk5ODQtNjRjOWZiMTc3NzYy"}},{"__typename":"Person","displayName":"Dana Rubinstein","id":"UGVyc29uOm55dDovL3BlcnNvbi8yYTUwNjhjNy1jZjIyLTVmZjAtYTUwOS02NWFjMzE5ZGViYjA=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F06\u002F23\u002Freader-center\u002Fauthor-dana-rubinstein\u002Fauthor-dana-rubinstein-thumbLarge-v3.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjliM2VkMzQtZTMzMi01NjZkLTliYzItOGNkYzMzNWQ5Yjc5"}}],"renderedRepresentation":"By William K. Rashbaum, Michael Rothfeld and Dana Rubinstein"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-eae58626-ae12-58cf-a801-1c30238d52ed\u002Fjob-1740729628148\u002Farticle-eae58626-ae12-58cf-a801-1c30238d52ed-job-1740729628148.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Amid Furor Over Adams Case, a Glimpse of a Charge Never Brought"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vMGEwMDQzOWItNzg4OS01ZmY1LWJjYzktNjFhYmNkODY2YjIy","length":473,"sourceId":"100000010018641","uri":"nyt:\u002F\u002Faudio\u002F0a00439b-7889-5ff5-bcc9-61abcd866b22"}},"firstPublished":"2025-02-28T08:00:07.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Amid Furor Over Adams Case, a Glimpse of a Charge Never Brought"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2VhZTU4NjI2LWFlMTItNThjZi1hODAxLTFjMzAyMzhkNTJlZA==","lastModified":"2025-02-28T11:29:28.060Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNzk3Yzc1NmMtMzA4Yi01MmU0LThkMzAtNWEzNjVlMWM2ZDFh"}},"section":{"__typename":"Section","displayName":"New York","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzM5NDgwMzc0LTY2ZDMtNTYwMy05Y2UxLTU4Y2ZhMTI5ODhlMg=="},"sourceId":"100000010011265","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Feae58626-ae12-58cf-a801-1c30238d52ed","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fadams-corruption-new-charge.html","wordCount":1299},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Ginia Bellafante","id":"UGVyc29uOm55dDovL3BlcnNvbi9lZDBlNmNmOC02YTZhLTUzM2ItODY2Yy0zNTlkOWU0MzE0NTk=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F12\u002F06\u002Fmultimedia\u002Fauthor-ginia-bellafante\u002Fauthor-ginia-bellafante-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYmJjODJiMzMtODYyMy01ZjQ5LWFiNDctMTY0OGU0NzExOTU2"}}],"renderedRepresentation":"By Ginia Bellafante"}],"featuredAudio":null,"firstPublished":"2025-02-28T08:00:09.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"If Cuomo Became Mayor, Would He Really Stand Up to Trump?"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzJmOTM5MGU4LTYwN2ItNTFhMC1iM2VhLTQ3YmUyZjU4MjFkYw==","lastModified":"2025-02-28T10:13:56.828Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTgzZmY1YjgtMjA5ZC01OTNiLThmNGQtNzZhOTIwYjdjZmQx"}},"section":{"__typename":"Section","displayName":"New York","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzM5NDgwMzc0LTY2ZDMtNTYwMy05Y2UxLTU4Y2ZhMTI5ODhlMg=="},"sourceId":"100000010013632","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F2f9390e8-607b-51a0-b3ea-47be2f5821dc","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fandrew-cuomo-new-york-mayor.html","wordCount":1063}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:29:28.060Z","uri":"nyt:\u002F\u002Farticle\u002Feae58626-ae12-58cf-a801-1c30238d52ed","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fadams-corruption-new-charge.html"},{"__typename":"Article","lastModified":"2025-02-28T10:13:56.828Z","uri":"nyt:\u002F\u002Farticle\u002F2f9390e8-607b-51a0-b3ea-47be2f5821dc","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fandrew-cuomo-new-york-mayor.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fadcd1ce0f04443729a4694a22785757d","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"Image","altText":"A close-up of the face of Mayor Eric Adams.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Manhattan’s top federal prosecutor revealed earlier this month that her office was prepared to seek an additional charge against Mayor Eric Adams, who already was accused of bribery and corruption."},"captionOverride":null,"credit":"Graham Dickie\u002FThe New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002Fadams-obstruction-lbkc\u002Fadams-obstruction-lbkc-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T08:00:05.190Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNzk3Yzc1NmMtMzA4Yi01MmU0LThkMzAtNWEzNjVlMWM2ZDFh","lastModified":"2025-02-28T08:00:05.190Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010015304","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F797c756c-308b-52e4-8d30-5a365e1c6d1a","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002Fadams-obstruction-lbkc.html"},{"__typename":"Image","altText":"Andrew Cuomo, wearing a black suit and purple tie, speaks to members of the news media through the driver’s side window of a car.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Andrew Cuomo leads in the polls even though he has yet to officially enter the New York City mayor’s race."},"captionOverride":null,"credit":"Victor J. Blue for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fnyregion\u002F02big-vqkb\u002F28big-vqkb-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T08:00:07.384Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTgzZmY1YjgtMjA5ZC01OTNiLThmNGQtNzZhOTIwYjdjZmQx","lastModified":"2025-02-28T08:00:07.384Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"New York","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzM5NDgwMzc0LTY2ZDMtNTYwMy05Y2UxLTU4Y2ZhMTI5ODhlMg=="},"sourceId":"100000010016754","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fa83ff5b8-209d-593b-8f4d-76a920b7cfd1","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fnyregion\u002F02big-vqkb.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F797c756c-308b-52e4-8d30-5a365e1c6d1a\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Amid Furor Over Adams Case, a Glimpse of a Charge Never Brought\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Prosecutors said they had proposed a new charge against Mayor Eric Adams of New York. Court filings suggest it related to the conduct of an aide.\",\"thread\":null,\"sentence\":\"Amid Furor Over Adams Case, a Glimpse of a Charge Never Brought\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Feae58626-ae12-58cf-a801-1c30238d52ed\"},{\"showMedia\":false,\"media\":{\"headline\":\"\",\"uri\":\"nyt:\u002F\u002Fimage\u002Fa83ff5b8-209d-593b-8f4d-76a920b7cfd1\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"If Cuomo Became Mayor, Would He Really Stand Up to Trump?\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Behind the perception of Andrew Cuomo as “Mr. Tough Guy” is the reality: The former governor has rarely criticized President Trump by name, our columnist writes.\",\"thread\":null,\"sentence\":\"If Cuomo Became Mayor, Would He Really Stand Up to Trump?\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F2f9390e8-607b-51a0-b3ea-47be2f5821dc\"}]}","sourceId":"12f85400-a106-479a-ac47-7f13329141d1","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fb0b76c4a-9d31-504d-9d29-f4ae296dbf33","url":"","variantAllocation":null},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Jordyn Holman","id":"UGVyc29uOm55dDovL3BlcnNvbi80YjAwZTU1OC04MDkxLTU4YTMtYjBlMS1mYTY4NDdmMDRiNTg=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F10\u002F06\u002Freader-center\u002Fauthor-jordyn-holman\u002Fauthor-jordyn-holman-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMDI5Y2FiYTMtNDAzMS01OTUzLWJmMWYtMmI2ODE3MDdhYTM2"}},{"__typename":"Person","displayName":"Aimee Ortiz","id":"UGVyc29uOm55dDovL3BlcnNvbi83Mjg5YTMyNS04M2NhLTU5NjYtYTU3Zi1iN2IyODk0MjdhM2E=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F11\u002F11\u002Freader-center\u002Fauthor-aimee-ortiz\u002Fauthor-aimee-ortiz-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjM4ZmJlYjgtMTlhOS01ZTViLWJlYmQtYTk5ZmU0MzQxODYx"}}],"renderedRepresentation":"By Jordyn Holman and Aimee Ortiz"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-f04154fe-aaa0-5b58-bdd7-66b4439e06e5\u002Fjob-1740736840080\u002Farticle-f04154fe-aaa0-5b58-bdd7-66b4439e06e5-job-1740736840080.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for What Does It Take to Quit Shopping? Mute, Delete and Unsubscribe."},"id":"QXVkaW86bnl0Oi8vYXVkaW8vYWNjM2Y0ZGUtMWUwNS01OGY1LWFlNzctNDQ5NTQwYjVlYTc4","length":666,"sourceId":"100000010019011","uri":"nyt:\u002F\u002Faudio\u002Facc3f4de-1e05-58f5-ae77-449540b5ea78"}},"firstPublished":"2025-02-28T10:00:22.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"What Does It Take to Quit Shopping? Mute, Delete and Unsubscribe."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2YwNDE1NGZlLWFhYTAtNWI1OC1iZGQ3LTY2YjQ0MzllMDZlNQ==","lastModified":"2025-02-28T10:10:47.390Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjY3Y2RlYmItY2Q0ZC01Nzc3LWE0NzktNTdlYjNjYTZjMTBh"}},"section":{"__typename":"Section","displayName":"Business","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzA0MTViMmIwLTUxM2EtNWU3OC04MGRhLTIxYWI3NzBjYjc1Mw=="},"sourceId":"100000009973928","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Ff04154fe-aaa0-5b58-bdd7-66b4439e06e5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fshopping-addiction-no-buy-2025.html","wordCount":1847}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T10:10:47.390Z","uri":"nyt:\u002F\u002Farticle\u002Ff04154fe-aaa0-5b58-bdd7-66b4439e06e5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fshopping-addiction-no-buy-2025.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F0431ae4600bc4244b9315686c0a53afc","hierarchyType":"package","isPersonalized":false,"layoutType":"HighlightLevel1","media":[{"__typename":"Image","altText":"Cassandra Orakpo in a denim dress holds a phone in front of four shelves filled with perfume bottles and a rack of clothes behind her.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Cassandra Orakpo keeps at least 80 bottles of perfume in a closet. She pledged this year to slow down her buying habit."},"captionOverride":null,"credit":"Michael Stravato for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-square640.jpg","width":640},{"__typename":"ImageRendition","height":1799,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":999,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F18\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp\u002F00shopaholics-orakpo-kbqp-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T10:00:19.234Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjY3Y2RlYmItY2Q0ZC01Nzc3LWE0NzktNTdlYjNjYTZjMTBh","lastModified":"2025-02-28T10:00:19.234Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000009998208","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F267cdebb-cd4d-5777-a479-57eb3ca6c10a","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F00shopaholics-orakpo-kbqp.html"}],"personalizationConfig":null,"properties":"{\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isBundle\":false,\"assets\":[{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F267cdebb-cd4d-5777-a479-57eb3ca6c10a\"},\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"display\":\"SUMMARY\",\"kicker\":null,\"headline\":\"What Does It Take to Quit Shopping? Mute, Delete and Unsubscribe.\",\"summary\":\"Marketers followed consumers to social media and their phones. “Low Buy 2025” influencers are sharing tips for how to resist them.\",\"bullets\":[\"\",\"\"],\"style\":\"STANDARD_LIGHT\",\"position\":\"LEFT\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Ff04154fe-aaa0-5b58-bdd7-66b4439e06e5\"}]}","sourceId":"38ce04b8-598a-478d-9ff3-4acba5ac77ec","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fff3c5678-03e6-5ce0-8c90-3780092b4c64","url":"","variantAllocation":null}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:30:16.929Z","uri":"nyt:\u002F\u002Farticle\u002F720399f2-c1ec-5608-9963-cfaa03abd183","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fasia\u002Fchina-military-drills-pacific.html"},{"__typename":"Article","lastModified":"2025-02-28T10:33:26.206Z","uri":"nyt:\u002F\u002Farticle\u002Ff86860c9-bc29-598c-bc53-5b81b1def8a5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fwest-bank-jenin-palestinian-authority-israel.html"},{"__typename":"Article","lastModified":"2025-02-28T11:02:22.739Z","uri":"nyt:\u002F\u002Farticle\u002Feed2db6f-2fad-5bf8-b96f-b8ad776652ff","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fsyria-ramadan-economy-cash-shortage.html"},{"__typename":"Article","lastModified":"2025-02-28T02:57:42.743Z","uri":"nyt:\u002F\u002Farticle\u002F2734f206-c837-5724-a971-94aedb7c0cc5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fiowa-transgender-civil-rights-bill.html"},{"__typename":"Article","lastModified":"2025-02-28T02:03:23.518Z","uri":"nyt:\u002F\u002Farticle\u002F2b8e7341-5edb-508e-81d3-079dc1d8f638","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrans-troops-pentagon-figures.html"},{"__typename":"Interactive","lastModified":"2025-02-28T06:21:51.581Z","uri":"nyt:\u002F\u002Finteractive\u002F1e284d42-0f2f-5814-a3d3-ad7fe8a1f4a1","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmedicaid-enrollment.html"},{"__typename":"Article","lastModified":"2025-02-28T10:03:42.174Z","uri":"nyt:\u002F\u002Farticle\u002F61bfc8df-d91b-50a6-aecc-b9a82d7da8d9","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fbest-picture-nominees-oscars-conclave-wicked.html"},{"__typename":"Article","lastModified":"2025-02-28T10:01:46.736Z","uri":"nyt:\u002F\u002Farticle\u002Fdd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Fdance\u002Fzoe-saldana-emilia-perez-dance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:39:21.985Z","uri":"nyt:\u002F\u002Farticle\u002Fb0813f6c-840e-5ae7-a88c-d5d430d3c564","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Femilia-perez-mexico-oscars.html"},{"__typename":"Article","lastModified":"2025-02-28T11:29:28.060Z","uri":"nyt:\u002F\u002Farticle\u002Feae58626-ae12-58cf-a801-1c30238d52ed","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fadams-corruption-new-charge.html"},{"__typename":"Article","lastModified":"2025-02-28T10:13:56.828Z","uri":"nyt:\u002F\u002Farticle\u002F2f9390e8-607b-51a0-b3ea-47be2f5821dc","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fandrew-cuomo-new-york-mayor.html"},{"__typename":"Article","lastModified":"2025-02-28T10:10:47.390Z","uri":"nyt:\u002F\u002Farticle\u002Ff04154fe-aaa0-5b58-bdd7-66b4439e06e5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fshopping-addiction-no-buy-2025.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F3c82ec400d5f4724855f42804ffeb966","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"97c0caab-9ed3-4d99-acd6-741a732ca0fd","trackingName":"More Top Stories","uri":"nyt:\u002F\u002Fprogrammingnode\u002F7a8e3bad-2085-57d7-886e-226abdb83758","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F5e0e97b63b704d5ea299eb809ddf5904","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"290ceb59-f95b-45e2-b634-5e415d5a4155","trackingName":"Reordered More Top Stories","uri":"nyt:\u002F\u002Fprogrammingnode\u002F23a53ec4-f82b-5643-9981-d9ff73f8fd66","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"EmbeddedInteractive","bylines":[],"compatibility":"INLINE","credit":"","displayProperties":{"__typename":"CreativeWorkDisplayProperties","displayOverrides":["DARK_MODE_COMPATIBLE","HIDE_HEADER"]},"firstPublished":"2024-07-25T16:10:31.000Z","html":"\u003C!-- birdkit: do not modify this file --\u003E\n\u003Chead\u003E\n\t\n\t\t\n\t\t\n\t\t\n\t\t\n\t\t\n\t\t\n\u003C\u002Fhead\u003E\n\n\t\t\u003Cdiv\n\t\t\tid=\"g-weather-hp-strip\"\n\t\t\tclass=\"birdkit-body g-weather-hp-strip\"\n\t\t\tdata-preview-slug=\"weather-hp-strip\"\n\t\t\tdata-birdkit-hydrate=\"b1b90d249ea4f59d\"\n\t\t\u003E\t\n\t\t\t\n\t \u003Cdiv class=\"weather-hp-strip-grid s-zxpogMpJrieb\"\u003E\u003Cdiv class=\"g-screenreader-only s-zxpogMpJrieb\"\u003E\u003Ch2 class=\"g-screenreader\" data-svelte-h=\"svelte-yjtbeg\"\u003EWeather\u003C\u002Fh2\u003E\u003C\u002Fdiv\u003E \u003Cdiv class=\"modules_wrapper modules_mobile s-IKV1CDMPALxt\"\u003E\u003Cdiv class=\"weather-card-container s-IKV1CDMPALxt\"\u003E\u003Carticle class=\"loading-card s-wKKTKMWUBRLJ\"\u003E \u003Cdiv class=\"loading-header s-wKKTKMWUBRLJ\"\u003E\u003C\u002Fdiv\u003E \u003Cdiv class=\"loading-body s-wKKTKMWUBRLJ\"\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003C\u002Fdiv\u003E \u003Cdiv class=\"loading-credit s-wKKTKMWUBRLJ\"\u003E\u003C\u002Fdiv\u003E \u003C\u002Farticle\u003E \u003C\u002Fdiv\u003E\u003Cdiv class=\"weather-card-container s-IKV1CDMPALxt\"\u003E\u003Carticle class=\"loading-card s-wKKTKMWUBRLJ\"\u003E \u003Cdiv class=\"loading-header s-wKKTKMWUBRLJ\"\u003E\u003C\u002Fdiv\u003E \u003Cdiv class=\"loading-body s-wKKTKMWUBRLJ\"\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003C\u002Fdiv\u003E \u003Cdiv class=\"loading-credit s-wKKTKMWUBRLJ\"\u003E\u003C\u002Fdiv\u003E \u003C\u002Farticle\u003E \u003C\u002Fdiv\u003E\u003Cdiv class=\"weather-card-container s-IKV1CDMPALxt\"\u003E\u003Carticle class=\"loading-card s-wKKTKMWUBRLJ\"\u003E \u003Cdiv class=\"loading-header s-wKKTKMWUBRLJ\"\u003E\u003C\u002Fdiv\u003E \u003Cdiv class=\"loading-body s-wKKTKMWUBRLJ\"\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003Cdiv class=\"loading-row\"\u003E\u003C\u002Fdiv\u003E\u003C\u002Fdiv\u003E \u003Cdiv class=\"loading-credit s-wKKTKMWUBRLJ\"\u003E\u003C\u002Fdiv\u003E \u003C\u002Farticle\u003E \u003C\u002Fdiv\u003E\u003C\u002Fdiv\u003E \u003Cdiv class=\"wea-nav-container s-IKV1CDMPALxt\"\u003E \u003C\u002Fdiv\u003E \u003C\u002Fdiv\u003E \n\t\t\t\n\t\t\t\u003Cscript\u003E\n\t\t\t\t{\n\t\t\t\t\t__sveltekit_1r4ocwm = {\n\t\t\t\t\t\tbase: new URL(\".\", location).pathname.slice(0, -1),\n\t\t\t\t\t\tenv: {\"PUBLIC_BIRDKIT_CSS_SCOPED_CLASS\":\"g-weather-hp-strip\",\"PUBLIC_BIRDKIT_PROJECT_SLUG\":\"weather-hp-strip\"}\n\t\t\t\t\t};\n\n\t\t\t\t\tconst element = document.querySelector('[data-birdkit-hydrate=\"b1b90d249ea4f59d\"]');\n\n\t\t\t\t\tconst data = [null,{\"type\":\"data\",\"data\":{body:[{type:\"svelte\",value:{component:\"WeatherModules\",marginInline:false,maxWidth:\"1000px\",wrapper:false,theme:\"v1\",modules:[{module:\"WeatherCardToday\",use_overrides:false,props:{credit:\"Source: Tomorrow.io\"}},{module:\"WeatherCardWindChill\",use_overrides:false,props:{}},{module:\"WeatherCardRisk\",use_overrides:false,hazard_type:\"wssi\",props:{}},{module:\"WeatherCardAirQuality\",use_overrides:false,props:{}}]}}],theme:\"news\",sheets:{},scoopProps:{uri:\"nyt:\u002F\u002Fembeddedinteractive\u002F557659e5-f688-5fe8-bdc0-ed9d03c1fa1e\",slug:\"weather-hp-strip-hp-strip\"}},\"uses\":{\"url\":1}}];\n\n\t\t\t\t\tPromise.all([\n\t\t\t\t\t\timport(\"https:\u002F\u002Fstatic01.nytimes.com\u002Fnewsgraphics\u002Fweather-hp-strip\u002Fcb02c00a-e5f5-41fb-b9ab-85ea8382b647\u002F_assets\u002F_app\u002Fimmutable\u002Fentry\u002Fstart.6f861287.js\"),\n\t\t\t\t\t\timport(\"https:\u002F\u002Fstatic01.nytimes.com\u002Fnewsgraphics\u002Fweather-hp-strip\u002Fcb02c00a-e5f5-41fb-b9ab-85ea8382b647\u002F_assets\u002F_app\u002Fimmutable\u002Fentry\u002Fapp.21f3107c.js\")\n\t\t\t\t\t]).then(([kit, app]) =\u003E {\n\t\t\t\t\t\tkit.start(app, element, {\n\t\t\t\t\t\t\tnode_ids: [0, 3],\n\t\t\t\t\t\t\tdata,\n\t\t\t\t\t\t\tform: null,\n\t\t\t\t\t\t\terror: null,\n\t\t\t\t\t\t\tparams: {},\n\t\t\t\t\t\t\troute: {\"id\":\"\u002Fhp-strip\"}\n\t\t\t\t\t\t});\n\t\t\t\t\t});\n\t\t\t\t}\n\t\t\t\u003C\u002Fscript\u003E\n\t\t\n\n\t\t\u003C\u002Fdiv\u003E\n\t\t\n","id":"RW1iZWRkZWRJbnRlcmFjdGl2ZTpueXQ6Ly9lbWJlZGRlZGludGVyYWN0aXZlLzU1NzY1OWU1LWY2ODgtNWZlOC1iZGMwLWVkOWQwM2MxZmExZQ==","lastModified":"2025-02-25T13:43:51.656Z","promotionalImage":null,"promotionalMedia":null,"section":{"__typename":"Section","displayName":"Weather","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q0MDczNDY1LWY5MDEtNTcwMS1iZGJmLTRjZGVmZTM4Mzc1Yw=="},"slug":"weather-hp-strip","sourceId":"100000009592393","storyFormat":null,"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F557659e5-f688-5fe8-bdc0-ed9d03c1fa1e","url":"null"}],"children":[],"descendantAssets":[{"__typename":"EmbeddedInteractive","lastModified":"2025-02-25T13:43:51.656Z","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F557659e5-f688-5fe8-bdc0-ed9d03c1fa1e","url":"null"}],"eventId":"pubp:\u002F\u002Fevent\u002F4f705fd46fe54b05b736e5382b373a36","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":null,\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":false,\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":null,\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":null,\"thread\":null,\"sentence\":null,\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Fembeddedinteractive\u002F557659e5-f688-5fe8-bdc0-ed9d03c1fa1e\"}]}","sourceId":"b66615c9-2b45-442f-8c3a-03ebe6e63809","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F9637d990-f8ee-5ef1-b9e6-bfb395b77e5d","url":"","variantAllocation":null}],"descendantAssets":[{"__typename":"EmbeddedInteractive","lastModified":"2025-02-25T13:43:51.656Z","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F557659e5-f688-5fe8-bdc0-ed9d03c1fa1e","url":"null"}],"eventId":"pubp:\u002F\u002Fevent\u002F9a84e36744344d41beeeff7c0b2754e3","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"c160f54d-535a-4773-bbad-b8a4309c6a67","trackingName":"Weather","uri":"nyt:\u002F\u002Fprogrammingnode\u002Fb55a96f5-c2f9-5377-8de1-252208985d37","url":"","variantAllocation":null,"assets":undefined,"media":undefined}],"hierarchyType":"zone","trackingName":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNodeEmbedded","children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fcd50dd4498b6403290ff59f6e8485b79","hierarchyType":"addOn","isPersonalized":true,"layoutType":"Carousel2","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":4,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002F9a70b5e5-9f3d-5095-8e74-04d1941c3578","surface":"home-packages-rightrail-addon"},"properties":"{\"isOpinion\":false,\"isBundle\":false,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"HEADLINE\",\"mediaEmphasis\":\"SMALL\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":null,\"assets\":[]}","sourceId":"4e061504-f27e-4b49-9b1d-5dce09b65289","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F279ec7a1-5d8e-5353-82ee-cada8b9d35a0","url":"","variantAllocation":null,"children":[]}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fef1bf7cfa22f4088a3230ff5e8dfa6aa","hierarchyType":"dropzone","sourceId":"fdcba73a-bc9a-4e78-84c0-424fd772c55bdefault","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F014ca7a4-d0b0-5365-a0bb-bd9abccc65c8","url":"","variantAllocation":null,"zoneKey":"default","assets":undefined,"media":undefined}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fa597b5ec2c754b90b37783787460f3ef","hierarchyType":"package","isPersonalized":true,"layoutType":"HighlightLevel1","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":1,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002Fe6fefa52-1c59-5d8d-a635-bb206a9edf57","surface":"home-packages-rightrail-primary"},"properties":"{\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":null,\"isBundle\":false,\"assets\":[]}","sourceId":"36e29982-49cf-453f-9d29-c48bff7fb8a9","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F8d50cc79-d57b-5218-9103-ba22a1eb4a88","url":"","variantAllocation":null}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F59f23ba36e5f4a2fb7fb56d7ac8a3027","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"2ad10b6b-9784-45a2-8ae9-5e25ab984026","trackingName":"Right Rail","uri":"nyt:\u002F\u002Fprogrammingnode\u002F2ee7f3a7-10c2-5888-b567-1886488e22a2","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ListBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"The Editorial Board","id":"UGVyc29uOm55dDovL3BlcnNvbi9jYjVhYmEzYS0wN2E2LTVlZjctODVlMC05ZDdhODYxOTc5YmY=","promotionalMedia":null}],"renderedRepresentation":"By The Editorial Board"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-351b30ec-12d0-5d59-9f63-5d81a895ded1\u002Fjob-1740737039361\u002Farticle-351b30ec-12d0-5d59-9f63-5d81a895ded1-job-1740737039361.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for The MAGA War on Speech"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vODI5YmU1YzQtYzFlYS01ODc4LWI2YWEtNGE2ZmE1N2Y3NWI0","length":800,"sourceId":"100000010019039","uri":"nyt:\u002F\u002Faudio\u002F829be5c4-c1ea-5878-b6aa-4a6fa57f75b4"}},"firstPublished":"2025-02-28T10:03:58.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"The MAGA War on Speech"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzM1MWIzMGVjLTEyZDAtNWQ1OS05ZjYzLTVkODFhODk1ZGVkMQ==","lastModified":"2025-02-28T12:01:50.458Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNDA4MjExZTEtMmFhZi01YzRkLWFiMzQtMjE4MjJhOTllZTg0"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010013695","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002F351b30ec-12d0-5d59-9f63-5d81a895ded1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ffree-speech-trump-maga.html","wordCount":2154},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DiptychBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DiptychBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DiptychBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Noah Shachtman","id":"UGVyc29uOm55dDovL3BlcnNvbi9kMGQyNjM0NC0xZjJiLTU3MmEtOTk5Ni05ZDM4ZTBhNzNkMmM=","promotionalMedia":null}],"renderedRepresentation":"By Noah Shachtman"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-6717e682-229e-51b8-9947-2b735defd735\u002Fjob-1740736814449\u002Farticle-6717e682-229e-51b8-9947-2b735defd735-job-1740736814449.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Dead Athletes. Empty Stands. Why Are We Paying Billions to Keep This Sport Alive?"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vODJkN2ZiODctZWRmNC01NjM2LThhNTEtNzgwYzkxYTEzNjk0","length":1554,"sourceId":"100000010019010","uri":"nyt:\u002F\u002Faudio\u002F82d7fb87-edf4-5636-8a51-780c91a13694"}},"firstPublished":"2025-02-28T10:00:12.600Z","headline":{"__typename":"CreativeWorkHeadline","default":"Dead Athletes. Empty Stands. Why Are We Paying Billions to Keep This Sport Alive?"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzY3MTdlNjgyLTIyOWUtNTFiOC05OTQ3LTJiNzM1ZGVmZDczNQ==","lastModified":"2025-02-28T10:00:12.600Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-square640-v2.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-threeByTwoLargeAt2X-v2.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNTZkNTUyNTYtOTgxZi01ZDAxLTkyZTItYjE2YjczMWY1ZGFj"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000009963657","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002F6717e682-229e-51b8-9947-2b735defd735","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fhorse-racing-government-subsidies.html","wordCount":4527}],"children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Frank Bruni","id":"UGVyc29uOm55dDovL3BlcnNvbi82M2MzMWU1ZC1jNmVhLTUyZTAtYTJjYS1hNzMxNzMzMmE0NzU=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F04\u002F03\u002Fopinion\u002Ffrank-bruni\u002Ffrank-bruni-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMWUwNGNiMjUtYjJlNy01MTI1LTlhNDItNjI1OGJjODVjNmI3"}},{"__typename":"Person","displayName":"Nicole Hemmer","id":"UGVyc29uOm55dDovL3BlcnNvbi8xNDNmZjQ5My1kMzk2LTVhYzEtOTMzOC0zOWJlMjNjYWU1MTc=","promotionalMedia":null},{"__typename":"Person","displayName":"Tim Miller","id":"UGVyc29uOm55dDovL3BlcnNvbi9hMWJlNTUxMC00NTU4LTVhYzMtODk5Ni04YjMwMTYyNTA3ZjQ=","promotionalMedia":null}],"renderedRepresentation":"By Frank Bruni, Nicole Hemmer and Tim Miller"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-f08fbb32-8818-558e-8473-71631754acea\u002Fjob-1740736921738\u002Farticle-f08fbb32-8818-558e-8473-71631754acea-job-1740736921738.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for ‘An Emotional Torture Chamber for Liberals’: 3 Writers on Trump’s First Month"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vYWNjYTNhMDktYmIyMy01YTlkLThiNWQtYWQ2ZTMzYjljMzM1","length":1219,"sourceId":"100000010019026","uri":"nyt:\u002F\u002Faudio\u002Facca3a09-bb23-5a9d-8b5d-ad6e33b9c335"}},"firstPublished":"2025-02-28T10:02:00.449Z","headline":{"__typename":"CreativeWorkHeadline","default":"‘An Emotional Torture Chamber for Liberals’: 3 Writers on Trump’s First Month"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2YwOGZiYjMyLTg4MTgtNTU4ZS04NDczLTcxNjMxNzU0YWNlYQ==","lastModified":"2025-02-28T10:02:00.449Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTg3MTk5ODEtZGRlMy01OTAzLWIxOWEtYjQ1OTAyZjBmZGJi"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010004727","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002Ff08fbb32-8818-558e-8473-71631754acea","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fdonald-trump-birthright-citizenship.html","wordCount":3505},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Michelle Goldberg","id":"UGVyc29uOm55dDovL3BlcnNvbi85YzM3YWYyZS0wNmE0LTU5NzAtYjUzMi00MzMzYjcyZjQzNzE=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F04\u002F02\u002Fopinion\u002Fmichelle-goldberg\u002Fmichelle-goldberg-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMmRmZTA1ZWMtYzhmMC01MWUxLWJkMTktNDZmNjMxMjliZTQ3"}}],"renderedRepresentation":"By Michelle Goldberg"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-96f9592e-54a6-5dea-845b-785346ba26b1\u002Fjob-1740737063810\u002Farticle-96f9592e-54a6-5dea-845b-785346ba26b1-job-1740737063810.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Andrew Tate, Accused Sex Trafficker, Is Trump’s Kind of Guy"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZjk5YTA2NzUtZTMzNy01YWE0LTg2ZTAtNDExNzYwMGM4MjUz","length":364,"sourceId":"100000010019043","uri":"nyt:\u002F\u002Faudio\u002Ff99a0675-e337-5aa4-86e0-4117600c8253"}},"firstPublished":"2025-02-28T10:04:23.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Andrew Tate, Accused Sex Trafficker, Is Trump’s Kind of Guy"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzk2Zjk1OTJlLTU0YTYtNWRlYS04NDViLTc4NTM0NmJhMjZiMQ==","lastModified":"2025-02-28T12:08:49.046Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNTI3YjRmZGYtN2VjOS01MjNiLWI4ZDYtM2YzYjMwZDA4ZGJl"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010017487","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002F96f9592e-54a6-5dea-845b-785346ba26b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fandrew-tate-donald-trump.html","wordCount":1060}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T10:02:00.449Z","uri":"nyt:\u002F\u002Farticle\u002Ff08fbb32-8818-558e-8473-71631754acea","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fdonald-trump-birthright-citizenship.html"},{"__typename":"Article","lastModified":"2025-02-28T12:08:49.046Z","uri":"nyt:\u002F\u002Farticle\u002F96f9592e-54a6-5dea-845b-785346ba26b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fandrew-tate-donald-trump.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fc5b4c859d26b41e59e79878ff6ae348c","hierarchyType":"addOn","isPersonalized":false,"layoutType":"StandardAddon","media":[{"__typename":"Image","altText":"A collage of images including close-ups of the faces of Donald Trump and Elon Musk, their mouths and a government building.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Illustration by Shoshana Schultz\u002FThe New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-square640.jpg","width":640},{"__typename":"ImageRendition","height":980,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-mediumSquareAt3X.jpg","width":981}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1001,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28bruni-hemmer-miller\u002F28bruni-hemmer-miller-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-27T23:41:23.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTg3MTk5ODEtZGRlMy01OTAzLWIxOWEtYjQ1OTAyZjBmZGJi","lastModified":"2025-02-28T10:01:58.310Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010018088","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F98719981-dde3-5903-b19a-b45902f0fdbb","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fopinion\u002F28bruni-hemmer-miller.html"},{"__typename":"Image","altText":"Andrew Tate’s head partly obscuring Tristan Tate’s head.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Tristan, left, and Andrew Tate"},"captionOverride":null,"credit":"Alon Skuy\u002FGetty Images","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1001,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28goldberg-wczt\u002F28goldberg-wczt-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-27T22:26:16.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNTI3YjRmZGYtN2VjOS01MjNiLWI4ZDYtM2YzYjMwZDA4ZGJl","lastModified":"2025-02-28T10:04:20.146Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017885","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F527b4fdf-7ec9-523b-b8d6-3f3b30d08dbe","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F28goldberg-wczt.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"isOpinion\":true,\"isBundle\":false,\"assets\":[{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F98719981-dde3-5903-b19a-b45902f0fdbb\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"SMALL\",\"fullWidthMedia\":false,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"‘Like Raptors Flinging Themselves Against an Electric Fence’: 3 Writers on Trump’s First Month\",\"overrideByline\":true,\"byline\":\"Frank Bruni, Nicole Hemmer and Tim Miller\",\"italicizeHeadline\":false,\"summary\":\"A discussion of stunt politics and how Republicans leverage emotionally charged issues over a long horizon.\",\"thread\":null,\"sentence\":\"‘An Emotional Torture Chamber for Liberals’: 3 Writers on Trump’s First Month\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Ff08fbb32-8818-558e-8473-71631754acea\"},{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F527b4fdf-7ec9-523b-b8d6-3f3b30d08dbe\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"SMALL\",\"fullWidthMedia\":false,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Andrew Tate, Accused Sex Trafficker, Is Trump’s Kind of Guy\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The president ushers in a new age of aggressive male impunity.\",\"thread\":null,\"sentence\":\"Andrew Tate, Accused Sex Trafficker, Is Trump’s Kind of Guy\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F96f9592e-54a6-5dea-845b-785346ba26b1\"}]}","sourceId":"9112140b-d8ab-43f0-b316-8954b333a52c","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F37302586-9518-51ea-ba75-48867c16287d","url":"","variantAllocation":null,"children":[]},{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"David Brooks","id":"UGVyc29uOm55dDovL3BlcnNvbi81ODlkYjI4NS1jM2UwLTU1NTItYmVmYi1jYzMyYzEyMzYxMWU=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F04\u002F03\u002Fopinion\u002Fdavid-brooks\u002Fdavid-brooks-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjc2OTI1MmItNzRkNC01YTNiLTk5YjgtNTJkNTJjMWZiNmVl"}}],"renderedRepresentation":"By David Brooks"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-896e3424-8c01-5485-bb02-1560953263ba\u002Fjob-1740693606332\u002Farticle-896e3424-8c01-5485-bb02-1560953263ba-job-1740693606332.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for We Can Achieve Great Things"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vNDI0NjViZTctODE4ZS01ZGE4LTg1ZjUtZDZlNjU4ZWMyNDZi","length":576,"sourceId":"100000010017831","uri":"nyt:\u002F\u002Faudio\u002F42465be7-818e-5da8-85f5-d6e658ec246b"}},"firstPublished":"2025-02-27T22:00:05.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"We Can Achieve Great Things"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzg5NmUzNDI0LThjMDEtNTQ4NS1iYjAyLTE1NjA5NTMyNjNiYQ==","lastModified":"2025-02-28T01:29:26.255Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F27brooks-jpgv\u002F27brooks-jpgv-square640-v2.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F27brooks-jpgv\u002F27brooks-jpgv-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMWQwZjNkMWQtNDkzMy01NzljLTkwZmQtYTA3NzczNmVlMWY4"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010014267","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002F896e3424-8c01-5485-bb02-1560953263ba","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Fgovernment-great-progressive-abundance.html","wordCount":1578},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"William Shoki","id":"UGVyc29uOm55dDovL3BlcnNvbi80MGZiZDEyOC1jOWY3LTVmYzQtODlkNi1lYzliYzcxMTJiODA=","promotionalMedia":null}],"renderedRepresentation":"By William Shoki"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-5452cb92-2c7c-5c19-b4c5-863234fce6ab\u002Fjob-1740740448161\u002Farticle-5452cb92-2c7c-5c19-b4c5-863234fce6ab-job-1740740448161.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Elon Musk Is South African. It Explains a Lot."},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZWYyMzdjM2EtYjlmNi01NTM4LTlkMTQtOWFkYTg3YmY4ODll","length":459,"sourceId":"100000010018592","uri":"nyt:\u002F\u002Faudio\u002Fef237c3a-b9f6-5538-9d14-9ada87bf889e"}},"firstPublished":"2025-02-28T06:00:05.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Elon Musk Is South African. It Explains a Lot."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzU0NTJjYjkyLTJjN2MtNWMxOS1iNGM1LTg2MzIzNGZjZTZhYg==","lastModified":"2025-02-28T11:00:46.809Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002F26shoki-pmzw\u002F26shoki-pmzw-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F26\u002Fmultimedia\u002F26shoki-pmzw\u002F26shoki-pmzw-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMmI5ZTBmZmQtMTM1Yi01MGY2LWI3YTItYWYzNmE4YzE0NThi"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010004043","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002F5452cb92-2c7c-5c19-b4c5-863234fce6ab","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Felon-musk-south-africa.html","wordCount":1225},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Zeynep Tufekci","id":"UGVyc29uOm55dDovL3BlcnNvbi82ZjUwMDViMi1mMzkwLTVhYmItOWE2Ny1iNGQzMWMzOGQxNDY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2015\u002F03\u002F16\u002Fopinion\u002FTufekci-Zeynep-circular\u002FTufekci-Zeynep-circular-thumbLarge-v3.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZTE2MzhhMmEtMGQ5NS01Y2QwLTk4ZWUtNGJiNjg3ZjU0ODU1"}}],"renderedRepresentation":"By Zeynep Tufekci"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a\u002Fjob-1740737049913\u002Farticle-0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a-job-1740737049913.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for The Texas Measles Outbreak Is Even Scarier Than It Looks"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vODVjZGVhNDktYjdlOC01OGE0LWE5M2UtM2UyMTEyMTgzMDM2","length":422,"sourceId":"100000010019040","uri":"nyt:\u002F\u002Faudio\u002F85cdea49-b7e8-58a4-a93e-3e2112183036"}},"firstPublished":"2025-02-28T10:04:09.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"The Texas Measles Outbreak Is Even Scarier Than It Looks"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzBmNTViOWYyLTRhMWQtNWUwOS05Mjc1LWRhMjlmOWJmYmUxYQ==","lastModified":"2025-02-28T12:03:51.145Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02tufekci-lfpg\u002F28tufekci-lfpg-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02tufekci-lfpg\u002F28tufekci-lfpg-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMTJhNDRlYjEtNjI3YS01ZTJlLTgyNWUtYTRjM2VjZWVkN2Fj"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010016489","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002F0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ftexas-measles-vaccine.html","wordCount":1213},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Michael Kinnucan","id":"UGVyc29uOm55dDovL3BlcnNvbi9jYmQwYmExNS0zNTJhLTU3MDctOTg4Ni1jZTY5MzE0ZjBkZWI=","promotionalMedia":null}],"renderedRepresentation":"By Michael Kinnucan"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-f716ba76-77d6-558b-9a94-bf1f5f1718b1\u002Fjob-1740736927100\u002Farticle-f716ba76-77d6-558b-9a94-bf1f5f1718b1-job-1740736927100.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Republicans Want to Gut Medicaid. They Might Regret It."},"id":"QXVkaW86bnl0Oi8vYXVkaW8vYmIzZDc0NTAtMDhlNS01Y2I0LThlMDgtOGU3OTNkMzc3OWU2","length":472,"sourceId":"100000010019022","uri":"nyt:\u002F\u002Faudio\u002Fbb3d7450-08e5-5cb4-8e08-8e793d3779e6"}},"firstPublished":"2025-02-28T10:02:05.958Z","headline":{"__typename":"CreativeWorkHeadline","default":"Republicans Want to Gut Medicaid. They Might Regret It."},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2Y3MTZiYTc2LTc3ZDYtNTU4Yi05YTk0LWJmMWY1ZjE3MThiMQ==","lastModified":"2025-02-28T10:02:05.958Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28kinnucan\u002F28kinnucan-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28kinnucan\u002F28kinnucan-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNGY1ODZkNTEtYjNlZi01M2RkLTllOWYtZGYxYTUzNTlmYTYz"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010004796","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002Ff716ba76-77d6-558b-9a94-bf1f5f1718b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmedicaid-republicans.html","wordCount":1220},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderMultimediaBlock","media":{"__typename":"AudioBlock","media":{"__typename":"Audio","fileUrl":"https:\u002F\u002Fnyt.simplecastaudio.com\u002F1fe58c15-392f-4bd2-9c69-5e2935220d3c\u002Fepisodes\u002Ff2ed2fbf-62e3-4620-a8e6-4d42d80c305b\u002Faudio\u002F128\u002Fdefault.mp3?awCollectionId=1fe58c15-392f-4bd2-9c69-5e2935220d3c&awEpisodeId=f2ed2fbf-62e3-4620-a8e6-4d42d80c305b&nocache","headline":{"__typename":"CreativeWorkHeadline","default":"Your Questions Answered, and a Big Announcement"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZDAxNDI1NWEtNmJiNC01MjlkLWIzMWYtNGEwMmJlMjE5ZGRk","length":2743,"podcastSeries":{"__typename":"AudioPodcastSeries","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F05\u002F08\u002Fpodcasts\u002Fmatter-of-opinion-album-art\u002Fmatter-of-opinion-album-art-square640-v2.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNTBmNjc5YmQtNzZjYS01ZTkyLTk4YzctNzNiNzM0ZjJkNDcy"},"title":"Matter of Opinion"},"promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F05\u002F09\u002Fpodcasts\u002F28moo-mailbag-audio\u002F28moo-mailbag-audio-square640-v4.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F05\u002F09\u002Fpodcasts\u002F28moo-mailbag-audio\u002Fmatter-of-opinion-promo-art-threeByTwoLargeAt2X-v3.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYzQ4ZGQwNzctMDY3OS01NThmLTliNTQtNTljZTc3NWZiMTI4"}},"sourceId":"100000010009123","uri":"nyt:\u002F\u002Faudio\u002Fd014255a-6bb4-529d-b31f-4a02be219ddd"}}},{"__typename":"BylineBlock"},{"__typename":"Heading3Block"},{"__typename":"RuleBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Michelle Cottle","id":"UGVyc29uOm55dDovL3BlcnNvbi9mMjg4OTE1NS1jOWUxLTVkYTQtYjhkOC02MTc1MzIwMDRhNmM=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2019\u002F06\u002F25\u002Fopinion\u002Fmichelle-cottle-circular\u002Fmichelle-cottle-circular-thumbLarge-v4.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMGQxZThlYzMtMDRkOS01MzVmLThlNGUtYTU3YWQxNWVkOTA3"}},{"__typename":"Person","displayName":"Ross Douthat","id":"UGVyc29uOm55dDovL3BlcnNvbi8wNmE5ODFiNC00ODc3LTVjNGMtODVhMy01NGMwYzE1MTUxMmE=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F04\u002F03\u002Fopinion\u002Fross-douthat\u002Fross-douthat-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZGI3MmFjOTItYzM1Mi01ZjdmLWI1MDktNDg5ODM5YWU2Mzlh"}},{"__typename":"Person","displayName":"Carlos Lozada","id":"UGVyc29uOm55dDovL3BlcnNvbi84NDU4Y2Y5NS04YzgwLTVjYmYtYjY3Ny0xZGQ5MGNiN2IzZjA=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F09\u002F09\u002Fopinion\u002Fcarlos-lozada-circle\u002Fcarlos-lozada-circle-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZjk2NGI0ZWUtMzRmNy01MjM4LWJjN2UtMzcwZWI3YWVkOWQ2"}}],"renderedRepresentation":"By Michelle Cottle, Ross Douthat and Carlos Lozada"}],"featuredAudio":null,"firstPublished":"2025-02-28T10:02:16.383Z","headline":{"__typename":"CreativeWorkHeadline","default":"Your Questions Answered, and a Big Announcement"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2U0YzRmY2RjLTJmNTAtNWJjYS04M2Q3LThiODhjOWM0ZWM4Yw==","lastModified":"2025-02-28T10:02:16.383Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F05\u002F09\u002Fpodcasts\u002F28moo-mailbag-audio\u002F28moo-mailbag-audio-square640-v4.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F05\u002F09\u002Fpodcasts\u002F28moo-mailbag-audio\u002Fmatter-of-opinion-promo-art-threeByTwoLargeAt2X-v3.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYzQ4ZGQwNzctMDY3OS01NThmLTliNTQtNTljZTc3NWZiMTI4"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010009132","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002Fe4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmatter-of-opinion-final-episode.html","wordCount":270},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Bret Stephens","id":"UGVyc29uOm55dDovL3BlcnNvbi82YzYxNGU2Ni1hZjYyLTVhZTMtYmMzOS05OTkwY2ViZjk1M2M=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2017\u002F08\u002F27\u002Finsider\u002Fbretstephens\u002Fbretstephens-thumbLarge-v6.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNGIwNDhiY2UtMTZkNi01OTIwLWIwYTYtZDMwMmVmZmVmZDIw"}}],"renderedRepresentation":"By Bret Stephens"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-a3f3b0d1-5608-54dd-a08b-83d185b5db46\u002Fjob-1740682761654\u002Farticle-a3f3b0d1-5608-54dd-a08b-83d185b5db46-job-1740682761654.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Earth to Barnard College: Enough"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZTI0Y2YzYmUtMzlmNi01NWYwLWEyOTMtZGFjOTUyNzYxMTE5","length":176,"sourceId":"100000010017241","uri":"nyt:\u002F\u002Faudio\u002Fe24cf3be-39f6-55f0-a293-dac952761119"}},"firstPublished":"2025-02-27T18:59:04.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Earth to Barnard College: Enough"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2EzZjNiMGQxLTU2MDgtNTRkZC1hMDhiLTgzZDE4NWI1ZGI0Ng==","lastModified":"2025-02-28T01:29:38.344Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010017011","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002Fa3f3b0d1-5608-54dd-a08b-83d185b5db46","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F25\u002Fopinion\u002Fthepoint\u002Fbarnard-college-sit-in-palestinian-israel-protest","wordCount":455},{"__typename":"Article","associatedNewsletter":{"__typename":"AssociatedNewsletter","newsletterProduct":{"__typename":"HelixNewsletterProduct","id":"SGVsaXhOZXdzbGV0dGVyUHJvZHVjdDpueXQ6Ly9uZXdzbGV0dGVycHJvZHVjdC81MjFjMmE5MS05ODgyLTQ5MWEtYmQ4ZS02YWUxYTliZTBiOWI=","name":"David French"}},"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RuleBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"BlockquoteBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"BlockquoteBlock"},{"__typename":"RelatedLinksBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"David French","id":"UGVyc29uOm55dDovL3BlcnNvbi9lYTA2NWVjYy1lODdiLTViZGYtYmQxOS00ZWIxNjhmNmQ4Y2I=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F01\u002F10\u002Fopinion\u002Fdavid-french\u002Fdavid-french-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTQ0MDUyZjMtNTAyYi01M2QwLTkzZjEtNTE4ZjY5ZWMwNDIz"}}],"renderedRepresentation":"By David French"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-e5fb45b5-1082-5fcc-ba9a-5bcc56a75975\u002Fjob-1740687427037\u002Farticle-e5fb45b5-1082-5fcc-ba9a-5bcc56a75975-job-1740687427037.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for There Is Nothing Normal About Any of This"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZjQ1M2FlNGQtNTBiYy01Mzg3LWJjY2UtNTY0M2I1Nzg1NTBh","length":851,"sourceId":"100000010016131","uri":"nyt:\u002F\u002Faudio\u002Ff453ae4d-50bc-5387-bcce-5643b578550a"}},"firstPublished":"2025-02-27T10:04:24.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Trump’s Decision to Fire the JAG Generals Gives the Game Away"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2U1ZmI0NWI1LTEwODItNWZjYy1iYTlhLTViY2M1NmE3NTk3NQ==","lastModified":"2025-02-28T12:12:33.018Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fopinion\u002F27french-newsletter\u002F27french-newsletter-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fopinion\u002F27french-newsletter\u002F27french-newsletter-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDYyNzllNzItOTM3Zi01M2VkLWFhOWQtYzUzMTdlY2RlODBj"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010015506","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002Fe5fb45b5-1082-5fcc-ba9a-5bcc56a75975","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-hegseth-pentagon.html","wordCount":2261},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"InteractiveBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"InteractiveBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"RelatedLinksBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Michael Tesler","id":"UGVyc29uOm55dDovL3BlcnNvbi83ZTBmNTBlNC1hNWM3LTU3MzQtYTdkMC01NjQ2ZThkODE4ZDE=","promotionalMedia":null},{"__typename":"Person","displayName":"John Sides","id":"UGVyc29uOm55dDovL3BlcnNvbi9jODlhN2IzMS1hYTJjLTU1MTItYmU3YS02YTZjNmZiN2ExNWU=","promotionalMedia":null},{"__typename":"Person","displayName":"Colette Marcellin","id":"UGVyc29uOm55dDovL3BlcnNvbi8zYzUxMWJmNy0xNTM5LTVjNTItYmQ2Ny0xY2UyNjJiNWE5NjQ=","promotionalMedia":null}],"renderedRepresentation":"By Michael Tesler, John Sides and Colette Marcellin"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-1b78bf9c-210e-5ae9-99a4-980a3696891d\u002Fjob-1740696682684\u002Farticle-1b78bf9c-210e-5ae9-99a4-980a3696891d-job-1740696682684.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Republicans Really Do Care More About ‘Masculine Energy’"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZGFkNmI2NmItZGFhZi01YzZmLWI3NzYtOWIwMGZjNzM4ZGMx","length":501,"sourceId":"100000010016129","uri":"nyt:\u002F\u002Faudio\u002Fdad6b66b-daaf-5c6f-b776-9b00fc738dc1"}},"firstPublished":"2025-02-27T10:04:12.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Republican Men and Women Are Changing Their Minds About How Women Should Behave"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzFiNzhiZjljLTIxMGUtNWFlOS05OWE0LTk4MGEzNjk2ODkxZA==","lastModified":"2025-02-28T02:33:52.611Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fopinion\u002F27tesler-promo\u002F27tesler-promo-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fopinion\u002F27tesler-promo\u002F27tesler-promo-threeByTwoLargeAt2X-v2.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTAzOTdiMDQtZjcwNy01MTMzLTg0N2ItYzNjZjA5NzUxODAy"}},"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010014789","tone":"OPINION","uri":"nyt:\u002F\u002Farticle\u002F1b78bf9c-210e-5ae9-99a4-980a3696891d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-republicans-masculinity-gender-traditional.html","wordCount":1306}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T01:29:26.255Z","uri":"nyt:\u002F\u002Farticle\u002F896e3424-8c01-5485-bb02-1560953263ba","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Fgovernment-great-progressive-abundance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:00:46.809Z","uri":"nyt:\u002F\u002Farticle\u002F5452cb92-2c7c-5c19-b4c5-863234fce6ab","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Felon-musk-south-africa.html"},{"__typename":"Article","lastModified":"2025-02-28T12:03:51.145Z","uri":"nyt:\u002F\u002Farticle\u002F0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ftexas-measles-vaccine.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:05.958Z","uri":"nyt:\u002F\u002Farticle\u002Ff716ba76-77d6-558b-9a94-bf1f5f1718b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmedicaid-republicans.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:16.383Z","uri":"nyt:\u002F\u002Farticle\u002Fe4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmatter-of-opinion-final-episode.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:38.344Z","uri":"nyt:\u002F\u002Farticle\u002Fa3f3b0d1-5608-54dd-a08b-83d185b5db46","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F25\u002Fopinion\u002Fthepoint\u002Fbarnard-college-sit-in-palestinian-israel-protest"},{"__typename":"Article","lastModified":"2025-02-28T12:12:33.018Z","uri":"nyt:\u002F\u002Farticle\u002Fe5fb45b5-1082-5fcc-ba9a-5bcc56a75975","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-hegseth-pentagon.html"},{"__typename":"Article","lastModified":"2025-02-28T02:33:52.611Z","uri":"nyt:\u002F\u002Farticle\u002F1b78bf9c-210e-5ae9-99a4-980a3696891d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-republicans-masculinity-gender-traditional.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F08ef6db660fd4b648117c07646784072","hierarchyType":"addOn","isPersonalized":false,"layoutType":"StandardLevel2","media":[],"personalizationConfig":null,"properties":"{\"isOpinion\":true,\"isBundle\":false,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"display\":\"HEADLINE_ONLY\",\"assets\":[{\"headline\":\"We Can Achieve Great Things\",\"overrideByline\":false,\"byline\":null,\"thread\":null,\"sentence\":\"We Can Achieve Great Things\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F896e3424-8c01-5485-bb02-1560953263ba\"},{\"headline\":\"Elon Musk Is South African. We Shouldn’t Forget It.\",\"overrideByline\":false,\"byline\":null,\"thread\":null,\"sentence\":\"Elon Musk Is South African. It Explains a Lot.\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F5452cb92-2c7c-5c19-b4c5-863234fce6ab\"},{\"headline\":\"The Texas Measles Outbreak Is Even Scarier Than It Looks\",\"overrideByline\":false,\"byline\":null,\"thread\":null,\"sentence\":\"The Texas Measles Outbreak Is Even Scarier Than It Looks\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a\"},{\"headline\":\"Republicans Want to Gut Medicaid. They Might Regret It.\",\"overrideByline\":false,\"byline\":null,\"thread\":null,\"sentence\":\"Republicans Want to Gut Medicaid. They Might Regret It.\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Ff716ba76-77d6-558b-9a94-bf1f5f1718b1\"},{\"headline\":\"Your Questions Answered, and a Big Announcement\",\"overrideByline\":true,\"byline\":\"\",\"thread\":null,\"sentence\":\"Your Questions Answered, and a Big Announcement\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fe4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c\"},{\"headline\":\"Earth to Barnard College: Enough\",\"overrideByline\":false,\"byline\":null,\"thread\":null,\"sentence\":\"Earth to Barnard College: Enough\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fa3f3b0d1-5608-54dd-a08b-83d185b5db46\"},{\"headline\":\"Trump’s Decision to Fire the JAG Generals Gives the Game Away\",\"overrideByline\":false,\"byline\":null,\"thread\":null,\"sentence\":\"There Is Nothing Normal About Any of This\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fe5fb45b5-1082-5fcc-ba9a-5bcc56a75975\"},{\"headline\":\"Republican Men and Women Are Changing Their Minds About How Women Should Behave\",\"overrideByline\":true,\"byline\":\"Michael Tesler, John Sides and Colette Marcellin\",\"thread\":null,\"sentence\":\"Republicans Really Do Care More About ‘Masculine Energy’\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F1b78bf9c-210e-5ae9-99a4-980a3696891d\"}]}","sourceId":"af161d48-f72e-4359-8baf-bdc2dfa44ba8","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F3d33b6e2-74d4-51a0-bed1-7f32a4a87d57","url":"","variantAllocation":null,"children":[]}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T10:02:00.449Z","uri":"nyt:\u002F\u002Farticle\u002Ff08fbb32-8818-558e-8473-71631754acea","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fdonald-trump-birthright-citizenship.html"},{"__typename":"Article","lastModified":"2025-02-28T12:08:49.046Z","uri":"nyt:\u002F\u002Farticle\u002F96f9592e-54a6-5dea-845b-785346ba26b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fandrew-tate-donald-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:26.255Z","uri":"nyt:\u002F\u002Farticle\u002F896e3424-8c01-5485-bb02-1560953263ba","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Fgovernment-great-progressive-abundance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:00:46.809Z","uri":"nyt:\u002F\u002Farticle\u002F5452cb92-2c7c-5c19-b4c5-863234fce6ab","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Felon-musk-south-africa.html"},{"__typename":"Article","lastModified":"2025-02-28T12:03:51.145Z","uri":"nyt:\u002F\u002Farticle\u002F0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ftexas-measles-vaccine.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:05.958Z","uri":"nyt:\u002F\u002Farticle\u002Ff716ba76-77d6-558b-9a94-bf1f5f1718b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmedicaid-republicans.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:16.383Z","uri":"nyt:\u002F\u002Farticle\u002Fe4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmatter-of-opinion-final-episode.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:38.344Z","uri":"nyt:\u002F\u002Farticle\u002Fa3f3b0d1-5608-54dd-a08b-83d185b5db46","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F25\u002Fopinion\u002Fthepoint\u002Fbarnard-college-sit-in-palestinian-israel-protest"},{"__typename":"Article","lastModified":"2025-02-28T12:12:33.018Z","uri":"nyt:\u002F\u002Farticle\u002Fe5fb45b5-1082-5fcc-ba9a-5bcc56a75975","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-hegseth-pentagon.html"},{"__typename":"Article","lastModified":"2025-02-28T02:33:52.611Z","uri":"nyt:\u002F\u002Farticle\u002F1b78bf9c-210e-5ae9-99a4-980a3696891d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-republicans-masculinity-gender-traditional.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F1b9f41a32ef34c05b02a810e355e788c","hierarchyType":"dropzone","sourceId":"5094a4ac-2b3e-4e98-b86e-7ad5379c3f319b0e9b26-ebed-47bb-ae2d-fcc3272b4a8cbelowPackage","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F62b0f830-920f-5721-a14a-7b165fe81f30","url":"","variantAllocation":null,"zoneKey":"belowPackage","assets":undefined,"media":undefined}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T10:02:00.449Z","uri":"nyt:\u002F\u002Farticle\u002Ff08fbb32-8818-558e-8473-71631754acea","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fdonald-trump-birthright-citizenship.html"},{"__typename":"Article","lastModified":"2025-02-28T12:08:49.046Z","uri":"nyt:\u002F\u002Farticle\u002F96f9592e-54a6-5dea-845b-785346ba26b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fandrew-tate-donald-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:26.255Z","uri":"nyt:\u002F\u002Farticle\u002F896e3424-8c01-5485-bb02-1560953263ba","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Fgovernment-great-progressive-abundance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:00:46.809Z","uri":"nyt:\u002F\u002Farticle\u002F5452cb92-2c7c-5c19-b4c5-863234fce6ab","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Felon-musk-south-africa.html"},{"__typename":"Article","lastModified":"2025-02-28T12:03:51.145Z","uri":"nyt:\u002F\u002Farticle\u002F0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ftexas-measles-vaccine.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:05.958Z","uri":"nyt:\u002F\u002Farticle\u002Ff716ba76-77d6-558b-9a94-bf1f5f1718b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmedicaid-republicans.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:16.383Z","uri":"nyt:\u002F\u002Farticle\u002Fe4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmatter-of-opinion-final-episode.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:38.344Z","uri":"nyt:\u002F\u002Farticle\u002Fa3f3b0d1-5608-54dd-a08b-83d185b5db46","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F25\u002Fopinion\u002Fthepoint\u002Fbarnard-college-sit-in-palestinian-israel-protest"},{"__typename":"Article","lastModified":"2025-02-28T12:12:33.018Z","uri":"nyt:\u002F\u002Farticle\u002Fe5fb45b5-1082-5fcc-ba9a-5bcc56a75975","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-hegseth-pentagon.html"},{"__typename":"Article","lastModified":"2025-02-28T02:33:52.611Z","uri":"nyt:\u002F\u002Farticle\u002F1b78bf9c-210e-5ae9-99a4-980a3696891d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-republicans-masculinity-gender-traditional.html"},{"__typename":"Article","lastModified":"2025-02-28T12:01:50.458Z","uri":"nyt:\u002F\u002Farticle\u002F351b30ec-12d0-5d59-9f63-5d81a895ded1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ffree-speech-trump-maga.html"},{"__typename":"Article","lastModified":"2025-02-28T10:00:12.600Z","uri":"nyt:\u002F\u002Farticle\u002F6717e682-229e-51b8-9947-2b735defd735","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fhorse-racing-government-subsidies.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fe4bcd0dd20ef41d5b251b4c8850bd8e2","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"EmbeddedInteractive","bylines":[],"compatibility":"INLINE","credit":"","displayProperties":{"__typename":"CreativeWorkDisplayProperties","displayOverrides":["DARK_MODE_COMPATIBLE"]},"firstPublished":"2024-10-24T16:11:37.000Z","html":"\r\n\u003Cdiv class=\"g-large-opinion-label\"\u003E\r\n\u003Ca href=\"https:\u002F\u002Fwww.nytimes.com\u002Fsection\u002Fopinion\"\u003E\r\nOpinion\r\n\u003C\u002Fa\u003E\r\n\u003C\u002Fdiv\u003E","id":"RW1iZWRkZWRJbnRlcmFjdGl2ZTpueXQ6Ly9lbWJlZGRlZGludGVyYWN0aXZlL2RmNDM1OTVjLWJkMjgtNWZkOS1iN2Y4LWUyMDkxMWNhZWQwOQ==","lastModified":"2025-02-07T15:49:39.621Z","promotionalImage":null,"promotionalMedia":null,"section":{"__typename":"Section","displayName":"Admin","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q0MmQzYTNmLTAyYTgtNWRlNy1hOTEyLTUyMGZhNmI0NGY0ZQ=="},"slug":"large-opinion-label","sourceId":"100000009780651","storyFormat":null,"tone":"NEWS","uri":"nyt:\u002F\u002Fembeddedinteractive\u002Fdf43595c-bd28-5fd9-b7f8-e20911caed09","url":"null"},{"__typename":"Image","altText":"A computer keyboard against a black background. Each of the letter keys have been replaced so that the keyboard repetitively spells out M, A, G, A.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Illustration by Rebecca Chew\u002FThe New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-square640.jpg","width":640},{"__typename":"ImageRendition","height":702,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-mediumSquareAt3X.jpg","width":701}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":717,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-threeByTwoMediumAt2X.jpg","width":1076},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fopinion\u002F28editorial-speech-image\u002F28editorial-speech-image-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T00:50:18.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNDA4MjExZTEtMmFhZi01YzRkLWFiMzQtMjE4MjJhOTllZTg0","lastModified":"2025-02-28T10:03:55.243Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Opinion","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2Q3YTcxMTg1LWFhNjAtNTYzNS1iY2UwLTVmYWI3NmM3YzI5Nw=="},"sourceId":"100000010018225","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F408211e1-2aaf-5c4d-ab34-21822a99ee84","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fopinion\u002F28editorial-speech-image.html"},{"__typename":"Image","altText":"A brown racehorse in mid gallop, with a jockey perched atop. ","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"Justin Kaneps for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-smallSquare252-v2.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-square640-v2.jpg","width":640},{"__typename":"ImageRendition","height":1799,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-mediumSquareAt3X-v2.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-threeByTwoMediumAt2X-v2.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-threeByTwoSmallAt2X-v2.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-videoSixteenByNine1050-v2.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1102,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F03\u002F02\u002Fmultimedia\u002F02cover1-kphj\u002F02cover1-kphj-verticalTwoByThree735-v2.jpg","width":735}]}],"firstPublished":"2025-02-28T10:00:08.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNTZkNTUyNTYtOTgxZi01ZDAxLTkyZTItYjE2YjczMWY1ZGFj","lastModified":"2025-02-28T10:24:40.962Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010009652","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F56d55256-981f-5d01-92e2-b16b731f5dac","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F02cover1-kphj.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"packageTitleOverride\":\"nyt:\u002F\u002Fembeddedinteractive\u002Fdf43595c-bd28-5fd9-b7f8-e20911caed09\",\"enablePackageTitleLink\":true,\"packageTitleSection\":\"OPINION\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"nytimes:\u002F\u002Fhome\u002Fopinion\",\"isOpinion\":true,\"isBundle\":false,\"assets\":[{\"showMedia\":false,\"media\":{\"headline\":\"\",\"uri\":\"nyt:\u002F\u002Fimage\u002F408211e1-2aaf-5c4d-ab34-21822a99ee84\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Language Police Have Taken Over the White House \",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"If only the powerful are free to speak their minds, it’s not free speech.\",\"thread\":null,\"sentence\":\"The MAGA War on Speech\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F351b30ec-12d0-5d59-9f63-5d81a895ded1\"},{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F56d55256-981f-5d01-92e2-b16b731f5dac\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"HEADLINE_ONLY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Why Does the Government Pay Billions for This Deadly, Unpopular Sport?\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Let the sport of kings stand on its own.\",\"thread\":null,\"sentence\":\"Dead Athletes. Empty Stands. Why Are We Paying Billions to Keep This Sport Alive?\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F6717e682-229e-51b8-9947-2b735defd735\"}]}","sourceId":"9b0e9b26-ebed-47bb-ae2d-fcc3272b4a8c","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F911ec4be-a1df-5e54-8cdf-a4d980514578","url":"","variantAllocation":null}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T10:02:00.449Z","uri":"nyt:\u002F\u002Farticle\u002Ff08fbb32-8818-558e-8473-71631754acea","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fdonald-trump-birthright-citizenship.html"},{"__typename":"Article","lastModified":"2025-02-28T12:08:49.046Z","uri":"nyt:\u002F\u002Farticle\u002F96f9592e-54a6-5dea-845b-785346ba26b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fandrew-tate-donald-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:26.255Z","uri":"nyt:\u002F\u002Farticle\u002F896e3424-8c01-5485-bb02-1560953263ba","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Fgovernment-great-progressive-abundance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:00:46.809Z","uri":"nyt:\u002F\u002Farticle\u002F5452cb92-2c7c-5c19-b4c5-863234fce6ab","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Felon-musk-south-africa.html"},{"__typename":"Article","lastModified":"2025-02-28T12:03:51.145Z","uri":"nyt:\u002F\u002Farticle\u002F0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ftexas-measles-vaccine.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:05.958Z","uri":"nyt:\u002F\u002Farticle\u002Ff716ba76-77d6-558b-9a94-bf1f5f1718b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmedicaid-republicans.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:16.383Z","uri":"nyt:\u002F\u002Farticle\u002Fe4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmatter-of-opinion-final-episode.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:38.344Z","uri":"nyt:\u002F\u002Farticle\u002Fa3f3b0d1-5608-54dd-a08b-83d185b5db46","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F25\u002Fopinion\u002Fthepoint\u002Fbarnard-college-sit-in-palestinian-israel-protest"},{"__typename":"Article","lastModified":"2025-02-28T12:12:33.018Z","uri":"nyt:\u002F\u002Farticle\u002Fe5fb45b5-1082-5fcc-ba9a-5bcc56a75975","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-hegseth-pentagon.html"},{"__typename":"Article","lastModified":"2025-02-28T02:33:52.611Z","uri":"nyt:\u002F\u002Farticle\u002F1b78bf9c-210e-5ae9-99a4-980a3696891d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-republicans-masculinity-gender-traditional.html"},{"__typename":"Article","lastModified":"2025-02-28T12:01:50.458Z","uri":"nyt:\u002F\u002Farticle\u002F351b30ec-12d0-5d59-9f63-5d81a895ded1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ffree-speech-trump-maga.html"},{"__typename":"Article","lastModified":"2025-02-28T10:00:12.600Z","uri":"nyt:\u002F\u002Farticle\u002F6717e682-229e-51b8-9947-2b735defd735","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fhorse-racing-government-subsidies.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F0a9fbb0a9939499ca49337e3513bfe73","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"zxbzybkhjnoohrdnloysqfghhdsujjhr","trackingName":"Opinion","uri":"nyt:\u002F\u002Fprogrammingnode\u002F47d310cd-e996-583c-a6d6-c8b36289804b","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"BlockquoteBlock"},{"__typename":"YouTubeEmbedBlock"},{"__typename":"BlockquoteBlock"},{"__typename":"ParagraphBlock"},{"__typename":"BlockquoteBlock"},{"__typename":"Heading2Block"},{"__typename":"TwitterEmbedBlock"},{"__typename":"BlockquoteBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"YouTubeEmbedBlock"},{"__typename":"Heading2Block"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Trish Bendix","id":"UGVyc29uOm55dDovL3BlcnNvbi85ZWVhNjNlNS1lNGJmLTU3MmItODgyNy1hYzYxOTlkNDQ0ZTY=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F12\u002F14\u002Farts\u002Fauthor-trish-bendix\u002Fauthor-trish-bendix-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNTdkMTUyYzAtZDE3Ny01MTk3LWEwZDYtNDczZDQzN2FhZjIw"}}],"renderedRepresentation":"By Trish Bendix"}],"featuredAudio":null,"firstPublished":"2025-02-28T08:27:54.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Colbert Has Little Sympathy for Trump Voters With Buyer’s Remorse"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2ZlM2FhOTI5LTA4NWItNTI5Yy05NTk4LWUxNmZjNTRjMzYzYg==","lastModified":"2025-02-28T09:01:38.204Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight\u002F28latenight-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight\u002F28latenight-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjA4MTY2YzYtMzY5OC01ZDJkLTg5NTItZmU5ZGY1ZTRjNDE4"}},"section":{"__typename":"Section","displayName":"Arts","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzZlNmVlMjkyLWI0YmQtNTAwNi1hNjE5LTljZWFiMDM1MjRmMg=="},"sourceId":"100000010018521","tone":"FEATURE","uri":"nyt:\u002F\u002Farticle\u002Ffe3aa929-085b-529c-9598-e16fc54c363b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Ftelevision\u002Fstephen-colbert-trump-voters-remorse.html","wordCount":405}],"children":[],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T09:01:38.204Z","uri":"nyt:\u002F\u002Farticle\u002Ffe3aa929-085b-529c-9598-e16fc54c363b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Ftelevision\u002Fstephen-colbert-trump-voters-remorse.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Ff57bb386236e480ea56f3854c0c1dc5f","hierarchyType":"package","isPersonalized":false,"layoutType":"List","media":[{"__typename":"Image","altText":"Stephen Colbert, in a suit, stands onstage.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Stephen Colbert addressed voter disappointment after the Trump didn’t stop inflation on Day 1 as promised: “Somewhere in Delaware, Joe Biden is shaking his head, chuckling to himself, and thinking, ‘Why did I come into this room?’ ”"},"captionOverride":null,"credit":"CBS","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight\u002F28latenight-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight\u002F28latenight-square640.jpg","width":640},{"__typename":"ImageRendition","height":820,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight\u002F28latenight-mediumSquareAt3X.jpg","width":820}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":820,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight\u002F28latenight-threeByTwoMediumAt2X.jpg","width":1230},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight\u002F28latenight-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight\u002F28latenight-videoSixteenByNine1050.jpg","width":1050}]}],"firstPublished":"2025-02-28T06:28:50.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjA4MTY2YzYtMzY5OC01ZDJkLTg5NTItZmU5ZGY1ZTRjNDE4","lastModified":"2025-02-28T08:27:51.658Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Arts","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzZlNmVlMjkyLWI0YmQtNTAwNi1hNjE5LTljZWFiMDM1MjRmMg=="},"sourceId":"100000010018602","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F608166c6-3698-5d2d-8952-fe9df5e4c418","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Farts\u002F28latenight.html"}],"personalizationConfig":null,"properties":"{\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"SUMMARY\",\"twoUp\":false,\"showMedia\":true,\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"isNewsletter\":false,\"assets\":[{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F608166c6-3698-5d2d-8952-fe9df5e4c418\"},\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Late Night: Buyer’s Remorse\",\"summary\":\"Stephen Colbert discussed a new poll that indicated that some Trump voters were regretting their decision.\",\"thread\":null,\"sentence\":\"Colbert Has Little Sympathy for Trump Voters With Buyer’s Remorse\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Ffe3aa929-085b-529c-9598-e16fc54c363b\"}]}","sourceId":"5ed6670a-e4fc-47a5-9b0e-5b7a02278218","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fce1c7c52-2d3d-5734-8884-691ce2c1bb25","url":"","variantAllocation":null}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T09:01:38.204Z","uri":"nyt:\u002F\u002Farticle\u002Ffe3aa929-085b-529c-9598-e16fc54c363b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Ftelevision\u002Fstephen-colbert-trump-voters-remorse.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fa6c799dbb0244d21a5cc12d3d6b563e5","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"5748db9e-6b46-4fc9-a05a-24a497adeadd","trackingName":"Toolbox","uri":"nyt:\u002F\u002Fprogrammingnode\u002F425afb84-cc72-5266-8a7f-64a39051f207","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F9fdebfbadc1b4a239259ec50282e1e71","hierarchyType":"list","isPersonalized":true,"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":1,"poolOverrideUri":null,"surface":"home-icymi-zone-reordering"},"sourceId":"92c59b12-2820-40dc-bca6-135f94530cf7","trackingName":"ICYMI - Reorder","uri":"nyt:\u002F\u002Fprogrammingnode\u002Fd3c3f091-1807-58c5-9653-c218b92e769f","url":"","variantAllocation":null,"assets":undefined,"media":undefined}],"hierarchyType":"zone","trackingName":null,"assets":undefined,"media":undefined}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:37:15.494Z","uri":"nyt:\u002F\u002Farticle\u002F95773dee-1b8a-5f08-b78a-29ed1903e90c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-federal-bureaucracy-takeover.html"},{"__typename":"Article","lastModified":"2025-02-28T10:04:16.382Z","uri":"nyt:\u002F\u002Farticle\u002F89c13edf-2ccb-5223-91c7-a96b2e7b0998","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-doge-timeline-takeaways.html"},{"__typename":"Article","lastModified":"2025-02-28T11:20:12.055Z","uri":"nyt:\u002F\u002Farticle\u002F8b200636-8df5-5caa-8daa-308efea287b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-headlines\u002Fiowa-trans-rights-zelensky-trump.html"},{"__typename":"LegacyCollection","lastModified":"2025-02-28T13:10:16.130Z","uri":"nyt:\u002F\u002Flegacycollection\u002F452b1d37-7b45-5f33-86f9-23ba2244d6b3","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F28\u002Fus\u002Ftrump-news"},{"__typename":"Article","lastModified":"2025-02-28T12:13:45.314Z","uri":"nyt:\u002F\u002Farticle\u002Fe5314031-2e3d-5d39-83d9-210e3f501e95","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Feurope\u002Ftrump-starmer-king-charles.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:43.670Z","uri":"nyt:\u002F\u002Farticle\u002F3ab13ed6-8bfc-561a-926e-13bc18df7495","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fstarmer-ukraine-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T11:36:27.694Z","uri":"nyt:\u002F\u002Farticle\u002Fb0b07911-d060-5a9a-a3e6-e0db0d473d84","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002Ftrump-minerals-ukraine-republican-budget.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:49.168Z","uri":"nyt:\u002F\u002Farticle\u002Ffe1a7c93-0a1a-5d69-9592-73c1b4faa68b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Feconomy\u002Ftrump-tariff-goals-contradictions.html"},{"__typename":"Article","lastModified":"2025-02-28T12:39:54.210Z","uri":"nyt:\u002F\u002Farticle\u002F40788135-75c9-5cdc-bbf8-f46ef5b6d02d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fdealbook\u002Finflation-trump-tariffs.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:43.134Z","uri":"nyt:\u002F\u002Farticle\u002F9a41ce77-0343-5ae2-81c8-56cb5cb081cb","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrump-federal-worker-layoffs.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:42.943Z","uri":"nyt:\u002F\u002Farticle\u002Fd32ab414-1972-50fe-8c0a-208302f972b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ffederal-layoffs-trump-opm.html"},{"__typename":"EmbeddedInteractive","lastModified":"2025-01-22T20:54:52.365Z","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F0df9455a-1c52-5a54-9400-7443c43d9a68","url":"null"},{"__typename":"Article","lastModified":"2025-02-28T11:45:26.642Z","uri":"nyt:\u002F\u002Farticle\u002Fdcdfc485-364a-5a09-bb3d-79f645a6f129","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Fargentina-crypto-scandal-president.html"},{"__typename":"Article","lastModified":"2025-02-28T11:25:49.237Z","uri":"nyt:\u002F\u002Farticle\u002F85713c47-6ee2-52cc-84eb-02be4ce1ee1f","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fgene-hackman-death-new-mexico.html"},{"__typename":"Article","lastModified":"2025-02-28T12:44:37.741Z","uri":"nyt:\u002F\u002Farticle\u002Fcd0c66fd-7767-5885-b4d3-597efd65d1a0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fgene-hackman-death-updates.html"},{"__typename":"Interactive","lastModified":"2025-02-28T00:40:02.920Z","uri":"nyt:\u002F\u002Finteractive\u002Fd41992cc-6b6c-544d-a568-309e7846c9c9","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fmovies\u002Fhackman-affidavit.html"},{"__typename":"Article","lastModified":"2025-02-28T02:12:14.299Z","uri":"nyt:\u002F\u002Farticle\u002Fcb0fd188-e910-5caf-b97f-9959fe27aad8","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fmovies\u002Fgene-hackman-unforgiven-bonnie-clyde.html"},{"__typename":"Article","lastModified":"2025-02-28T11:30:16.929Z","uri":"nyt:\u002F\u002Farticle\u002F720399f2-c1ec-5608-9963-cfaa03abd183","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fasia\u002Fchina-military-drills-pacific.html"},{"__typename":"Article","lastModified":"2025-02-28T10:33:26.206Z","uri":"nyt:\u002F\u002Farticle\u002Ff86860c9-bc29-598c-bc53-5b81b1def8a5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fwest-bank-jenin-palestinian-authority-israel.html"},{"__typename":"Article","lastModified":"2025-02-28T11:02:22.739Z","uri":"nyt:\u002F\u002Farticle\u002Feed2db6f-2fad-5bf8-b96f-b8ad776652ff","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fsyria-ramadan-economy-cash-shortage.html"},{"__typename":"Article","lastModified":"2025-02-28T02:57:42.743Z","uri":"nyt:\u002F\u002Farticle\u002F2734f206-c837-5724-a971-94aedb7c0cc5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fiowa-transgender-civil-rights-bill.html"},{"__typename":"Article","lastModified":"2025-02-28T02:03:23.518Z","uri":"nyt:\u002F\u002Farticle\u002F2b8e7341-5edb-508e-81d3-079dc1d8f638","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrans-troops-pentagon-figures.html"},{"__typename":"Interactive","lastModified":"2025-02-28T06:21:51.581Z","uri":"nyt:\u002F\u002Finteractive\u002F1e284d42-0f2f-5814-a3d3-ad7fe8a1f4a1","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmedicaid-enrollment.html"},{"__typename":"Article","lastModified":"2025-02-28T10:03:42.174Z","uri":"nyt:\u002F\u002Farticle\u002F61bfc8df-d91b-50a6-aecc-b9a82d7da8d9","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fbest-picture-nominees-oscars-conclave-wicked.html"},{"__typename":"Article","lastModified":"2025-02-28T10:01:46.736Z","uri":"nyt:\u002F\u002Farticle\u002Fdd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Fdance\u002Fzoe-saldana-emilia-perez-dance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:39:21.985Z","uri":"nyt:\u002F\u002Farticle\u002Fb0813f6c-840e-5ae7-a88c-d5d430d3c564","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Femilia-perez-mexico-oscars.html"},{"__typename":"Article","lastModified":"2025-02-28T11:29:28.060Z","uri":"nyt:\u002F\u002Farticle\u002Feae58626-ae12-58cf-a801-1c30238d52ed","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fadams-corruption-new-charge.html"},{"__typename":"Article","lastModified":"2025-02-28T10:13:56.828Z","uri":"nyt:\u002F\u002Farticle\u002F2f9390e8-607b-51a0-b3ea-47be2f5821dc","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fandrew-cuomo-new-york-mayor.html"},{"__typename":"Article","lastModified":"2025-02-28T10:10:47.390Z","uri":"nyt:\u002F\u002Farticle\u002Ff04154fe-aaa0-5b58-bdd7-66b4439e06e5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fshopping-addiction-no-buy-2025.html"},{"__typename":"EmbeddedInteractive","lastModified":"2025-02-25T13:43:51.656Z","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F557659e5-f688-5fe8-bdc0-ed9d03c1fa1e","url":"null"},{"__typename":"Article","lastModified":"2025-02-28T10:02:00.449Z","uri":"nyt:\u002F\u002Farticle\u002Ff08fbb32-8818-558e-8473-71631754acea","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fdonald-trump-birthright-citizenship.html"},{"__typename":"Article","lastModified":"2025-02-28T12:08:49.046Z","uri":"nyt:\u002F\u002Farticle\u002F96f9592e-54a6-5dea-845b-785346ba26b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fandrew-tate-donald-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:26.255Z","uri":"nyt:\u002F\u002Farticle\u002F896e3424-8c01-5485-bb02-1560953263ba","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Fgovernment-great-progressive-abundance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:00:46.809Z","uri":"nyt:\u002F\u002Farticle\u002F5452cb92-2c7c-5c19-b4c5-863234fce6ab","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Felon-musk-south-africa.html"},{"__typename":"Article","lastModified":"2025-02-28T12:03:51.145Z","uri":"nyt:\u002F\u002Farticle\u002F0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ftexas-measles-vaccine.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:05.958Z","uri":"nyt:\u002F\u002Farticle\u002Ff716ba76-77d6-558b-9a94-bf1f5f1718b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmedicaid-republicans.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:16.383Z","uri":"nyt:\u002F\u002Farticle\u002Fe4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmatter-of-opinion-final-episode.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:38.344Z","uri":"nyt:\u002F\u002Farticle\u002Fa3f3b0d1-5608-54dd-a08b-83d185b5db46","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F25\u002Fopinion\u002Fthepoint\u002Fbarnard-college-sit-in-palestinian-israel-protest"},{"__typename":"Article","lastModified":"2025-02-28T12:12:33.018Z","uri":"nyt:\u002F\u002Farticle\u002Fe5fb45b5-1082-5fcc-ba9a-5bcc56a75975","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-hegseth-pentagon.html"},{"__typename":"Article","lastModified":"2025-02-28T02:33:52.611Z","uri":"nyt:\u002F\u002Farticle\u002F1b78bf9c-210e-5ae9-99a4-980a3696891d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-republicans-masculinity-gender-traditional.html"},{"__typename":"Article","lastModified":"2025-02-28T12:01:50.458Z","uri":"nyt:\u002F\u002Farticle\u002F351b30ec-12d0-5d59-9f63-5d81a895ded1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ffree-speech-trump-maga.html"},{"__typename":"Article","lastModified":"2025-02-28T10:00:12.600Z","uri":"nyt:\u002F\u002Farticle\u002F6717e682-229e-51b8-9947-2b735defd735","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fhorse-racing-government-subsidies.html"},{"__typename":"Article","lastModified":"2025-02-28T09:01:38.204Z","uri":"nyt:\u002F\u002Farticle\u002Ffe3aa929-085b-529c-9598-e16fc54c363b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Ftelevision\u002Fstephen-colbert-trump-voters-remorse.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Fcf10533b38444756bae93964a8419fb7","hierarchyType":"container","layoutType":"splitWidth","sourceId":"1a06d271-0acf-490f-80a9-2374d186ac4b","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F102ad4db-eae6-57df-8fe4-2ee24efce11f","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNodeEmbedded","children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Alan Feuer","id":"UGVyc29uOm55dDovL3BlcnNvbi84YmNlNDlmNC1lMjdiLTUyYTktOTRjNi1lM2Q2YzRlMDI0MzA=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F10\u002F20\u002Freader-center\u002Fauthor-alan-feuer\u002Fauthor-alan-feuer-thumbLarge.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjU3OTQyZWYtYTU0My01N2UzLTgzYWQtZjFjMTU0MjNlYjI3"}}],"renderedRepresentation":"By Alan Feuer"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-7cd260c2-752a-5a3c-9978-3e999d9b5dfa\u002Fjob-1740708896916\u002Farticle-7cd260c2-752a-5a3c-9978-3e999d9b5dfa-job-1740708896916.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Mexico Begins to Release Dozens of Cartel Operatives Into U.S. Custody"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vNDdkNzIyZDctYTcyNi01MGE2LWFkZTAtN2I4NGQyYzgzMDll","length":443,"sourceId":"100000010017605","uri":"nyt:\u002F\u002Faudio\u002F47d722d7-a726-50a6-ade0-7b84d2c8309e"}},"firstPublished":"2025-02-27T20:56:39.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Mexico Transfers Dozens of Cartel Operatives to U.S. Custody"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzdjZDI2MGMyLTc1MmEtNWEzYy05OTc4LTNlOTk5ZDliNWRmYQ==","lastModified":"2025-02-28T02:14:56.238Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZGE2MGRhY2QtZTNjNS01YzIwLWEyNTQtMzQxMGRiMjA4N2Zm"}},"section":{"__typename":"Section","displayName":"U.S.","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2EzNGQzZDZjLWM3N2YtNTkzMS1iOTUxLTI0MWI0ZTI4NjgxYw=="},"sourceId":"100000010017147","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002F7cd260c2-752a-5a3c-9978-3e999d9b5dfa","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmexico-cartel-sheinbaum-trump.html","wordCount":1146},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Vjosa Isai","id":"UGVyc29uOm55dDovL3BlcnNvbi81ZjAwZGE0Zi04YzM2LTU4NDgtODllMy1jNDU5ZmMyOGJmOTI=","promotionalMedia":null}],"renderedRepresentation":"By Vjosa Isai"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-adab900a-3ca2-5afe-b1d4-477fc6bb909e\u002Fjob-1740736914820\u002Farticle-adab900a-3ca2-5afe-b1d4-477fc6bb909e-job-1740736914820.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for How Canadians Are Making Their Anger Toward the U.S. Loud and Clear"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vNDNlNGU1OTEtMDM4NS01OWNmLWEzY2QtNmRhMzAyMmRmNTlh","length":415,"sourceId":"100000010019020","uri":"nyt:\u002F\u002Faudio\u002F43e4e591-0385-59cf-a3cd-6da3022df59a"}},"firstPublished":"2025-02-28T10:01:54.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"How Canadians Are Making Their Anger Toward the U.S. Loud and Clear"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2FkYWI5MDBhLTNjYTItNWFmZS1iMWQ0LTQ3N2ZjNmJiOTA5ZQ==","lastModified":"2025-02-28T11:31:37.813Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDY4MDdhOGQtZmEwNS01ZDVhLTgyZWMtNWFlNTczZGRlNWU0"}},"section":{"__typename":"Section","displayName":"World","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzcwZTg2NWI2LWNjNzAtNTE4MS04NGM5LTgzNjhiM2E1YzM0Yg=="},"sourceId":"100000010001722","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Fadab900a-3ca2-5afe-b1d4-477fc6bb909e","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fcanada\u002Fcanada-us-trump-tariffs-reactions.html","wordCount":1058},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DetailBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Matthew Goldstein","id":"UGVyc29uOm55dDovL3BlcnNvbi8xYTRkNGY3OC1jZDBmLTVjMDYtYTc0MS1lZjdhMmJkZGZkNTI=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F11\u002F06\u002Fmultimedia\u002Fauthor-matthew-goldstein\u002Fauthor-matthew-goldstein-thumbLarge-v2.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvMjU4NzQ1YzQtNjkwZi01ZWE4LTgxODQtYTRlMzg5YTY2OGQ4"}},{"__typename":"Person","displayName":"Glenn Thrush","id":"UGVyc29uOm55dDovL3BlcnNvbi9jODVkYzhjNC0xYmQ5LTU2MWUtYmMwNi0yNjhlZDFkZDFkYTc=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F01\u002F06\u002Freader-center\u002Fauthor-glenn-thrush\u002Fauthor-glenn-thrush-thumbLarge-v3.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZTU3YTBjZWItN2JkOS01MGUyLTg2ZDYtNDc0OWU0YThjZjVk"}}],"renderedRepresentation":"By Matthew Goldstein and Glenn Thrush"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-aff5b023-b154-5fd7-ac1a-bc207716b35c\u002Fjob-1740718469962\u002Farticle-aff5b023-b154-5fd7-ac1a-bc207716b35c-job-1740718469962.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for ‘Epstein Files’ Release That Pam Bondi Hyped Falls Short"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vNmVkNmM4MzktY2QyNC01MjQ4LWFhYTEtMjZhOWQ1ZjkxYTFl","length":405,"sourceId":"100000010018188","uri":"nyt:\u002F\u002Faudio\u002F6ed6c839-cd24-5248-aaa1-26a9d5f91a1e"}},"firstPublished":"2025-02-28T00:35:04.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"‘Epstein Files’ Release, Hyped by Pam Bondi, Falls Short of Expectations"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2FmZjViMDIzLWIxNTQtNWZkNy1hYzFhLWJjMjA3NzE2YjM1Yw==","lastModified":"2025-02-28T04:54:29.304Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjNlZGU3YjUtOWE1OS01ZTM5LWFmMTYtYzk5ZDlmY2IxNjhj"}},"section":{"__typename":"Section","displayName":"Business","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzA0MTViMmIwLTUxM2EtNWU3OC04MGRhLTIxYWI3NzBjYjc1Mw=="},"sourceId":"100000010016596","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Faff5b023-b154-5fd7-ac1a-bc207716b35c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fbusiness\u002Fjeffrey-epstein-files-pam-bondi.html","wordCount":1053},{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderBasicBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"DetailBlock"},{"__typename":"RelatedLinksBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Jonathan Wolfe","id":"UGVyc29uOm55dDovL3BlcnNvbi80ZDBjNmVlYS1mYjE1LTVjZDItODE3YS02ZmZmZDY0ZmUyODE=","promotionalMedia":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":150,"name":"thumbLarge","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2018\u002F08\u002F24\u002Fmultimedia\u002Fauthor-jonathan-wolfe\u002Fauthor-jonathan-wolfe-thumbLarge-v4.png","width":150}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZjU3NDExZjQtZThmZS01MzU3LTljYzEtYWFhNDU5MjA4YjE0"}}],"renderedRepresentation":"By Jonathan Wolfe"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-d1966252-a51e-5c6f-8d72-f5b7f05eb56d\u002Fjob-1740746998566\u002Farticle-d1966252-a51e-5c6f-8d72-f5b7f05eb56d-job-1740746998566.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Citigroup Makes an $81 Trillion Mistake"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vYTA2N2U0NTUtNDZjNi01MmJlLWI5ZDgtNGVlZDJkNzE2OTYw","length":149,"sourceId":"100000010019132","uri":"nyt:\u002F\u002Faudio\u002Fa067e455-46c6-52be-b9d8-4eed2d716960"}},"firstPublished":"2025-02-28T12:20:20.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Citigroup Makes (and Then Fixes) an $81 Trillion Mistake"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlL2QxOTY2MjUyLWE1MWUtNWM2Zi04ZDcyLWY1YjdmMDVlYjU2ZA==","lastModified":"2025-02-28T12:49:57.620Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZTE0Yjg4YTItNDQwMS01YzYzLTgyZmMtZGU2YWM2OGM3OWQ5"}},"section":{"__typename":"Section","displayName":"New York","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzM5NDgwMzc0LTY2ZDMtNTYwMy05Y2UxLTU4Y2ZhMTI5ODhlMg=="},"sourceId":"100000010019004","tone":"NEWS","uri":"nyt:\u002F\u002Farticle\u002Fd1966252-a51e-5c6f-8d72-f5b7f05eb56d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fcitigroup-81-trillion-error.html","wordCount":348}],"children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F196224a834d8415f8625d9dd2971fa2d","hierarchyType":"addOn","isPersonalized":true,"layoutType":"List","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":6,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002F2e6a9953-af59-5b39-9d52-992ea5831717","surface":"home-packages-morenews-addon"},"properties":"{\"packageTitleDisplay\":\"NONE\",\"packageTitle\":null,\"packageTitleDescription\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"HEADLINE\",\"twoUp\":false,\"showMedia\":false,\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"isNewsletter\":false,\"assets\":[]}","sourceId":"41e20e14-668c-4bc4-819a-5d1423088297","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F821410bc-70e6-5198-98e0-d97fbca531f7","url":"","variantAllocation":null,"children":[]}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fa6412bfef25c40a2b79ebace86524598","hierarchyType":"dropzone","sourceId":"05fabf27-8d2c-4a60-a74c-f9a778a11486b4ef7022-f2e2-41a4-8b74-d69187c998b0default","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F45ee3e57-97b2-5db6-b50d-07babdaddf7c","url":"","variantAllocation":null,"zoneKey":"default","assets":undefined,"media":undefined}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T02:14:56.238Z","uri":"nyt:\u002F\u002Farticle\u002F7cd260c2-752a-5a3c-9978-3e999d9b5dfa","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmexico-cartel-sheinbaum-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T11:31:37.813Z","uri":"nyt:\u002F\u002Farticle\u002Fadab900a-3ca2-5afe-b1d4-477fc6bb909e","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fcanada\u002Fcanada-us-trump-tariffs-reactions.html"},{"__typename":"Article","lastModified":"2025-02-28T04:54:29.304Z","uri":"nyt:\u002F\u002Farticle\u002Faff5b023-b154-5fd7-ac1a-bc207716b35c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fbusiness\u002Fjeffrey-epstein-files-pam-bondi.html"},{"__typename":"Article","lastModified":"2025-02-28T12:49:57.620Z","uri":"nyt:\u002F\u002Farticle\u002Fd1966252-a51e-5c6f-8d72-f5b7f05eb56d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fcitigroup-81-trillion-error.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F22b1dc11c72c405aa933191c05fdb67a","hierarchyType":"package","isPersonalized":false,"layoutType":"Standard","media":[{"__typename":"Image","altText":"A man in a yellow T-shirt behind prison bars.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Getting hold of Rafael Caro Quintero, a founding member of the Sinaloa drug cartel who was convicted in Mexico of masterminding the 1985 murder of an agent with the Drug Enforcement Administration, has been a priority for the agency."},"captionOverride":null,"credit":"Reuters","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-square640.jpg","width":640},{"__typename":"ImageRendition","height":1365,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-mediumSquareAt3X.jpg","width":1365}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl\u002F7dc-cartel1-bpwl-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-27T20:56:37.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZGE2MGRhY2QtZTNjNS01YzIwLWEyNTQtMzQxMGRiMjA4N2Zm","lastModified":"2025-02-27T22:39:46.359Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017450","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fda60dacd-e3c5-5c20-a254-3410db2087ff","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F7dc-cartel1-bpwl.html"},{"__typename":"Image","altText":"A sign in a store says, “Buy Canadian Instead.’’","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"A liquor store in Vancouver encouraging domestic purchases. Many Canadians are shunning U.S. products. "},"captionOverride":null,"credit":"Chris Helgren\u002FReuters","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz\u002F28canada-angry-explainer-1-vgkz-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T10:01:50.671Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDY4MDdhOGQtZmEwNS01ZDVhLTgyZWMtNWFlNTczZGRlNWU0","lastModified":"2025-02-28T10:01:50.671Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017397","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fd6807a8d-fa05-5d5a-82ec-5ae573dde5e4","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fmultimedia\u002F28canada-angry-explainer-1-vgkz.html"},{"__typename":"Image","altText":"Three people hold up binders in front of White House.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Several conservative influencers were offered a preview of the documents hours before they were released publicly."},"captionOverride":null,"credit":"Evan Vucci\u002FAssociated Press","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw\u002F00Epstein-bondi-vhbw-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T00:35:02.419Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYjNlZGU3YjUtOWE1OS01ZTM5LWFmMTYtYzk5ZDlmY2IxNjhj","lastModified":"2025-02-28T00:35:02.419Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010017804","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fb3ede7b5-9a59-5e39-af16-c99d9fcb168c","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F00Epstein-bondi-vhbw.html"},{"__typename":"Image","altText":"A woman walks into a door of a Citibank location in Manhattan.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Regulators have fined Citigroup repeatedly after a number of glitches in recent years."},"captionOverride":null,"credit":"Spencer Platt\u002FGetty Images","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-square640.jpg","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-mediumSquareAt3X.jpg","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02\u002F28xp-citigroup-00-02-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-28T12:20:18.448Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZTE0Yjg4YTItNDQwMS01YzYzLTgyZmMtZGU2YWM2OGM3OWQ5","lastModified":"2025-02-28T12:20:18.448Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"World","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzcwZTg2NWI2LWNjNzAtNTE4MS04NGM5LTgzNjhiM2E1YzM0Yg=="},"sourceId":"100000010019057","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fe14b88a2-4401-5c63-82fc-de6ac68c79d9","url":"\u002Fimagepages\u002F2025\u002F02\u002F28\u002Fworld\u002F28xp-citigroup-00-02.html"}],"personalizationConfig":null,"properties":"{\"packageDisplay\":null,\"packageMediaEmphasis\":null,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"More News\",\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"NONE\",\"isOpinion\":false,\"isBundle\":false,\"assets\":[{\"showMedia\":false,\"media\":{\"showCaption\":false,\"mutedAutoplayOverlay\":false,\"headline\":\"\",\"mobileAspectRatioCrop\":\"SQUARE\",\"uri\":\"nyt:\u002F\u002Fimage\u002Fda60dacd-e3c5-5c20-a254-3410db2087ff\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Mexico Transfers Dozens of Cartel Operatives to U.S. Custody\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The traffickers will face charges in U.S. courts. Among them is Rafael Caro Quintero, a Sinaloa cartel founder whom U.S. officials have been after for 40 years.\",\"thread\":null,\"sentence\":\"Mexico Begins to Release Dozens of Cartel Operatives Into U.S. Custody\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F7cd260c2-752a-5a3c-9978-3e999d9b5dfa\"},{\"showMedia\":true,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fd6807a8d-fa05-5d5a-82ec-5ae573dde5e4\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"How Canadians Are Making Their Anger Toward the U.S. Loud and Clear\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"Canadians are shunning U.S. products and abandoning trips to America to protest the economic punishment President Trump has threatened to impose with tariffs.\",\"thread\":null,\"sentence\":\"How Canadians Are Making Their Anger Toward the U.S. Loud and Clear\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fadab900a-3ca2-5afe-b1d4-477fc6bb909e\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fb3ede7b5-9a59-5e39-af16-c99d9fcb168c\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"‘Epstein Files’ Release, Hyped by Pam Bondi, Falls Short of Expectations\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The release of flight logs and Jeffrey Epstein’s contact list by the attorney general was met with criticism from those who had expected new information.\",\"thread\":null,\"sentence\":\"‘Epstein Files’ Release, Hyped by Pam Bondi, Falls Short\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Faff5b023-b154-5fd7-ac1a-bc207716b35c\"},{\"showMedia\":false,\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fe14b88a2-4401-5c63-82fc-de6ac68c79d9\"},\"videoSize\":\"LARGE\",\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"mediaCompanion\":null,\"mutedAutoplayOverlay\":false,\"display\":\"SUMMARY\",\"toneOverride\":\"NO_TONE_SET\",\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Citigroup Accidentally Credits Customer’s Account With $81 Trillion\",\"overrideByline\":false,\"byline\":null,\"italicizeHeadline\":false,\"summary\":\"The bank temporarily credited a customer’s account with trillions of dollars, adding to scrutiny of risk management systems after a series of errors.\",\"thread\":null,\"sentence\":\"Citigroup Makes an $81 Trillion Mistake\",\"bullets\":[\"\",\"\"],\"tickerLabel\":\"\",\"title\":null,\"hideLivePromoUpdatesLabel\":false,\"numberOfHeadlines\":2,\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002Fd1966252-a51e-5c6f-8d72-f5b7f05eb56d\"}]}","sourceId":"b4ef7022-f2e2-41a4-8b74-d69187c998b0","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fb692d35c-2c1d-54c0-ad47-106b902018b7","url":"","variantAllocation":null}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T02:14:56.238Z","uri":"nyt:\u002F\u002Farticle\u002F7cd260c2-752a-5a3c-9978-3e999d9b5dfa","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmexico-cartel-sheinbaum-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T11:31:37.813Z","uri":"nyt:\u002F\u002Farticle\u002Fadab900a-3ca2-5afe-b1d4-477fc6bb909e","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fcanada\u002Fcanada-us-trump-tariffs-reactions.html"},{"__typename":"Article","lastModified":"2025-02-28T04:54:29.304Z","uri":"nyt:\u002F\u002Farticle\u002Faff5b023-b154-5fd7-ac1a-bc207716b35c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fbusiness\u002Fjeffrey-epstein-files-pam-bondi.html"},{"__typename":"Article","lastModified":"2025-02-28T12:49:57.620Z","uri":"nyt:\u002F\u002Farticle\u002Fd1966252-a51e-5c6f-8d72-f5b7f05eb56d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fcitigroup-81-trillion-error.html"}],"eventId":"pubp:\u002F\u002Fevent\u002Ffad98ab9d7d44b61b68d2b3ade2f3cde","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"oslnqaefbhiyhbiggypdsxrbvrnzwuew","trackingName":"More News","uri":"nyt:\u002F\u002Fprogrammingnode\u002F95181666-a215-5349-91cf-a3906cd8c200","url":"","variantAllocation":null,"assets":undefined,"media":undefined}],"hierarchyType":"zone","trackingName":null,"assets":undefined,"media":undefined}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T02:14:56.238Z","uri":"nyt:\u002F\u002Farticle\u002F7cd260c2-752a-5a3c-9978-3e999d9b5dfa","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmexico-cartel-sheinbaum-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T11:31:37.813Z","uri":"nyt:\u002F\u002Farticle\u002Fadab900a-3ca2-5afe-b1d4-477fc6bb909e","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fcanada\u002Fcanada-us-trump-tariffs-reactions.html"},{"__typename":"Article","lastModified":"2025-02-28T04:54:29.304Z","uri":"nyt:\u002F\u002Farticle\u002Faff5b023-b154-5fd7-ac1a-bc207716b35c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fbusiness\u002Fjeffrey-epstein-files-pam-bondi.html"},{"__typename":"Article","lastModified":"2025-02-28T12:49:57.620Z","uri":"nyt:\u002F\u002Farticle\u002Fd1966252-a51e-5c6f-8d72-f5b7f05eb56d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fcitigroup-81-trillion-error.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F7fbeb03d26f24deaadb8211c49e41fa8","hierarchyType":"container","layoutType":"fullWidth","sourceId":"cdde64f0-1e18-45ac-8cd6-b49d603190da","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fd737b473-4f56-5e0b-af6f-3f83854dfdbe","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNodeEmbedded","children":[{"__typename":"ProgrammingNode","children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fab7500cdabf1488fa47a78c824163a8c","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"51d869e7-76ae-4873-a7a2-1d66a769d3c5","trackingName":"The Athletic - International","uri":"nyt:\u002F\u002Fprogrammingnode\u002F8db3c158-0355-5c86-bdc9-f82af151c18e","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F904c88b9e24941f5862725fa0852d407","hierarchyType":"package","isPersonalized":true,"layoutType":"Carousel2","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":5,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002Fe1f22e62-8dba-51f4-87bc-e552a87bb958","surface":"home-packages-well"},"properties":"{\"isOpinion\":false,\"isBundle\":false,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"Well\",\"packageTitleDescription\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"HEADLINE\",\"mediaEmphasis\":\"LARGE\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":null,\"assets\":[]}","sourceId":"50bc1c9e-9925-4b03-8e32-51281a57d8f9","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F585f0d2b-6b86-56a8-8548-9e742a96ffae","url":"","variantAllocation":null}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fa9a112534c714c269c8a26b020db17bd","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"sunntpzucepyujvaclvcfokoedpmwlgp","trackingName":"Well","uri":"nyt:\u002F\u002Fprogrammingnode\u002Fb8b5c3d2-e504-5a96-8549-a939b363d395","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Article","associatedNewsletter":null,"body":{"__typename":"DocumentBlock","content":[{"__typename":"HeaderFullBleedHorizontalBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"Heading2Block"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ImageBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"},{"__typename":"ParagraphBlock"}]},"bylines":[{"__typename":"Byline","creators":[{"__typename":"Person","displayName":"Lizzy Goodman","id":"UGVyc29uOm55dDovL3BlcnNvbi80MWE1M2E2OC00N2FjLTU0MTItODE2Ny1kN2U1ODZiMjNjOTg=","promotionalMedia":null}],"renderedRepresentation":"By Lizzy Goodman"}],"featuredAudio":{"__typename":"FeaturedAudio","asset":{"__typename":"Audio","audioContentType":"SYNTHETIC_NARRATION","fileUrl":"https:\u002F\u002Fstatic.nytimes.com\u002Fnarrated-articles\u002Fsynthetic\u002Farticle-911b0155-1b65-5576-91a0-215b59fb3215\u002Fjob-1740643232589\u002Farticle-911b0155-1b65-5576-91a0-215b59fb3215-job-1740643232589.mp3","headline":{"__typename":"CreativeWorkHeadline","default":"Synthetic Narration for Sharon Van Etten Finds Her Way Home"},"id":"QXVkaW86bnl0Oi8vYXVkaW8vZGJmMzA1ODgtMGI2MC01YTBiLTlhNDEtYzljMDFkMjE3MWU3","length":561,"sourceId":"100000010015840","uri":"nyt:\u002F\u002Faudio\u002Fdbf30588-0b60-5a0b-9a41-c9c01d2171e7"}},"firstPublished":"2025-02-27T08:00:14.000Z","headline":{"__typename":"CreativeWorkHeadline","default":"Sharon Van Etten Finds Her Way Home"},"id":"QXJ0aWNsZTpueXQ6Ly9hcnRpY2xlLzkxMWIwMTU1LTFiNjUtNTU3Ni05MWEwLTIxNWI1OWZiMzIxNQ==","lastModified":"2025-02-27T14:31:36.919Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-square640.jpg"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-threeByTwoLargeAt2X.jpg"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTZkOTAzMzItZWRmNC01MDQ3LWI4MjEtZDgyZjEyNDJkZjhm"}},"section":{"__typename":"Section","displayName":"Style","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzE0NmUyYzQ1LTY1ODYtNTllZi1iYzIzLTkwZTg4ZmUyY2YwYQ=="},"sourceId":"100000010004975","tone":"FEATURE","uri":"nyt:\u002F\u002Farticle\u002F911b0155-1b65-5576-91a0-215b59fb3215","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fstyle\u002Fsharon-van-etten-album.html","wordCount":1712}],"children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fc8a3c9b9b6774c9f8b13925da445dbb9","hierarchyType":"addOn","isPersonalized":true,"layoutType":"List","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":3,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002F674ce888-ee50-5046-ae86-1aaa16f9ad69","surface":"home-packages-culturelifestyle-addon"},"properties":"{\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"SUMMARY\",\"twoUp\":false,\"showMedia\":true,\"deepLink\":null,\"isOpinion\":false,\"isBundle\":false,\"isNewsletter\":false,\"assets\":[]}","sourceId":"615edc69-a64f-47e6-b6ac-2bc20dce7004","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F9683424b-e1c7-5595-8e51-5f9d69e6a6ea","url":"","variantAllocation":null,"children":[]}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F95a53a8ba9374c45993d4f7483263948","hierarchyType":"dropzone","sourceId":"489c7507-81b0-4292-9da5-f597ddcd7fc7f6b1fb86-2a9c-4767-87c3-e79ca3eb5f8cdefault","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fb5e92adf-16a5-5550-83ad-4ffb650dc44d","url":"","variantAllocation":null,"zoneKey":"default","assets":undefined,"media":undefined}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-27T14:31:36.919Z","uri":"nyt:\u002F\u002Farticle\u002F911b0155-1b65-5576-91a0-215b59fb3215","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fstyle\u002Fsharon-van-etten-album.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F4ac252b4422e4b438434896a3d4b29ed","hierarchyType":"package","isPersonalized":false,"layoutType":"HighlightLevel1","media":[{"__typename":"Image","altText":"A woman in a black coat sitting on the porch of a house, there are two doors behind her.","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":"Sharon Van Etten, who is currently touring for her seventh album, “Sharon Van Etten & The Attachment Theory,” returned to the New Jersey home she grew up in."},"captionOverride":null,"credit":"Sabrina Santiago for The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-smallSquare252.jpg","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-square640.jpg","width":640},{"__typename":"ImageRendition","height":1586,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-mediumSquareAt3X.jpg","width":1585}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1001,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-threeByTwoMediumAt2X.jpg","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-threeByTwoSmallAt2X.jpg","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-videoSixteenByNine1050.jpg","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm\u002F25SHARON-VAN-ETTEN-05-pkgm-verticalTwoByThree735.jpg","width":735}]}],"firstPublished":"2025-02-27T08:00:11.478Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTZkOTAzMzItZWRmNC01MDQ3LWI4MjEtZDgyZjEyNDJkZjhm","lastModified":"2025-02-27T08:00:11.478Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000010009423","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fa6d90332-edf4-5047-b821-d82f1242df8f","url":"\u002Fimagepages\u002F2025\u002F02\u002F27\u002Fmultimedia\u002F25SHARON-VAN-ETTEN-05-pkgm.html"}],"personalizationConfig":null,"properties":"{\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"Culture and Lifestyle\",\"packageTitleDescription\":null,\"packageTitleOverride\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"nytimes:\u002F\u002Fhome\u002Flifestyle\",\"isBundle\":false,\"assets\":[{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fa6d90332-edf4-5047-b821-d82f1242df8f\"},\"mediaEmphasis\":\"LARGE\",\"fullWidthMedia\":false,\"display\":\"SUMMARY\",\"kicker\":null,\"headline\":\"Sharon Van Etten Finds Her Way Home\",\"summary\":\"For her seventh album, the indie singer-songwriter comes to New Jersey to play on her old turf and process the past.\",\"bullets\":[\"\",\"\"],\"style\":\"STANDARD_LIGHT\",\"position\":\"LEFT\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"uri\":\"nyt:\u002F\u002Farticle\u002F911b0155-1b65-5576-91a0-215b59fb3215\"}]}","sourceId":"f6b1fb86-2a9c-4767-87c3-e79ca3eb5f8c","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F8010feff-26a9-5eae-987d-7faafe33368c","url":"","variantAllocation":null}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-27T14:31:36.919Z","uri":"nyt:\u002F\u002Farticle\u002F911b0155-1b65-5576-91a0-215b59fb3215","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fstyle\u002Fsharon-van-etten-album.html"}],"eventId":"pubp:\u002F\u002Fevent\u002F7e3b0e6bbe634790b6536fe272243fb1","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"qhvjwqipkalpjewgtqmnnsiiwbcfjywa","trackingName":"Culture and Lifestyle","uri":"nyt:\u002F\u002Fprogrammingnode\u002F8dea1c5d-236f-5d22-9a4f-9ecaa1111ed9","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F3e0a7c5a1d444238baf51cc69d2b9aaf","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"3ae701a0-049e-4983-9a85-a1f9b8b20031","trackingName":"Burst Mobile Only Below C&L","uri":"nyt:\u002F\u002Fprogrammingnode\u002Ffcb15af4-b4b7-583a-b1f5-c8e161562a26","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F0b2967dab25c4f2b81f862f8c0eece55","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"f3a33914-af8b-4119-9b2c-bb26ae307c94","trackingName":"Subbrand Reorder List","uri":"nyt:\u002F\u002Fprogrammingnode\u002F8b2344f4-fd68-5f26-92d0-2223dfde0c8b","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Ffcb9ba72e7a1420593e4515dc5ecd9f6","hierarchyType":"addOn","isPersonalized":true,"layoutType":"List","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":3,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002F0e21e8fc-4567-5b8a-9e8f-9e786aed2736","surface":"home-packages-athletic-addon"},"properties":"{\"isOpinion\":false,\"isBundle\":true,\"isNewsletter\":false,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":null,\"packageTitleDescription\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"HEADLINE\",\"twoUp\":false,\"showMedia\":false,\"deepLink\":null,\"assets\":[]}","sourceId":"d70317e2-6c2e-422b-8b60-97476c333763","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F37736f4f-9cb9-5d34-a7f6-c31108a906cf","url":"","variantAllocation":null,"children":[]},{"__typename":"ProgrammingNode","assets":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F843fac76242a488a873415371b2a66a0","hierarchyType":"addOn","isPersonalized":false,"layoutType":"AthleticGeoAddOn","media":[],"personalizationConfig":null,"properties":"{\"banner\":null,\"assets\":[]}","sourceId":"977413cb-625f-4bb8-90dc-c05dde343a59","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F8c32bb3f-3b4f-5ba6-b2bb-3474ec225279","url":"","variantAllocation":null,"children":[]}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Ff7f6e2c234b94a0c8551670052b03615","hierarchyType":"dropzone","sourceId":"529d8ed7-a1a9-41fb-beeb-00eb01fdf5ffdefault","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F4b186ab3-d145-5f6e-a1e3-30f359081140","url":"","variantAllocation":null,"zoneKey":"default","assets":undefined,"media":undefined}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F6ee9b83e1db74681a7ae74d97e776eb5","hierarchyType":"package","isPersonalized":true,"layoutType":"Standard","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":3,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002F0e21e8fc-4567-5b8a-9e8f-9e786aed2736","surface":"home-packages-athletic-primary"},"properties":"{\"packageDisplay\":\"ALL_HEDSUMS\",\"packageMediaEmphasis\":\"LARGE\",\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"The Athletic\",\"packageTitleDescription\":\"Sports coverage\",\"packageTitleOverride\":null,\"enablePackageTitleLink\":true,\"packageTitleSection\":\"ATHLETIC\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"nytimes:\u002F\u002Fhome\u002Fthe_athletic\",\"isOpinion\":false,\"isBundle\":true,\"assets\":[]}","sourceId":"a269d6b6-967e-4c2c-bd5a-65e224967c41","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F32849ce2-de18-5952-9e88-531bf38d85c8","url":"","variantAllocation":null}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002Fb14ca12dc105414887bc6d72504d920c","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"25c07b2f-d417-46fa-a820-b1761ebbe820","trackingName":"The Athletic","uri":"nyt:\u002F\u002Fprogrammingnode\u002Fd382e15c-9954-5bb5-9a25-62bb583b36e0","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F55c1a207c50943ff923bee0ab53beee9","hierarchyType":"package","isPersonalized":true,"layoutType":"List","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":4,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002Facab6b50-b23d-57e0-a603-d84178673340","surface":"home-packages-audio"},"properties":"{\"isOpinion\":false,\"isBundle\":true,\"isNewsletter\":false,\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"Audio\",\"packageTitleDescription\":\"Podcasts and narrated articles\",\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"HEADLINE\",\"twoUp\":false,\"showMedia\":true,\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"nytimes:\u002F\u002Flisten\",\"assets\":[]}","sourceId":"e2f6d91b-ce35-4364-a811-fdc89269f86d","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Fad4c4839-7a9d-576b-b405-888facf931b1","url":"","variantAllocation":null}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F416a51bec3d34771a0d56d03e940cc3b","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"5ff48d3c-3f95-4055-a561-b616fb9de817","trackingName":"Audio","uri":"nyt:\u002F\u002Fprogrammingnode\u002Fbc01bae0-4bab-5c16-a6fe-13d5e61a9084","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"children":[{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F656820661ef5439297cd8228f64a7e13","hierarchyType":"addOn","isPersonalized":true,"layoutType":"List","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":4,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002Ff19717b4-1fea-5cd7-881b-984b32713de7","surface":"home-packages-cooking-addon"},"properties":"{\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"Most Popular This Week\",\"packageTitleDescription\":null,\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"SUMMARY\",\"twoUp\":true,\"showMedia\":true,\"deepLink\":null,\"isOpinion\":false,\"isBundle\":false,\"isNewsletter\":false,\"assets\":[]}","sourceId":"f238d022-c728-4001-9de1-a5f5baeccebb","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F5768f238-e5cc-5c61-b48e-a38b28e49419","url":"","variantAllocation":null,"children":[]}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F78b49ec9a86b49cebde41c7f865356b2","hierarchyType":"dropzone","sourceId":"6305e572-30e0-4011-b3b6-4692c39cd5f448dde270-7ae6-482b-b2fe-1ac690350deddefault","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F9241f13c-75e3-57ec-b6bb-3856b274920a","url":"","variantAllocation":null,"zoneKey":"default","assets":undefined,"media":undefined}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F1a6d313076894c728c70656f3ca17e6c","hierarchyType":"package","isPersonalized":true,"layoutType":"Standard","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":2,"poolOverrideUri":"nyt:\u002F\u002Fprogrammingnode\u002Fc05837eb-bbce-5e4a-8fe8-31725e361b43","surface":"home-packages-cooking-primary"},"properties":"{\"packageDisplay\":\"ALL_HEDSUMS\",\"packageMediaEmphasis\":\"LARGE\",\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"Cooking\",\"packageTitleDescription\":\"Recipes and guides\",\"packageTitleOverride\":null,\"enablePackageTitleLink\":true,\"packageTitleSection\":\"COOKING\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"nytimes:\u002F\u002Fhome\u002Fcooking\",\"isOpinion\":false,\"isBundle\":true,\"assets\":[]}","sourceId":"48dde270-7ae6-482b-b2fe-1ac690350ded","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F3dedfaae-3f8f-58e6-84f2-598bcfd61c7f","url":"","variantAllocation":null}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F801fcd514a6d4e3db1ba15ab8cb9f69b","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"5eea0342-435d-45be-8312-0fa3a737d920","trackingName":"Cooking","uri":"nyt:\u002F\u002Fprogrammingnode\u002F0b3ff182-4f1d-5094-b7a8-bbdd257dc3e2","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[],"children":[],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F84c72c802af14417b3cabc95c456494f","hierarchyType":"package","isPersonalized":true,"layoutType":"Standard","media":[],"personalizationConfig":{"__typename":"PersonalizationConfig","assetCount":4,"poolOverrideUri":null,"surface":"home-packages-wirecutter"},"properties":"{\"packageDisplay\":\"ALL_HEDSUMS\",\"packageMediaEmphasis\":\"LARGE\",\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"Wirecutter\",\"packageTitleDescription\":\"Product recommendations\",\"packageTitleOverride\":null,\"enablePackageTitleLink\":true,\"packageTitleSection\":\"WIRECUTTER\",\"bannerDisplay\":\"NONE\",\"banner\":null,\"bannerOverride\":null,\"italicizeBanner\":false,\"bannerNewsStatus\":\"NONE\",\"mobileOverlay\":false,\"position\":\"BOTTOM\",\"color\":\"WHITE\",\"gradient\":\"SHOW\",\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"nytimes:\u002F\u002Fhome\u002Fwirecutter\",\"isOpinion\":false,\"isBundle\":true,\"assets\":[]}","sourceId":"8d18bf34-a1b5-47aa-8529-daa52a6edc4a","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F471920bf-1478-59cc-bdc5-e6f239b8c13c","url":"","variantAllocation":null}],"descendantAssets":[],"eventId":"pubp:\u002F\u002Fevent\u002F105208c6af194871b7bd78aa2525a870","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"cf87b184-e7a2-4ce7-be00-e79eaff04264","trackingName":"Wirecutter","uri":"nyt:\u002F\u002Fprogrammingnode\u002F456ed27e-f2cb-5fda-adbe-516178e45545","url":"","variantAllocation":null,"assets":undefined,"media":undefined},{"__typename":"ProgrammingNode","children":[{"__typename":"ProgrammingNode","assets":[{"__typename":"Promo","firstPublished":"2025-02-27T21:33:26.309Z","id":"UHJvbW86bnl0Oi8vcHJvbW8vYzUyNjY4MGEtNDZiOS01YzYzLTgzMTMtYzNhZWZiMGMyZGYz","lastModified":"2025-02-27T21:33:26.309Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F03\u002F02\u002Fcrosswords\u002Falpha-wordle-icon-new\u002Falpha-wordle-icon-new-threeByTwoLargeAt2X-v3.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjJkNjgzZDAtZGI2Ni01MDE2LWI5ZWQtYTY1NGU3ODJhYmQy"}},"sourceId":"100000008226470","targetUrl":"https:\u002F\u002Fwww.nytimes.com\u002Fgames\u002Fwordle\u002Findex.html","uri":"nyt:\u002F\u002Fpromo\u002Fc526680a-46b9-5c63-8313-c3aefb0c2df3","url":""},{"__typename":"Promo","firstPublished":"2025-02-27T21:33:26.346Z","id":"UHJvbW86bnl0Oi8vcHJvbW8vMDNiNDYxZTMtMzFiYi01ZmQwLWJlNTItOGZhM2UyN2M4ZTZi","lastModified":"2025-02-27T21:33:26.346Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F08\u002F25\u002Fcrosswords\u002Falpha-connections-icon-original\u002Falpha-connections-icon-original-square640.png"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F08\u002F25\u002Fcrosswords\u002Falpha-connections-icon-original\u002Falpha-connections-icon-original-threeByTwoLargeAt2X.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNGNiZTQ0ZGQtNDcxYS01NGVlLTkyYWUtOGRlNTMxNTliMjhj"}},"sourceId":"100000009395807","targetUrl":"https:\u002F\u002Fwww.nytimes.com\u002Fgames\u002Fconnections?GAMES_connectionsRollout_1130=1_ConnectionsV2","uri":"nyt:\u002F\u002Fpromo\u002F03b461e3-31bb-5fd0-be52-8fa3e27c8e6b","url":""},{"__typename":"Promo","firstPublished":"2025-02-27T21:33:26.322Z","id":"UHJvbW86bnl0Oi8vcHJvbW8vYjJhNTZiZGQtNDBkYS01NGUwLTljNzEtNDJjYzc0YzcxNzY1","lastModified":"2025-02-27T21:33:26.322Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-square640.png"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-threeByTwoLargeAt2X.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTkxZmM1MWMtYzUzZS01Y2UwLWFkYWQtNGUwZTQ3NDIxN2E1"}},"sourceId":"100000009323601","targetUrl":"https:\u002F\u002Fwww.nytimes.com\u002Fgames\u002Fstrands","uri":"nyt:\u002F\u002Fpromo\u002Fb2a56bdd-40da-54e0-9c71-42cc74c71765","url":""},{"__typename":"Promo","firstPublished":"2025-02-27T21:33:26.334Z","id":"UHJvbW86bnl0Oi8vcHJvbW8vODc4OGE2ZTktMGRiMS01YzdjLWE4ZTgtZDgyYzM3Y2NmZGQw","lastModified":"2025-02-27T21:33:26.334Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-square640-v4.png"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-threeByTwoLargeAt2X-v4.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOWEyYzkzNTktMjQ2Ny01MTQ0LTg2MzItMzA2NTliYjcwMWRl"}},"sourceId":"100000006468040","targetUrl":"https:\u002F\u002Fwww.nytimes.com\u002Fpuzzles\u002Fspelling-bee","uri":"nyt:\u002F\u002Fpromo\u002F8788a6e9-0db1-5c7c-a8e8-d82c37ccfdd0","url":""},{"__typename":"Promo","firstPublished":"2025-02-27T21:33:26.309Z","id":"UHJvbW86bnl0Oi8vcHJvbW8vZjdmMTIyNWQtNmJiYS01ODY0LWFkODAtNjgyMTIyYTlmYmJm","lastModified":"2025-02-27T21:33:26.309Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-square640-v3.png"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-threeByTwoLargeAt2X-v4.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTg4MjcxYzctYWFhNi01ZGE1LTkxZTgtNjMxMzEwODE3YmZl"}},"sourceId":"100000005085855","targetUrl":"https:\u002F\u002Fwww.nytimes.com\u002Fcrosswords","uri":"nyt:\u002F\u002Fpromo\u002Ff7f1225d-6bba-5864-ad80-682122a9fbbf","url":""},{"__typename":"Promo","firstPublished":"2025-02-27T21:33:26.323Z","id":"UHJvbW86bnl0Oi8vcHJvbW8vYzM5N2QyZDItNzU4YS01YTNhLWE4OTctMmFkZDZjZjlhZTc4","lastModified":"2025-02-27T21:33:26.323Z","promotionalImage":{"__typename":"PromotionalImage","image":{"__typename":"Image","crops":[{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-square640-v4.png"}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-threeByTwoLargeAt2X-v4.png"}]}],"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDAxMWU4ODktZDEwYi01N2IyLWFiMWQtMTg3MmZiYTBkMjU5"}},"sourceId":"100000005085847","targetUrl":"http:\u002F\u002Fwww.nytimes.com\u002Fcrosswords\u002Fgame\u002Fmini","uri":"nyt:\u002F\u002Fpromo\u002Fc397d2d2-758a-5a3a-a897-2add6cf9ae78","url":""}],"children":[],"descendantAssets":[{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.309Z","uri":"nyt:\u002F\u002Fpromo\u002Fc526680a-46b9-5c63-8313-c3aefb0c2df3","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.346Z","uri":"nyt:\u002F\u002Fpromo\u002F03b461e3-31bb-5fd0-be52-8fa3e27c8e6b","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.322Z","uri":"nyt:\u002F\u002Fpromo\u002Fb2a56bdd-40da-54e0-9c71-42cc74c71765","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.334Z","uri":"nyt:\u002F\u002Fpromo\u002F8788a6e9-0db1-5c7c-a8e8-d82c37ccfdd0","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.309Z","uri":"nyt:\u002F\u002Fpromo\u002Ff7f1225d-6bba-5864-ad80-682122a9fbbf","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.323Z","uri":"nyt:\u002F\u002Fpromo\u002Fc397d2d2-758a-5a3a-a897-2add6cf9ae78","url":""}],"eventId":"pubp:\u002F\u002Fevent\u002F556571055af742ee9332ddd6c388de48","hierarchyType":"package","isPersonalized":false,"layoutType":"List","media":[{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F03\u002F02\u002Fcrosswords\u002Falpha-wordle-icon-new\u002Falpha-wordle-icon-new-smallSquare252-v3.png","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":321,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F03\u002F02\u002Fcrosswords\u002Falpha-wordle-icon-new\u002Falpha-wordle-icon-new-mediumSquareAt3X-v3.png","width":321}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":214,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F03\u002F02\u002Fcrosswords\u002Falpha-wordle-icon-new\u002Falpha-wordle-icon-new-threeByTwoMediumAt2X-v3.png","width":321},{"__typename":"ImageRendition","height":214,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2022\u002F03\u002F02\u002Fcrosswords\u002Falpha-wordle-icon-new\u002Falpha-wordle-icon-new-threeByTwoSmallAt2X-v3.png","width":321}]}],"firstPublished":"2022-03-02T15:35:59.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNjJkNjgzZDAtZGI2Ni01MDE2LWI5ZWQtYTY1NGU3ODJhYmQy","lastModified":"2024-06-21T21:08:11.727Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Gameplay","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2QyMzE0Mzc0LWQ4OWUtNTU1Mi1iMGE3LTc2NDBiMjNiNzM4Zg=="},"sourceId":"100000008235682","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F62d683d0-db66-5016-b9ed-a654e782abd2","url":"\u002Fimagepages\u002F2022\u002F03\u002F02\u002Fcrosswords\u002Falpha-wordle-icon-new.html"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"NYT Games","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F08\u002F25\u002Fcrosswords\u002Falpha-connections-icon-original\u002Falpha-connections-icon-original-smallSquare252.png","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F08\u002F25\u002Fcrosswords\u002Falpha-connections-icon-original\u002Falpha-connections-icon-original-square640.png","width":640},{"__typename":"ImageRendition","height":640,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F08\u002F25\u002Fcrosswords\u002Falpha-connections-icon-original\u002Falpha-connections-icon-original-mediumSquareAt3X.png","width":640}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":428,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F08\u002F25\u002Fcrosswords\u002Falpha-connections-icon-original\u002Falpha-connections-icon-original-threeByTwoMediumAt2X.png","width":641},{"__typename":"ImageRendition","height":401,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2023\u002F08\u002F25\u002Fcrosswords\u002Falpha-connections-icon-original\u002Falpha-connections-icon-original-threeByTwoSmallAt2X.png","width":600}]}],"firstPublished":"2023-08-25T14:26:25.735Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvNGNiZTQ0ZGQtNDcxYS01NGVlLTkyYWUtOGRlNTMxNTliMjhj","lastModified":"2023-08-25T14:26:25.735Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Gameplay","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2QyMzE0Mzc0LWQ4OWUtNTU1Mi1iMGE3LTc2NDBiMjNiNzM4Zg=="},"sourceId":"100000009057582","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F4cbe44dd-471a-54ee-92ae-8de53159b28c","url":"\u002Fimagepages\u002F2023\u002F08\u002F25\u002Fcrosswords\u002Falpha-connections-icon-original.html"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-smallSquare252.png","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-square640.png","width":640},{"__typename":"ImageRendition","height":1281,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-mediumSquareAt3X.png","width":1281}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":854,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-threeByTwoMediumAt2X.png","width":1281},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-threeByTwoSmallAt2X.png","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-videoSixteenByNine1050.png","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1101,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon\u002Falpha-strands-icon-verticalTwoByThree735.png","width":735}]}],"firstPublished":"2024-01-16T14:09:02.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOTkxZmM1MWMtYzUzZS01Y2UwLWFkYWQtNGUwZTQ3NDIxN2E1","lastModified":"2024-07-20T00:35:31.118Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Gameplay","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2QyMzE0Mzc0LWQ4OWUtNTU1Mi1iMGE3LTc2NDBiMjNiNzM4Zg=="},"sourceId":"100000009267557","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F991fc51c-c53e-5ce0-adad-4e0e474217a5","url":"\u002Fimagepages\u002F2024\u002F01\u002F16\u002Fcrosswords\u002Falpha-strands-icon.html"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-smallSquare252-v4.png","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-square640-v4.png","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-mediumSquareAt3X-v4.png","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-threeByTwoMediumAt2X-v4.png","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-threeByTwoSmallAt2X-v4.png","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-videoSixteenByNine1050-v4.png","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res\u002Fspelling-bee-logo-nytgames-hi-res-verticalTwoByThree735-v4.png","width":735}]}],"firstPublished":"2020-03-24T00:43:09.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvOWEyYzkzNTktMjQ2Ny01MTQ0LTg2MzItMzA2NTliYjcwMWRl","lastModified":"2021-04-25T13:24:30.402Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Gameplay","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2QyMzE0Mzc0LWQ4OWUtNTU1Mi1iMGE3LTc2NDBiMjNiNzM4Zg=="},"sourceId":"100000007050686","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002F9a2c9359-2467-5144-8632-30659bb701de","url":"\u002Fimagepages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fspelling-bee-logo-nytgames-hi-res.html"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"The New York Times","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-smallSquare252-v3.png","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-square640-v3.png","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-mediumSquareAt3X-v3.png","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-threeByTwoMediumAt2X-v4.png","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-threeByTwoSmallAt2X-v4.png","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-videoSixteenByNine1050-v4.png","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires\u002Fcrossword-logo-nytgames-hires-verticalTwoByThree735-v3.png","width":735}]}],"firstPublished":"2020-03-23T16:50:35.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvYTg4MjcxYzctYWFhNi01ZGE1LTkxZTgtNjMxMzEwODE3YmZl","lastModified":"2024-07-02T16:53:05.127Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Gameplay","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uL2QyMzE0Mzc0LWQ4OWUtNTU1Mi1iMGE3LTc2NDBiMjNiNzM4Zg=="},"sourceId":"100000007049575","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fa88271c7-aaa6-5da5-91e8-631310817bfe","url":"\u002Fimagepages\u002F2020\u002F03\u002F23\u002Fcrosswords\u002Fcrossword-logo-nytgames-hires.html"},{"__typename":"Image","altText":"","bylines":[],"caption":{"__typename":"TextOnlyDocumentBlock","text":""},"captionOverride":null,"credit":"","crops":[{"__typename":"ImageCrop","name":"SMALL_SQUARE","renditions":[{"__typename":"ImageRendition","height":252,"name":"smallSquare252","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-smallSquare252-v4.png","width":252}]},{"__typename":"ImageCrop","name":"MEDIUM_SQUARE","renditions":[{"__typename":"ImageRendition","height":640,"name":"square640","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-square640-v4.png","width":640},{"__typename":"ImageRendition","height":1800,"name":"mediumSquareAt3X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-mediumSquareAt3X-v4.png","width":1800}]},{"__typename":"ImageCrop","name":"THREE_BY_TWO","renditions":[{"__typename":"ImageRendition","height":1000,"name":"threeByTwoMediumAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-threeByTwoMediumAt2X-v4.png","width":1500},{"__typename":"ImageRendition","height":400,"name":"threeByTwoSmallAt2X","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-threeByTwoSmallAt2X-v4.png","width":600}]},{"__typename":"ImageCrop","name":"SIXTEEN_BY_NINE","renditions":[{"__typename":"ImageRendition","height":591,"name":"videoSixteenByNine1050","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-videoSixteenByNine1050-v4.png","width":1050}]},{"__typename":"ImageCrop","name":"TWO_BY_THREE","renditions":[{"__typename":"ImageRendition","height":1103,"name":"verticalTwoByThree735","url":"https:\u002F\u002Fstatic01.nyt.com\u002Fimages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800\u002Falpha-mini-promo-1616527576800-verticalTwoByThree735-v4.png","width":735}]}],"firstPublished":"2021-03-23T19:27:34.000Z","hideCaption":false,"id":"SW1hZ2U6bnl0Oi8vaW1hZ2UvZDAxMWU4ODktZDEwYi01N2IyLWFiMWQtMTg3MmZiYTBkMjU5","lastModified":"2024-07-12T21:01:06.551Z","promotionalImage":null,"section":{"__typename":"Section","displayName":"Multimedia\u002FPhotos","id":"U2VjdGlvbjpueXQ6Ly9zZWN0aW9uLzRkMmVhODg1LWE3NWQtNTIyZi05NjNjLThmNWE1NjNkZTk0MA=="},"sourceId":"100000007670527","summary":"","timesTags":[],"tone":"NO_TONE_SET","uri":"nyt:\u002F\u002Fimage\u002Fd011e889-d10b-57b2-ab1d-1872fba0d259","url":"\u002Fimagepages\u002F2021\u002F03\u002F23\u002Fmultimedia\u002Falpha-mini-promo-1616527576800.html"}],"personalizationConfig":null,"properties":"{\"packageTitleDisplay\":\"SHOW\",\"packageTitle\":\"Games\",\"packageTitleDescription\":\"Daily puzzles\",\"enablePackageTitleLink\":false,\"packageTitleSection\":\"\",\"display\":\"SUMMARY\",\"twoUp\":false,\"showMedia\":true,\"kickersDisplay\":\"DISPLAY_ALL\",\"deepLink\":\"nytimes:\u002F\u002Fplay\",\"isOpinion\":false,\"isBundle\":true,\"isNewsletter\":false,\"assets\":[{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F62d683d0-db66-5016-b9ed-a654e782abd2\"},\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Wordle\",\"summary\":\"Guess the 5-letter word with 6 chances.\",\"thread\":null,\"sentence\":\"Wordle\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Fpromo\u002Fc526680a-46b9-5c63-8313-c3aefb0c2df3\"},{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F4cbe44dd-471a-54ee-92ae-8de53159b28c\"},\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Connections\",\"summary\":\"Group words that share a common thread.\",\"thread\":null,\"sentence\":\"Connections\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Fpromo\u002F03b461e3-31bb-5fd0-be52-8fa3e27c8e6b\"},{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F991fc51c-c53e-5ce0-adad-4e0e474217a5\"},\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Strands\",\"summary\":\"Uncover hidden words and reveal the theme.\",\"thread\":null,\"sentence\":\"Try Spelling Bee\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Fpromo\u002Fb2a56bdd-40da-54e0-9c71-42cc74c71765\"},{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002F9a2c9359-2467-5144-8632-30659bb701de\"},\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"Spelling Bee\",\"summary\":\"How many words can you make with 7 letters?\",\"thread\":null,\"sentence\":\"Try Spelling Bee\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Fpromo\u002F8788a6e9-0db1-5c7c-a8e8-d82c37ccfdd0\"},{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fa88271c7-aaa6-5da5-91e8-631310817bfe\"},\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"The Crossword\",\"summary\":\"Get clued in with wordplay, every day.\",\"thread\":null,\"sentence\":\"The Crossword, Vertex and More\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Fpromo\u002Ff7f1225d-6bba-5864-ad80-682122a9fbbf\"},{\"media\":{\"headline\":null,\"uri\":\"nyt:\u002F\u002Fimage\u002Fd011e889-d10b-57b2-ab1d-1872fba0d259\"},\"newsStatus\":\"NONE\",\"kicker\":null,\"headline\":\"The Mini Crossword\",\"summary\":\"Solve this bite-sized puzzle in just a few minutes.\",\"thread\":null,\"sentence\":\"The Daily Mini Crossword\",\"disableTimeToRead\":false,\"disableBundleSignal\":false,\"isReporterRead\":false,\"uri\":\"nyt:\u002F\u002Fpromo\u002Fc397d2d2-758a-5a3a-a897-2add6cf9ae78\"}]}","sourceId":"8dc5c226-01c8-4920-8c0b-dd2de3111767","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F3446d55f-beb9-581f-91d0-769509969e74","url":"","variantAllocation":null}],"descendantAssets":[{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.309Z","uri":"nyt:\u002F\u002Fpromo\u002Fc526680a-46b9-5c63-8313-c3aefb0c2df3","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.346Z","uri":"nyt:\u002F\u002Fpromo\u002F03b461e3-31bb-5fd0-be52-8fa3e27c8e6b","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.322Z","uri":"nyt:\u002F\u002Fpromo\u002Fb2a56bdd-40da-54e0-9c71-42cc74c71765","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.334Z","uri":"nyt:\u002F\u002Fpromo\u002F8788a6e9-0db1-5c7c-a8e8-d82c37ccfdd0","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.309Z","uri":"nyt:\u002F\u002Fpromo\u002Ff7f1225d-6bba-5864-ad80-682122a9fbbf","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.323Z","uri":"nyt:\u002F\u002Fpromo\u002Fc397d2d2-758a-5a3a-a897-2add6cf9ae78","url":""}],"eventId":"pubp:\u002F\u002Fevent\u002Fea4d962547b14c319c36540272ca7736","hierarchyType":"list","isPersonalized":false,"personalizationConfig":null,"sourceId":"ed995e63-fa85-4a51-b597-f9da3bbf4e9e","trackingName":"Play","uri":"nyt:\u002F\u002Fprogrammingnode\u002Fc0597fe3-e09f-5499-976c-226fe8db9fca","url":"","variantAllocation":null,"assets":undefined,"media":undefined}],"hierarchyType":"zone","trackingName":null,"assets":undefined,"media":undefined}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-27T14:31:36.919Z","uri":"nyt:\u002F\u002Farticle\u002F911b0155-1b65-5576-91a0-215b59fb3215","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fstyle\u002Fsharon-van-etten-album.html"},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.309Z","uri":"nyt:\u002F\u002Fpromo\u002Fc526680a-46b9-5c63-8313-c3aefb0c2df3","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.346Z","uri":"nyt:\u002F\u002Fpromo\u002F03b461e3-31bb-5fd0-be52-8fa3e27c8e6b","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.322Z","uri":"nyt:\u002F\u002Fpromo\u002Fb2a56bdd-40da-54e0-9c71-42cc74c71765","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.334Z","uri":"nyt:\u002F\u002Fpromo\u002F8788a6e9-0db1-5c7c-a8e8-d82c37ccfdd0","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.309Z","uri":"nyt:\u002F\u002Fpromo\u002Ff7f1225d-6bba-5864-ad80-682122a9fbbf","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.323Z","uri":"nyt:\u002F\u002Fpromo\u002Fc397d2d2-758a-5a3a-a897-2add6cf9ae78","url":""}],"eventId":"pubp:\u002F\u002Fevent\u002F3fc3da8ff02f439595578afedaf2a826","hierarchyType":"container","layoutType":"fullWidth","sourceId":"b175a5ca-99b1-43f5-9e0b-60570527993f","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002Ffde305bf-7b6e-5fed-a0fa-6452ce98822c","url":"","variantAllocation":null,"assets":undefined,"media":undefined}],"descendantAssets":[{"__typename":"Article","lastModified":"2025-02-28T11:37:15.494Z","uri":"nyt:\u002F\u002Farticle\u002F95773dee-1b8a-5f08-b78a-29ed1903e90c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-federal-bureaucracy-takeover.html"},{"__typename":"Article","lastModified":"2025-02-28T10:04:16.382Z","uri":"nyt:\u002F\u002Farticle\u002F89c13edf-2ccb-5223-91c7-a96b2e7b0998","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fpolitics\u002Fmusk-doge-timeline-takeaways.html"},{"__typename":"Article","lastModified":"2025-02-28T11:20:12.055Z","uri":"nyt:\u002F\u002Farticle\u002F8b200636-8df5-5caa-8daa-308efea287b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-headlines\u002Fiowa-trans-rights-zelensky-trump.html"},{"__typename":"LegacyCollection","lastModified":"2025-02-28T13:10:16.130Z","uri":"nyt:\u002F\u002Flegacycollection\u002F452b1d37-7b45-5f33-86f9-23ba2244d6b3","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F28\u002Fus\u002Ftrump-news"},{"__typename":"Article","lastModified":"2025-02-28T12:13:45.314Z","uri":"nyt:\u002F\u002Farticle\u002Fe5314031-2e3d-5d39-83d9-210e3f501e95","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Feurope\u002Ftrump-starmer-king-charles.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:43.670Z","uri":"nyt:\u002F\u002Farticle\u002F3ab13ed6-8bfc-561a-926e-13bc18df7495","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fstarmer-ukraine-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T11:36:27.694Z","uri":"nyt:\u002F\u002Farticle\u002Fb0b07911-d060-5a9a-a3e6-e0db0d473d84","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fpodcasts\u002Fthe-daily\u002Ftrump-minerals-ukraine-republican-budget.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:49.168Z","uri":"nyt:\u002F\u002Farticle\u002Ffe1a7c93-0a1a-5d69-9592-73c1b4faa68b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Feconomy\u002Ftrump-tariff-goals-contradictions.html"},{"__typename":"Article","lastModified":"2025-02-28T12:39:54.210Z","uri":"nyt:\u002F\u002Farticle\u002F40788135-75c9-5cdc-bbf8-f46ef5b6d02d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fdealbook\u002Finflation-trump-tariffs.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:43.134Z","uri":"nyt:\u002F\u002Farticle\u002F9a41ce77-0343-5ae2-81c8-56cb5cb081cb","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrump-federal-worker-layoffs.html"},{"__typename":"Article","lastModified":"2025-02-28T13:00:42.943Z","uri":"nyt:\u002F\u002Farticle\u002Fd32ab414-1972-50fe-8c0a-208302f972b0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ffederal-layoffs-trump-opm.html"},{"__typename":"EmbeddedInteractive","lastModified":"2025-01-22T20:54:52.365Z","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F0df9455a-1c52-5a54-9400-7443c43d9a68","url":"null"},{"__typename":"Article","lastModified":"2025-02-28T11:45:26.642Z","uri":"nyt:\u002F\u002Farticle\u002Fdcdfc485-364a-5a09-bb3d-79f645a6f129","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Fargentina-crypto-scandal-president.html"},{"__typename":"Article","lastModified":"2025-02-28T11:25:49.237Z","uri":"nyt:\u002F\u002Farticle\u002F85713c47-6ee2-52cc-84eb-02be4ce1ee1f","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fgene-hackman-death-new-mexico.html"},{"__typename":"Article","lastModified":"2025-02-28T12:44:37.741Z","uri":"nyt:\u002F\u002Farticle\u002Fcd0c66fd-7767-5885-b4d3-597efd65d1a0","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fus\u002Fgene-hackman-death-updates.html"},{"__typename":"Interactive","lastModified":"2025-02-28T00:40:02.920Z","uri":"nyt:\u002F\u002Finteractive\u002Fd41992cc-6b6c-544d-a568-309e7846c9c9","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fmovies\u002Fhackman-affidavit.html"},{"__typename":"Article","lastModified":"2025-02-28T02:12:14.299Z","uri":"nyt:\u002F\u002Farticle\u002Fcb0fd188-e910-5caf-b97f-9959fe27aad8","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fmovies\u002Fgene-hackman-unforgiven-bonnie-clyde.html"},{"__typename":"Article","lastModified":"2025-02-28T11:30:16.929Z","uri":"nyt:\u002F\u002Farticle\u002F720399f2-c1ec-5608-9963-cfaa03abd183","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fasia\u002Fchina-military-drills-pacific.html"},{"__typename":"Article","lastModified":"2025-02-28T10:33:26.206Z","uri":"nyt:\u002F\u002Farticle\u002Ff86860c9-bc29-598c-bc53-5b81b1def8a5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fwest-bank-jenin-palestinian-authority-israel.html"},{"__typename":"Article","lastModified":"2025-02-28T11:02:22.739Z","uri":"nyt:\u002F\u002Farticle\u002Feed2db6f-2fad-5bf8-b96f-b8ad776652ff","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fmiddleeast\u002Fsyria-ramadan-economy-cash-shortage.html"},{"__typename":"Article","lastModified":"2025-02-28T02:57:42.743Z","uri":"nyt:\u002F\u002Farticle\u002F2734f206-c837-5724-a971-94aedb7c0cc5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fiowa-transgender-civil-rights-bill.html"},{"__typename":"Article","lastModified":"2025-02-28T02:03:23.518Z","uri":"nyt:\u002F\u002Farticle\u002F2b8e7341-5edb-508e-81d3-079dc1d8f638","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Ftrans-troops-pentagon-figures.html"},{"__typename":"Interactive","lastModified":"2025-02-28T06:21:51.581Z","uri":"nyt:\u002F\u002Finteractive\u002F1e284d42-0f2f-5814-a3d3-ad7fe8a1f4a1","url":"https:\u002F\u002Fwww.nytimes.com\u002Finteractive\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmedicaid-enrollment.html"},{"__typename":"Article","lastModified":"2025-02-28T10:03:42.174Z","uri":"nyt:\u002F\u002Farticle\u002F61bfc8df-d91b-50a6-aecc-b9a82d7da8d9","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fmovies\u002Fbest-picture-nominees-oscars-conclave-wicked.html"},{"__typename":"Article","lastModified":"2025-02-28T10:01:46.736Z","uri":"nyt:\u002F\u002Farticle\u002Fdd9e6f3c-8c3c-5c1d-8b2c-aba404ce5cd6","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Fdance\u002Fzoe-saldana-emilia-perez-dance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:39:21.985Z","uri":"nyt:\u002F\u002Farticle\u002Fb0813f6c-840e-5ae7-a88c-d5d430d3c564","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Famericas\u002Femilia-perez-mexico-oscars.html"},{"__typename":"Article","lastModified":"2025-02-28T11:29:28.060Z","uri":"nyt:\u002F\u002Farticle\u002Feae58626-ae12-58cf-a801-1c30238d52ed","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fadams-corruption-new-charge.html"},{"__typename":"Article","lastModified":"2025-02-28T10:13:56.828Z","uri":"nyt:\u002F\u002Farticle\u002F2f9390e8-607b-51a0-b3ea-47be2f5821dc","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fandrew-cuomo-new-york-mayor.html"},{"__typename":"Article","lastModified":"2025-02-28T10:10:47.390Z","uri":"nyt:\u002F\u002Farticle\u002Ff04154fe-aaa0-5b58-bdd7-66b4439e06e5","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fbusiness\u002Fshopping-addiction-no-buy-2025.html"},{"__typename":"EmbeddedInteractive","lastModified":"2025-02-25T13:43:51.656Z","uri":"nyt:\u002F\u002Fembeddedinteractive\u002F557659e5-f688-5fe8-bdc0-ed9d03c1fa1e","url":"null"},{"__typename":"Article","lastModified":"2025-02-28T10:02:00.449Z","uri":"nyt:\u002F\u002Farticle\u002Ff08fbb32-8818-558e-8473-71631754acea","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fdonald-trump-birthright-citizenship.html"},{"__typename":"Article","lastModified":"2025-02-28T12:08:49.046Z","uri":"nyt:\u002F\u002Farticle\u002F96f9592e-54a6-5dea-845b-785346ba26b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fandrew-tate-donald-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:26.255Z","uri":"nyt:\u002F\u002Farticle\u002F896e3424-8c01-5485-bb02-1560953263ba","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Fgovernment-great-progressive-abundance.html"},{"__typename":"Article","lastModified":"2025-02-28T11:00:46.809Z","uri":"nyt:\u002F\u002Farticle\u002F5452cb92-2c7c-5c19-b4c5-863234fce6ab","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Felon-musk-south-africa.html"},{"__typename":"Article","lastModified":"2025-02-28T12:03:51.145Z","uri":"nyt:\u002F\u002Farticle\u002F0f55b9f2-4a1d-5e09-9275-da29f9bfbe1a","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ftexas-measles-vaccine.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:05.958Z","uri":"nyt:\u002F\u002Farticle\u002Ff716ba76-77d6-558b-9a94-bf1f5f1718b1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmedicaid-republicans.html"},{"__typename":"Article","lastModified":"2025-02-28T10:02:16.383Z","uri":"nyt:\u002F\u002Farticle\u002Fe4c4fcdc-2f50-5bca-83d7-8b88c9c4ec8c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fmatter-of-opinion-final-episode.html"},{"__typename":"Article","lastModified":"2025-02-28T01:29:38.344Z","uri":"nyt:\u002F\u002Farticle\u002Fa3f3b0d1-5608-54dd-a08b-83d185b5db46","url":"https:\u002F\u002Fwww.nytimes.com\u002Flive\u002F2025\u002F02\u002F25\u002Fopinion\u002Fthepoint\u002Fbarnard-college-sit-in-palestinian-israel-protest"},{"__typename":"Article","lastModified":"2025-02-28T12:12:33.018Z","uri":"nyt:\u002F\u002Farticle\u002Fe5fb45b5-1082-5fcc-ba9a-5bcc56a75975","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-hegseth-pentagon.html"},{"__typename":"Article","lastModified":"2025-02-28T02:33:52.611Z","uri":"nyt:\u002F\u002Farticle\u002F1b78bf9c-210e-5ae9-99a4-980a3696891d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fopinion\u002Ftrump-republicans-masculinity-gender-traditional.html"},{"__typename":"Article","lastModified":"2025-02-28T12:01:50.458Z","uri":"nyt:\u002F\u002Farticle\u002F351b30ec-12d0-5d59-9f63-5d81a895ded1","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Ffree-speech-trump-maga.html"},{"__typename":"Article","lastModified":"2025-02-28T10:00:12.600Z","uri":"nyt:\u002F\u002Farticle\u002F6717e682-229e-51b8-9947-2b735defd735","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fopinion\u002Fhorse-racing-government-subsidies.html"},{"__typename":"Article","lastModified":"2025-02-28T09:01:38.204Z","uri":"nyt:\u002F\u002Farticle\u002Ffe3aa929-085b-529c-9598-e16fc54c363b","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Farts\u002Ftelevision\u002Fstephen-colbert-trump-voters-remorse.html"},{"__typename":"Article","lastModified":"2025-02-28T02:14:56.238Z","uri":"nyt:\u002F\u002Farticle\u002F7cd260c2-752a-5a3c-9978-3e999d9b5dfa","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fus\u002Fpolitics\u002Fmexico-cartel-sheinbaum-trump.html"},{"__typename":"Article","lastModified":"2025-02-28T11:31:37.813Z","uri":"nyt:\u002F\u002Farticle\u002Fadab900a-3ca2-5afe-b1d4-477fc6bb909e","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fworld\u002Fcanada\u002Fcanada-us-trump-tariffs-reactions.html"},{"__typename":"Article","lastModified":"2025-02-28T04:54:29.304Z","uri":"nyt:\u002F\u002Farticle\u002Faff5b023-b154-5fd7-ac1a-bc207716b35c","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fbusiness\u002Fjeffrey-epstein-files-pam-bondi.html"},{"__typename":"Article","lastModified":"2025-02-28T12:49:57.620Z","uri":"nyt:\u002F\u002Farticle\u002Fd1966252-a51e-5c6f-8d72-f5b7f05eb56d","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F28\u002Fnyregion\u002Fcitigroup-81-trillion-error.html"},{"__typename":"Article","lastModified":"2025-02-27T14:31:36.919Z","uri":"nyt:\u002F\u002Farticle\u002F911b0155-1b65-5576-91a0-215b59fb3215","url":"https:\u002F\u002Fwww.nytimes.com\u002F2025\u002F02\u002F27\u002Fstyle\u002Fsharon-van-etten-album.html"},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.309Z","uri":"nyt:\u002F\u002Fpromo\u002Fc526680a-46b9-5c63-8313-c3aefb0c2df3","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.346Z","uri":"nyt:\u002F\u002Fpromo\u002F03b461e3-31bb-5fd0-be52-8fa3e27c8e6b","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.322Z","uri":"nyt:\u002F\u002Fpromo\u002Fb2a56bdd-40da-54e0-9c71-42cc74c71765","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.334Z","uri":"nyt:\u002F\u002Fpromo\u002F8788a6e9-0db1-5c7c-a8e8-d82c37ccfdd0","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.309Z","uri":"nyt:\u002F\u002Fpromo\u002Ff7f1225d-6bba-5864-ad80-682122a9fbbf","url":""},{"__typename":"Promo","lastModified":"2025-02-27T21:33:26.323Z","uri":"nyt:\u002F\u002Fpromo\u002Fc397d2d2-758a-5a3a-a897-2add6cf9ae78","url":""}],"eventId":"pubp:\u002F\u002Fevent\u002Faba4ec625d454405a49f2e76f5c90756","hierarchyType":"feed","sourceId":"https:\u002F\u002Fwww.nytimes.com\u002Froot-home-node-onewebview","trackingName":null,"uri":"nyt:\u002F\u002Fprogrammingnode\u002F1999c500-b740-5ba9-b2c1-57ff6b183315","url":"https:\u002F\u002Fwww.nytimes.com\u002Froot-home-node-onewebview","variantAllocation":null,"assets":undefined,"media":undefined}}},"initialState":{},"config":{"gqlUrlClient":"https:\u002F\u002Fsamizdat-graphql.nytimes.com\u002Fgraphql\u002Fv2","gqlRequestHeaders":{"nyt-app-type":"project-vi","nyt-app-version":"0.0.5","nyt-token":"MIIBIjANBgkqhkiG9w0BAQEFAAOCAQ8AMIIBCgKCAQEAs+\u002FoUCTBmD\u002FcLdmcecrnBMHiU\u002FpxQCn2DDyaPKUOXxi4p0uUSZQzsuq1pJ1m5z1i0YGPd1U1OeGHAChWtqoxC7bFMCXcwnE1oyui9G1uobgpm1GdhtwkR7ta7akVTcsF8zxiXx7DNXIPd2nIJFH83rmkZueKrC4JVaNzjvD+Z03piLn5bHWU6+w+rA+kyJtGgZNTXKyPh6EC6o5N+rknNMG5+CdTq35p8f99WjFawSvYgP9V64kgckbTbtdJ6YhVP58TnuYgr12urtwnIqWP9KSJ1e5vmgf3tunMqWNm6+AnsqNj8mCLdCuc5cEB74CwUeQcP2HQQmbCddBy2y0mEwIDAQAB","x-nyt-internal-meter-override":undefined,"x-nyt-programming-abtest":".ver=23710.000","x-nyt-targeting-dimensions-map":"newsTenure=anon_user,agent_id=uUPwe3rT6adYXJo-C9KZFi,regi_id"},"gqlFetchTimeout":1500,"disablePersistedQueries":false,"initialDeviceType":"desktop","fastlyAbraConfig":{".ver":"23577.000","AMS_FrictionCircumventionDesktop_cwv":"2_low-mid-truncation","AMS_FrictionCircumventionMobile_cwv":"2_low-mid-truncation","DFP_MediaNet_0123":"","HOME_cwv_chartbeat":"0_Control",".programming_ver":"23710.000"},"fastlyEntitlements":[],"platform":undefined,"internalPreviewConfig":{"meter":undefined,"swg":undefined},"webviewEnvironment":{"isInWebview":false,"isPreloaded":false},"isOptimisticallyTruncated":false,"optimisticTruncationDropzone":6,"requestPath":"\u002F","isProvisionallyLoggedIn":false,"routeName":"homepage","serviceWorkerFile":"service-worker-test-1740698352070.js","preloadedServiceWorkerFile":"\u002Fvi-assets\u002Fstatic-assets\u002Fpreloaded-service-worker-1740698539526.js"},"ssrQuery":{},"initialLocation":{"pathname":"\u002F","search":""}};</script>
<script>!function(){try{var e="undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:{},a=(new Error).stack;a&&(e._sentryDebugIds=e._sentryDebugIds||{},e._sentryDebugIds[a]="e5d7c438-eb76-4062-ad01-0f7493eb1b58",e._sentryDebugIdIdentifier="sentry-dbid-e5d7c438-eb76-4062-ad01-0f7493eb1b58")}catch(e){}}();var _global="undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:{};_global.SENTRY_RELEASE={id:"a33e3b3f2d9932550881a21bdebe3aaec6164b65"},function(e){function a(a){for(var c,r,s=a[0],t=a[1],b=a[2],i=0,l=[];i<s.length;i++)r=s[i],Object.prototype.hasOwnProperty.call(n,r)&&n[r]&&l.push(n[r][0]),n[r]=0;for(c in t)Object.prototype.hasOwnProperty.call(t,c)&&(e[c]=t[c]);for(f&&f(a);l.length;)l.shift()();return o.push.apply(o,b||[]),d()}function d(){for(var e,a=0;a<o.length;a++){for(var d=o[a],c=!0,s=1;s<d.length;s++){var t=d[s];0!==n[t]&&(c=!1)}c&&(o.splice(a--,1),e=r(r.s=d[0]))}return e}var c={},n={154:0},o=[];function r(a){if(c[a])return c[a].exports;var d=c[a]={i:a,l:!1,exports:{}};return e[a].call(d.exports,d,d.exports,r),d.l=!0,d.exports}r.e=function(e){var a=[],d=n[e];if(0!==d)if(d)a.push(d[2]);else{var c=new Promise((function(a,c){d=n[e]=[a,c]}));a.push(d[2]=c);var o,s=document.createElement("script");s.charset="utf-8",s.timeout=120,r.nc&&s.setAttribute("nonce",r.nc),s.src=function(e){return r.p+""+({0:"vendor",1:"vendors~accessCodeLPAllAccess~accessCodeLPAudio~accessCodeLPCooking~accessCodeLPGames~accessCodeLPHy~ae19e677",2:"vendors~account~byline~capsule~clientSideCapsule~collections~explainer~getstarted~liveAsset~livePane~32214921",4:"vendors~audio~bestsellers~card~collections~explainer~home~liveAsset~markets~paidpost~reviews~search~~b0abd9a2",5:"vendors~audio~byline~capsule~card~cardpanel~clientSideCapsule~collections~explainer~liveAsset~livePa~5a3d4357",6:"vendors~activateAudioLoginPage~allAccessLandingPage~athleticGiftLandingPage~audioLandingPage~brandLa~c146d5af",7:"vendors~activateAudioLoginPage~allAccessLandingPage~athleticGiftLandingPage~brandLandingPage~gamesGi~c8843a55",8:"vendors~audio~byline~capsule~carddeck~cardpanel~clientSideCapsule~home~livePanel~paidpost~trending~video",9:"vendors~accessCodeLPAllAccess~accessCodeLPAudio~accessCodeLPCooking~accessCodeLPGames~accessCodeLPHy~8802538a",10:"vendors~accessCodeLPAllAccess~accessCodeLPAudio~accessCodeLPCooking~accessCodeLPGames~accessCodeLPHy~bb6edae8",11:"vendors~accessCodeLPAllAccess~accessCodeLPAudio~accessCodeLPCooking~accessCodeLPGames~accessCodeLPHy~ed77dc00",12:"vendors~athleticGiftLandingPage~audioLandingPage~brandLandingPage~cookingLandingPage~gamesGiftLandin~3c1aa463",13:"vendors~getstarted~newsletter~newsletters~newsletterssubscriberonly~recirculation~welcomesubscriber~~a6f3c374",14:"bestsellers~markets~reviews~search~trending~your-list",15:"vendors~athleticLandingPage~audioLandingPage~cookingLandingPage~homeDeliveryLandingPage~hyundaiCardL~6dd8d2d8",16:"account~activateaccess~newslettersmanage~newslettersoptout~newslettersreengagement",17:"markets~reviews~timeswire~trending",18:"newsletter~newsletters~newsletterssubscriberonly~your-places-global-update",19:"vendors~CardDeck~carddeck~cardpanel~home",20:"giftArticles~subRecircBottomSheet~timeswire",21:"vendors~bestsellers~card~slideshow",22:"vendors~giftArticles~timeswire~your-list",25:"caHamburgerNestedNavData~nestedNav",26:"emailsignup~your-space",27:"internationalHamburgerNestedNavData",28:"nestedNav~usHamburgerNestedNavData",29:"vendors~account~newslettersoptout",30:"vendors~gamesOnboardingOfferLandingPage~newsOnboardingOfferLandingPage",31:"vendors~getstarted~welcomesubscriber",32:"vendors~groupsHigherEdLandingPage~groupsLandingPage",37:"CardDeck",38:"ChineseHanLogo",39:"DealbookLogo",40:"InsiderLogo",41:"NYTCommunitiesFundHeader",42:"Rio2016",43:"SportsFromTheAthleticLogo",44:"TMagazineLogo",45:"UpshotLogo",46:"WorldCupLogo2018",47:"accessCodeLPAllAccess",48:"accessCodeLPAudio",49:"accessCodeLPCooking",50:"accessCodeLPGames",51:"accessCodeLPHyundaicard",52:"accessCodeLPNews",53:"accessCodeLPNexo",54:"accessCodeLPTheAthletic",55:"accessCodeLPWirecutter",56:"account",57:"activateAudioLoginPage",58:"activateaccess",59:"additionalPlaylists",61:"allAccessLandingPage",62:"ask",63:"athleticGiftLandingPage",64:"athleticLandingPage",65:"audio",66:"audioApp",67:"audioLandingPage",68:"audioblock",69:"autoSave",70:"bestsellers",71:"blank",72:"book-review",73:"brandLandingPage",74:"byline",75:"caHamburgerNestedNavData",76:"canadaSiteIndexData",77:"canonicalUrlOverride",78:"capsule",79:"card",80:"carddeck",81:"carddeckadslot",82:"cardpanel",83:"clientSideCapsule",84:"collectionnewsletterform",85:"collections",86:"commentsForm",87:"commentsV2",88:"cookingAppDownloadLandingPage",89:"cookingLandingPage",90:"datasubjectrequest",91:"datasubjectrequestverification",92:"dealbook",93:"defaultSiteIndexData",94:"desktopLogoNav",95:"emailsignup",96:"episodefooter",97:"esHamburgerNestedNavData",98:"explainer",99:"explainerPostHeader",100:"explainerRecirculation",101:"fabActual",102:"featuredproperties",103:"foo",104:"gamesGiftLandingPage",105:"gamesLandingPage",106:"gamesOnboardingOfferLandingPage",107:"gatewayLandingPage",108:"getstarted",109:"giftArticles",110:"giftLandingPage",111:"groupsHigherEdLandingPage",112:"groupsLandingPage",113:"hamburgerDrawer",114:"headerfullbleedhorizontal",115:"headerfullbleedvertical",116:"headerlivebriefingvi",117:"home",118:"homeDeliveryLandingPage",119:"hyundaiCardLandingPage",120:"instacartLandingPage",122:"internationalSiteIndexData",123:"leMondeLandingPage",124:"lens",125:"liveAsset",126:"livePanel",127:"livePostHeader",128:"lottieJSON",130:"markets",131:"mortgagecalculator",132:"nestedNav",133:"newsAppLandingPage",134:"newsOnboardingOfferLandingPage",135:"newsletter",136:"newsletterRecirculation",137:"newsletters",138:"newslettersmanage",139:"newslettersoptout",140:"newslettersreengagement",141:"newsletterssubscriberonly",142:"onsiteMessagingExampleLP",143:"opinion",144:"paidpost",145:"privacy",146:"producernotes",147:"query-and-select",148:"recirculation",149:"related-coverage-chunk",150:"reviewheader",151:"reviews",155:"search",156:"siteIndexContent",157:"sitemap",158:"slideshow",159:"slideshowinline",160:"stickyfilljs",161:"story",162:"subRecircBottomSheet",163:"submittedComment",164:"subscribeWithGoogleLP",165:"surveywithdrawconsent",166:"tabActual",167:"the-athletic",168:"timeswire",169:"trending",170:"upshot",171:"usHamburgerNestedNavData",172:"vanity",174:"vendors~athleticLandingPage",175:"vendors~audioblock",176:"vendors~carddeck",177:"vendors~commentsForm",178:"vendors~cookingAppDownloadLandingPage",179:"vendors~emailsignup",180:"vendors~episodefooter",181:"vendors~explainerRecirculation",182:"vendors~giftArticles",183:"vendors~headerfullbleedhorizontal",184:"vendors~headerfullbleedvertical",185:"vendors~headerlivebriefingvi",186:"vendors~homeDeliveryLandingPage",187:"vendors~instacartLandingPage",188:"vendors~mortgagecalculator",189:"vendors~newsAppLandingPage",190:"vendors~producernotes",191:"vendors~recirculation",192:"vendors~reviewheader",193:"vendors~search",194:"vendors~slideshowinline",195:"vendors~subscribeWithGoogleLP",196:"vendors~viToolbar",197:"vendors~videoblock",198:"vendors~wellContent",199:"viToolbar",200:"video",201:"videoblock",202:"welcomeBannerGames",203:"welcomesubscriber",204:"wellContent",205:"wirecutterLandingPage",206:"world-cup-2019",207:"xpnMobileCooking",208:"xpnMobileWirecutter",209:"your-list",210:"your-places-global-update",211:"your-space"}[e]||e)+"-"+{0:"d219039692ab55462dfb",1:"47822365b65b4f1d8d05",2:"5dcb734a7d903abdc2c5",3:"fe0e45e07f2b6d8f62b3",4:"a46b15b690850d4c4bec",5:"b545d4fc76bdc1d9468c",6:"c85a4bee2c08684414a5",7:"eacfd2b5329bd4ebc92f",8:"a0c6dd032d669c9f2564",9:"4e7e4469218011b49504",10:"27ed8d477e86da0a8fa0",11:"2cd45a448a749cf5f2fa",12:"12d1d6da311314f8a766",13:"36d956112179e7fffe43",14:"3545f97dc25220d7705c",15:"750dafd55fc6e759f239",16:"e9a9761a2ecf3e50adb3",17:"7f49f11dab060206a06a",18:"4c8ca6af796a191df941",19:"5fdbdd8376b3b4221ad8",20:"ae0aa39d9379c1d58ee2",21:"ae0e67b552badcf6be5b",22:"d1ffb503b2d0f0e9494b",23:"775b6f79c1f4a1d2b893",24:"e422d27331df45ab1b7a",25:"3da65e046267008aa9f9",26:"bfa439d8b4d0ca561199",27:"a399dadba8921745daa6",28:"0b0e8d68fc5d51db387d",29:"5776546a844c24616ade",30:"b5569459f8464e8e3453",31:"46053312238edc061540",32:"0420561bd67d9209409e",33:"d0862160a7f843729dc9",34:"cb4115515edd39c84482",35:"4ae56d8255e49901f223",36:"af1d9e5b572ec95e54b9",37:"5a10e317d52d3b7838d3",38:"b4764d0211a6ad80f713",39:"e7e8015f51e85bd0de14",40:"83aa02c0d4c31bc64a51",41:"8de57d2c7f1fc331b2ce",42:"4ecc893f14204bdbf2ce",43:"800723ba756702e07977",44:"b6085f2e646a29e65aa7",45:"e6e8dda8cff330ea83c9",46:"3e7453780935f351725e",47:"bf643e33de3803b229a6",48:"14ffb21877060acab5d8",49:"78d79ed26b6e3c9a0ec0",50:"224a605e23945790adcd",51:"4e3fbd666fc2f627c6e2",52:"cc82b35f4da6b2be052b",53:"035e3a94f161a7f17f7f",54:"2b8e84dd4d0fc12016a8",55:"12595c095e58b1a5f5c7",56:"d078af178d9f3e688ed7",57:"67334add5394bd2bc1c9",58:"941aeedeb6628e54422b",59:"83ce31e97082cd560ad4",61:"9425394d387cfeb3607c",62:"ce6d6e0728746e33ba6b",63:"05db919635f236899166",64:"08700b6871d19c18643e",65:"41d2b21d374f0547c300",66:"df6f34e76408bbb7ec54",67:"85581a2181b27f261858",68:"b41b7f43d2955af08132",69:"9e96895511e6e447cb0a",70:"0346c8ce3978a2f71d9e",71:"45841bf3816c71515d37",72:"6a83c8a50852208b1fa5",73:"f027dd265840548354b3",74:"1dfd2ce9e449b57b1b0a",75:"c718e6fff8333c59bcfb",76:"1510004e601c02c678ee",77:"b94f2d02e27f6b032048",78:"4b85838289976b20051e",79:"2e20f32255f098ecfbe2",80:"e5b2f2f2d73aaca4b963",81:"de4dd9102e924533c5f0",82:"3a4199850d6b84ecb245",83:"65e8ce09a6f8d511923e",84:"3677da2fd53dc97deb1a",85:"0829ff01ed3864874d6b",86:"3b3070a2efef7cfdaf11",87:"37a527dc0657663609f5",88:"15af18ea6605c93a6512",89:"2e29294b55cea58f36d9",90:"9343642aeae83011fed2",91:"47f7b08fd57dc13902b1",92:"b7f20c3c2631d0715a82",93:"155980ae0b792bd33110",94:"e6f7e04a61e3dc0422ba",95:"e6afeac8ec86e51be512",96:"fd6bf5380eb86adfd978",97:"cf2fed2c00c9ccf6bccc",98:"c476d784aea28987f9b3",99:"2ef25a485bbc7e21f602",100:"46b12ab620ccc66fc5ea",101:"4b8559bdc796c1cb067c",102:"fd8ee22e9ee868c321a2",103:"106ba876e0725f8f89c2",104:"dd7df7aada9634c5f94b",105:"2765a946216e235a5473",106:"6fbdd7246eec2a009813",107:"a6059e8c55970d421fc6",108:"74fcdeef9ab9caef6c7e",109:"3bc31b3d449fe1af789c",110:"4d824248c05ab0cb5cbf",111:"92eed74da36bddf07df3",112:"3edc100307f3510314a2",113:"ff85920c2a6707666868",114:"26966603bf80747f62c3",115:"5313c1f13f05ee1d9bb8",116:"562717c5275f88e25ef1",117:"73c67ccfc9048ca765b3",118:"d4e7578296212f141db1",119:"e1b4aca1df3f26f065f2",120:"c7b51bed50c58f0b42bc",122:"7154378e933540a80ead",123:"99016e6b1a4e78171c74",124:"4e56282e982c40f245a3",125:"01a3be6105825bb0e19b",126:"6bfe8c67bfdaf6a28eff",127:"5ee4a7cf6f30974de8c0",128:"8ea2ad3fdd9c921c2e91",130:"055d1262129143e51c38",131:"7c6420272b3e73b9dd49",132:"c1c1ae8dcdf9efc0daec",133:"6e9f1449772b37f4301b",134:"9e2b949a321a4cff9854",135:"efe055c381d99009f158",136:"76840afb997d6eb4a61d",137:"c4db2b60409eebc0a174",138:"35ee01bd3e5d78bb64a5",139:"8490b475a57235db7636",140:"1a5bab1e093e4d94092d",141:"c47dc79061ba31539cf9",142:"dfed5f431e0afe61a7de",143:"56999d0ccaccbc10bd5f",144:"bf0d28549df4704c0f91",145:"c3f56b102202e429a35c",146:"bc8125569a3746d72507",147:"553826e4dc62592254ae",148:"48423ea4848a4cd730ab",149:"d4880c29990415727cdf",150:"55bc9f2859831db9c0d4",151:"f2fcdb00741568a7d412",155:"683a2581489930e2ea88",156:"507066a5102c9dcc247b",157:"91a9bf360ee141828442",158:"947a9bf317a6d05fdd78",159:"cb364c8f7593532b8676",160:"68d87ae6266b5d44bec3",161:"91c08dd385ffc6495a27",162:"c78ec45b1b354fdc3b6c",163:"1479c777557163bd826a",164:"43e47b37ad10e04b0b10",165:"29f4b4670fba4ba794bc",166:"7989f4c8681054e2fb33",167:"5cf68f6db2623ec33526",168:"e8cad9303225a68646f8",169:"3a4487867ee0636e2001",170:"088545b66b9ea9a46243",171:"f103b4f66d7507a4e900",172:"9561b312f62cde9257b3",174:"1a24234072b03c586095",175:"792f30d6ee846707efc4",176:"51bf7e5649ae551d2220",177:"c62e883e3d8f0a790e2f",178:"a9372ad50138806a7f64",179:"e7a8b586b75bd38bbfac",180:"bfbba3f628b87e0e175c",181:"091019c59f432a43e88f",182:"ab0a722f00470f491cef",183:"f60d24e12b70a65f0989",184:"0478b6afa4dd8e20180a",185:"96323f0593089c71c13f",186:"5088c5ac0508246653c2",187:"ecce2f407277a22f94aa",188:"484805055055739a2617",189:"4600da10cbe37b42d2c8",190:"976a26f765ff955a01cd",191:"67a2c00856dace71eedf",192:"9c69ec380b7da357585e",193:"c5b01e96d313ffbe0866",194:"0f3e2a7011fa886f09b5",195:"7a2bd510c6d82d41717e",196:"cb87d906f09ec36724f7",197:"236905e6d22e4234d62e",198:"ce284b5628db06c84cf6",199:"036ad156222f44c5f840",200:"c0e4d440217c3e30672c",201:"95b22f71a9e7858e9d32",202:"be4851309bf9a51e7067",203:"7aef9a451ba4e4f1a1e1",204:"610be42c810cbd71a063",205:"c72b52ee474e02fe80b2",206:"a1482ba0e4515436e426",207:"60f4c1c9157fb2b47aae",208:"3614c220f56bf5287524",209:"7dd662f653897eede655",210:"27efef1f6046b32eaee4",211:"fa60ea09e0ea94af2566",212:"1376f2425506358537f1",213:"c1e468185acfdcadc982",214:"81e2c106c2d5fa7a7084",215:"3e26ef3cd5bc503704d9",216:"70a81b2411b3365031a4",217:"ea10cbead8c54242dfc3",218:"ebcf6be06516d1af3756",219:"bcffdaeb3853cfb51b89",220:"c1a219af58e2b8bdabe5",221:"68bb726381faa0054e03",222:"22f0008566bdfb05b664",223:"1ee6c595356e16e5b574",224:"c6fd6ac7f4827c603dcb",225:"a76478517e960096b306",226:"bef81c0cecabbb950620",227:"b2c7254ffa133bd088f6",228:"cc39c368b672537bc345",229:"d7e78410d5d810d13414",230:"c8557f9d1a736f677e4d"}[e]+".js"}(e);var t=new Error;o=function(a){s.onerror=s.onload=null,clearTimeout(b);var d=n[e];if(0!==d){if(d){var c=a&&("load"===a.type?"missing":a.type),o=a&&a.target&&a.target.src;t.message="Loading chunk "+e+" failed.\n("+c+": "+o+")",t.name="ChunkLoadError",t.type=c,t.request=o,d[1](t)}n[e]=void 0}};var b=setTimeout((function(){o({type:"timeout",target:s})}),12e4);s.onerror=s.onload=o,document.head.appendChild(s)}return Promise.all(a)},r.m=e,r.c=c,r.d=function(e,a,d){r.o(e,a)||Object.defineProperty(e,a,{enumerable:!0,get:d})},r.r=function(e){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(e,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(e,"__esModule",{value:!0})},r.t=function(e,a){if(1&a&&(e=r(e)),8&a)return e;if(4&a&&"object"==typeof e&&e&&e.__esModule)return e;var d=Object.create(null);if(r.r(d),Object.defineProperty(d,"default",{enumerable:!0,value:e}),2&a&&"string"!=typeof e)for(var c in e)r.d(d,c,function(a){return e[a]}.bind(null,c));return d},r.n=function(e){var a=e&&e.__esModule?function(){return e.default}:function(){return e};return r.d(a,"a",a),a},r.o=function(e,a){return Object.prototype.hasOwnProperty.call(e,a)},r.p="/vi-assets/static-assets/",r.oe=function(e){throw console.error(e),e};var s=window.webpackJsonp=window.webpackJsonp||[],t=s.push.bind(s);s.push=a,s=s.slice();for(var b=0;b<s.length;b++)a(s[b]);var f=t;d()}([]);
//# sourceMappingURL=runtime~main-7441ad2570b92c13be17.js.map</script>
<script defer="" src="/vi-assets/static-assets/vendor-d219039692ab55462dfb.js"></script>
<script defer="" src="/vi-assets/static-assets/home-73c67ccfc9048ca765b3.js"></script>
<script defer="" src="/vi-assets/static-assets/desktopLogoNav-e6f7e04a61e3dc0422ba.js"></script>
<script defer="" src="/vi-assets/static-assets/nestedNav-c1c1ae8dcdf9efc0daec.js"></script>
<script defer="" src="/vi-assets/static-assets/main-c38519546ac2d543a71b.js"></script>
<script>(function () { var _f=function(){try{var e=["first-paint","first-contentful-paint","userBtnRender","appRenderTime"];new window.PerformanceObserver(function(r){for(var n=r.getEntries(),a=0;a<n.length;a+=1){var t=n[a];if(e.indexOf(t.name)>-1){var i={};i[t.name]=Math.round(t.duration||t.startTime),(window.dataLayer=window.dataLayer||[]).push({event:"performance",pageview:{performance:i}})}}}).observe({entryTypes:["mark","measure","paint"]})}catch(e){}};;_f.apply(null, []); })();(function () { var _f=function(){!function(){if(1===Math.floor(20*Math.random())&&(!window.BOOMR||!window.BOOMR.version&&!window.BOOMR.snippetExecuted)){window.BOOMR=window.BOOMR||{},window.BOOMR.snippetStart=(new Date).getTime(),window.BOOMR.snippetExecuted=!0,window.BOOMR.snippetVersion=14,window.BOOMR.url="https://s.go-mpulse.net/boomerang/ATH8A-MAMN8-XPXCH-N5KAX-8D239";var e=(document.currentScript||document.getElementsByTagName("script")[0]).parentNode,n=!1,t=document.createElement("link");t.relList&&"function"==typeof t.relList.supports&&t.relList.supports("preload")&&"as"in t?(window.BOOMR.snippetMethod="p",t.href=window.BOOMR.url,t.rel="preload",t.as="script",t.addEventListener("load",function(){if(!n){var t=document.createElement("script");t.id="boomr-scr-as",t.src=window.BOOMR.url,t.async=!0,e.appendChild(t),n=!0}}),t.addEventListener("error",function(){o(!0)}),setTimeout(function(){n||o(!0)},3e3),BOOMR_lstart=(new Date).getTime(),e.appendChild(t)):o(!1),window.addEventListener?window.addEventListener("load",i,!1):window.attachEvent&&window.attachEvent("onload",i)}function o(t){n=!0;var o,i,d,a,r=document,s=window;if(window.BOOMR.snippetMethod=t?"if":"i",i=function(e,n){var t=r.createElement("script");t.id=n||"boomr-if-as",t.src=window.BOOMR.url,BOOMR_lstart=(new Date).getTime(),(e=e||r.body).appendChild(t)},!window.addEventListener&&window.attachEvent&&navigator.userAgent.match(/MSIE [67]./))return window.BOOMR.snippetMethod="s",void i(e,"boomr-async");(d=document.createElement("IFRAME")).src="about:blank",d.title="",d.role="presentation",d.loading="eager",(a=(d.frameElement||d).style).width=0,a.height=0,a.border=0,a.display="none",e.appendChild(d);try{s=d.contentWindow,r=s.document.open()}catch(e){o=document.domain,d.src="javascript:var d=document.open();d.domain='"+o+"';void 0;",s=d.contentWindow,r=s.document.open()}o?(r._boomrl=function(){this.domain=o,i()},r.write("<body onload='document._boomrl();'>")):(s._boomrl=function(){i()},s.addEventListener?s.addEventListener("load",s._boomrl,!1):s.attachEvent&&s.attachEvent("onload",s._boomrl)),r.close()}function i(e){window.BOOMR_onload=e&&e.timeStamp||(new Date).getTime()}}()};;_f.apply(null, []); })();</script>
<script>
(function(w, l) {
w[l] = w[l] || [];
w[l].push({
'gtm.start': new Date().getTime(),
event: 'gtm.js'
});
})(window, 'dataLayer');
</script>
<script defer="" src="https://www.googletagmanager.com/gtm.js?id=GTM-P528B3>m_auth=tfAzqo1rYDLgYhmTnSjPqw>m_preview=env-130>m_cookies_win=x"></script>
<noscript>
<iframe height="0" src="https://www.googletagmanager.com/ns.html?id=GTM-P528B3>m_auth=tfAzqo1rYDLgYhmTnSjPqw>m_preview=env-130>m_cookies_win=x" style="display:none;visibility:hidden" width="0"></iframe>
</noscript>
<!-- RELEASE a33e3b3f2d9932550881a21bdebe3aaec6164b65 -->
<!-- hoisted interactive js-->
<link href="https://static01.nytimes.com/newsgraphics/weather-hp-strip/cb02c00a-e5f5-41fb-b9ab-85ea8382b647/_assets/_app/immutable/entry/start.6f861287.js" rel="modulepreload"/><link href="https://static01.nytimes.com/newsgraphics/weather-hp-strip/cb02c00a-e5f5-41fb-b9ab-85ea8382b647/_assets/_app/immutable/chunks/weather-hp-modules.28eb8722.js" rel="modulepreload"/><link href="https://static01.nytimes.com/newsgraphics/weather-hp-strip/cb02c00a-e5f5-41fb-b9ab-85ea8382b647/_assets/_app/immutable/entry/app.21f3107c.js" rel="modulepreload"/><link href="https://static01.nytimes.com/newsgraphics/weather-hp-strip/cb02c00a-e5f5-41fb-b9ab-85ea8382b647/_assets/_app/immutable/nodes/0.4900526e.js" rel="modulepreload"/><link href="https://static01.nytimes.com/newsgraphics/weather-hp-strip/cb02c00a-e5f5-41fb-b9ab-85ea8382b647/_assets/_app/immutable/nodes/3.7ab7a0dd.js" rel="modulepreload"/>
<!-- end hoisted interactive js-->
</body>
</html>
What is this mess? There is clearly useful information, like headlines, here, but it’s really hard to figure out how to scrape it. There doesn’t seem to be an inherent structure of the site that we can take advantage of, as we did in the last notebook. Instead, we are going to use Selenium
to render this site in a browser, so we can then use access the HTML output without dealing with the back-end of the site, which we don’t have access to.
Selenium
: what is it and how does it works#
Selenium
is a software package that automates an instance of a particular browser in the runtime of many programming languages, including Ruby, JavaScript and of course Python.
In order to use Selenium in any environment, one must first download the driver to a web browser (we’ll be using Chrome) and then also install the software package itself (we’ll be using pip).
I chose to put this notebook lesson on Colab because the Linux environment makes it very easy to download and install the dependencies needed to use Selenium
, but if you prefer to use this notebook locally, either run it in a WSL terminal (CHECK ON THIS) or follow the official documentation.
## installing all of the dependencies as well as the selenium package
%%shell
# Add debian buster
cat > /etc/apt/sources.list.d/debian.list <<'EOF'
deb [arch=amd64 signed-by=/usr/share/keyrings/debian-buster.gpg] http://deb.debian.org/debian buster main
deb [arch=amd64 signed-by=/usr/share/keyrings/debian-buster-updates.gpg] http://deb.debian.org/debian buster-updates main
deb [arch=amd64 signed-by=/usr/share/keyrings/debian-security-buster.gpg] http://deb.debian.org/debian-security buster/updates main
EOF
# Add keys
apt-key adv --keyserver keyserver.ubuntu.com --recv-keys DCC9EFBF77E11517
apt-key adv --keyserver keyserver.ubuntu.com --recv-keys 648ACFD622F3D138
apt-key adv --keyserver keyserver.ubuntu.com --recv-keys 112695A0E562B32A
apt-key export 77E11517 | gpg --dearmour -o /usr/share/keyrings/debian-buster.gpg
apt-key export 22F3D138 | gpg --dearmour -o /usr/share/keyrings/debian-buster-updates.gpg
apt-key export E562B32A | gpg --dearmour -o /usr/share/keyrings/debian-security-buster.gpg
# Prefer debian repo for chromium* packages only
# Note the double-blank lines between entries
cat > /etc/apt/preferences.d/chromium.pref << 'EOF'
Package: *
Pin: release a=eoan
Pin-Priority: 500
Package: *
Pin: origin "deb.debian.org"
Pin-Priority: 300
Package: chromium*
Pin: origin "deb.debian.org"
Pin-Priority: 700
EOF
# Install chromium and chromium-driver
apt-get update
apt-get install chromium chromium-driver
# Install selenium
pip install selenium
Cell In[3], line 13
apt-key adv --keyserver keyserver.ubuntu.com --recv-keys 648ACFD622F3D138
^
SyntaxError: invalid decimal literal
from selenium import webdriver # the main entry point for the selenium API, the webdriver
chrome_options = webdriver.ChromeOptions() # some useful features for the chrome driver
chrome_options.add_argument('--headless')
chrome_options.add_argument('--no-sandbox')
chrome_options.add_argument('--disable-dev-shm-usage')
wd = webdriver.Chrome(options=chrome_options) # final webdriver object
WARNING:selenium.webdriver.common.selenium_manager:The chromedriver version (90.0.4430.212) detected in PATH at /usr/bin/chromedriver might not be compatible with the detected chrome version (116.0.5845.96); currently, chromedriver 116.0.5845.96 is recommended for chrome 116.*, so it is advised to delete the driver in PATH and retry
---------------------------------------------------------------------------
SessionNotCreatedException Traceback (most recent call last)
<ipython-input-3-0dcea64e7d7c> in <cell line: 6>()
4 chrome_options.add_argument('--no-sandbox')
5 chrome_options.add_argument('--disable-dev-shm-usage')
----> 6 wd = webdriver.Chrome(options=chrome_options) # final webdriver object
/usr/local/lib/python3.10/dist-packages/selenium/webdriver/chrome/webdriver.py in __init__(self, options, service, keep_alive)
43 options = options if options else Options()
44
---> 45 super().__init__(
46 DesiredCapabilities.CHROME["browserName"],
47 "goog",
/usr/local/lib/python3.10/dist-packages/selenium/webdriver/chromium/webdriver.py in __init__(self, browser_name, vendor_prefix, options, service, keep_alive)
54
55 try:
---> 56 super().__init__(
57 command_executor=ChromiumRemoteConnection(
58 remote_server_addr=self.service.service_url,
/usr/local/lib/python3.10/dist-packages/selenium/webdriver/remote/webdriver.py in __init__(self, command_executor, keep_alive, file_detector, options)
204 self._authenticator_id = None
205 self.start_client()
--> 206 self.start_session(capabilities)
207
208 def __repr__(self):
/usr/local/lib/python3.10/dist-packages/selenium/webdriver/remote/webdriver.py in start_session(self, capabilities)
288
289 caps = _create_caps(capabilities)
--> 290 response = self.execute(Command.NEW_SESSION, caps)["value"]
291 self.session_id = response.get("sessionId")
292 self.caps = response.get("capabilities")
/usr/local/lib/python3.10/dist-packages/selenium/webdriver/remote/webdriver.py in execute(self, driver_command, params)
343 response = self.command_executor.execute(driver_command, params)
344 if response:
--> 345 self.error_handler.check_response(response)
346 response["value"] = self._unwrap_value(response.get("value", None))
347 return response
/usr/local/lib/python3.10/dist-packages/selenium/webdriver/remote/errorhandler.py in check_response(self, response)
227 alert_text = value["alert"].get("text")
228 raise exception_class(message, screen, stacktrace, alert_text) # type: ignore[call-arg] # mypy is not smart enough here
--> 229 raise exception_class(message, screen, stacktrace)
SessionNotCreatedException: Message: session not created: This version of ChromeDriver only supports Chrome version 90
Current browser version is 116.0.5845.96 with binary path /root/.cache/selenium/chrome/linux64/116.0.5845.96/chrome
Stacktrace:
#0 0x5d01b8e7e7f9 <unknown>
#1 0x5d01b8e1e3b3 <unknown>
#2 0x5d01b8b66016 <unknown>
#3 0x5d01b8b8ce4a <unknown>
#4 0x5d01b8b8899a <unknown>
#5 0x5d01b8b8589a <unknown>
#6 0x5d01b8bc300a <unknown>
#7 0x5d01b8bbdc93 <unknown>
#8 0x5d01b8b8fce4 <unknown>
#9 0x5d01b8b914d2 <unknown>
#10 0x5d01b8e4a542 <unknown>
#11 0x5d01b8e59ce7 <unknown>
#12 0x5d01b8e599e4 <unknown>
#13 0x5d01b8e5e13a <unknown>
#14 0x5d01b8e5a5b9 <unknown>
#15 0x5d01b8e3fe00 <unknown>
#16 0x5d01b8e715d2 <unknown>
#17 0x5d01b8e71778 <unknown>
#18 0x5d01b8e89a1f <unknown>
#19 0x79373e040b43 <unknown>
#20 0x79373e0d2a00 <unknown>
wd
Just like when we used requests
, we can use the get method to load the site into our runtime
wd.get('https://www.nytimes.com/')
If we want to get the headlines from each article, we need to select the section
tags. In BeautifulSoup
, we could use something like soup.find_all('section')
, but the syntax is slightly different in Selenium
.
Check out the documentation here.
[i.get_attribute('outerHTML') for i in wd.find_elements(By.XPATH, './/section//h3')]
## first, this By class allows us to select many options to search by
## here, we'll query by HTML tag
from selenium.webdriver.common.by import By
sections = wd.find_elements(By.TAG_NAME, 'section') # NOTE: I used find_elements with an 's', if you were to use find_element, it would only return the first element that meets the condiction, which can be useful
sections[6].find_element(By.TAG_NAME,'h3')#.get_attribute('outerHTML')
for section in sections:
if (len(section.text) > 0): # omitting all of the empty titles
print(type(section), section.text.replace('\n', ''), sep='\t')
else:
print(type(section), section.find_element(By.TAG_NAME,'h3'), sep='\t')
This is getting there, but there is still a lot of stuff in here that we don’t want. We could just remove the end of the list and hard code and indexing statement like list_of_tags[:idx_of_last_headline]
, but this is dangerous, as the New York Times changes at least everyday, sometimes multiple times a day, so we will use a more robust method of querying the webpage than just HTML tag.
Instead, we are going to select headlines by how they are styled on the webpage. Below, I have printed out all of the links of the site, which will have all of the headlines we want. Go through this list and determine which class attributes we want for our headlines.
a_tags = wd.find_elements(By.TAG_NAME, 'a')
for a in a_tags:
print(a.text, a.get_attribute("class"), sep='\t')
SKIP TO CONTENT css-kgn7zc
SKIP TO SITE INDEX css-kgn7zc
SKIP ADVERTISEMENT css-777zgl
css-nhjhh0 ell52qj1
css-ogiugu
css-ogiugu
css-ogiugu
css-ogiugu
css-ogiugu
nytcp-opt css-1kj7lfb
css-1kj7lfb
css-129gw94
css-hnzl8o
SUBSCRIBE FOR $1/WEEK nytcp-opt
css-1q2j1fr eoab3xr0
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-1wjnrbv
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
css-13phoew
Ukraine Seeks a Breakthrough in the South but Faces Big Obstacles
The goal of Ukraine’s counteroffensive is to drive a wedge through Russian-occupied territory. But the execution has proved difficult.
See more headlines 9+ css-9mylee
President Vladimir Putin promised free grain to at least six African countries at a summit, hoping to shore up Russia’s image. css-9mylee
css-777zgl
css-9mylee
css-9mylee
css-9mylee
css-1rsvbdu
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
us_heat_link svelte-mkrnf3
intl_heat_link svelte-mkrnf3
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-1rsvbdu
css-9mylee
css-9mylee
css-9mylee
game-holder svelte-5gtd79
game-holder svelte-5gtd79
game-holder svelte-5gtd79
game-holder svelte-5gtd79
game-holder svelte-5gtd79
game-holder svelte-5gtd79
game-holder svelte-5gtd79
game-holder svelte-5gtd79
css-9mylee
css-9mylee
css-9mylee
css-777zgl
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-rgq5s4
css-rgq5s4
css-9mylee
css-rgq5s4
css-rgq5s4
css-rgq5s4
css-9mylee
css-rgq5s4
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-777zgl
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-777zgl
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-777zgl
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-9mylee
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
css-jq1cx6
Terms of Sale css-sur6ab
Terms of Service css-sur6ab
Privacy Policy css-sur6ab
From this list, I found css-9mylee
and css-rgq5s4
as the two main headline classes. Now we can use XPATH filtering to isolate them.
## here, we are going to use the XPATH attribute of the By class
## XPATH is a common practice for XML parsing that can be extended to HTML using XHTML
## in order to process two XPATH patterns, we can make a list of them and feed them into
## the find_elements method one by one
patterns = [
"//a[@class='css-9mylee']",
"//a[@class='css-rgq5s4']"
]
for pattern in patterns:
hls = wd.find_elements(By.XPATH, pattern)
for hl in hls:
if (len(hl.text) > 0):
print(hl.text)
print('_____________________') ## print this here so we see where each a tag stops
Ukraine Seeks a Breakthrough in the South but Faces Big Obstacles
The goal of Ukraine’s counteroffensive is to drive a wedge through Russian-occupied territory. But the execution has proved difficult.
See more headlines 9+
_____________________
President Vladimir Putin promised free grain to at least six African countries at a summit, hoping to shore up Russia’s image.
_____________________
But, now there is stuff here, like the author name or how long a read the article is, that isn’t the headline and we still haven’t been able to scrape the link to the article itself.
Now, we want to look at the raw HTML at these place and try to deal with the problems above with BeautifulSoup parsing.
for pattern in patterns:
hls = wd.find_elements(By.XPATH, pattern)
for hl in hls:
if (len(hl.text) > 0):
print(hl.get_attribute('outerHTML')) ## this is the only line different from above
print('_____________________')
<a class="css-9mylee" href="https://www.nytimes.com/live/2023/07/27/world/russia-ukraine-news" data-uri="nyt://legacycollection/463190d1-6363-5d86-ba23-4d5060307987" aria-hidden="false"><div><div class="css-xdandi"><h3 class="indicate-hover css-vf1hbp">Ukraine Seeks a Breakthrough in the South but Faces Big Obstacles</h3></div><p class="summary-class css-dcsqcp">The goal of Ukraine’s counteroffensive is to drive a wedge through Russian-occupied territory. But the execution has proved difficult.</p></div><p class="css-ikai89">See more headlines <span role="link" aria-label="9 or more more headlines available" class="css-h6rqug">9+</span></p></a>
_____________________
<a class="css-9mylee" href="https://www.nytimes.com/2023/07/27/world/europe/putin-russia-africa-summit.html" data-uri="nyt://article/6458bd89-437b-5db4-bc22-cb1ed0f3b225" aria-hidden="false"><div><div class="css-xdandi"><h3 class="indicate-hover css-1gb49m4">President Vladimir Putin promised free grain to at least six African countries at a summit, hoping to shore up Russia’s image.</h3></div><p class="css-og0y9l">2 min read</p></div></a>
_____________________
Because we now have the raw HTML, we can call BeautifulSoup and sort out any issues that way.
We are going to use the href
value in the a tag to govern what type of headline we are going to look for in each a
tag.
First, we don’t want any of the games or podcast titles, so we can search the link to see if it has any keywords that would suggest it is one of these. Using the
in
operator and treating the link like any string, we can filter out the results we don’t wantOnce we have all of the headlines, we can again search the link to see if it is in the opinion section. If it is, then both the author’s name and the article’s name will be in a
h3
tag, and we can select which one we want accordingly.
Note that for both of these operations, I needed a familiarity with the webpage beyond just want it looks like in my browser. I needed to experiement with what worked and follow the HTML of the site as closely as I could.
from bs4 import BeautifulSoup
for pattern in patterns:
hls = wd.find_elements(By.XPATH, pattern)
for hl in hls:
if (len(hl.text) > 0):
soup = BeautifulSoup(hl.get_attribute('outerHTML'))
link = soup.find('a')['href']
if not isinstance(soup.find('h3'), type(None)): ## filtering out any none objects, that is links with no h3 tags
if ('tips' not in link) and ('puzzles' not in link) and ('crossword' not in link) and ('games' not in link) and ('podcasts' not in link) and ('briefing' not in link):
if ('opinion' in link):
headline = soup.find_all('h3')[1].text
print(link, headline)
else:
headline = soup.find('h3').text
print(link, headline)
https://www.nytimes.com/live/2023/07/27/world/russia-ukraine-news Ukraine Seeks a Breakthrough in the South but Faces Big Obstacles
https://www.nytimes.com/2023/07/27/world/europe/putin-russia-africa-summit.html President Vladimir Putin promised free grain to at least six African countries at a summit, hoping to shore up Russia’s image.
## now let's move this to a dataframe
import pandas as pd
headline_dict = {}
for pattern in patterns:
hls = wd.find_elements(By.XPATH, pattern)
for hl in hls:
if (len(hl.text) > 0):
soup = BeautifulSoup(hl.get_attribute('outerHTML'))
link = soup.find('a')['href']
if not isinstance(soup.find('h3'), type(None)):
if ('tips' not in link) and ('puzzles' not in link) and ('crossword' not in link) and ('games' not in link) and ('podcasts' not in link) and ('briefing' not in link) and ('theathletic' not in link):
if ('opinion' in link):
headline = soup.find_all('h3')[1].text
headline_dict[headline] = link
else:
headline = soup.find('h3').text
headline_dict[headline] = link
headline_df = pd.DataFrame.from_dict(headline_dict, orient='index').reset_index().rename(columns={'index':'headline', 0:'link'})
headline_df
headline | link | |
---|---|---|
0 | World Cup: Croatia vs. Japan | https://www.nytimes.com/live/2022/12/05/sports... |
1 | Russia-Ukraine War | https://www.nytimes.com/live/2022/12/05/world/... |
2 | Supreme Court Gay Rights Case | https://www.nytimes.com/live/2022/12/05/us/sup... |
3 | Supreme Court Hears Case Pitting Gay Rights Ag... | https://www.nytimes.com/live/2022/12/05/us/sup... |
4 | Blasts Reported at 2 Military Bases Deep Insid... | https://www.nytimes.com/live/2022/12/05/world/... |
5 | Advice for Europeans: Bundle Up and Get Ready ... | https://www.nytimes.com/2022/12/05/business/eu... |
6 | War and sanctions are threatening to thrust Ru... | https://www.nytimes.com/2022/12/05/world/europ... |
7 | DealBook: Energy traders pushed crude prices h... | https://www.nytimes.com/2022/12/05/business/de... |
8 | Covid Protests in China Raise Hope for Solidar... | https://www.nytimes.com/2022/12/05/world/asia/... |
9 | China has stemmed the wave of mass protests, b... | https://www.nytimes.com/2022/12/05/world/asia/... |
10 | Chinese expatriates in the U.S. are elated but... | https://www.nytimes.com/2022/12/05/nyregion/ne... |
11 | Croatia vs. Japan: Former Finalist Takes On th... | https://www.nytimes.com/live/2022/12/05/sports... |
12 | As the World Focuses on Soccer, a Women’s Team... | https://www.nytimes.com/2022/12/03/sports/socc... |
13 | Soccer may be a global game, but corner kicks ... | https://www.nytimes.com/interactive/2022/12/05... |
14 | Last Day of Campaigning Gets Underway in Georg... | https://www.nytimes.com/live/2022/12/04/us/war... |
15 | Senator Raphael Warnock’s time in Harlem as a ... | https://www.nytimes.com/2022/12/04/us/politics... |
16 | Former President Trump’s call for the “termina... | https://www.nytimes.com/2022/12/04/us/politics... |
17 | Twin Friends of Eric Adams Are Dogged by Alleg... | https://www.nytimes.com/2022/12/05/nyregion/er... |
18 | Few details were made public of New York Mayor... | https://www.nytimes.com/2022/12/04/nyregion/er... |
19 | The Crypto Industry Struggles for a Way Forward | https://www.nytimes.com/2022/12/05/technology/... |
20 | Iran Has Abolished Morality Police, an Officia... | https://www.nytimes.com/2022/12/04/world/middl... |
21 | Skyrocketing Prices in Turkey Hurt Families an... | https://www.nytimes.com/2022/12/05/world/europ... |
22 | The Best Theater of 2022 | https://www.nytimes.com/2022/12/05/theater/bes... |
23 | The Best Comedy of 2022 | https://www.nytimes.com/2022/12/05/arts/best-c... |
24 | One Dough, Six Cookies | https://www.nytimes.com/interactive/2022/12/04... |
25 | Here’s what we learned from Week 13 in the N.F.L. | https://www.nytimes.com/2022/12/04/sports/foot... |
26 | Giving a rescue animal as a gift is sweet, but... | https://www.nytimes.com/2022/12/05/style/rescu... |
27 | My Mother Has Two Sons: Me and a Squirrel | https://www.nytimes.com/2022/12/05/opinion/my-... |
28 | If You Want to Give Something Back to Nature, ... | https://www.nytimes.com/interactive/2022/12/05... |
29 | The Big Thing Effective Altruism (Still) Gets ... | https://www.nytimes.com/2022/12/04/opinion/cha... |
30 | How Wildlife Rescue Can Heal the Human Heart | https://www.nytimes.com/2022/12/05/opinion/wil... |
31 | What Euthanasia Has Done to Canada | https://www.nytimes.com/2022/12/03/opinion/can... |
32 | Read the Well Newsletter | https://www.nytimes.com/2022/12/01/well/holida... |
33 | Listen to the ‘Book Review’ Podcast | https://www.nytimes.com/2022/12/02/books/revie... |
34 | Plan Tests Tense Relationship Between N.Y.P.D.... | https://www.nytimes.com/2022/12/05/nyregion/me... |
35 | May ‘Bad Spaniels’ Mock Jack Daniel’s? The Sup... | https://www.nytimes.com/2022/12/05/us/politics... |
36 | Popular Pastor Returns After Absence Over an ‘... | https://www.nytimes.com/2022/12/04/us/matt-cha... |
37 | North Carolina Power Outages Caused by Gunfire... | https://www.nytimes.com/2022/12/04/us/power-ou... |
38 | Brussels Terrorist Attack Trial Opens, Revivin... | https://www.nytimes.com/2022/12/05/world/europ... |
39 | Mysterious Object Emerges on a Florida Beach, ... | https://www.nytimes.com/2022/12/05/us/mysterio... |
40 | Paul Pelosi Makes First Public Appearance Sinc... | https://www.nytimes.com/2022/12/05/arts/music/... |
41 | Bob McGrath, Longtime ‘Sesame Street’ Star, Di... | https://www.nytimes.com/2022/12/04/arts/televi... |
42 | Her Baby Needs Heart Surgery. But She Is Deman... | https://www.nytimes.com/2022/12/05/world/austr... |
43 | Sudan Military and Pro-Democracy Coalition Sig... | https://www.nytimes.com/2022/12/05/world/afric... |
44 | Courtroom Drama: New Legal Battle Over ‘To Kil... | https://www.nytimes.com/2022/12/02/theater/to-... |
45 | Review: ‘A Beautiful Noise’ | https://www.nytimes.com/2022/12/04/theater/a-b... |
46 | Review: The Met’s Grand Old ‘Aida’ | https://www.nytimes.com/2022/12/04/arts/music/... |
47 | Are Solar Panels a Good Investment? | https://www.nytimes.com/2022/11/26/realestate/... |
48 | The Many Layers of Lari Pittman | https://www.nytimes.com/2022/11/30/arts/design... |
49 | The Political Winds Are Blowing. And Blowing. ... | https://www.nytimes.com/2022/12/05/opinion/bid... |
50 | Everything Democrats Could Do if Warnock Wins | https://www.nytimes.com/2022/12/05/opinion/war... |
51 | The Supreme Court Is About to Ask the Wrong Qu... | https://www.nytimes.com/2022/12/05/opinion/303... |
52 | Biden Is Putting South Carolina First. I Won’t... | https://www.nytimes.com/2022/12/05/opinion/iow... |
53 | The Man Who Neutered Trump | https://www.nytimes.com/2022/12/04/opinion/bri... |
54 | Free to Be You and Me. Or Not. | https://www.nytimes.com/2022/12/04/opinion/fre... |
Now we have a really clean DataFrame
that we can use to then get the text from each of these articles.
# let's use our new Selenium skills to pull the dynamically generated text from the article
a_link = headline_df['link'].iloc[9]
# here, I am making a new webdriver object, we don't need to but it can be helpful if you have multiple
article_wd = webdriver.Chrome('chromedriver',options=chrome_options)
article_wd.get(a_link)
# the paragraphs are predictably held in p tags
for p in article_wd.find_elements(By.TAG_NAME, 'p'):
print(BeautifulSoup(p.get_attribute('outerHTML')).text)
Advertisement
Supported by
Students, residents, lawyers and workers are still challenging the country’s Covid-19 restrictions, even though the intensity of the political chants has been dialed back.
Send any friend a story
As a subscriber, you have 10 gift articles to give each month. Anyone can read what you share.
By Chang Che, Chris Buckley, Amy Chang Chien and Joy Dong
In central China, students chanted demands for more transparency about Covid rules, while avoiding the bold slogans that riled the Communist Party a week earlier. In Shanghai, residents successfully negotiated with the local authorities to stop a lockdown of their neighborhood. And despite pressure from officials, a team of volunteer lawyers across China, committed to defending the right of citizens to voice their views, fielded anxious calls from protesters.
The recent wave of demonstrations that washed over China was prompted by frustration about pandemic restrictions, but the unrest also sometimes resulted in calls for China’s leader, Xi Jinping, to resign. Since then, the police have been out in force to prevent a resurgence, and the mass protests have subsided. In the aftermath, a low-key hum of resistance against the authorities has persisted, suggesting that the big rallies emboldened a small but significant number of people, including students, professionals and blue-collar workers.
None of those local acts amount to a major challenge to Mr. Xi and the Communist Party. But they suggest that residents are less afraid of challenging officialdom, albeit in more measured, tactical ways. They often invoke China’s own laws and policy pledges, an approach that is less likely to draw the wrath of Communist Party leaders.
“There are people yelling out demands that are also my own, and I’m extremely grateful — grateful that they were able to speak out for me,” said Wang Shengsheng, a lawyer in Zhengzhou, central China. Ms. Wang helped compile a list of more than a dozen lawyers available to give free advice by phone to people in Shanghai and elsewhere worried about repercussions from taking part in vigils and protests.
Advertisement
“I’m sure that the number of people who expressed themselves this time, especially the youth, will later shape some policy changes,” she said. “I’m sure that the decision makers are not a monolithic lump of iron.”
In late November, dozens of protests broke out across China, ignited by fury over a deadly fire in Urumqi, capital of the Xinjiang region in the west. The result was the boldest and most widespread demonstrations in China since the pro-democracy movement of 1989.
The Urumqi government had firmly denied widespread rumors that the residents killed in the fire — 10 by the official count — had been trapped in their apartments by Covid restrictions. But many Chinese were unconvinced, and grief turned into wider anger at pervasive lockdowns, virus testing and limits on travel. At demonstrations in Shanghai, Beijing and other cities, some protesters called for Mr. Xi and the Communist Party to give up power.
Since then, the Chinese government has taken a two-pronged approach: detaining some protesters and warning would-be protesters, and letting local governments abandon some of the Covid rules that have frustrated the population. Mr. Xi has not spoken publicly about the protests, and it is unclear how far the displays of dissent played into his decision to adjust policy. But plenty of Chinese people seem to believe that the nationwide defiance played a big role. They may now try to keep up pressure in smaller ways.
Advertisement
“I think what’s going to happen is people will coordinate, it will be low-level, it will look individualized and spontaneous, but there will be learning and discussion behind the scenes,” said Mary Gallagher, a professor at the University of Michigan who studies politics and social change in China.
“That’s what you need to do in a politically repressive environment,” she said. “It’s really going to put pressure on the local governments not to lock down.”
Despite China’s hulking authoritarian government, local protests are not uncommon. Before Covid, they often focused on government land seizures, pollution outbreaks and unpaid wages. Since the pandemic, outbursts of discontent have continued. But this renewed pattern of local unrest will test Mr. Xi’s government at a particularly delicate time as China seeks to ease Covid restrictions while trying to avoid an uncontrolled surge of infections.
Hundreds of students at Wuhan University, in the city where the pandemic first took hold starting in late 2019, rallied on a recent rainy evening to call for changes to Covid policies, according to a video that has been verified by The New York Times. “An open process, transparent information,” they chanted while holding umbrellas over their heads.
That relatively mild slogan appeared to be a considered move. A student at the university said that classmates were unhappy about the university’s plans to restore in-person teaching, which had upset their plans to go home for a break after months of living under restrictions. The student, who asked to be identified only by his surname, Wu, fearing repercussions, said that he had not attended the rally but had seen videos shared by classmates. He noted that none of the protesters had held pieces of white paper, which have become a symbol of defiance of the government.
The school relented, allowing students to return home and choose between online and in-person classes, Mr. Wu said.
Advertisement
While some cities in China have begun to ease lockdown restrictions, not all local officials have followed suit. They remain under heavy pressure to contain outbreaks, even as more senior leaders want to appear sympathetic to public impatience.
In a wealthy district of Shanghai on Sunday afternoon, security teams blocked the entry to an apartment complex after a local committee ordered a lockdown upon discovering a Covid case in one building.
Angry residents soon confronted the guards, challenging the closure as unlawful. “You don’t have the right!” one woman is seen yelling repeatedly in a video posted on Twitter. Hours later, the police arrived and backed the residents. A neighborhood committee worker for the apartment complex told The New York Times that the lockdowns were lifted “after engagement and coordination.”
In Wuhan over the weekend, residents in one neighborhood took matters into their own hands, pouring into the street after breaking down barriers that had held them in lockdown, as seen in a video posted on Twitter.
With so much risk from taking part in protests, Chinese residents are using an older tactic: citing the central leaders’ words to push back against local officials. For centuries, disgruntled people have seized on central government edicts to make their case, often appealing to the idea — sincerely held or as a tactic — that a well-intentioned ruler in Beijing has been misled by corrupt or disloyal functionaries.
“It’s this idea that you can use the central government’s words against local overreach,” Professor Gallagher said. “And it protects you, because the central government is supposed to be benevolent.”
Advertisement
Chinese are invoking the law to negotiate and push back against persisting pandemic restrictions. In areas that have failed to ease lockdowns, residents have pointed to the government’s move in early November to push for local authorities to take a more targeted approach in controlling Covid.
The local confrontations in Shanghai and Wuhan point to the impatience of residents under lockdown who are more worried about paying mortgages, reviving battered businesses and getting children back to regular school.
“We want to lift the lockdown, our kids need to go to school,” residents of an apartment complex in Wuxi, eastern China, shouted as they resisted a lockdown of their complex, a video posted on Twitter showed. “We need to make money to feed our families. We want to eat.”
Members of the legal community have also stepped up to help raise residents’ awareness of their rights. As the authorities mobilized to detain protesters and search residents’ phones in recent days, often without clear justification, legal advice has circulated on the Chinese internet. One such article outlined citizens’ rights in the event that a police officer demands to search their phones.
In that article, the author, who belongs to a Shanghai law firm, invokes the Chinese Constitution and concludes: “Arbitrary content checks of citizens’ cellphones are a serious infringement of citizens’ privacy and an abuse of public power.”
Some of those who have been speaking out, however, continue to face greater pressures. Ms. Wang, 37, the lawyer who helped coordinate advice for worried protesters and their friends and families, said that she had received phone calls from local officials.
Advertisement
She said that she had decided to help the protesters and their families after seeing images circulate on Chinese social media of the vigil in Shanghai commemorating those killed in Urumqi. She had taken a couple of dozen calls, she said, including from people who had been detained and questioned and who wanted to know their rights.
The Chinese authorities have over the past decade tried to silence rights attorneys by revoking their law licenses or by detaining and imprisoning them. But Ms. Wang said that she felt no reason to worry.
“To my mind, I’m just providing a little bit of legal advice services” to people who took part in protests, she said.
“How is it that if some people believe that they were in the wrong, then I’m also in the wrong simply by providing them legal advice?” she added. “That’s fundamentally against the idea of rule of law.”
Advertisement
Support independent journalism.
Again, there’s some text here that we don’t want, mostly at the beginning and a couple throoughout.
for p in article_wd.find_elements(By.TAG_NAME, 'p'):
print(p.get_attribute('class'))
css-1n0orw4 e1wiw3jv0
css-6yj280
css-4m7ryc
css-4anu6l e1jsehar1
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
vhs-data
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
vhs-data
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
vhs-data
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-at9mc1 evys1bk0
css-i0n974
It seems that the css class css-at9mc1 evys1bk0
will select all of the text paragraphs, while leaving out the other information we don’t want.
paragraphs = []
for p in article_wd.find_elements(By.TAG_NAME, 'p'):
if p.get_attribute('class') == 'css-at9mc1 evys1bk0':
paragraphs.append(BeautifulSoup(p.get_attribute('outerHTML')).text)
paragraphs
['In central China, students chanted demands for more transparency about Covid rules, while avoiding the bold slogans that riled the Communist Party a week earlier. In Shanghai, residents successfully negotiated with the local authorities to stop a lockdown of their neighborhood. And despite pressure from officials, a team of volunteer lawyers across China, committed to defending the right of citizens to voice their views, fielded anxious calls from protesters.',
'The recent wave of demonstrations that washed over China was prompted by frustration about pandemic restrictions, but the unrest also sometimes resulted in calls for China’s leader, Xi Jinping, to resign. Since then, the police have been out in force to prevent a resurgence, and the mass protests have subsided. In the aftermath, a low-key hum of resistance against the authorities has persisted, suggesting that the big rallies emboldened a small but significant number of people, including students, professionals and blue-collar workers.',
'None of those local acts amount to a major challenge to Mr. Xi and the Communist Party. But they suggest that residents are less afraid of challenging officialdom, albeit in more measured, tactical ways. They often invoke China’s own laws and policy pledges, an approach that is less likely to draw the wrath of Communist Party leaders.',
'“There are people yelling out demands that are also my own, and I’m extremely grateful — grateful that they were able to speak out for me,” said Wang Shengsheng, a lawyer in Zhengzhou, central China. Ms. Wang helped compile a list of more than a dozen lawyers available to give free advice by phone to people in Shanghai and elsewhere worried about repercussions from taking part in vigils and protests.',
'“I’m sure that the number of people who expressed themselves this time, especially the youth, will later shape some policy changes,” she said. “I’m sure that the decision makers are not a monolithic lump of iron.”',
'In late November, dozens of protests broke out across China, ignited by fury over a deadly fire in Urumqi, capital of the Xinjiang region in the west. The result was the boldest and most widespread demonstrations in China since the pro-democracy movement of 1989.',
'The Urumqi government had firmly denied widespread rumors that the residents killed in the fire — 10 by the official count — had been trapped in their apartments by Covid restrictions. But many Chinese were unconvinced, and grief turned into wider anger at pervasive lockdowns, virus testing and limits on travel. At demonstrations in Shanghai, Beijing and other cities, some protesters called for Mr. Xi and the Communist Party to give up power.',
'Since then, the Chinese government has taken a two-pronged approach: detaining some protesters and warning would-be protesters, and letting local governments abandon some of the Covid rules that have frustrated the population. Mr. Xi has not spoken publicly about the protests, and it is unclear how far the displays of dissent played into his decision to adjust policy. But plenty of Chinese people seem to believe that the nationwide defiance played a big role. They may now try to keep up pressure in smaller ways.',
'“I think what’s going to happen is people will coordinate, it will be low-level, it will look individualized and spontaneous, but there will be learning and discussion behind the scenes,” said Mary Gallagher, a professor at the University of Michigan who studies politics and social change in China.',
'“That’s what you need to do in a politically repressive environment,” she said. “It’s really going to put pressure on the local governments not to lock down.”',
'Despite China’s hulking authoritarian government, local protests are not uncommon. Before Covid, they often focused on government land seizures, pollution outbreaks and unpaid wages. Since the pandemic, outbursts of discontent have continued. But this renewed pattern of local unrest will test Mr. Xi’s government at a particularly delicate time as China seeks to ease Covid restrictions while trying to avoid an uncontrolled surge of infections.',
'Hundreds of students at Wuhan University, in the city where the pandemic first took hold starting in late 2019, rallied on a recent rainy evening to call for changes to Covid policies, according to a video that has been verified by The New York Times. “An open process, transparent information,” they chanted while holding umbrellas over their heads.',
'That relatively mild slogan appeared to be a considered move. A student at the university said that classmates were unhappy about the university’s plans to restore in-person teaching, which had upset their plans to go home for a break after months of living under restrictions. The student, who asked to be identified only by his surname, Wu, fearing repercussions, said that he had not attended the rally but had seen videos shared by classmates. He noted that none of the protesters had held pieces of white paper, which have become a symbol of defiance of the government.',
'The school relented, allowing students to return home and choose between online and in-person classes, Mr. Wu said.',
'While some cities in China have begun to ease lockdown restrictions, not all local officials have followed suit. They remain under heavy pressure to contain outbreaks, even as more senior leaders want to appear sympathetic to public impatience.',
'In a wealthy district of Shanghai on Sunday afternoon, security teams blocked the entry to an apartment complex after a local committee ordered a lockdown upon discovering a Covid case in one building.',
'Angry residents soon confronted the guards, challenging the closure as unlawful. “You don’t have the right!” one woman is seen yelling repeatedly in a video posted on Twitter. Hours later, the police arrived and backed the residents. A neighborhood committee worker for the apartment complex told The New York Times that the lockdowns were lifted “after engagement and coordination.”',
'In Wuhan over the weekend, residents in one neighborhood took matters into their own hands, pouring into the street after breaking down barriers that had held them in lockdown, as seen in a video posted on Twitter.',
'With so much risk from taking part in protests, Chinese residents are using an older tactic: citing the central leaders’ words to push back against local officials. For centuries, disgruntled people have seized on central government edicts to make their case, often appealing to the idea — sincerely held or as a tactic — that a well-intentioned ruler in Beijing has been misled by corrupt or disloyal functionaries.',
'“It’s this idea that you can use the central government’s words against local overreach,” Professor Gallagher said. “And it protects you, because the central government is supposed to be benevolent.”',
'Chinese are invoking the law to negotiate and push back against persisting pandemic restrictions. In areas that have failed to ease lockdowns, residents have pointed to the government’s move in early November to push for local authorities to take a more targeted approach in controlling Covid.',
'The local confrontations in Shanghai and Wuhan point to the impatience of residents under lockdown who are more worried about paying mortgages, reviving battered businesses and getting children back to regular school.',
'“We want to lift the lockdown, our kids need to go to school,” residents of an apartment complex in Wuxi, eastern China, shouted as they resisted a lockdown of their complex, a video posted on Twitter showed. “We need to make money to feed our families. We want to eat.”',
'Members of the legal community have also stepped up to help raise residents’ awareness of their rights. As the authorities mobilized to detain protesters and search residents’ phones in recent days, often without clear justification, legal advice has circulated on the Chinese internet. One such article outlined citizens’ rights in the event that a police officer demands to search their phones.',
'In that article, the author, who belongs to a Shanghai law firm, invokes the Chinese Constitution and concludes: “Arbitrary content checks of citizens’ cellphones are a serious infringement of citizens’ privacy and an abuse of public power.”',
'Some of those who have been speaking out, however, continue to face greater pressures. Ms. Wang, 37, the lawyer who helped coordinate advice for worried protesters and their friends and families, said that she had received phone calls from local officials.',
'She said that she had decided to help the protesters and their families after seeing images circulate on Chinese social media of the vigil in Shanghai commemorating those killed in Urumqi. She had taken a couple of dozen calls, she said, including from people who had been detained and questioned and who wanted to know their rights.',
'The Chinese authorities have over the past decade tried to silence rights attorneys by revoking their law licenses or by detaining and imprisoning them. But Ms. Wang said that she felt no reason to worry.',
'“To my mind, I’m just providing a little bit of legal advice services” to people who took part in protests, she said.',
'“How is it that if some people believe that they were in the wrong, then I’m also in the wrong simply by providing them legal advice?” she added. “That’s fundamentally against the idea of rule of law.”']
headline_df
headline | link | |
---|---|---|
0 | World Cup: Croatia vs. Japan | https://www.nytimes.com/live/2022/12/05/sports... |
1 | Russia-Ukraine War | https://www.nytimes.com/live/2022/12/05/world/... |
2 | Supreme Court Gay Rights Case | https://www.nytimes.com/live/2022/12/05/us/sup... |
3 | Supreme Court Hears Case Pitting Gay Rights Ag... | https://www.nytimes.com/live/2022/12/05/us/sup... |
4 | Blasts Reported at 2 Military Bases Deep Insid... | https://www.nytimes.com/live/2022/12/05/world/... |
5 | Advice for Europeans: Bundle Up and Get Ready ... | https://www.nytimes.com/2022/12/05/business/eu... |
6 | War and sanctions are threatening to thrust Ru... | https://www.nytimes.com/2022/12/05/world/europ... |
7 | DealBook: Energy traders pushed crude prices h... | https://www.nytimes.com/2022/12/05/business/de... |
8 | Covid Protests in China Raise Hope for Solidar... | https://www.nytimes.com/2022/12/05/world/asia/... |
9 | China has stemmed the wave of mass protests, b... | https://www.nytimes.com/2022/12/05/world/asia/... |
10 | Chinese expatriates in the U.S. are elated but... | https://www.nytimes.com/2022/12/05/nyregion/ne... |
11 | Croatia vs. Japan: Former Finalist Takes On th... | https://www.nytimes.com/live/2022/12/05/sports... |
12 | As the World Focuses on Soccer, a Women’s Team... | https://www.nytimes.com/2022/12/03/sports/socc... |
13 | Soccer may be a global game, but corner kicks ... | https://www.nytimes.com/interactive/2022/12/05... |
14 | Last Day of Campaigning Gets Underway in Georg... | https://www.nytimes.com/live/2022/12/04/us/war... |
15 | Senator Raphael Warnock’s time in Harlem as a ... | https://www.nytimes.com/2022/12/04/us/politics... |
16 | Former President Trump’s call for the “termina... | https://www.nytimes.com/2022/12/04/us/politics... |
17 | Twin Friends of Eric Adams Are Dogged by Alleg... | https://www.nytimes.com/2022/12/05/nyregion/er... |
18 | Few details were made public of New York Mayor... | https://www.nytimes.com/2022/12/04/nyregion/er... |
19 | The Crypto Industry Struggles for a Way Forward | https://www.nytimes.com/2022/12/05/technology/... |
20 | Iran Has Abolished Morality Police, an Officia... | https://www.nytimes.com/2022/12/04/world/middl... |
21 | Skyrocketing Prices in Turkey Hurt Families an... | https://www.nytimes.com/2022/12/05/world/europ... |
22 | The Best Theater of 2022 | https://www.nytimes.com/2022/12/05/theater/bes... |
23 | The Best Comedy of 2022 | https://www.nytimes.com/2022/12/05/arts/best-c... |
24 | One Dough, Six Cookies | https://www.nytimes.com/interactive/2022/12/04... |
25 | Here’s what we learned from Week 13 in the N.F.L. | https://www.nytimes.com/2022/12/04/sports/foot... |
26 | Giving a rescue animal as a gift is sweet, but... | https://www.nytimes.com/2022/12/05/style/rescu... |
27 | My Mother Has Two Sons: Me and a Squirrel | https://www.nytimes.com/2022/12/05/opinion/my-... |
28 | If You Want to Give Something Back to Nature, ... | https://www.nytimes.com/interactive/2022/12/05... |
29 | The Big Thing Effective Altruism (Still) Gets ... | https://www.nytimes.com/2022/12/04/opinion/cha... |
30 | How Wildlife Rescue Can Heal the Human Heart | https://www.nytimes.com/2022/12/05/opinion/wil... |
31 | What Euthanasia Has Done to Canada | https://www.nytimes.com/2022/12/03/opinion/can... |
32 | Read the Well Newsletter | https://www.nytimes.com/2022/12/01/well/holida... |
33 | Listen to the ‘Book Review’ Podcast | https://www.nytimes.com/2022/12/02/books/revie... |
34 | Plan Tests Tense Relationship Between N.Y.P.D.... | https://www.nytimes.com/2022/12/05/nyregion/me... |
35 | May ‘Bad Spaniels’ Mock Jack Daniel’s? The Sup... | https://www.nytimes.com/2022/12/05/us/politics... |
36 | Popular Pastor Returns After Absence Over an ‘... | https://www.nytimes.com/2022/12/04/us/matt-cha... |
37 | North Carolina Power Outages Caused by Gunfire... | https://www.nytimes.com/2022/12/04/us/power-ou... |
38 | Brussels Terrorist Attack Trial Opens, Revivin... | https://www.nytimes.com/2022/12/05/world/europ... |
39 | Mysterious Object Emerges on a Florida Beach, ... | https://www.nytimes.com/2022/12/05/us/mysterio... |
40 | Paul Pelosi Makes First Public Appearance Sinc... | https://www.nytimes.com/2022/12/05/arts/music/... |
41 | Bob McGrath, Longtime ‘Sesame Street’ Star, Di... | https://www.nytimes.com/2022/12/04/arts/televi... |
42 | Her Baby Needs Heart Surgery. But She Is Deman... | https://www.nytimes.com/2022/12/05/world/austr... |
43 | Sudan Military and Pro-Democracy Coalition Sig... | https://www.nytimes.com/2022/12/05/world/afric... |
44 | Courtroom Drama: New Legal Battle Over ‘To Kil... | https://www.nytimes.com/2022/12/02/theater/to-... |
45 | Review: ‘A Beautiful Noise’ | https://www.nytimes.com/2022/12/04/theater/a-b... |
46 | Review: The Met’s Grand Old ‘Aida’ | https://www.nytimes.com/2022/12/04/arts/music/... |
47 | Are Solar Panels a Good Investment? | https://www.nytimes.com/2022/11/26/realestate/... |
48 | The Many Layers of Lari Pittman | https://www.nytimes.com/2022/11/30/arts/design... |
49 | The Political Winds Are Blowing. And Blowing. ... | https://www.nytimes.com/2022/12/05/opinion/bid... |
50 | Everything Democrats Could Do if Warnock Wins | https://www.nytimes.com/2022/12/05/opinion/war... |
51 | The Supreme Court Is About to Ask the Wrong Qu... | https://www.nytimes.com/2022/12/05/opinion/303... |
52 | Biden Is Putting South Carolina First. I Won’t... | https://www.nytimes.com/2022/12/05/opinion/iow... |
53 | The Man Who Neutered Trump | https://www.nytimes.com/2022/12/04/opinion/bri... |
54 | Free to Be You and Me. Or Not. | https://www.nytimes.com/2022/12/04/opinion/fre... |
# now let's formalize this into a function and call it on the whole link column using apply
# this cell will take 10-15 minutes to run as the article_wd.get line will take some time to process for each article
import time
from tqdm import tqdm
tqdm.pandas()
def getParagraphs(link):
paragraphs = []
article_wd = webdriver.Chrome('chromedriver',options=chrome_options)
time.sleep(2)
article_wd.get(link)
for p in article_wd.find_elements(By.TAG_NAME, 'p'):
if p.get_attribute('class') == 'css-at9mc1 evys1bk0':
paragraphs.append(BeautifulSoup(p.get_attribute('outerHTML')).text)
return ' '.join(paragraphs).strip() # this line takes the list of paragraphs and converts them into a single string
headline_df['article_text'] = headline_df['link'].progress_apply(getParagraphs)
100%|██████████| 55/55 [15:43<00:00, 17.15s/it]
headline_df
headline | link | article_text | |
---|---|---|---|
0 | World Cup: Croatia vs. Japan | https://www.nytimes.com/live/2022/12/05/sports... | |
1 | Russia-Ukraine War | https://www.nytimes.com/live/2022/12/05/world/... | |
2 | Supreme Court Gay Rights Case | https://www.nytimes.com/live/2022/12/05/us/sup... | |
3 | Supreme Court Hears Case Pitting Gay Rights Ag... | https://www.nytimes.com/live/2022/12/05/us/sup... | |
4 | Blasts Reported at 2 Military Bases Deep Insid... | https://www.nytimes.com/live/2022/12/05/world/... | |
5 | Advice for Europeans: Bundle Up and Get Ready ... | https://www.nytimes.com/2022/12/05/business/eu... | Life in some European cities may soon look lik... |
6 | War and sanctions are threatening to thrust Ru... | https://www.nytimes.com/2022/12/05/world/europ... | KALUGA, Russia— Valery Volodin, a welder at a ... |
7 | DealBook: Energy traders pushed crude prices h... | https://www.nytimes.com/2022/12/05/business/de... | Crude oil prices rose this morning after a whi... |
8 | Covid Protests in China Raise Hope for Solidar... | https://www.nytimes.com/2022/12/05/world/asia/... | Three years ago in Melbourne, Australia, Ronni... |
9 | China has stemmed the wave of mass protests, b... | https://www.nytimes.com/2022/12/05/world/asia/... | In central China, students chanted demands for... |
10 | Chinese expatriates in the U.S. are elated but... | https://www.nytimes.com/2022/12/05/nyregion/ne... | Huanjie Li, 26, has never been more worried ab... |
11 | Croatia vs. Japan: Former Finalist Takes On th... | https://www.nytimes.com/live/2022/12/05/sports... | |
12 | As the World Focuses on Soccer, a Women’s Team... | https://www.nytimes.com/2022/12/03/sports/socc... | When the Afghanistan women’s national soccer t... |
13 | Soccer may be a global game, but corner kicks ... | https://www.nytimes.com/interactive/2022/12/05... | |
14 | Last Day of Campaigning Gets Underway in Georg... | https://www.nytimes.com/live/2022/12/04/us/war... | |
15 | Senator Raphael Warnock’s time in Harlem as a ... | https://www.nytimes.com/2022/12/04/us/politics... | Four days before the November midterm election... |
16 | Former President Trump’s call for the “termina... | https://www.nytimes.com/2022/12/04/us/politics... | An extraordinary antidemocratic statement from... |
17 | Twin Friends of Eric Adams Are Dogged by Alleg... | https://www.nytimes.com/2022/12/05/nyregion/er... | Vadim Shubaderov, a 35-year-old businessman, t... |
18 | Few details were made public of New York Mayor... | https://www.nytimes.com/2022/12/04/nyregion/er... | He met with heads of state. He visited the Acr... |
19 | The Crypto Industry Struggles for a Way Forward | https://www.nytimes.com/2022/12/05/technology/... | Not long after several Wall Street banks colla... |
20 | Iran Has Abolished Morality Police, an Officia... | https://www.nytimes.com/2022/12/04/world/middl... | A senior Iranian official said this weekend th... |
21 | Skyrocketing Prices in Turkey Hurt Families an... | https://www.nytimes.com/2022/12/05/world/europ... | ISTANBUL — As Turkey’s annual inflation rate h... |
22 | The Best Theater of 2022 | https://www.nytimes.com/2022/12/05/theater/bes... | Musicals are exceedingly difficult; they not o... |
23 | The Best Comedy of 2022 | https://www.nytimes.com/2022/12/05/arts/best-c... | This year was a “best of times, worst of times... |
24 | One Dough, Six Cookies | https://www.nytimes.com/interactive/2022/12/04... | |
25 | Here’s what we learned from Week 13 in the N.F.L. | https://www.nytimes.com/2022/12/04/sports/foot... | The Bengals completed a rare trifecta — three ... |
26 | Giving a rescue animal as a gift is sweet, but... | https://www.nytimes.com/2022/12/05/style/rescu... | You know the scene: A child ambles downstairs ... |
27 | My Mother Has Two Sons: Me and a Squirrel | https://www.nytimes.com/2022/12/05/opinion/my-... | During the summer of 2020, I returned home to ... |
28 | If You Want to Give Something Back to Nature, ... | https://www.nytimes.com/interactive/2022/12/05... | |
29 | The Big Thing Effective Altruism (Still) Gets ... | https://www.nytimes.com/2022/12/04/opinion/cha... | This article is part of Times Opinion’s Holida... |
30 | How Wildlife Rescue Can Heal the Human Heart | https://www.nytimes.com/2022/12/05/opinion/wil... | NASHVILLE — As a young college student, I work... |
31 | What Euthanasia Has Done to Canada | https://www.nytimes.com/2022/12/03/opinion/can... | La Maison Simons, commonly known as Simons, is... |
32 | Read the Well Newsletter | https://www.nytimes.com/2022/12/01/well/holida... | Next week, Well’s new columnist, Jancee Dunn, ... |
33 | Listen to the ‘Book Review’ Podcast | https://www.nytimes.com/2022/12/02/books/revie... | Heads up! The Book Review podcast returns with... |
34 | Plan Tests Tense Relationship Between N.Y.P.D.... | https://www.nytimes.com/2022/12/05/nyregion/me... | Each day, New York City’s 911 system is inunda... |
35 | May ‘Bad Spaniels’ Mock Jack Daniel’s? The Sup... | https://www.nytimes.com/2022/12/05/us/politics... | WASHINGTON — The next frontier in First Amendm... |
36 | Popular Pastor Returns After Absence Over an ‘... | https://www.nytimes.com/2022/12/04/us/matt-cha... | FLOWER MOUND, Texas — The prominent pastor of ... |
37 | North Carolina Power Outages Caused by Gunfire... | https://www.nytimes.com/2022/12/04/us/power-ou... | A county in central North Carolina where about... |
38 | Brussels Terrorist Attack Trial Opens, Revivin... | https://www.nytimes.com/2022/12/05/world/europ... | BRUSSELS — The mammoth trial against 10 men ac... |
39 | Mysterious Object Emerges on a Florida Beach, ... | https://www.nytimes.com/2022/12/05/us/mysterio... | There’s something protruding through the sand ... |
40 | Paul Pelosi Makes First Public Appearance Sinc... | https://www.nytimes.com/2022/12/05/arts/music/... | WASHINGTON — It is not easy to upstage the sta... |
41 | Bob McGrath, Longtime ‘Sesame Street’ Star, Di... | https://www.nytimes.com/2022/12/04/arts/televi... | Bob McGrath, who played the sweater-clad neigh... |
42 | Her Baby Needs Heart Surgery. But She Is Deman... | https://www.nytimes.com/2022/12/05/world/austr... | A New Zealand couple is refusing to allow thei... |
43 | Sudan Military and Pro-Democracy Coalition Sig... | https://www.nytimes.com/2022/12/05/world/afric... | NAIROBI, Kenya — Sudan’s military and a coalit... |
44 | Courtroom Drama: New Legal Battle Over ‘To Kil... | https://www.nytimes.com/2022/12/02/theater/to-... | In 2019, the producers of a Broadway adaptatio... |
45 | Review: ‘A Beautiful Noise’ | https://www.nytimes.com/2022/12/04/theater/a-b... | For decades, Neil Diamond was on top of the wo... |
46 | Review: The Met’s Grand Old ‘Aida’ | https://www.nytimes.com/2022/12/04/arts/music/... | “I’m not dead!” a decrepit old man croaks as h... |
47 | Are Solar Panels a Good Investment? | https://www.nytimes.com/2022/11/26/realestate/... | Q: We were considering installing solar panels... |
48 | The Many Layers of Lari Pittman | https://www.nytimes.com/2022/11/30/arts/design... | Lari Pittman’s career as a painter has been as... |
49 | The Political Winds Are Blowing. And Blowing. ... | https://www.nytimes.com/2022/12/05/opinion/bid... | Gail Collins: Bret, I think we’ve got good fig... |
50 | Everything Democrats Could Do if Warnock Wins | https://www.nytimes.com/2022/12/05/opinion/war... | Nearly two years ago, Raphael Warnock and Jon ... |
51 | The Supreme Court Is About to Ask the Wrong Qu... | https://www.nytimes.com/2022/12/05/opinion/303... | Can an artist be compelled to create a website... |
52 | Biden Is Putting South Carolina First. I Won’t... | https://www.nytimes.com/2022/12/05/opinion/iow... | President Biden’s three-part plan to reform th... |
53 | The Man Who Neutered Trump | https://www.nytimes.com/2022/12/04/opinion/bri... | It’s not surprising that Gov. Brian Kemp of Ge... |
54 | Free to Be You and Me. Or Not. | https://www.nytimes.com/2022/12/04/opinion/fre... | If you grew up in any remotely liberal enclave... |
Application#
Let’s see what we can do with this scraped data. This is just a test so that you can see what type of things you can now do with this data in this form. It will be a recap of what it is in the Gentle Introduction to NLP
workshop.
import nltk
nltk.download('punkt')
nltk.download('stopwords')
# sentence-level tokenization
headline_df['sents'] = headline_df['article_text'].apply(nltk.sent_tokenize)
headline_sents = headline_df.explode('sents').drop(['link','article_text'],axis=1).reset_index(drop=True).dropna()
# word-level tokenization
headline_sents['words'] = headline_sents['sents'].apply(nltk.word_tokenize)
[nltk_data] Downloading package punkt to /root/nltk_data...
[nltk_data] Package punkt is already up-to-date!
[nltk_data] Downloading package stopwords to /root/nltk_data...
[nltk_data] Package stopwords is already up-to-date!
# stopwords are very common words that we don't generally care about in statisical analysis
# sometimes, though, you may care about them...
# this stops object is a normal Python set, so you can change it accordingly
from nltk.corpus import stopwords
stops = list(stopwords.words('english'))
# i'll add some that are relevent here
stops.append('said') # very common in journalism
stops.append('would') # these two don't come pre-loaded though they should...
stops.append('could')
print(stops)
['i', 'me', 'my', 'myself', 'we', 'our', 'ours', 'ourselves', 'you', "you're", "you've", "you'll", "you'd", 'your', 'yours', 'yourself', 'yourselves', 'he', 'him', 'his', 'himself', 'she', "she's", 'her', 'hers', 'herself', 'it', "it's", 'its', 'itself', 'they', 'them', 'their', 'theirs', 'themselves', 'what', 'which', 'who', 'whom', 'this', 'that', "that'll", 'these', 'those', 'am', 'is', 'are', 'was', 'were', 'be', 'been', 'being', 'have', 'has', 'had', 'having', 'do', 'does', 'did', 'doing', 'a', 'an', 'the', 'and', 'but', 'if', 'or', 'because', 'as', 'until', 'while', 'of', 'at', 'by', 'for', 'with', 'about', 'against', 'between', 'into', 'through', 'during', 'before', 'after', 'above', 'below', 'to', 'from', 'up', 'down', 'in', 'out', 'on', 'off', 'over', 'under', 'again', 'further', 'then', 'once', 'here', 'there', 'when', 'where', 'why', 'how', 'all', 'any', 'both', 'each', 'few', 'more', 'most', 'other', 'some', 'such', 'no', 'nor', 'not', 'only', 'own', 'same', 'so', 'than', 'too', 'very', 's', 't', 'can', 'will', 'just', 'don', "don't", 'should', "should've", 'now', 'd', 'll', 'm', 'o', 're', 've', 'y', 'ain', 'aren', "aren't", 'couldn', "couldn't", 'didn', "didn't", 'doesn', "doesn't", 'hadn', "hadn't", 'hasn', "hasn't", 'haven', "haven't", 'isn', "isn't", 'ma', 'mightn', "mightn't", 'mustn', "mustn't", 'needn', "needn't", 'shan', "shan't", 'shouldn', "shouldn't", 'wasn', "wasn't", 'weren', "weren't", 'won', "won't", 'wouldn', "wouldn't", 'said', 'would', 'could']
# now let's add all of the punctuation to the stops list
import string
for punct in string.punctuation:
stops.append(punct)
# nyt uses these special characters
stops.append('’')
stops.append('“')
stops.append('”')
stops.append('—')
# simpliest way to do word frequency... count the words!
import collections
word_count = collections.defaultdict(int) # creates a dictionary that will increment its value everytime it sees the same key (in this case, every word used multiple times)
words = [word.lower() for sent in headline_sents.words.to_list() for word in sent] # all words taken from the DataFrame in a single list
for word in words:
if word not in stops:
word_count[word] += 1
count_df = pd.DataFrame.from_dict(dict(sorted(word_count.items(), key=lambda item: item[1], reverse=True)), orient='index').reset_index().rename(columns={'index':'word',0:'count'})
count_df
word | count | |
---|---|---|
0 | mr. | 358 |
1 | new | 147 |
2 | people | 138 |
3 | one | 134 |
4 | also | 87 |
... | ... | ... |
9286 | npr | 1 |
9287 | subsequent | 1 |
9288 | dated. | 1 |
9289 | spotify | 1 |
9290 | winding | 1 |
9291 rows × 2 columns
# let's look at the top 50 words by frequency
count_df[:50].plot.barh(x='word',y='count', figsize=(15,10))
<matplotlib.axes._subplots.AxesSubplot at 0x7f68c59e4e50>
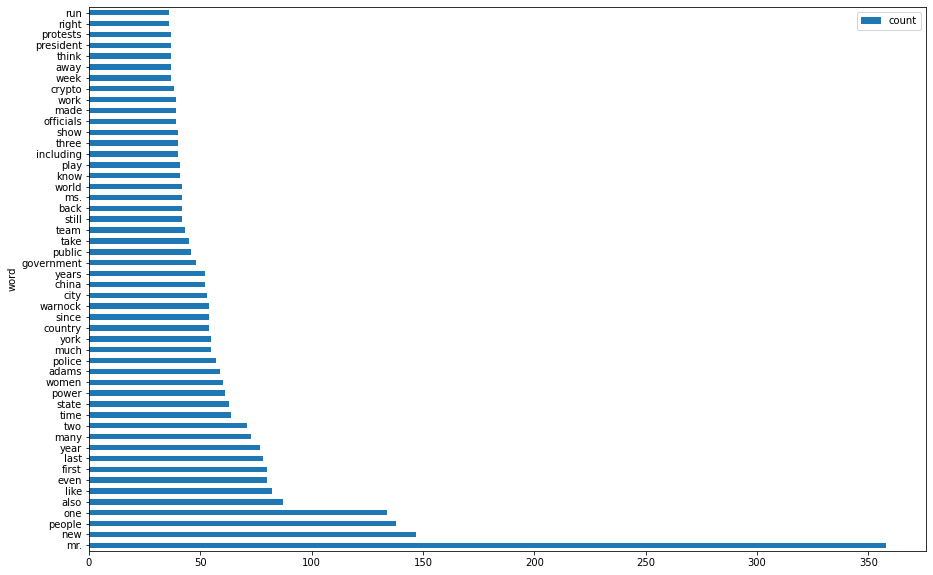
Reviewing what we learned#
The difference between dynamic and static websites
How to install
Selenium
in ColabHow to make a
get
request inSelenium
Navigating markup (HTML) in
Selenium
Using
Selenium
andBeautifulSoup
together to extract textPulling text and populating a
DataFrame
Plotting word frequency, excluding stopwards and punctuation
As a challenge, try scraping the New York Times archive. In Selenium
, though we didn’t cover it here, you can input queries into search bars and navigate through dynamically generated results. Read more about it here.
Once you have a DataFrame
, try doing what we did above and calculate word frequency, but not for a given day’s articles, but rather for a particular search. You could even package all of it into a single function whose only input is a search string. That way, we could visualize the most important subtopics for any given topic in the history of the Times. If you give that a try and get confused or want to show off what you did, feel free to reach out to me at peter.nadel@tufts.edu